In the primary three articles of this four-part collection evaluating totally different Python net frameworks, we coated the Pyramid, Flask, and Tornado net frameworks. We’ve constructed the identical app 3 times and have lastly made our technique to Django. Django is, by and huge, the key net framework for Python builders nowadays and it is not too laborious to see why. It excels in hiding plenty of the configuration logic and letting you give attention to with the ability to construct huge, rapidly.
That stated, with regards to small tasks, like our To-Do List app, Django is usually a bit like bringing a firehose to a water gun struggle. Let’s see the way it all comes collectively.
About Django
Django kinds itself as “a high-level Python web framework that encourages rapid development and clean, pragmatic design. Built by experienced developers, it takes care of much of the hassle of web development, so you can focus on writing your app without needing to reinvent the wheel.” And they actually imply it! This large net framework comes with so many batteries included that oftentimes throughout improvement it may be a thriller as to how all the things manages to work collectively.
In addition to the framework itself being massive, the Django neighborhood is totally large. In truth, it is so huge and lively that there is a whole website dedicated to the third-party packages individuals have designed to plug into Django to do a complete host of issues. This consists of all the things from authentication and authorization, to full-on Django-powered content material administration techniques, to e-commerce add-ons, to integrations with Stripe. Talk about not re-inventing the wheel; chances are high if you’d like one thing accomplished with Django, somebody has already accomplished it and you may simply pull it into your venture.
For this function, we wish to construct a REST API with Django, so we’ll leverage the at all times fashionable Django REST framework. Its job is to show the Django framework, which was made to serve totally rendered HTML pages constructed with Django’s personal templating engine, right into a system particularly geared towards successfully dealing with REST interactions. Let’s get going with that.
Django startup and configuration
$ mkdir django_todo
$ cd django_todo
$ pipenv set up --python Three.6
$ pipenv shell
(django-someHash) $ pipenv set up django djangorestframework
For reference, we’re working with django-2.zero.7
and djangorestframework-Three.eight.2
.
Unlike Flask, Tornado, and Pyramid, we needn’t write our personal setup.py
file. We’re not making an installable Python distribution. As with many issues, Django takes care of that for us in its personal Django means. We’ll nonetheless want a necessities.txt
file to trace all our mandatory installs for deployment elsewhere. However, so far as focusing on modules inside our Django venture goes, Django will allow us to record the subdirectories we wish entry to, then enable us to import from these directories as in the event that they’re put in packages.
First, now we have to create a Django venture.
When we put in Django, we additionally put in the command-line script django-admin
. Its job is to handle all the varied Django-related instructions that assist put our venture collectively and keep it as we proceed to develop. Instead of getting us construct up the complete Django ecosystem from scratch, the django-admin
will enable us to get began with all of the completely mandatory recordsdata (and extra) we’d like for the standard Django venture.
The syntax for invoking django-admin
‘s start-project command is django-admin startproject <venture title> <listing the place we wish the recordsdata>
. We need the recordsdata to exist in our present working listing, so:
(django-someHash) $ django-admin startproject django_todo .
Typing ls
will present one new file and one new listing.
(django-someHash) $ ls
handle.py django_todo
handle.py
is a command-line-executable Python file that finally ends up simply being a wrapper round django-admin
. As such, its job is identical: to assist us handle our venture. Hence the title handle.py
.
The listing it created, the django_todo
within django_todo
, represents the configuration root for our venture. Let’s dig into that now.
Configuring Django
By calling the django_todo
listing the “configuration root,” we imply this listing holds the recordsdata mandatory for typically configuring our Django venture. Pretty a lot all the things exterior this listing will likely be targeted solely on the “business logic” related to the venture’s fashions, views, routes, and so forth. All factors that join the venture collectively will lead right here.
Calling ls
inside django_todo
reveals 4 recordsdata:
(django-someHash) $ cd django_todo
(django-someHash) $ ls
__init__.py settings.py urls.py wsgi.py
__init__.py
is empty, solely current to show this listing into an importable Python package deal.settings.py
is the place most configuration objects will likely be set, like whether or not the venture’s in DEBUG mode, what databases are in use, the place Django ought to search for recordsdata, and so forth. It is the “main configuration” a part of the configuration root, and we’ll dig into that momentarily.urls.py
is, because the title implies, the place the URLs are set. While we do not have to explicitly write each URL for the venture on this file, we do have to make this file conscious of every other locations the place URLs have been declared. If this file would not level to different URLs, these URLs do not exist. Period.wsgi.py
is for serving the appliance in manufacturing. Just like how Pyramid, Tornado, and Flask uncovered some “app” object that was the configured utility to be served, Django should additionally expose one. That’s accomplished right here. It can then be served with one thing like Gunicorn, Waitress, or uWSGI.
Setting the settings
Taking a glance inside settings.py
will reveal its appreciable dimension—and these are simply the defaults! This would not even embrace hooks for the database, static recordsdata, media recordsdata, any cloud integration, or any of the opposite dozens of ways in which a Django venture could be configured. Let’s see, prime to backside, what we have been given:
BASE_DIR
units absolutely the path to the bottom listing, or the listing the placehandle.py
is situated. This is helpful for finding recordsdata.SECRET_KEY
is a key used for cryptographic signing inside the Django venture. In observe, it is used for issues like classes, cookies, CSRF safety, and auth tokens. As quickly as potential, ideally earlier than the primary commit, the worth forSECRET_KEY
needs to be modified and moved into an surroundings variable.DEBUG
tells Django whether or not to run the venture in improvement mode or manufacturing mode. This is an especially vital distinction.- In improvement mode, when an error pops up, Django will present the total stack hint that led to the error, in addition to all of the settings and configurations concerned in operating the venture. This is usually a large safety subject if
DEBUG
was set toTrue
in a manufacturing surroundings. - In manufacturing, Django reveals a plain error web page when issues go incorrect. No info is given past an error code.
- A easy technique to safeguard our venture is to set
DEBUG
to an surroundings variable, likebool(os.environ.get('DEBUG', ''))
.
