In my previous article, I launched pure language processing (NLP) and the Natural Language Toolkit (NLTK), the NLP toolkit created on the University of Pennsylvania. I demonstrated how one can parse textual content and outline stopwords in Python and launched the idea of a corpus, a dataset of textual content that aids in textual content processing with out-of-the-box knowledge. In this text, I am going to proceed using datasets to match and analyze pure language.
The elementary constructing blocks lined on this article are:
- WordNet and synsets
- Similarity comparability
- Tree and treebank
- Named entity recognition
WordNet and synsets
WordNet is a big lexical database corpus in NLTK. WordNet maintains cognitive synonyms (generally known as synsets) of phrases correlated by nouns, verbs, adjectives, adverbs, synonyms, antonyms, and extra.
WordNet is a really useful gizmo for textual content evaluation. It is out there for a lot of languages (Chinese, English, Japanese, Russian, Spanish, and extra), below many licenses (starting from open supply to business). The first WordNet was created by Princeton University for English below an MIT-like license.
A phrase is usually related to a number of synsets based mostly on its meanings and components of speech. Each synset often gives these attributes:
Attribute | Definition | Example |
---|---|---|
Name | Name of the synset | Example: The phrase “code” has 5 synsets with names code.n.01 , code.n.02 , code.n.03 , code.v.01 , code.v.02 |
POS | Part of speech of the phrase for this synset | The phrase “code” has three synsets in noun type and two in verb type |
Definition | Definition of the phrase (in POS) | One of the definitions of “code” in verb type is: “(computer science) the symbolic arrangement of data or instructions in a computer program” |
Examples | Examples of phrase’s use | One of the examples of “code”: “We should encode the message for security reasons” |
Lemmas | Other phrase synsets this phrase+POC is expounded to (not strictly synonyms, however could be thought of so); lemmas are associated to different lemmas, to not phrases immediately | Lemmas of code.v.02 (as in “convert ordinary language into code”) are code.v.02.encipher , code.v.02.cipher , code.v.02.cypher , code.v.02.encrypt , code.v.02.inscribe , code.v.02.write_in_code |
Antonyms | Opposites | Antonym of lemma encode.v.01.encode is decode.v.01.decode |
Hypernym | A broad class that different phrases fall below | A hypernym of code.v.01 (as in “Code the pieces with numbers so that you can identify them later”) is tag.v.01 |
Meronym | A phrase that’s a part of (or subordinate to) a broad class | A meronym of “computer” is “chip” |
Holonym | The relationship between a mother or father phrase and its subordinate components | A hyponym of “window” is “computer screen” |
There are a number of different attributes, which yow will discover within the nltk/corpus/reader/wordnet.py
supply file in <your python set up>/Lib/site-packages
.
Some code could assist this make extra sense.
This helper operate:
def synset_info(synset):
print("Name", synset.title())
print("POS:", synset.pos())
print("Definition:", synset.definition())
print("Examples:", synset.examples())
print("Lemmas:", synset.lemmas())
print("Antonyms:", [lemma.antonyms() for lemma in synset.lemmas() if len(lemma.antonyms()) > zero])
print("Hypernyms:", synset.hypernyms())
print("Instance Hypernyms:", synset.instance_hypernyms())
print("Part Holonyms:", synset.part_holonyms())
print("Part Meronyms:", synset.part_meronyms())
print()
synsets = wordnet.synsets('code')
exhibits this:
5 synsets:
Name code.n.01
POS: n
Definition: a algorithm or ideas or legal guidelines (particularly written ones)
Examples: []
Lemmas: [Lemma('code.n.01.code'), Lemma('code.n.01.codification')]
Antonyms: []
Hypernyms: [Synset('written_communication.n.01')]
Instance Hpernyms: []
Part Holonyms: []
Part Meronyms: []...
