Recent developments in deep learning algorithms and efficiency have enabled researchers and firms to make big strides in areas comparable to picture recognition, speech recognition, suggestion engines, and machine translation. Six years in the past, the primary superhuman performance in visual pattern recognition was achieved. Two years in the past, the Google Brain crew unleashed TensorFlow, deftly slinging utilized deep studying to the lots. TensorFlow is outpacing many advanced instruments used for deep studying.
With TensorFlow, you will acquire entry to advanced options with huge energy. The keystone of its energy is TensorFlow’s ease of use.
In a two-part sequence, I am going to clarify the right way to shortly create a convolutional neural network for sensible picture recognition. The computation steps are embarrassingly parallel and may be deployed to carry out frame-by-frame video evaluation and prolonged for temporal-aware video evaluation.
This sequence cuts on to probably the most compelling materials. A fundamental understanding of the command line and Python is all it’s worthwhile to play alongside from residence. It goals to get you began shortly and encourage you to create your personal wonderful tasks. I will not dive into the depths of how TensorFlow works, however I am going to present loads of extra references for those who’re hungry for extra. All the libraries and instruments on this sequence are free/libre/open supply software program.
How it really works
Our objective on this tutorial is to take a novel picture that falls right into a class we have skilled and run it by a command that can inform us during which class the picture matches. We’ll observe these steps:
- Labeling is the method of curating coaching knowledge. For flowers, photographs of daisies are dragged into the “daisies” folder, roses into the “roses” folder, and so forth, for as many various flowers as desired. If we by no means label ferns, the classifier won’t ever return “ferns.” This requires many examples of every sort, so it is a vital and time-consuming course of. (We will use pre-labeled knowledge to start out, which can make this a lot faster.)
- Training is after we feed the labeled knowledge (photographs) to the mannequin. A instrument will seize a random batch of photographs, use the mannequin to guess what sort of flower is in every, check the accuracy of the guesses, and repeat till a lot of the coaching knowledge is used. The final batch of unused photographs is used to calculate the accuracy of the skilled mannequin.
- Classification is utilizing the mannequin on novel photographs. For instance, enter:
IMG207.JPG
, output:daisies
. This is the quickest and best step and is reasonable to scale.
Training and classification
In this tutorial, we’ll prepare a picture classifier to acknowledge various kinds of flowers. Deep studying requires plenty of coaching knowledge, so we’ll want a lot of sorted flower photographs. Thankfully, one other type soul has accomplished an superior job of accumulating and sorting photographs, so we’ll use this sorted knowledge set with a intelligent script that can take an current, totally skilled picture classification mannequin and retrain the final layers of the mannequin to do exactly what we wish. This method is known as switch studying.
The mannequin we’re retraining is known as Inception v3, initially specified within the December 2015 paper “Rethinking the Inception Architecture for Computer Vision.”
Inception does not know the right way to inform a tulip from a daisy till we do that coaching, which takes about 20 minutes. This is the “learning” a part of deep studying.
Installation
Step one to machine sentience: Install Docker in your platform of selection.
The first and solely dependency is Docker. This is the case in lots of TensorFlow tutorials (which ought to point out this can be a cheap approach to begin). I additionally desire this technique of putting in TensorFlow as a result of it retains your host (laptop computer or desktop) clear by not putting in a bunch of dependencies.
Bootstrap TensorFlow
With Docker put in, we’re prepared to fireside up a TensorFlow container for coaching and classification. Create a working listing someplace in your arduous drive with 2 gigabytes of free area. Create a subdirectory referred to as native
and be aware the total path to that listing.
docker run -v /path/to/native:/notebooks/native --rm -it --name tensorflow
tensorflow/tensorflow:nightly /bin/bash
Here’s a breakdown of that command.
-v /path/to/native:/notebooks/native
mounts thenative
listing you simply created to a handy place within the container. If utilizing RHEL, Fedora, or one other SELinux-enabled system, append:Z
to this to permit the container to entry the listing.--rm
tells Docker to delete the container after we’re accomplished.-it
attaches our enter and output to make the container interactive.--name tensorflow
offers our container the identifytensorflow
as a substitute ofsneaky_chowderhead
or no matter random identify Docker may decide for us.tensorflow/tensorflow:nightly
says run thenightly
picture oftensorflow/tensorflow
from Docker Hub (a public picture repository) as a substitute of newest (by default, probably the most lately constructed/out there picture). We are utilizing nightly as a substitute of newest as a result of (on the time of writing) newest comprises a bug that breaks TensorBoard, an information visualization instrument we’ll discover helpful later./bin/bash
says do not run the default command; run a Bash shell as a substitute.