- In improvement mode, when an error pops up, Django will present the total stack hint that led to the error, in addition to all of the settings and configurations concerned in operating the venture. This is usually a large safety subject if
ALLOWED_HOSTS
is the literal record of hostnames from which the appliance is being served. In improvement this may be empty, however in manufacturing our Django venture won’t run if the host that serves the venture is just not among the many record of ALLOWED_HOSTS. Another factor for the field of surroundings variables.INSTALLED_APPS
is the record of Django “apps” (consider them as subdirectories; extra on this later) that our Django venture has entry to. We’re given just a few by default to supply…- The built-in Django administrative web site
- Django’s built-in authentication system
- Django’s one-size-fits-all supervisor for knowledge fashions
- Session administration
- Cookie and session-based messaging
- Usage of static recordsdata inherent to the location, like
css
recordsdata,js
recordsdata, any photographs which can be part of our website’s design, and so forth.
MIDDLEWARE
is because it sounds: the middleware that helps our Django venture run. Much of it’s for dealing with varied forms of safety, though we will add others as we’d like them.ROOT_URLCONF
units the import path of our base-level URL configuration file. Thaturls.py
that we noticed earlier than? By default, Django factors to that file to assemble all our URLs. If we wish Django to look elsewhere, we’ll set the import path to that location right here.TEMPLATES
is the record of template engines that Django would use for our website’s frontend if we have been counting on Django to construct our HTML. Since we’re not, it is irrelevant.WSGI_APPLICATION
units the import path of our WSGI utility—the factor that will get served when in manufacturing. By default, it factors to anutility
object inwsgi.py
. This hardly ever, if ever, must be modified.DATABASES
units which databases our Django venture will entry. Thedefault
database should be set. We can set others by title, so long as we offer theHOST
,USER
,PASSWORD
,PORT
, databaseNAME
, and applicableENGINE
. As one may think, these are all delicate items of data, so it is best to cover them away in surroundings variables. Check the Django docs for extra particulars.- Note: If as a substitute of offering particular person items of a database’s location, you’d somewhat present the total database URL, take a look at dj_database_url.
AUTH_PASSWORD_VALIDATORS
is successfully a listing of capabilities that run to test enter passwords. We get just a few by default, but when we had different, extra complicated validation wants—greater than merely checking if the password matches a person’s attribute, if it exceeds the minimal size, if it is one of many 1,00zero commonest passwords, or if the password is completely numeric—we may record them right here.LANGUAGE_CODE
will set the language for the location. By default it is US English, however we may swap it as much as be different languages.TIME_ZONE
is the time zone for any autogenerated timestamps in our Django venture. I can’t stress sufficient how necessary it’s that we keep on with UTC and carry out any time zone-specific processing elsewhere as a substitute of attempting to reconfigure this setting. As this article states, UTC is the widespread denominator amongst all time zones as a result of there aren’t any offsets to fret about. If offsets are that necessary, we may calculate them as wanted with an applicable offset from UTC.USE_I18N
will let Django use its personal translation providers to translate strings for the entrance finish. I18N = internationalization (18 characters between “i” and “n”)USE_L10N
(L10N = localization [10 characters between “l” and “n”]) will use the widespread native formatting of knowledge if set toTrue
. An incredible instance is dates: within the US it is MM-DD-YYYY. In Europe, dates are typically written DD-MM-YYYYSTATIC_URL
is a component of a bigger physique of settings for serving static recordsdata. We’ll be constructing a REST API, so we can’t want to fret about static recordsdata. In basic, this units the foundation path after the area title for each static file. So, if we had a brand picture to serve, it might behttp://<domainname>/<STATIC_URL>/logo.gif
These settings are just about able to go by default. One factor we’ll have to vary is the DATABASES
setting. First, we create the database that we’ll be utilizing with:
(django-someHash) $ createdb django_todo
We wish to use a PostgreSQL database like we did with Flask, Pyramid, and Tornado. That means we’ll have to vary the DATABASES
setting to permit our server to entry a PostgreSQL database. First: the engine. By default, the database engine is django.db.backends.sqlite3
. We’ll be altering that to django.db.backends.postgresql
.
For extra details about Django’s accessible engines, check the docs. Note that whereas it’s technically potential to include a NoSQL answer right into a Django venture, out of the field, Django is strongly biased towards SQL options.
Next, now we have to specify the key-value pairs for the totally different components of the connection parameters.
NAME
is the title of the database we simply created.USER
is a person’s Postgres database usernamePASSWORD
is the password wanted to entry the databaseHOST
is the host for the database.localhost
or127.zero.zero.1
will work, as we’re growing regionally.PORT
is no matter PORT now we have open for Postgres; it is usually5432
.
settings.py
expects us to supply string values for every of those keys. However, that is extremely delicate info. That’s not going to work for any accountable developer. There are a number of methods to handle this downside, however we’ll simply arrange surroundings variables.
DATABASES =
'default':
'ENGINE': 'django.db.backends.postgresql',
'NAME': os.environ.get('DB_NAME', ''),
'USER': os.environ.get('DB_USER', ''),
'PASSWORD': os.environ.get('DB_PASS', ''),
'HOST': os.environ.get('DB_HOST', ''),
'PORT': os.environ.get('DB_PORT', ''),
Before going ahead, make certain to set the surroundings variables or Django won’t work. Also, we have to set up psycopg2
into this surroundings so we will speak to our database.
Django routes and views
Let’s make one thing perform inside this venture. We’ll be utilizing Django REST Framework to assemble our REST API, so now we have to verify we will use it by including rest_framework
to the tip of INSTALLED_APPS
in settings.py
.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.classes',
'django.contrib.messages',
'django.contrib.staticfiles',
'rest_framework'
]
While Django REST Framework would not completely require class-based views (like Tornado) to deal with incoming requests, it’s the popular technique for writing views. Let’s outline one.
Let’s create a file referred to as views.py
in django_todo
. Within views.py
, we’ll create our “Hello, world!” view.
# in django_todo/views.py
from rest_framework.response import JsonResponse
from rest_framework.views import APIViewclass HelloWorld(APIView):
def get(self, request, format=None):
"""Print 'Hello, world!' because the response physique."""
return JsonResponse("Hello, world!")
Every Django REST Framework class-based view inherits both immediately or not directly from APIView
. APIView
handles a ton of stuff, however for our functions it does these particular issues:
- Sets up the strategies wanted to direct site visitors based mostly on the HTTP technique (e.g. GET, POST, PUT, DELETE)
- Populates the
request
object with all the info and attributes we’ll want for parsing and processing any incoming request - Takes the
Response
orJsonResponse
that each dispatch technique (i.e., strategies namedget
,submit
,put
,delete
) returns and constructs a correctly formatted HTTP response.
