The oldest joke in open supply software program is the assertion that “the code is self-documenting.” Experience exhibits that studying the supply is akin to listening to the climate forecast: wise folks nonetheless go exterior and examine the sky. What follows are some recommendations on how you can examine and observe Linux programs at boot by leveraging information of acquainted debugging instruments. Analyzing the boot processes of programs which can be functioning effectively prepares customers and builders to cope with the inevitable failures.
In some methods, the boot course of is surprisingly easy. The kernel begins up single-threaded and synchronous on a single core and appears virtually understandable to the pitiful human thoughts. But how does the kernel itself get began? What capabilities do initrd
(initial ramdisk) and bootloaders carry out? And wait, why is the LED on the Ethernet port all the time on?
Read on for solutions to those and different questions; the code for the described demos and exercises can be obtainable on GitHub.
The starting of boot: the OFF state
Wake-on-LAN
The OFF state implies that the system has no energy, proper? The obvious simplicity is misleading. For instance, the Ethernet LED is illuminated as a result of wake-on-LAN (WOL) is enabled in your system. Check whether or not that is the case by typing:
$# sudo ethtool <interface title>
the place <interface title>
could be, for instance, eth0
. (ethtool
is present in Linux packages of the identical title.) If “Wake-on” within the output exhibits g
, distant hosts can boot the system by sending a MagicPacket. If you haven’t any intention of waking up your system remotely and don’t want others to take action, flip WOL off both within the system BIOS menu, or by way of:
$# sudo ethtool -s <interface title> wol d
The processor that responds to the MagicPacket could also be a part of the community interface or it could be the Baseboard Management Controller (BMC).
Intel Management Engine, Platform Controller Hub, and Minix
The BMC isn’t the one microcontroller (MCU) that could be listening when the system is nominally off. x86_64 programs additionally embody the Intel Management Engine (IME) software program suite for distant administration of programs. All kinds of units, from servers to laptops, contains this expertise, which enables functionality akin to KVM Remote Control and Intel Capability Licensing Service. The IME has unpatched vulnerabilities, in keeping with Intel’s own detection tool. The unhealthy information is, it is tough to disable the IME. Trammell Hudson has created an me_cleaner project that wipes a few of the extra egregious IME elements, just like the embedded net server, however might additionally brick the system on which it’s run.
The IME firmware and the System Management Mode (SMM) software program that follows it at boot are based on the Minix operating system and run on the separate Platform Controller Hub processor, not the principle system CPU. The SMM then launches the Universal Extensible Firmware Interface (UEFI) software program, about which a lot has already been written, on the principle processor. The Coreboot group at Google has began a breathtakingly formidable Non-Extensible Reduced Firmware (NERF) venture that goals to interchange not solely UEFI however early Linux userspace elements akin to systemd. While we await the end result of those new efforts, Linux customers could now buy laptops from Purism, System76, or Dell with IME disabled, plus we will hope for laptops with ARM 64-bit processors.
Bootloaders
Besides beginning buggy adware, what operate does early boot firmware serve? The job of a bootloader is to make obtainable to a newly powered processor the sources it must run a general-purpose working system like Linux. At power-on, there not solely is not any digital reminiscence, however no DRAM till its controller is introduced up. A bootloader then activates energy provides and scans buses and interfaces with a view to find the kernel picture and the basis filesystem. Popular bootloaders like U-Boot and GRUB have help for acquainted interfaces like USB, PCI, and NFS, in addition to extra embedded-specific units like NOR- and NAND-flash. Bootloaders additionally work together with hardware safety units like Trusted Platform Modules (TPMs) to determine a series of belief from earliest boot.