Name code.n.03
POS: n
Definition: (pc science) the symbolic association of information or directions in a pc program or the set of such directions
Examples: []
Lemmas: [Lemma('code.n.03.code'), Lemma('code.n.03.computer_code')]
Antonyms: []
Hypernyms: [Synset('coding_system.n.01')]
Instance Hpernyms: []
Part Holonyms: []
Part Meronyms: []...
Name code.v.02
POS: v
Definition: convert peculiar language into code
Examples: ['We should encode the message for security reasons']
Lemmas: [Lemma('code.v.02.code'), Lemma('code.v.02.encipher'), Lemma('code.v.02.cipher'), Lemma('code.v.02.cypher'), Lemma('code.v.02.encrypt'), Lemma('code.v.02.inscribe'), Lemma('code.v.02.write_in_code')]
Antonyms: []
Hypernyms: [Synset('encode.v.01')]
Instance Hpernyms: []
Part Holonyms: []
Part Meronyms: []
Synsets and lemmas comply with a tree construction you may visualize:
def hypernyms(synset):
return synset.hypernyms()synsets = wordnet.synsets('soccer')
for synset in synsets:
print(synset.title() + " tree:")
pprint(synset.tree(rel=hypernyms))
print()
code.n.01 tree:
[Synset('code.n.01'),
[Synset('written_communication.n.01'),
...code.n.02 tree:
[Synset('code.n.02'),
[Synset('coding_system.n.01'),
...code.n.03 tree:
[Synset('code.n.03'),
...code.v.01 tree:
[Synset('code.v.01'),
[Synset('tag.v.01'),
...code.v.02 tree:
[Synset('code.v.02'),
[Synset('encode.v.01'),
...
WordNet does not cowl all phrases and their info (there are about 170,000 phrases in English right this moment and about 155,000 within the newest model of WordNet), nevertheless it’s a very good place to begin. After you be taught the ideas of this constructing block, in case you discover it insufficient in your wants, you may migrate to a different. Or, you may construct your personal WordNet!
Try it your self
Using the Python libraries, obtain Wikipedia’s web page on open source and record the synsets and lemmas of all of the phrases.
Similarity comparability
Similarity comparability is a constructing block that identifies similarities between two items of textual content. It has many functions in search engines like google, chatbots, and extra.
For instance, are the phrases “football” and “soccer” associated?
syn1 = wordnet.synsets('soccer')
syn2 = wordnet.synsets('soccer')# A phrase could have a number of synsets, so want to match every synset of word1 with synset of word2
for s1 in syn1:
for s2 in syn2:
print("Path similarity of: ")
print(s1, '(', s1.pos(), ')', '[', s1.definition(), ']')
print(s2, '(', s2.pos(), ')', '[', s2.definition(), ']')
print(" is", s1.path_similarity(s2))
print()
Path similarity of:
Synset('soccer.n.01') ( n ) [ any of various games played with a ball (round or oval) in which two teams try to kick or carry or propel the ball into each other's goal ]
Synset('soccer.n.01') ( n ) [ a football game in which two teams of 11 players try to kick or head a ball into the opponents' goal ]
is zero.5Path similarity of:
Synset('soccer.n.02') ( n ) [ the inflated oblong ball used in playing American football ]
Synset('soccer.n.01') ( n ) [ a football game in which two teams of 11 players try to kick or head a ball into the opponents' goal ]
is zero.05
The highest path similarity rating of the phrases is zero.5, indicating they’re carefully associated.
What about “code” and “bug”? Similarity scores for these phrases utilized in pc science are:
Path similarity of:
Synset('code.n.01') ( n ) [ a set of rules or principles or laws (especially written ones) ]
Synset('bug.n.02') ( n ) [ a fault or defect in a computer program, system, or machine ]
is zero.1111111111111111
...
Path similarity of:
Synset('code.n.02') ( n ) [ a coding system used for transmitting messages requiring brevity or secrecy ]
Synset('bug.n.02') ( n ) [ a fault or defect in a computer program, system, or machine ]
is zero.09090909090909091
...