Yay, now we have a view! On its personal it does nothing. We want to attach it to a route.
If we hop into django_todo/urls.py
, we attain our default URL configuration file. As talked about earlier: If a route in our Django venture is just not included right here, it would not exist.
We add desired URLs by including them to the given urlpatterns
record. By default, we get a complete set of URLs for Django’s built-in website administration backend. We’ll delete that utterly.
We additionally get some very useful doc strings that inform us precisely how you can add routes to our Django venture. We’ll want to supply a name to path()
with three parameters:
- The desired route, as a string (with out the main slash)
- The view perform (solely ever a perform!) that can deal with that route
- The title of the route in our Django venture
Let’s import our HelloWorld
view and fasten it to the house route "/"
. We can even take away the trail to the admin
from urlpatterns
, as we can’t be utilizing it.
# django_todo/urls.py, after the large doc string
from django.urls import path
from django_todo.views import HelloWorldurlpatterns = [
path('', HelloWorld.as_view(), title="hello"),
]
Well, that is totally different. The route we specified is only a clean string. Why does that work? Django assumes that each path we declare begins with a number one slash. We’re simply specifying routes to assets after the preliminary area title. If a route is not going to a selected useful resource and is as a substitute simply the house web page, the route is simply ""
, or successfully “no resource.”
The HelloWorld
view is imported from that views.py
file we simply created. In order to do that import, we have to replace settings.py
to incorporate django_todo
within the record of INSTALLED_APPS
. Yeah, it is a bit bizarre. Here’s a technique to consider it.
INSTALLED_APPS
refers back to the record of directories or packages that Django sees as importable. It’s Django’s means of treating particular person elements of a venture like put in packages with out going by way of a setup.py
. We need the django_todo
listing to be handled like an importable package deal, so we embrace that listing in INSTALLED_APPS
. Now, any module inside that listing can be importable. So we get our view.
The path
perform will ONLY take a view perform as that second argument, not only a class-based view by itself. Luckily, all legitimate Django class-based views embrace this .as_view()
technique. Its job is to roll up all of the goodness of the class-based view right into a view perform and return that view perform. So, we by no means have to fret about making that translation. Instead, we solely have to consider the enterprise logic, letting Django and Django REST Framework deal with the remaining.
Let’s crack this open within the browser!
Django comes packaged with its personal native improvement server, accessible by way of handle.py
. Let’s navigate to the listing containing handle.py
and kind:
(django-someHash) $ ./handle.py runserver
Performing system checks...System test recognized no points (zero silenced).
August 01, 2018 - 16:47:24
Django model 2.zero.7, utilizing settings 'django_todo.settings'
Starting improvement server at http://127.0.0.1:8000/
Quit the server with CONTROL-C.
When runserver
is executed, Django does a test to verify the venture is (roughly) wired collectively appropriately. It’s not fool-proof, but it surely does catch some obvious points. It additionally notifies us if our database is out of sync with our code. Undoubtedly ours is as a result of we’ve not dedicated any of our utility’s stuff to our database, however that is fantastic for now. Let’s go to http://127.0.0.1:8000
to see the output of the HelloWorld
view.
Huh. That’s not the plaintext knowledge we noticed in Pyramid, Flask, and Tornado. When Django REST Framework is used, the HTTP response (when considered within the browser) is that this type of rendered HTML, displaying our precise JSON response in pink.
But do not fret! If we do a fast curl
taking a look at http://127.0.0.1:8000
within the command line, we do not get any of that fancy HTML. Just the content material.
# Note: do this in a special terminal window, exterior of the digital surroundings above
$ curl http://127.0.0.1:8000
"Hello, world!"
Bueno!
Django REST Framework desires us to have a human-friendly interface when utilizing the browser. This is sensible; if JSON is considered within the browser, it is usually as a result of a human desires to test that it appears proper or get a way of what the JSON response will appear like as they design some client of an API. It’s loads like what you’d get from a service like Postman.
Either means, we all know our view is working! Woo! Let’s recap what we have accomplished:
- Started the venture with
django-admin startproject <venture title>
- Updated the
django_todo/settings.py
to make use of surroundings variables forDEBUG
,SECRET_KEY
, and values within theDATABASES
dict - Installed
Django REST Framework
and added it to the record ofINSTALLED_APPS
- Created
django_todo/views.py
to incorporate our first view class to say Hello to the World - Updated
django_todo/urls.py
with a path to our new dwelling route - Updated
INSTALLED_APPS
indjango_todo/settings.py
to incorporate thedjango_todo
package deal
Creating fashions
Let’s create our knowledge fashions now.
A Django venture’s whole infrastructure is constructed round knowledge fashions. It’s written so every knowledge mannequin can have its personal little universe with its personal views, its personal set of URLs that concern its assets, and even its personal assessments (if we’re so inclined).
If we needed to construct a easy Django venture, we may circumvent this by simply writing our personal fashions.py
file within the django_todo
listing and importing it into our views. However, we’re attempting to jot down a Django venture the “right” means, so we should always divide up our fashions as finest we will into their very own little packages The Django Way™.
The Django Way includes creating what are referred to as Django “apps.” Django “apps” aren’t separate functions per se; they do not have their very own settings and whatnot (though they’ll). They can, nonetheless, have nearly all the things else one would possibly consider being in a standalone utility:
- Set of self-contained URLs
- Set of self-contained HTML templates (if we wish to serve HTML)
- One or extra knowledge fashions
- Set of self-contained views
- Set of self-contained assessments
They are made to be impartial to allow them to be simply shared like standalone functions. In truth, Django REST Framework is an instance of a Django app. It comes packaged with its personal views and HTML templates for serving up our JSON. We simply leverage that Django app to show our venture right into a full-on RESTful API with much less problem.
To create the Django app for our To-Do List objects, we’ll wish to use the startapp
command with handle.py
.
(django-someHash) $ ./handle.py startapp todo
The startapp
command will succeed silently. We can test that it did what it ought to’ve accomplished through the use of ls
.
(django-someHash) $ ls
Pipfile Pipfile.lock django_todo handle.py todo
Look at that: We’ve acquired a model new todo
listing. Let’s look inside!