The open supply, extensively used U-Boot bootloader is supported on programs starting from Raspberry Pi to Nintendo units to automotive boards to Chromebooks. There is not any syslog, and when issues go sideways, usually not even any console output. To facilitate debugging, the U-Boot crew presents a sandbox during which patches could be examined on the build-host, and even in a nightly Continuous Integration system. Playing with U-Boot’s sandbox is comparatively easy on a system the place frequent growth instruments like Git and the GNU Compiler Collection (GCC) are put in:
$# git clone git://git.denx.de/u-boot; cd u-boot
$# make ARCH=sandbox defconfig
$# make; ./u-boot
=> printenv
=> assist
That’s it: you are working U-Boot on x86_64 and may take a look at tough options like mock storage device repartitioning, TPM-based secret-key manipulation, and hotplug of USB units. The U-Boot sandbox may even be single-stepped below the GDB debugger. Development utilizing the sandbox is 10x sooner than testing by reflashing the bootloader onto a board, and a “bricked” sandbox could be recovered with Ctrl+C.
Starting up the kernel
Provisioning a booting kernel
Upon completion of its duties, the bootloader will execute a leap to kernel code that it has loaded into important reminiscence and start execution, passing alongside any command-line choices that the consumer has specified. What sort of program is the kernel? file /boot/vmlinuz
signifies that it’s a bzImage, which means a giant compressed one. The Linux supply tree comprises an extract-vmlinux tool that can be utilized to uncompress the file:
$# scripts/extract-vmlinux /boot/vmlinuz-$(uname -r) > vmlinux
$# file vmlinux
vmlinux: ELF 64-bit LSB executable, x86-64, model 1 (SYSV), statically
linked, stripped
The kernel is an Executable and Linking Format (ELF) binary, like Linux userspace applications. That means we will use instructions from the binutils
package deal like readelf
to examine it. Compare the output of, for instance:
$# readelf -S /bin/date
$# readelf -S vmlinux
The checklist of sections within the binaries is essentially the identical.
So the kernel should begin up one thing like different Linux ELF binaries … however how do userspace applications really begin? In the important()
operate, proper? Not exactly.
Before the important()
operate can run, applications want an execution context that features heap and stack reminiscence plus file descriptors for stdio
, stdout
, and stderr
. Userspace applications receive these sources from the usual library, which is glibc
on most Linux programs. Consider the next:
$# file /bin/date
/bin/date: ELF 64-bit LSB shared object, x86-64, model 1 (SYSV), dynamically
linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 2.6.32,
BuildID[sha1]=14e8563676febeb06d701dbee35d225c5a8e565a,
stripped
ELF binaries have an interpreter, simply as Bash and Python scripts do, however the interpreter needn’t be specified with #!
as in scripts, as ELF is Linux’s native format. The ELF interpreter provisions a binary with the wanted sources by calling _start()
, a operate obtainable from the glibc
supply package deal that may be inspected via GDB. The kernel clearly has no interpreter and should provision itself, however how?
Inspecting the kernel’s startup with GDB offers the reply. First set up the debug package deal for the kernel that comprises an unstripped model of vmlinux
, for instance apt-get set up linux-image-amd64-dbg
, or compile and set up your personal kernel from supply, for instance, by following directions within the wonderful Debian Kernel Handbook. gdb vmlinux
adopted by data recordsdata
exhibits the ELF part init.textual content
. List the beginning of program execution in init.textual content
with l *(deal with)
, the place deal with
is the hexadecimal begin of init.textual content
. GDB will point out that the x86_64 kernel begins up within the kernel’s file arch/x86/kernel/head_64.S, the place we discover the meeting operate start_cpu0()
and code that explicitly creates a stack and decompresses the zImage earlier than calling the x86_64 start_kernel()
operate. ARM 32-bit kernels have the same arch/arm/kernel/head.S. start_kernel()
isn’t architecture-specific, so the operate lives within the kernel’s init/main.c. start_kernel()
is arguably Linux’s true important()
operate.
From start_kernel() to PID 1
The kernel’s hardware manifest: the device-tree and ACPI tables
At boot, the kernel wants details about the hardware past the processor kind for which it has been compiled. The directions within the code are augmented by configuration information that’s saved individually. There are two important strategies of storing this information: device-trees and ACPI tables. The kernel learns what hardware it should run at every boot by studying these recordsdata.
