This article was co-written with Lacey Williams Henschel.
Sometimes the fitting device for the job is a command-line software. A command-line software is a program that you just work together with and run from one thing like your shell or Terminal. Git and Curl are examples of command-line functions that you just may already be acquainted with.
Command-line apps are helpful when you could have a little bit of code you wish to run a number of occasions in a row or regularly. Django builders run instructions like ./handle.py runserver
to start out their internet servers; Docker builders run docker-compose up
to spin up their containers. The causes you may wish to write a command-line app are as diverse as the explanations you may wish to write code within the first place.
For this month’s Python column, we now have three libraries to suggest to Pythonistas trying to write their very own command-line instruments.
Click
Click is our favourite Python package deal for command-line functions. It:
- Has nice documentation stuffed with examples
- Includes directions on packaging your app as a Python software so it is simpler to run
- Automatically generates helpful assist textual content
- Lets you stack non-obligatory and required arguments and even several commands
- Has a Django model (
django-click
) for writing administration instructions
Click makes use of its @click on.command()
to declare a operate as a command and specify required or non-obligatory arguments.
# howdy.py
import click on@click on.command()
@click on.choice('--name', default='', assist='Your identify')
def say_hello(identify):
click on.echo("Hello !".format(identify))if __name__ == '__main__':
howdy()
The @click on.choice()
decorator declares an optional argument, and the @click on.argument()
decorator declares a required argument. You can mix non-obligatory and required arguments by stacking the decorators. The echo()
technique prints outcomes to the console.
$ python howdy.py --name='Lacey'
Hello Lacey!
Docopt
Docopt is a command-line software parser, form of like Markdown on your command-line apps. If you want writing the documentation on your apps as you go, Docopt has by far the best-formatted assist textual content of the choices on this article. It is not our favourite command-line app library as a result of its documentation throws you into the deep finish instantly, which makes it a bit harder to get began. Still, it is a light-weight library that could be very well-liked, particularly if exceptionally good documentation is vital to you.
Docopt could be very explicit about the way you format the required docstring on the prime of your file. The prime aspect in your docstring after the identify of your device should be “Usage,” and it ought to listing the methods you count on your command to be known as (e.g., by itself, with arguments, and so on.). Usage ought to embrace assist
and model
flags.
The second aspect in your docstring needs to be “Options,” and it ought to present extra details about the choices and arguments you recognized in “Usage.” The content material of your docstring turns into the content material of your assist textual content.
"""HELLO CLIUsage:
howdy.py
howdy.py <identify>
howdy.py -h|--help
howdy.py -v|--versionOptions:
<identify> Optional identify argument.
-h --help Show this display.
-v --version Show model.
"""from docopt import docopt
def say_hello(identify):
return("Hello !".format(identify))if __name__ == '__main__':
arguments = docopt(__doc__, model='DEMO 1.zero')
if arguments['<identify>']:
print(say_hello(arguments['<identify>']))
else:
print(arguments)
At its most simple stage, Docopt is designed to return your arguments to the console as key-value pairs. If I name the above command with out specifying a reputation, I get a dictionary again:
$ python howdy.py
This reveals me I didn’t enter the assist
or model
flags, and the identify
argument is None
.
But if I name it with a reputation, the say_hello
operate will execute.
$ python howdy.py Jeff
Hello Jeff!
Docopt permits each required and non-obligatory arguments and has completely different syntax conventions for every. Required arguments needs to be represented in ALLCAPS
or in <carets>
, and choices needs to be represented with double or single dashes, like --name
. Read extra about Docopt’s patterns within the docs.
Fire
Fire is a Google library for writing command-line apps. We particularly prefer it when your command must take extra difficult arguments or cope with Python objects, because it tries to deal with parsing your argument sorts intelligently.
Fire’s docs embrace a ton of examples, however I want the docs have been a bit higher organized. Fire can deal with multiple commands in one file, instructions as strategies on objects, and grouping instructions.
Its weak point is the documentation it makes obtainable to the console. Docstrings in your instructions do not seem within the assist textual content, and the assistance textual content would not essentially determine arguments.
import hearthdef say_hello(identify=''):
return 'Hello !'.format(identify)if __name__ == '__main__':
hearth.Fire()
Arguments are made required or non-obligatory relying on whether or not you specify a default worth for them in your operate or technique definition. To name this command, you could specify the filename and the operate identify, extra like Click’s syntax:
$ python howdy.py say_hello Rikki
Hello Rikki!
You also can move arguments as flags, like --name=Rikki
.
Bonus: Packaging!
Click consists of directions (and extremely recommends you comply with them) for packaging your instructions utilizing setuptools
.
To package deal our first instance, add this content material to your setup.py
file:
from setuptools import setupsetup(
identify='howdy',
model='zero.1',
py_modules=['howdy'],
install_requires=[
'Click',
],
entry_points='''
[console_scripts]
howdy=howdy:say_hello
''',
)
Everywhere you see howdy
, substitute the identify of your module however omit the .py
extension. Where you see say_hello
, substitute the identify of your operate.
Then, run pip set up --editable
to make your command obtainable to the command line.
You can now name your command like this:
$ howdy --name='Jeff'
Hello Jeff!
By packaging your command, you omit the additional step within the console of getting to kind python howdy.py --name='Jeff'
and save your self a number of keystrokes. These directions will most likely additionally work for the opposite libraries we talked about.
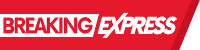