(django-someHash) $ ls todo
__init__.py admin.py apps.py migrations fashions.py assessments.py views.py
Here are the recordsdata that handle.py startapp
created:
__init__.py
is empty; it exists so this listing could be seen as a legitimate import path for fashions, views, and so forth.admin.py
is just not fairly empty; it is used for formatting this app’s fashions within the Django admin, which we’re not entering into on this article.apps.py
… not a lot work to do right here both; it helps with formatting fashions for the Django admin.migrations
is a listing that’ll comprise snapshots of our knowledge fashions; it is used for updating our database. This is without doubt one of the few frameworks that comes with database administration built-in, and a part of that’s permitting us to replace our database as a substitute of getting to tear it down and rebuild it to vary the schema.fashions.py
is the place the info fashions dwell.assessments.py
is the place assessments would go—if we wrote any.views.py
is for the views we write that pertain to the fashions on this app. They do not should be written right here. We may, for instance, write all our views indjango_todo/views.py
. It’s right here, nonetheless, so it is simpler to separate our issues. This turns into way more related with sprawling functions that cowl many conceptual areas.
What hasn’t been created for us is a urls.py
file for this app. We could make that ourselves.
(django-someHash) $ contact todo/urls.py
Before transferring ahead we should always do ourselves a favor and add this new Django app to our record of INSTALLED_APPS
in django_todo/settings.py
.
# in settings.py
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.classes',
'django.contrib.messages',
'django.contrib.staticfiles',
'rest_framework',
'django_todo',
'todo' # <--- the road was added
]
Inspecting todo/fashions.py
reveals that handle.py
already wrote a little bit of code for us to get began. Diverging from how fashions have been created within the Flask, Tornado, and Pyramid implementations, Django would not leverage a 3rd social gathering to handle database classes or the development of its object cases. It’s all rolled into Django’s django.db.fashions
submodule.
The means a mannequin is constructed, nonetheless, is kind of the identical. To create a mannequin in Django, we’ll have to construct a class
that inherits from fashions.Model
. All the fields that can apply to cases of that mannequin ought to seem as class attributes. Instead of importing columns and area sorts from SQLAlchemy like now we have prior to now, all of our fields will come immediately from django.db.fashions
.
# todo/fashions.py
from django.db import fashionsclass Task(fashions.Model):
"""Tasks for the To Do record."""
title = fashions.CharField(max_length=256)
observe = fashions.TextField(clean=True, null=True)
creation_date = fashions.DateTimeField(auto_now_add=True)
due_date = fashions.DateTimeField(clean=True, null=True)
accomplished = fashions.BooleanField(default=False)
While there are some particular variations between what Django wants and what SQLAlchemy-based techniques want, the general contents and construction are roughly the identical. Let’s level out the variations.
We not have to declare a separate area for an auto-incremented ID quantity for our object cases. Django builds one for us until we specify a special area as the first key.
Instead of instantiating Column
objects which can be handed datatype objects, we simply immediately reference the datatypes because the columns themselves.
The Unicode
area grew to become both fashions.CharField
or fashions.TextField
. CharField
is for small textual content fields of a selected most size, whereas TextField
is for any quantity of textual content.
The TextField
ought to be capable of be clean, and we specify this in TWO methods. clean=True
says that when an occasion of this mannequin is constructed, and the info hooked up to this area is being validated, it is OK for that knowledge to be empty. This is totally different from null=True
, which says when the desk for this mannequin class is constructed, the column similar to observe
will enable for clean or NULL
entries. So, to sum that every one up, clean=True
controls how knowledge will get added to mannequin cases whereas null=True
controls how the database desk holding that knowledge is constructed within the first place.
The DateTime
area grew some muscle and have become capable of do some work for us as a substitute of us having to change the __init__
technique for the category. For the creation_date
area, we specify auto_now_add=True
. What this implies in a sensible sense is that when a brand new mannequin occasion is created Django will mechanically file the date and time of now as that area’s worth. That’s helpful!
When neither auto_now_add
nor its shut cousin auto_now
are set to True
, DateTimeField
will anticipate knowledge like every other area. It’ll should be fed with a correct datetime
object to be legitimate. The due_date
column has clean
and null
each set to True
in order that an merchandise on the To-Do List can simply be an merchandise to be accomplished sooner or later sooner or later, with no outlined date or time.
BooleanField
simply finally ends up being a area that may take certainly one of two values: True
or False
. Here, the default worth is about to be False
.
Managing the database
As talked about earlier, Django has its personal means of doing database administration. Instead of getting to jot down… actually any code in any respect concerning our database, we leverage the handle.py
script that Django supplied on building. It’ll handle not simply the development of the tables for our database, but additionally any updates we want to make to these tables with out essentially having to blow the entire thing away!
Because we have constructed a new mannequin, we have to make our database conscious of it. First, we have to put into code the schema that corresponds to this mannequin. The makemigrations
command of handle.py
will take a snapshot of the mannequin class we constructed and all its fields. It’ll take that info and package deal it right into a Python script that’ll dwell on this specific Django app’s migrations
listing. There won’t ever be a purpose to run this migration script immediately. It’ll exist solely in order that Django can use it as a foundation to replace our database desk or to inherit info after we replace our mannequin class.
(django-someHash) $ ./handle.py makemigrations
Migrations for 'todo':
todo/migrations/0001_initial.py
- Create mannequin Task
This will take a look at each app listed in INSTALLED_APPS
and test for fashions that exist in these apps. It’ll then test the corresponding migrations
listing for migration recordsdata and examine them to the fashions in every of these INSTALLED_APPS
apps. If a mannequin has been upgraded past what the most recent migration says ought to exist, a brand new migration file will likely be created that inherits from the latest one. It’ll be mechanically named and in addition be given a message that claims what modified because the final migration.
If it has been some time because you final labored in your Django venture and may’t bear in mind in case your fashions have been in sync along with your migrations, you don’t have any have to concern. makemigrations
is an idempotent operation; your migrations
listing could have just one copy of the present mannequin configuration whether or not you run makemigrations
as soon as or 20 occasions. Even higher than that, after we run ./handle.py runserver
, Django will detect that our fashions are out of sync with our migrations, and it will simply flat out inform us in coloured textual content so we will make the suitable selection.
This subsequent level is one thing that journeys everyone up at the very least as soon as: Creating a migration file doesn’t instantly have an effect on our database. When we ran makemigrations
, we ready our Django venture to outline how a given desk needs to be created and find yourself wanting. It’s nonetheless on us to use these adjustments to our database. That’s what the migrate
command is for.
