I journey so much on enterprise. I am not a lot of a automotive man, so when I’ve some free time, I desire to stroll or bike round a metropolis. Many of the cities I’ve visited on enterprise have bikeshare methods, which allow you to lease a motorbike for a number of hours. Most of those methods have an app to assist customers find and lease their bikes, however it might be extra useful for customers like me to have a single place to get data on all of the bikes in a metropolis which might be out there to lease.
To resolve this downside and reveal the ability of open supply so as to add location-aware options to an online software, I mixed publicly out there bikeshare information, the Python programming language, and the open supply Redis in-memory information construction server to index and question geospatial information.
The ensuing bikeshare software incorporates information from many various sharing methods, together with the Citi Bike bikeshare in New York City. It takes benefit of the General Bikeshare Feed supplied by the Citi Bike system and makes use of its information to reveal a few of the options that may be constructed utilizing Redis to index geospatial information. The Citi Bike information is supplied underneath the Citi Bike data license agreement.
General Bikeshare Feed Specification
The General Bikeshare Feed Specification (GBFS) is an open data specification developed by the North American Bikeshare Association to make it simpler for map and transportation purposes so as to add bikeshare methods into their platforms. The specification is at the moment in use by over 60 completely different sharing methods on this planet.
The feed consists of a number of easy JSON information information containing details about the state of the system. The feed begins with a top-level JSON file referencing the URLs of the sub-feed information:
"data": ,
"last_updated": 1506370010,
"ttl": 10
The first step is loading details about the bikesharing stations into Redis utilizing information from the system_information
and station_information
feeds.
The system_information
feed gives the system ID, which is a brief code that can be utilized to create namespaces for Redis keys. The GBFS spec would not specify the format of the system ID, however does assure it’s globally distinctive. Many of the bikeshare feeds use brief names like coast_bike_share, boise_greenbike, or topeka_metro_bikes for system IDs. Others use acquainted geographic abbreviations akin to NYC or BA, and one makes use of a universally distinctive identifier (UUID). The bikesharing software makes use of the identifier as a prefix to assemble distinctive keys for the given system.
The station_information
feed gives static details about the sharing stations that comprise the system. Stations are represented by JSON objects with a number of fields. There are a number of necessary fields within the station object that present the ID, title, and site of the bodily bike stations. There are additionally a number of non-obligatory fields that present useful data akin to the closest cross avenue or accepted fee strategies. This is the first supply of knowledge for this a part of the bikesharing software.
Building the database
I’ve written a pattern software, load_station_data.py, that mimics what would occur in a backend course of for loading information from exterior sources.
Finding the bikeshare stations
Loading the bikeshare information begins with the systems.csv file from the GBFS repository on GitHub.
The repository’s systems.csv file gives the invention URL for registered bikeshare methods with an out there GBFS feed. The discovery URL is the start line for processing bikeshare data.
The load_station_data
software takes every discovery URL discovered within the methods file and makes use of it to seek out the URL for 2 sub-feeds: system data and station data. The system data feed gives a key piece of knowledge: the distinctive ID of the system. (Note: the system ID can be supplied within the methods.csv file, however a few of the identifiers in that file don’t match the identifiers within the feeds, so I at all times fetch the identifier from the feed.) Details on the system, like bikeshare URLs, telephone numbers, and emails, could possibly be added in future variations of the appliance, so the information is saved in a Redis hash utilizing the important thing $system_id:system_info
.
Loading the station information
The station data gives information about each station within the system, together with the system’s location. The load_station_data
software iterates over each station within the station feed and shops the information about every right into a Redis hash utilizing a key of the shape $system_id:station:$station_id
. The location of every station is added to a geospatial index for the bikeshare utilizing the GEOADD
command.
Updating information
On subsequent runs, I do not need the code to take away all of the feed information from Redis and reload it into an empty Redis database, so I rigorously thought-about learn how to deal with in-place updates of the information.
The code begins by loading the dataset with data on all of the bikesharing stations for the system being processed into reminiscence. When data is loaded for a station, the station (by key) is faraway from the in-memory set of stations. Once all station information is loaded, we’re left with a set containing all of the station information that should be eliminated for that system.
The software iterates over this set of stations and creates a transaction to delete the station data, take away the station key from the geospatial indexes, and take away the station from the listing of stations for the system.
Notes on the code
There are a number of attention-grabbing issues to notice in the sample code. First, objects are added to the geospatial indexes utilizing the GEOADD
command however eliminated with the ZREM
command. As the underlying implementation of the geospatial sort makes use of sorted units, objects are eliminated utilizing ZREM
. A phrase of warning: For simplicity, the pattern code demonstrates working with a single Redis node; the transaction blocks would have to be restructured to run in a cluster setting.
If you might be utilizing Redis four.zero (or later), you’ve got some alternate options to the DELETE
and HMSET
instructions within the code. Redis four.zero gives the UNLINK
command as an asynchronous various to the DELETE
command. UNLINK
will take away the important thing from the keyspace, nevertheless it reclaims the reminiscence in a separate thread. The HMSET
command is deprecated in Redis 4.0 and the HSET
command is now variadic (that’s, it accepts an indefinite variety of arguments).