For embedded units, the device-tree is a manifest of put in hardware. The device-tree is just a file that’s compiled concurrently kernel supply and is often positioned in /boot
alongside vmlinux
. To see what’s within the binary device-tree on an ARM machine, simply use the strings
command from the binutils
package deal on a file whose title matches /boot/*.dtb
, as dtb
refers to a device-tree binary. Clearly the device-tree could be modified just by enhancing the JSON-like recordsdata that compose it and rerunning the particular dtc
compiler that is supplied with the kernel supply. While the device-tree is a static file whose file path is often handed to the kernel by the bootloader on the command line, a device-tree overlay facility has been added lately, the place the kernel can dynamically load further fragments in response to hotplug occasions after boot.
x86-family and plenty of enterprise-grade ARM64 units make use of the choice Advanced Configuration and Power Interface (ACPI) mechanism. In distinction to the device-tree, the ACPI data is saved within the /sys/firmware/acpi/tables
digital filesystem that’s created by the kernel at boot by accessing onboard ROM. The straightforward strategy to learn the ACPI tables is with the acpidump
command from the acpica-tools
package deal. Here’s an instance:
Yes, your Linux system is prepared for Windows 2001, must you care to put in it. ACPI has each strategies and information, not like the device-tree, which is extra of a hardware-description language. ACPI strategies proceed to be energetic post-boot. For instance, beginning the command acpi_listen
(from package deal apcid
) and opening and shutting the laptop computer lid will present that ACPI performance is working on a regular basis. While quickly and dynamically overwriting the ACPI tables is feasible, completely altering them entails interacting with the BIOS menu at boot or reflashing the ROM. If you are going to that a lot hassle, maybe it is best to simply install coreboot, the open supply firmware alternative.
From start_kernel() to userspace
The code in init/main.c is surprisingly readable and, amusingly, nonetheless carries Linus Torvalds’ unique copyright from 1991-1992. The traces present in dmesg | head
on a newly booted system originate largely from this supply file. The first CPU is registered with the system, international information buildings are initialized, and the scheduler, interrupt handlers (IRQs), timers, and console are introduced one-by-one, in strict order, on-line. Until the operate timekeeping_init()
runs, all timestamps are zero. This a part of the kernel initialization is synchronous, which means that execution happens in exactly one thread, and no operate is executed till the final one completes and returns. As a end result, the dmesg
output can be fully reproducible, even between two programs, so long as they’ve the identical device-tree or ACPI tables. Linux is behaving like one of many RTOS (real-time working programs) that runs on MCUs, for instance QNX or VxWorks. The scenario persists into the operate rest_init()
, which known as by start_kernel()
at its termination.
The fairly humbly named rest_init()
spawns a brand new thread that runs kernel_init()
, which invokes do_initcalls()
. Users can spy on initcalls
in motion by appending initcall_debug
to the kernel command line, leading to dmesg
entries each time an initcall
operate runs. initcalls
cross by means of seven sequential ranges: early, core, postcore, arch, subsys, fs, machine, and late. The most user-visible a part of the initcalls
is the probing and setup of all of the processors’ peripherals: buses, community, storage, shows, and so on., accompanied by the loading of their kernel modules. rest_init()
additionally spawns a second thread on the boot processor that begins by working cpu_idle()
whereas it waits for the scheduler to assign it work.
kernel_init()
additionally sets up symmetric multiprocessing (SMP). With more moderen kernels, discover this level in dmesg
output by searching for “Bringing up secondary CPUs…” SMP proceeds by “hotplugging” CPUs, which means that it manages their lifecycle with a state machine that’s notionally just like that of units like hotplugged USB sticks. The kernel’s power-management system regularly takes particular person cores offline, then wakes them as wanted, in order that the identical CPU hotplug code known as again and again on a machine that’s not busy. Observe the power-management system’s invocation of CPU hotplug with the BCC tool known as offcputime.py
.
Note that the code in init/important.c
is sort of completed executing when smp_init()
runs: The boot processor has accomplished many of the one-time initialization that the opposite cores needn’t repeat. Nonetheless, the per-CPU threads have to be spawned for every core to handle interrupts (IRQs), workqueues, timers, and energy occasions on every. For instance, see the per-CPU threads that service softirqs and workqueues in motion by way of the ps -o psr
command.