Train the mannequin
Inside the container, run these instructions to obtain and sanity test the coaching knowledge.
curl -O http://obtain.tensorflow.org/example_images/flower_photos.tgz
echo 'db6b71d5d3afff90302ee17fd1fefc11d57f243f flower_photos.tgz' | sha1sum -c
If you do not see the message flower_photos.tgz: OK
, you do not have the proper file. If the above curl
or sha1sum
steps fail, manually obtain and explode the training data tarball (SHA-1 checksum: db6b71d5d3afff90302ee17fd1fefc11d57f243f
) within the native
listing in your host.
Now put the coaching knowledge in place, then obtain and sanity test the retraining script.
mv flower_photos.tgz native/
cd native
curl -O https://uncooked.githubusercontent.com/tensorflow/tensorflow/10cf65b48e1b2f16eaa82
6d2793cb67207a085d0/tensorflow/examples/image_retraining/retrain.py
echo 'a74361beb4f763dc2d0101cfe87b672ceae6e2f5 retrain.py' | sha1sum -c
Look for affirmation that retrain.py
has the proper contents. You ought to see retrain.py: OK
.
Finally, it is time to be taught! Run the retraining script.
python retrain.py --image_dir flower_photos --output_graph output_graph.pb
--output_labels output_labels.txt
If you encounter this error, ignore it:TypeError: not all arguments transformed throughout string formatting Logged from file
.
tf_logging.py, line 82
As retrain.py
proceeds, the coaching photographs are routinely separated into batches of training, test, and validation data sets.
In the output, we’re hoping for prime “Train accuracy” and “Validation accuracy” and low “Cross entropy.” See How to retrain Inception’s final layer for new categories for an in depth rationalization of those phrases. Expect coaching to take round 30 minutes on trendy .
Pay consideration to the final line of output in your console:
INFO:tensorflow:Final check accuracy = 89.1% (N=340)
This says we have a mannequin that can, 9 instances out of 10, accurately guess which one in all 5 potential flower sorts is proven in a given picture. Your accuracy will seemingly differ due to randomness injected into the coaching course of.
Classify
With yet another small script, we will feed new flower photographs to the mannequin and it will output its guesses. This is picture classification.
Save the next as classify.py
within the native
listing in your host:
import tensorflow as tf, sys
image_path = sys.argv[1]
graph_path = 'output_graph.pb'
labels_path = 'output_labels.txt'
# Read within the image_data
image_data = tf.gfile.FastGFile(image_path, 'rb').learn()
# Loads label file, strips off carriage return
label_lines = [line.rstrip() for line
in tf.gfile.GFile(labels_path)]
# Unpersists graph from file
with tf.gfile.FastGFile(graph_path, 'rb') as f:
graph_def = tf.GraphDef()
graph_def.ParseFromString(f.learn())
_ = tf.import_graph_def(graph_def, identify='')
# Feed the image_data as enter to the graph and get first prediction
with tf.Session() as sess:
softmax_tensor = sess.graph.get_tensor_by_name('final_result:zero')
predictions = sess.run(softmax_tensor,
'DecodeJpeg/contents:zero': image_data)
# Sort to point out labels of first prediction so as of confidence
top_k = predictions[zero].argsort()[-len(predictions[zero]):][::-1]
for node_id in top_k:
human_string = label_lines[node_id]
rating = predictions[zero][node_id]
print('%s (rating = %.5f)' % (human_string, rating))
To check your personal picture, put it aside as check.jpg
in your native
listing and run (within the container) python classify.py check.jpg
. The output will look one thing like this:
sunflowers (rating = zero.78311)
daisy (rating = zero.20722)
dandelion (rating = zero.00605)
tulips (rating = zero.00289)
roses (rating = zero.00073)
The numbers point out confidence. The mannequin is 78.311% certain the flower within the picture is a sunflower. The next rating signifies a extra seemingly match. Note that there can be only one match. Multi-label classification requires a different approach.
For extra element, view this great line-by-line explanation of classify.py
.
The graph loading code within the classifier script was damaged, so I utilized the graph_def = tf.GraphDef()
, and so forth. graph loading code.
With zero rocket science and a handful of code, we have created an honest flower picture classifier that may course of about 5 photographs per second on an off-the-shelf laptop computer laptop.
In the second a part of this sequence, which publishes subsequent week, we’ll use this info to coach a distinct picture classifier, then have a look underneath the hood with TensorBoard. If you wish to check out TensorBoard, preserve this container operating by making certain docker run
isn’t terminated.
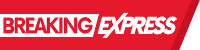