(django-someHash) $ ./handle.py migrate
Operations to carry out:
Apply all migrations: admin, auth, contenttypes, classes, todo
Running migrations:
Applying contenttypes.0001_initial... OK
Applying auth.0001_initial... OK
Applying admin.0001_initial... OK
Applying admin.0002_logentry_remove_auto_add... OK
Applying contenttypes.0002_remove_content_type_name... OK
Applying auth.0002_alter_permission_name_max_length... OK
Applying auth.0003_alter_user_email_max_length... OK
Applying auth.0004_alter_user_username_opts... OK
Applying auth.0005_alter_user_last_login_null... OK
Applying auth.0006_require_contenttypes_0002... OK
Applying auth.0007_alter_validators_add_error_messages... OK
Applying auth.0008_alter_user_username_max_length... OK
Applying auth.0009_alter_user_last_name_max_length... OK
Applying classes.0001_initial... OK
Applying todo.0001_initial... OK
When we apply our migrations, Django first checks to see if the opposite INSTALLED_APPS
have migrations to be utilized. It checks them in roughly the order they’re listed. We need our app to be listed final, as a result of we wish to make it possible for, in case our mannequin is determined by any of Django’s built-in fashions, the database updates we make do not undergo from dependency issues.
We have one other mannequin to construct: the User mannequin. However, the sport has modified a bit since we’re utilizing Django. So many functions require some type of User mannequin that Django’s django.contrib.auth
package deal constructed its personal for us to make use of. If it weren’t for the authentication token we require for our customers, we may simply transfer on and use it as a substitute of reinventing the wheel.
However, we’d like that token. There are a pair of how we will deal with this.
- Inherit from Django’s
User
object, making our personal object that extends it by including atoken
area - Create a brand new object that exists in a one-to-one relationship with Django’s
User
object, whose solely function is to carry a token
I am within the behavior of constructing object relationships, so let’s go together with the second choice. Let’s name it an Owner
because it principally has the same connotation as a User
, which is what we wish.
Out of sheer laziness, we may simply embrace this new Owner
object in todo/fashions.py
, however let’s chorus from that. Owner
would not explicitly should do with the creation or upkeep of things on the duty record. Conceptually, the Owner
is solely the proprietor of the duty. There could even come a time the place we wish to broaden this Owner
to incorporate different knowledge that has completely nothing to do with duties.
Just to be secure, let’s make an proprietor
app whose job is to accommodate and deal with this Owner
object.
(django-someHash) $ ./handle.py startapp proprietor
Don’t overlook so as to add it to the record of INSTALLED_APPS
in settings.py
.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.classes',
'django.contrib.messages',
'django.contrib.staticfiles',
'rest_framework',
'django_todo',
'todo',
'proprietor'
]
If we take a look at the foundation of our Django venture, we now have two Django apps:
(django-someHash) $ ls
Pipfile Pipfile.lock django_todo handle.py proprietor todo
In proprietor/fashions.py
, let’s construct this Owner
mannequin. As talked about earlier, it will have a one-to-one relationship with Django’s built-in User
object. We can implement this relationship with Django’s fashions.OneToOneSubject
# proprietor/fashions.py
from django.db import fashions
from django.contrib.auth.fashions import User
import secrets and techniquesclass Owner(fashions.Model):
"""The object that owns duties."""
person = fashions.OneToOneSubject(User, on_delete=fashions.CASCADE)
token = fashions.CharField(max_length=256)def __init__(self, *args, **kwargs):
"""On building, set token."""
self.token = secrets and techniques.token_urlsafe(64)
tremendous().__init__(*args, **kwargs)
This says the Owner
object is linked to the User
object, with one proprietor
occasion per person
occasion. on_delete=fashions.CASCADE
dictates that if the corresponding User
will get deleted, the Owner
occasion it is linked to may even get deleted. Let’s run makemigrations
and migrate
to bake this new mannequin into our database.
(django-someHash) $ ./handle.py makemigrations
Migrations for 'proprietor':
proprietor/migrations/0001_initial.py
- Create mannequin Owner
(django-someHash) $ ./handle.py migrate
Operations to carry out:
Apply all migrations: admin, auth, contenttypes, proprietor, classes, todo
Running migrations:
Applying proprietor.0001_initial... OK
Now our Owner
must personal some Task
objects. It’ll be similar to the OneToOneSubject
seen above, besides that we’ll stick a ForeignKey
area on the Task
object pointing to an Owner
.
# todo/fashions.py
from django.db import fashions
from proprietor.fashions import Ownerclass Task(fashions.Model):
"""Tasks for the To Do record."""
title = fashions.CharField(max_length=256)
observe = fashions.TextField(clean=True, null=True)
creation_date = fashions.DateTimeField(auto_now_add=True)
due_date = fashions.DateTimeField(clean=True, null=True)
accomplished = fashions.BooleanField(default=False)
proprietor = fashions.ForeignKey(Owner, on_delete=fashions.CASCADE)
Every To-Do List job has precisely one proprietor who can personal a number of duties. When that proprietor is deleted, any job they personal goes with them.
Let’s now run makemigrations
to take a brand new snapshot of our knowledge mannequin setup, then migrate
to use these adjustments to our database.
(django-someHash) django $ ./handle.py makemigrations
You try so as to add a non-nullable area 'proprietor' to job with out a default; we will not do this (the database wants one thing to populate current rows).
Please choose a repair:
1) Provide a one-off default now (will likely be set on all current rows with a null worth for this column)
2) Quit, and let me add a default in fashions.py
Oh no! We have an issue! What occurred? Well, after we created the Owner
object and added it as a ForeignKey
to Task
, we principally required that each Task
requires an Owner
. However, the primary migration we made for the Task
object did not embrace that requirement. So, despite the fact that there is no knowledge in our database’s desk, Django is doing a pre-check on our migrations to verify they’re appropriate and this new migration we’re proposing is just not.
There are just a few methods to cope with this type of downside:
- Blow away the present migration and construct a brand new one that features the present mannequin configuration
- Add a default worth to the
proprietor
area on theTask
object - Allow duties to have
NULL
values for theproprietor
area.
Option 2 would not make a lot sense right here; we would be proposing that any Task
that was created would, by default, be linked to some default proprietor regardless of none essentially current.