Path similarity of:
Synset('code.n.03') ( n ) [ (computer science) the symbolic arrangement of data or instructions in a computer program or the set of such instructions ]
Synset('bug.n.02') ( n ) [ a fault or defect in a computer program, system, or machine ]
is zero.09090909090909091
These are the best similarity scores, which signifies they’re associated.
NLTK gives a number of similarity scorers, reminiscent of:
- path_similarity
- lch_similarity
- wup_similarity
- res_similarity
- jcn_similarity
- lin_similarity
See the Similarity part of the WordNet Interface web page to find out the suitable one in your utility.
Try it your self
Using Python libraries, begin from the Wikipedia Category: Lists of computer terms web page and put together an inventory of terminologies, then see how the phrases correlate.
Tree and treebank
With NLTK, you may signify a textual content’s construction in tree type to assist with textual content evaluation.
Here is an instance:
A easy textual content pre-processed and part-of-speech (POS)-tagged:
import nltktextual content = "I love open source"
# Tokenize to phrases
phrases = nltk.tokenize.word_tokenize(textual content)
# POS tag the phrases
words_tagged = nltk.pos_tag(phrases)
You should outline a grammar to transform the textual content to a tree construction. This instance makes use of a easy grammar based mostly on the Penn Treebank tags.
# A easy grammar to create tree
grammar = "NP: "
Next, use the grammar to create a tree:
# Create tree
parser = nltk.RegexpParser(grammar)
tree = parser.parse(words_tagged)
pprint(tree)
This produces:
Tree('S', [('I', 'PRP'), ('love', 'VBP'), Tree('NP', [('open', 'JJ'), ('source', 'NN')])])
You can see it higher graphically.
tree.draw()
This construction helps clarify the textual content’s that means appropriately. As an instance, establish the subject on this textual content:
subject_tags = ["NN", "NNS", "NP", "NNP", "NNPS", "PRP", "PRP$"]
def topic(sentence_tree):
for tagged_word in sentence_tree:
# A crude logic for this case - first phrase with these tags is taken into account topic
if tagged_word[1] in subject_tags:
return tagged_word[zero]print("Subject:", topic(tree))
It exhibits “I” is the topic:
Subject: I
This is a fundamental textual content evaluation constructing block that’s relevant to bigger functions. For instance, when a consumer says, “Book a flight for my mom, Jane, to NY from London on January 1st,” a chatbot utilizing this block can interpret the request as:
Action: Book
What: Flight
Traveler: Jane
From: London
To: New York
Date: 1 Jan (of the subsequent 12 months)
A treebank refers to a corpus with pre-tagged bushes. Open supply, conditional free-for-use, and business treebanks can be found for a lot of languages. The mostly used one for English is Penn Treebank, extracted from the Wall Street Journal, a subset of which is included in NLTK. Some methods of utilizing a treebank:
phrases = nltk.corpus.treebank.phrases()
print(len(phrases), "words:")
print(phrases)tagged_sents = nltk.corpus.treebank.tagged_sents()
print(len(tagged_sents), "sentences:")
print(tagged_sents)
100676 phrases:
['Pierre', 'Vinken', ',', '61', 'years', 'old', ',', ...]
3914 sentences:
[[('Pierre', 'NNP'), ('Vinken', 'NNP'), (',', ','), ('61', 'CD'), ('years', 'NNS'), ('old', 'JJ'), (',', ','), ('will', 'MD'), ('join', 'VB'), ('the', 'DT'), ('board', 'NN'), ('as', 'IN'), ('a', 'DT'), ('nonexecutive', 'JJ'), ('director', 'NN'), ...]
See tags in a sentence:
sent0 = tagged_sents[zero]
pprint(sent0)
[('Pierre', 'NNP'),
('Vinken', 'NNP'),
(',', ','),
('61', 'CD'),
('years', 'NNS'),
...
