In my first article in this series, I defined find out how to use Python to create a easy, text-based cube sport. This time, I am going to show find out how to use the Python module Pygame to create a graphical sport. It will take a number of articles to get a sport that really does something, however by the tip of the collection, you should have a greater understanding of find out how to discover and study new Python modules and find out how to construct an software from the bottom up.
Before you begin, it’s essential to set up Pygame.
Installing new Python modules
There are a number of methods to put in Python modules, however the two most typical are:
- From your distribution’s software program repository
- Using the Python package deal supervisor, pip
Both strategies work nicely, and every has its personal set of benefits. If you are creating on Linux or BSD, leveraging your distribution’s software program repository ensures automated and well timed updates.
However, utilizing Python’s built-in package deal supervisor provides you management over when modules are up to date. Also, it isn’t OS-specific, that means you should utilize it even if you’re not in your common improvement machine. Another benefit of pip is that it permits native installs of modules, which is useful if you do not have administrative rights to a pc you are utilizing.
Using pip
If you’ve each Python and Pythonthree put in in your system, the command you wish to use might be pip3
, which differentiates it from Python 2.x’s pip
command. If you are uncertain, attempt pip3
first.
The pip
command works lots like most Linux package deal managers. You can seek for Python modules with search
, then set up them with set up
. If you do not have permission to put in software program on the pc you are utilizing, you should utilize the --user
choice to only set up the module into your own home listing.
$ pip3 search pygame
[...]
Pygame (1.9.three) - Python Game Development
sge-pygame (1.5) - A 2-D sport engine for Python
pygame_camera (zero.1.1) - A Camera lib for PyGame
pygame_cffi (zero.2.1) - A cffi-based SDL wrapper that copies the pygame API.
[...]
$ pip3 set up Pygame --consumer
Pygame is a Python module, which implies that it is only a set of libraries that can be utilized in your Python packages. In different phrases, it is not a program that you just launch, like IDLE or Ninja-IDE are.
Getting began with Pygame
A online game wants a setting; a world through which it takes place. In Python, there are two alternative ways to create your setting:
- Set a background colour
- Set a background picture
Your background is simply a picture or a colour. Your online game characters cannot work together with issues within the background, so do not put something too essential again there. It’s simply set dressing.
Setting up your Pygame script
To begin a brand new Pygame challenge, create a folder in your laptop. All your sport recordsdata go into this listing. It’s vitally essential that you just preserve all of the recordsdata wanted to run your sport inside your challenge folder.
A Python script begins with the file sort, your identify, and the license you wish to use. Use an open supply license so your folks can enhance your sport and share their adjustments with you:
#!/usr/bin/env python3
# by Seth Kenlon## GPLv3
# This program is free software program: you possibly can redistribute it and/or
# modify it beneath the phrases of the GNU General Public License as
# printed by the Free Software Foundation, both model three of the
# License, or (at your choice) any later model.
#
# This program is distributed within the hope that it will likely be helpful, however
# WITHOUT ANY WARRANTY; with out even the implied guarantee of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
# General Public License for extra particulars.
#
# You ought to have acquired a duplicate of the GNU General Public License
# together with this program. If not, see <http://www.gnu.org/licenses/>.
Then you inform Python what modules you wish to use. Some of the modules are frequent Python libraries, and naturally, you wish to embrace the one you simply put in, Pygame.
import pygame # load pygame key phrases
import sys # let python use your file system
import os # assist python determine your OS
Since you will be working lots with this script file, it helps to make sections inside the file so the place to place stuff. You do that with block feedback, that are feedback which might be seen solely when your supply code. Create three blocks in your code.
'''
Objects
'''# put Python lessons and features right here
'''
Setup
'''# put run-once code right here
'''
Main Loop
'''# put sport loop right here
Next, set the window measurement to your sport. Keep in thoughts that not everybody has a giant laptop display screen, so it is best to make use of a display screen measurement that matches on most individuals’s computer systems.