Option 1 would require us to destroy and rebuild our migrations. We ought to depart these alone.
Let’s go together with choice Three. In this circumstance, it will not be the tip of the world if we enable the Task
desk to have null values for the house owners; any duties created from this level ahead will essentially have an proprietor. If you are in a state of affairs the place that is not an appropriate schema on your database desk, blow away your migrations, drop the desk, and rebuild the migrations.
# todo/fashions.py
from django.db import fashions
from proprietor.fashions import Ownerclass Task(fashions.Model):
"""Tasks for the To Do record."""
title = fashions.CharField(max_length=256)
observe = fashions.TextField(clean=True, null=True)
creation_date = fashions.DateTimeField(auto_now_add=True)
due_date = fashions.DateTimeField(clean=True, null=True)
accomplished = fashions.BooleanField(default=False)
proprietor = fashions.ForeignKey(Owner, on_delete=fashions.CASCADE, null=True)
(django-someHash) $ ./handle.py makemigrations
Migrations for 'todo':
todo/migrations/0002_task_owner.py
- Add area proprietor to job
(django-someHash) $ ./handle.py migrate
Operations to carry out:
Apply all migrations: admin, auth, contenttypes, proprietor, classes, todo
Running migrations:
Applying todo.0002_task_owner... OK
Woo! We have our fashions! Welcome to the Django means of declaring objects.
For good measure, let’s be certain that each time a User
is made, it is mechanically linked with a brand new Owner
object. We can do that utilizing Django’s indicators
system. Basically, we are saying precisely what we intend: “When we get the sign new User
has been constructed, assemble a brand new Owner
and set that new User
as that Owner
‘s person
area.” In observe that appears like:
# proprietor/fashions.py
from django.contrib.auth.fashions import User
from django.db import fashions
from django.db.fashions.indicators import post_save
from django.dispatch import receiverimport secrets and techniques
class Owner(fashions.Model):
"""The object that owns duties."""
person = fashions.OneToOneSubject(User, on_delete=fashions.CASCADE)
token = fashions.CharField(max_length=256)def __init__(self, *args, **kwargs):
"""On building, set token."""
self.token = secrets and techniques.token_urlsafe(64)
tremendous().__init__(*args, **kwargs)@receiver(post_save, sender=User)
def link_user_to_owner(sender, **kwargs):
"""If a brand new User is saved, create a corresponding Owner."""
if kwargs['created']:
proprietor = Owner(person=kwargs['occasion'])
proprietor.save()
We arrange a perform that listens for indicators to be despatched from the User
object constructed into Django. It’s ready for simply after a User
object has been saved. This can come from both a brand new User
or an replace to an current User
; we discern between the 2 situations inside the listening perform.
If the factor sending the sign was a newly created occasion, kwargs['created']
could have the worth of True
. We solely wish to do one thing if that is True
. If it is a new occasion, we create a brand new Owner
, setting its person
area to be the brand new User
occasion that was created. After that, we save()
the brand new Owner
. This will commit our change to the database if all is nicely. It’ll fail if the info would not validate towards the fields we declared.
Now let’s speak about how we’ll entry the info.
Accessing mannequin knowledge
In the Flask, Pyramid, and Tornado frameworks, we accessed mannequin knowledge by operating queries towards some database session. Maybe it was hooked up to a request
object, perhaps it was a standalone session
object. Regardless, we needed to set up a dwell connection to the database and question on that connection.
This is not the way in which Django works. Django, by default, would not leverage any third-party object-relational mapping (ORM) to converse with the database. Instead, Django permits the mannequin lessons to take care of their very own conversations with the database.
Every mannequin class that inherits from django.db.fashions.Model
could have hooked up to it an objects
object. This will take the place of the session
or dbsession
we have grow to be so conversant in. Let’s open the particular shell that Django offers us and examine how this objects
object works.
(django-someHash) $ ./handle.py shell
Python Three.7.zero (default, Jun 29 2018, 20:13:13)
[Clang 9.1.0 (clang-902.0.39.2)] on darwin
Type "help", "copyright", "credits" or "license" for extra info.
(InteractiveConsole)
>>>
The Django shell is totally different from a standard Python shell in that it is conscious of the Django venture we have been constructing and may do straightforward imports of our fashions, views, settings, and so forth. with out having to fret about putting in a package deal. We can entry our fashions with a easy import
.
>>> from proprietor.fashions import Owner
>>> Owner
<class 'proprietor.fashions.Owner'>
Currently, now we have no Owner
cases. We can inform by querying for them with Owner.objects.all()
.
>>> Owner.objects.all()
<QuestionSet []>
Anytime we run a question technique on the <Model>.objects
object, we’ll get a QuestionSet
again. For our functions, it is successfully a record
, and this record
is displaying us that it is empty. Let’s make an Owner
by making a User
.
>>> from django.contrib.auth.fashions import User
>>> new_user = User(username='kenyattamurphy', electronic mail='[email protected]')
>>> new_user.set_password('wakandaforever')
>>> new_user.save()
If we question for all of our Owner
s now, we should always discover Kenyatta.
>>> Owner.objects.all()
<QuestionSet [<Owner: Owner object (1)>]>
Yay! We’ve acquired knowledge!
Serializing fashions
We’ll be passing knowledge backwards and forwards past simply “Hello World.” As such, we’ll wish to see some type of JSON-ified output that represents that knowledge nicely. Taking that object’s knowledge and reworking it right into a JSON object for submission throughout HTTP is a model of knowledge serialization. In serializing knowledge, we’re taking the info we at present have and reformatting it to suit some customary, more-easily-digestible type.
If I have been doing this with Flask, Pyramid, and Tornado, I would create a brand new technique on every mannequin to present the person direct entry to name to_json()
. The solely job of to_json()
can be to return a JSON-serializable (i.e. numbers, strings, lists, dicts) dictionary with no matter fields I wish to be displayed for the thing in query.
It’d most likely look one thing like this for the Task
object:
class Task(Base):
...all the fields...def to_json(self):
"""Convert job attributes to a JSON-serializable dict."""
return
'id': self.id,
'title': self.title,
'observe': self.observe,
'creation_date': self.creation_date.strftime('%m/%d/%Y %H:%M:%S'),
'due_date': self.due_date.strftime('%m/%d/%Y %H:%M:%S'),
'accomplished': self.accomplished,
'person': self.user_id
It’s not fancy, but it surely does the job.