$# ps -o pid,psr,comm $(pgrep ksoftirqd)
PID PSR COMMAND
7 zero ksoftirqd/zero
16 1 ksoftirqd/1
22 2 ksoftirqd/2
28 three ksoftirqd/three$# ps -o pid,psr,comm $(pgrep kworker)
PID PSR COMMAND
four zero kworker/zero:0H
18 1 kworker/1:0H
24 2 kworker/2:0H
30 three kworker/three:0H
[ . . . ]
the place the PSR area stands for “processor.” Each core should additionally host its personal timers and cpuhp
hotplug handlers.
How is it, lastly, that userspace begins? Near its finish, kernel_init()
appears for an initrd
that may execute the init
course of on its behalf. If it finds none, the kernel immediately executes init
itself. Why then may one need an initrd
?
Early userspace: who ordered the initrd?
Besides the device-tree, one other file path that’s optionally supplied to the kernel at boot is that of the initrd
. The initrd
usually lives in /boot
alongside the bzImage file vmlinuz on x86, or alongside the same uImage and device-tree for ARM. List the contents of the initrd
with the lsinitramfs
device that’s a part of the initramfs-tools-core
package deal. Distro initrd
schemes comprise minimal /bin
, /sbin
, and /and so on
directories together with kernel modules, plus some recordsdata in /scripts
. All of those ought to look fairly acquainted, because the initrd
for probably the most half is just a minimal Linux root filesystem. The obvious similarity is a bit misleading, as almost all of the executables in /bin
and /sbin
contained in the ramdisk are symlinks to the BusyBox binary, leading to /bin
and /sbin
directories which can be 10x smaller than glibc’s.
Why trouble to create an initrd
if all it does is load some modules after which begin init
on the common root filesystem? Consider an encrypted root filesystem. The decryption could depend on loading a kernel module that’s saved in /lib/modules
on the basis filesystem … and, unsurprisingly, within the initrd
as effectively. The crypto module might be statically compiled into the kernel as an alternative of loaded from a file, however there are numerous causes for not wanting to take action. For instance, statically compiling the kernel with modules might make it too giant to suit on the obtainable storage, or static compilation could violate the phrases of a software program license. Unsurprisingly, storage, community, and human enter machine (HID) drivers might also be current within the initrd
—mainly any code that’s not a part of the kernel correct that’s wanted to mount the basis filesystem. The initrd
can be a spot the place customers can stash their very own custom ACPI desk code.
initrd
‘s are additionally nice for testing filesystems and data-storage units themselves. Stash these take a look at instruments within the initrd
and run your checks from reminiscence fairly than from the item below take a look at.
At final, when init
runs, the system is up! Since the secondary processors are actually working, the machine has turn out to be the asynchronous, preemptible, unpredictable, high-performance creature we all know and love. Indeed, ps -o pid,psr,comm -p 1
is liable to indicate that userspace’s init
course of is not working on the boot processor.
Summary
The Linux boot course of sounds forbidding, contemplating the variety of completely different items of software program that take part even on easy embedded units. Looked at in a different way, the boot course of is fairly easy, because the bewildering complexity attributable to options like preemption, RCU, and race situations are absent in boot. Focusing on simply the kernel and PID 1 overlooks the massive quantity of labor that bootloaders and subsidiary processors could do in getting ready the platform for the kernel to run. While the kernel is definitely distinctive amongst Linux applications, some perception into its construction could be gleaned by making use of to it a few of the identical instruments used to examine different ELF binaries. Studying the boot course of whereas it is working effectively arms system maintainers for failures after they come.
To be taught extra, attend Alison Chaiken’s discuss, Linux: The first second, at linux.conf.au, which can be held January 22-26 in Sydney.
Thanks to Akkana Peck for initially suggesting this matter and for a lot of corrections.
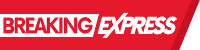