There is a method to toggle full-screen mode, the way in which many fashionable video video games do, however because you’re simply beginning out, preserve it easy and simply set one measurement.
'''
Setup
'''
worldx = 960
worldy = 720
The Pygame engine requires some fundamental setup earlier than you should utilize it in a script. You should set the body price, begin its inner clock, and begin (init
) Pygame.
fps = 40 # body price
ani = four # animation cycles
clock = pygame.time.Clock()
pygame.init()
Now you possibly can set your background.
Setting the background
Before you proceed, open a graphics software and create a background to your sport world. Save it as stage.png
inside a folder referred to as photos
in your challenge listing.
There are a number of free graphics functions you should utilize.
- Krita is a professional-level paint supplies emulator that can be utilized to create lovely photos. If you are very concerned about creating artwork for video video games, you possibly can even buy a collection of on-line game art tutorials.
- Pinta is a fundamental, simple to study paint software.
- Inkscape is a vector graphics software. Use it to attract with shapes, strains, splines, and Bézier curves.
Your graphic would not need to be advanced, and you’ll at all times return and alter it later. Once you’ve it, add this code within the setup part of your file:
world = pygame.show.set_mode([worldx,worldy])
backdrop = pygame.picture.load(os.path.be a part of('photos','stage.png').convert()
backdropbox = world.get_rect()
If you are simply going to fill the background of your sport world with a colour, all you want is:
world = pygame.show.set_mode([worldx,worldy])
You additionally should outline a colour to make use of. In your setup part, create some colour definitions utilizing values for crimson, inexperienced, and blue (RGB).
'''
Setup
'''BLUE = (25,25,200)
BLACK = (23,23,23 )
WHITE = (254,254,254)
At this level, you might theoretically begin your sport. The downside is, it might solely final for a millisecond.
To show this, save your file as your-name_game.py
(change your-name
along with your precise identify). Then launch your sport.
If you’re utilizing IDLE, run your sport by choosing Run Module
from the Run menu.
If you’re utilizing Ninja, click on the Run file
button within the left button bar.
You may run a Python script straight from a Unix terminal or a Windows command immediate.
$ python3 ./your-name_game.py
If you are utilizing Windows, use this command:
py.exe your-name_game.py
However you launch it, do not count on a lot, as a result of your sport solely lasts a couple of milliseconds proper now. You can repair that within the subsequent part.
Looping
Unless informed in any other case, a Python script runs as soon as and solely as soon as. Computers are very quick today, so your Python script runs in lower than a second.
To pressure your sport to remain open and energetic lengthy sufficient for somebody to see it (not to mention play it), use a whereas
loop. To make your sport stay open, you possibly can set a variable to some worth, then inform a whereas
loop to maintain looping for so long as the variable stays unchanged.
This is usually referred to as a “main loop,” and you should utilize the time period major
as your variable. Add this wherever in your setup part:
major = True
During the primary loop, use Pygame key phrases to detect if keys on the keyboard have been pressed or launched. Add this to your major loop part:
'''
Main loop
'''
whereas major == True:
for occasion in pygame.occasion.get():
if occasion.sort == pygame.QUIT:
pygame.stop(); sys.exit()
major = Falseif occasion.sort == pygame.KEYDOWN:
if occasion.key == ord('q'):
pygame.stop()
sys.exit()
major = False
Also in your major loop, refresh your world’s background.
If you’re utilizing a picture for the background:
world.blit(backdrop, backdropbox)
If you’re utilizing a colour for the background:
world.fill(BLUE)
Finally, inform Pygame to refresh every part on the display screen and advance the sport’s inner clock.
pygame.show.flip()
clock.tick(fps)
Save your file, and run it once more to see probably the most boring sport ever created.
To stop the sport, press q
in your keyboard.
In the next article of this collection, I am going to present you find out how to add to your at present empty sport world, so go forward and begin creating some graphics to make use of!
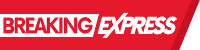