Django REST Framework, nonetheless, supplies us with an object that’ll not solely do this for us but additionally validate inputs after we wish to create new object cases or replace current ones. It’s referred to as the ModelSerializer.
Django REST Framework’s ModelSerializer
is successfully documentation for our fashions. They do not have lives of their very own if there aren’t any fashions hooked up (for that there is the Serializer class). Their most important job is to precisely characterize our mannequin and make the conversion to JSON inconsiderate when our mannequin’s knowledge must be serialized and despatched over a wire.
Django REST Framework’s ModelSerializer
works finest for easy objects. As an instance, think about that we did not have that ForeignKey
on the Task
object. We may create a serializer for our Task
that will convert its area values to JSON as mandatory with the next declaration:
# todo/serializers.py
from rest_framework import serializers
from todo.fashions import Taskclass TaskSerializer(serializers.ModelSerializer):
"""Serializer for the Task mannequin."""class Meta:
mannequin = Task
fields = ('id', 'title', 'observe', 'creation_date', 'due_date', 'accomplished')
Inside our new TaskSerializer
, we create a Meta
class. Meta
‘s job right here is simply to carry info (or metadata) in regards to the factor we’re making an attempt to serialize. Then, we observe the particular fields that we wish to present. If we needed to point out all of the fields, we may simply shortcut the method and use '__all__'
. We may, alternatively, use the exclude
key phrase as a substitute of fields
to inform Django REST Framework that we wish each area aside from a choose few. We can have as many serializers as we like, so perhaps we wish one for a small subset of fields and one for all of the fields? Go wild right here.
In our case, there’s a relation between every Task
and its proprietor Owner
that should be mirrored right here. As such, we have to borrow the serializers.PrimaryKeyRelatedField
object to specify that every Task
could have an Owner
and that relationship is one-to-one. Its proprietor will likely be discovered from the set of all house owners that exists. We get that set by doing a question for these house owners and returning the outcomes we wish to be related to this serializer: Owner.objects.all()
. We additionally want to incorporate proprietor
within the record of fields, as we at all times want an Owner
related to a Task
# todo/serializers.py
from rest_framework import serializers
from todo.fashions import Task
from proprietor.fashions import Ownerclass TaskSerializer(serializers.ModelSerializer):
"""Serializer for the Task mannequin."""
proprietor = serializers.PrimaryKeyRelatedField(queryset=Owner.objects.all())class Meta:
mannequin = Task
fields = ('id', 'title', 'observe', 'creation_date', 'due_date', 'accomplished', 'proprietor')
Now that this serializer is constructed, we will use it for all of the CRUD operations we would love to do for our objects:
- If we wish to
GET
a JSONified model of a selectedTask
, we will doTaskSerializer(some_task).knowledge
- If we wish to settle for a
POST
with the suitable knowledge to create a brand newTask
, we will useTaskSerializer(knowledge=new_data).save()
- If we wish to replace some current knowledge with a
PUT
, we will sayTaskSerializer(existing_task, knowledge=knowledge).save()
We’re not together with delete
as a result of we do not actually need to do something with info for a delete
operation. If you will have entry to an object you wish to delete, simply say object_instance.delete()
.
Here is an instance of what some serialized knowledge would possibly appear like:
>>> from todo.fashions import Task
>>> from todo.serializers import TaskSerializer
>>> from proprietor.fashions import Owner
>>> from django.contrib.auth.fashions import User
>>> new_user = User(username='kenyatta', electronic mail='[email protected]')
>>> new_user.save_password('wakandaforever')
>>> new_user.save() # creating the User that builds the Owner
>>> kenyatta = Owner.objects.first() # grabbing the Owner that's kenyatta
>>> new_task = Task(title="Buy roast beef for the Sunday potluck", proprietor=kenyatta)
>>> new_task.save()
>>> TaskSerializer(new_task).knowledge
There’s much more you are able to do with the ModelSerializer
objects, and I recommend checking the docs for these better capabilities. Otherwise, that is as a lot as we’d like. It’s time to dig into some views.
Views for reals
We’ve constructed the fashions and the serializers, and now we have to arrange the views and URLs for our utility. After all, we will not do something with an utility that has no views. We’ve already seen an instance with the HelloWorld
view above. However, that is at all times a contrived, proof-of-concept instance and would not actually present what could be accomplished with Django REST Framework’s views. Let’s filter the HelloWorld
view and URL so we will begin contemporary with our views.
The first view we’ll construct is the InfoView
. As within the earlier frameworks, we simply wish to package deal and ship out a dictionary of our proposed routes. The view itself can dwell in django_todo.views
because it would not pertain to a selected mannequin (and thus would not conceptually belong in a selected app).
# django_todo/views.py
from rest_framework.response import JsonResponse
from rest_framework.views import APIViewclass InfoView(APIView):
"""List of routes for this API."""
def get(self, request):
output =
return JsonResponse(output)
This is just about an identical to what we had in Tornado. Let’s hook it as much as an applicable route and be on our means. For good measure, we’ll additionally take away the admin/
route, as we can’t be utilizing the Django administrative backend right here.
# in django_todo/urls.py
from django_todo.views import InfoView
from django.urls import pathurlpatterns = [
path('api/v1', InfoView.as_view(), title="info"),
]
Connecting fashions to views
Let’s determine the following URL, which would be the endpoint for both creating a brand new Task
or itemizing a person’s current duties. This ought to exist in a urls.py
within the todo
app since this has to deal particularly with Task
objects as a substitute of being part of the entire venture.
# in todo/urls.py
from django.urls import path
from todo.views import TaskListViewurlpatterns = [
path('', TaskListView.as_view(), title="list_tasks")
]
What’s the cope with this route? We did not specify a selected person or a lot of a path in any respect. Since there can be a few routes requiring the bottom path /api/v1/accounts/<username>/duties
, why write it many times after we can simply write it as soon as?
Django permits us to take a complete suite of URLs and import them into the bottom django_todo/urls.py
file. We can then give each a kind of imported URLs the identical base path, solely worrying in regards to the variable components when, , they range.
# in django_todo/urls.py
from django.urls import embrace, path
from django_todo.views import InfoViewurlpatterns = [
path('api/v1', InfoView.as_view(), title="info"),
path('api/v1/accounts/<str:username>/duties', embrace('todo.urls'))
]
And now each URL coming from todo/urls.py
will likely be prefixed with the trail api/v1/accounts/<str:username>/duties
.