Create a grammar to transform this to a tree:
grammar = '''
Subject:
SubjectInfo:
Action: <MD><VB>
Object:
Stopwords: <IN><DT>
ObjectInfo:
When: <NNP><CD>
'''
parser = nltk.RegexpParser(grammar)
tree = parser.parse(sent0)
print(tree)
(S
(Subject Pierre/NNP Vinken/NNP)
,/,
(SubjectInfo 61/CD years/NNS outdated/JJ)
,/,
(Action will/MD be part of/VB)
(Object the/DT board/NN)
as/IN
a/DT
(ObjectInfo nonexecutive/JJ director/NN)
(Subject Nov./NNP)
29/CD
./.)
See it graphically:
tree.draw()
The idea of bushes and treebanks is a robust constructing block for textual content evaluation.
Try it your self
Using the Python libraries, obtain Wikipedia’s web page on open source and signify the textual content in a presentable view.
Named entity recognition
Text, whether or not spoken or written, accommodates vital knowledge. One of textual content processing’s main targets is extracting this key knowledge. This is required in virtually all functions, reminiscent of an airline chatbot that books tickets or a question-answering bot. NLTK gives a named entity recognition function for this.
Here’s a code instance:
sentence = 'Peterson first instructed the title "open source" at Palo Alto, California'
See if title and place are acknowledged on this sentence. Pre-process as typical:
import nltkphrases = nltk.word_tokenize(sentence)
pos_tagged = nltk.pos_tag(phrases)
Run the named-entity tagger:
ne_tagged = nltk.ne_chunk(pos_tagged)
print("NE tagged text:")
print(ne_tagged)
print()
NE tagged textual content:
(S
(PERSON Peterson/NNP)
first/RB
instructed/VBD
the/DT
title/NN
``/``
open/JJ
supply/NN
''/''
at/IN
(FACILITY Palo/NNP Alto/NNP)
,/,
(GPE California/NNP))
Name tags have been added; extract solely the named entities from this tree:
print("Recognized named entities:")
for ne in ne_tagged:
if hasattr(ne, "label"):
print(ne.label(), ne[zero:])
Recognized named entities:
PERSON [('Peterson', 'NNP')]
FACILITY [('Palo', 'NNP'), ('Alto', 'NNP')]
GPE [('California', 'NNP')]
See it graphically:
ne_tagged.draw()
NLTK’s built-in named-entity tagger, utilizing PENN’s Automatic Content Extraction (ACE) program, detects widespread entities reminiscent of ORGANIZATION, PERSON, LOCATION, FACILITY, and GPE (geopolitical entity).
NLTK can use different taggers, such because the Stanford Named Entity Recognizer. This skilled tagger is in-built Java, however NLTK gives an interface to work with it (See nltk.parse.stanford or nltk.tag.stanford).
Try it your self
Using the Python libraries, obtain Wikipedia’s web page on open source and establish individuals who had an affect on open supply and the place and once they contributed.
Advanced train
If you are prepared for it, strive constructing this superstructure utilizing the constructing blocks mentioned in these articles.
Using Python libraries, obtain Wikipedia’s Category: Computer science page and:
- Identify the most-occurring unigrams, bigrams, and trigrams and publish it as an inventory of key phrases or applied sciences that college students and engineers want to concentrate on on this area.
- Show the names, applied sciences, dates, and locations that matter on this discipline graphically. This is usually a good infographic.
- Create a search engine. Does your search engine carry out higher than Wikipedia’s search?
What’s subsequent?
NLP is a quintessential pillar in utility constructing. NLTK is a basic, wealthy, and highly effective package that gives the bricks and mortar to construct virtually interesting, purposeful functions for the true world.
In this sequence of articles, I defined what NLP makes attainable utilizing NLTK for instance. NLP and NLTK have much more to supply. This sequence is an inception level to assist get you began.
If your wants develop past NLTK’s capabilities, you can prepare new fashions or add capabilities to it. New NLP libraries that construct on NLTK are arising, and machine studying is getting used extensively in language processing.
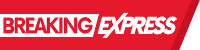