Let’s construct out the view in todo/views.py
# todo/views.py
from django.shortcuts import get_object_or_404
from rest_framework.response import JsonResponse
from rest_framework.views import APIViewfrom proprietor.fashions import Owner
from todo.fashions import Task
from todo.serializers import TaskSerializerclass TaskListView(APIView):
def get(self, request, username, format=None):
"""Get all the duties for a given person."""
proprietor = get_object_or_404(Owner, user__username=username)
duties = Task.objects.filter(proprietor=proprietor).all()
serialized = TaskSerializer(duties, many=True)
return JsonResponse()
There’s loads happening right here in somewhat little bit of code, so let’s stroll by way of it.
We begin out with the identical inheritance of the APIView
that we have been utilizing, laying the groundwork for what will likely be our view. We override the identical get
technique we have overridden earlier than, including a parameter that enables our view to obtain the username
from the incoming request.
Our get
technique will then use that username
to seize the Owner
related to that person. This get_object_or_404
perform permits us to just do that, with somewhat one thing particular added for ease of use.
It would make sense that there is no level in on the lookout for duties if the required person cannot be discovered. In truth, we would wish to return a 404 error. get_object_or_404
will get a single object based mostly on no matter standards we go in and both returns that object or raises an Http404 exception. We can set that standards based mostly on attributes of the thing. The Owner
objects are all hooked up to a User
by way of their person
attribute. We do not have a User
object to look with, although. We solely have a username
. So, we are saying to get_object_or_404
“if you search for an Owner
, test to see that the User
hooked up to it has the username
that I would like” by specifying user__username
. That’s TWO underscores. When filtering by way of a QuestionSet, the 2 underscores imply “attribute of this nested object.” Those attributes could be as deeply nested as wanted.
We now have the Owner
similar to the given username. We use that Owner
to filter by way of all of the duties, solely retrieving those it owns with Task.objects.filter
. We may’ve used the identical nested-attribute sample that we did with get_object_or_404
to drill into the User
linked to the Owner
linked to the Tasks
(duties = Task.objects.filter(owner__user__username=username).all()
) however there is no have to get that wild with it.
Task.objects.filter(proprietor=proprietor).all()
will present us with a QuestionSet
of all of the Task
objects that match our question. Great. The TaskSerializer
will then take that QuestionSet
and all its knowledge, together with the flag of many=True
to inform it as being a group of things as a substitute of only one merchandise, and return a serialized set of outcomes. Effectively a listing of dictionaries. Finally, we offer the outgoing response with the JSON-serialized knowledge and the username used for the question.
Handling the POST request
The submit
technique will look considerably totally different from what we have seen earlier than.
# nonetheless in todo/views.py
# ...different imports...
from rest_framework.parsers import JSONParser
from datetime import datetimeclass TaskListView(APIView):
def get(self, request, username, format=None):
...def submit(self, request, username, format=None):
"""Create a brand new Task."""
proprietor = get_object_or_404(Owner, user__username=username)
knowledge = JSONParser().parse(request)
knowledge['proprietor'] = proprietor.id
if knowledge['due_date']:
knowledge['due_date'] = datetime.strptime(knowledge['due_date'], '%d/%m/%Y %H:%M:%S')new_task = TaskSerializer(knowledge=knowledge)
if new_task.is_valid():
new_task.save()
return JsonResponse('msg': 'posted', standing=201)return JsonResponse(new_task.errors, standing=400)
When we obtain knowledge from the shopper, we parse it right into a dictionary utilizing JSONParser().parse(request)
. We add the proprietor to the info and format the due_date
for the duty if one exists.
Our TaskSerializer
does the heavy lifting. It first takes within the incoming knowledge and interprets it into the fields we specified on the mannequin. It then validates that knowledge to verify it suits the required fields. If the info being hooked up to the brand new Task
is legitimate, it constructs a brand new Task
object with that knowledge and commits it to the database. We then ship again an applicable “Yay! We made a new thing!” response. If not, we gather the errors that TaskSerializer
generated and ship these again to the shopper with a 400 Bad Request
standing code.
If we have been to construct out the put
view for updating a Task
, it could look similar to this. The most important distinction can be that after we instantiate the TaskSerializer
, as a substitute of simply passing within the new knowledge, we would go within the outdated object and the brand new knowledge for that object like TaskSerializer(existing_task, knowledge=knowledge)
. We’d nonetheless do the validity test and ship again the responses we wish to ship again.
Wrapping up
Django as a framework is extremely customizable, and everybody has their very own means of sewing collectively a Django venture. The means I’ve written it out right here is not essentially the precise means Django venture must be arrange; it is only a) what I am conversant in, and b) what leverages Django’s administration system. Django tasks develop in complexity as you separate ideas into their very own little silos. You do this so it is simpler for a number of individuals to contribute to the general venture with out stepping on one another’s toes.
The huge map of recordsdata that could be a Django venture, nonetheless, would not make it extra performant or naturally predisposed to a microservice structure. On the opposite, it might probably very simply grow to be a complicated monolith. That should be helpful on your venture. It may make it more durable on your venture to be manageable, particularly because it grows.
Consider your choices fastidiously and use the appropriate device for the appropriate job. For a easy venture like this, Django possible is not the appropriate device.
Django is supposed to deal with a number of units of fashions that cowl a wide range of totally different venture areas that will share some widespread floor. This venture is a small, two-model venture with a handful of routes. If we have been to construct this out extra, we would solely have seven routes and nonetheless the identical two fashions. It’s hardly sufficient to justify a full Django venture.
It can be an excellent choice if we anticipated this venture to broaden. This is just not a kind of tasks. This is selecting a flamethrower to mild a candle. It’s absolute overkill.
Still, an internet framework is an internet framework, no matter which one you utilize on your venture. It can soak up requests and reply in addition to every other, so that you do as you would like. Just concentrate on what overhead comes along with your selection of framework.
That’s it! We’ve reached the tip of this collection! I hope it has been an enlightening journey and can provide help to make extra than simply the most-familiar selection if you’re fascinated by how you can construct out your subsequent venture. Make positive to learn the documentation for every framework to broaden on something coated on this collection (as it is not even in the least complete). There’s a large world of stuff to get into for every. Happy coding!
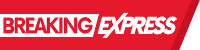