Notifying shoppers
At the tip of the method, a notification is distributed to the shoppers counting on our information. Using the Redis pub/sub mechanism, the notification goes out over the geobike:station_changed
channel with the ID of the system.
Data mannequin
When structuring information in Redis, an important factor to consider is how you’ll question the data. The two important queries the bikeshare software must assist are:
- Find stations close to us
- Display details about stations
Redis gives two important information sorts that can be helpful for storing our information: hashes and sorted units. The hash type maps effectively to the JSON objects that symbolize stations; since Redis hashes do not implement a schema, they can be utilized to retailer the variable station data.
Of course, discovering stations geographically requires a geospatial index to seek for stations relative to some coordinates. Redis gives several commands to construct up a geospatial index utilizing the sorted set information construction.
We assemble keys utilizing the format $system_id:station:$station_id
for the hashes containing details about the stations and keys utilizing the format $system_id:stations:location
for the geospatial index used to seek out stations.
Getting the consumer’s location
The subsequent step in constructing out the appliance is to find out the consumer’s present location. Most purposes accomplish this by means of built-in providers supplied by the working system. The OS can present purposes with a location based mostly on GPS hardware constructed into the gadget or approximated from the gadget’s out there WiFi networks.
Finding stations
After the consumer’s location is discovered, the subsequent step is finding close by bikesharing stations. Redis’ geospatial capabilities can return data on stations inside a given distance of the consumer’s present coordinates. Here’s an instance of this utilizing the Redis command-line interface.
Imagine I am on the Apple Store on Fifth Avenue in New York City, and I need to head downtown to Mood on West 37th to meet up with my buddy Swatch. I might take a taxi or the subway, however I would slightly bike. Are there any close by sharing stations the place I might get a motorbike for my journey?
The Apple retailer is situated at 40.76384, -73.97297. According to the map, two bikeshare stations—Grand Army Plaza & Central Park South and East 58th St. & Madison—fall inside a 500-foot radius (in blue on the map above) of the shop.
I can use Redis’ GEORADIUS
command to question the NYC system index for stations inside a 500-foot radius:
127.zero.zero.1:6379> GEORADIUS NYC:stations:location -73.97297 40.76384 500 ft
1) "NYC:station:3457"
2) "NYC:station:281"
Redis returns the 2 bikeshare areas discovered inside that radius, utilizing the weather in our geospatial index because the keys for the metadata a couple of explicit station. The subsequent step is trying up the names for the 2 stations:
127.zero.zero.1:6379> hget NYC:station:281 title
"Grand Army Plaza & Central Park S"
127.zero.zero.1:6379> hget NYC:station:3457 title
"E 58 St & Madison Ave"
Those keys correspond to the stations recognized on the map above. If I would like, I can add extra flags to the GEORADIUS
command to get a listing of components, their coordinates, and their distance from our present level:
127.zero.zero.1:6379> GEORADIUS NYC:stations:location -73.97297 40.76384 500 ft WITHDIST WITHCOORD ASC
1) 1) "NYC:station:281"
2) "289.1995"
three) 1) "-73.97371262311935425"
2) "40.76439830559216659"
2) 1) "NYC:station:3457"
2) "383.1782"
three) 1) "-73.97209256887435913"
2) "40.76302702144496237"
Looking up the names related to these keys generates an ordered listing of stations I can select from. Redis would not present instructions or routing functionality, so I exploit the routing options of my gadget’s OS to plot a course from my present location to the chosen bike station.
The GEORADIUS
operate will be simply carried out inside an API in your favourite improvement framework so as to add location performance to an app.
Other question instructions
In addition to the GEORADIUS
command, Redis gives three different instructions for querying information from the index: GEOPOS
, GEODIST
, and GEORADIUSBYMEMBER
.
The GEOPOS
command can present the coordinates for a given component from the geohash. For instance, if I do know there’s a bikesharing station at West 38th and eighth and its ID is 523, then the component title for that station is NYC:station:523. Using Redis, I can discover the station’s longitude and latitude:
127.zero.zero.1:6379> geopos NYC:stations:location NYC:station:523
1) 1) "-73.99138301610946655"
2) "40.75466497634030105"
The GEODIST
command gives the gap between two components of the index. If I wished to seek out the gap between the station at Grand Army Plaza & Central Park South and the station at East 58th St. & Madison, I’d problem the next command:
127.zero.zero.1:6379> GEODIST NYC:stations:location NYC:station:281 NYC:station:3457 ft
"671.4900"
Finally, the GEORADIUSBYMEMBER
command is just like the GEORADIUS
command, however as a substitute of taking a set of coordinates, the command takes the title of one other member of the index and returns all of the members inside a given radius centered on that member. To discover all of the stations inside 1,000 toes of the Grand Army Plaza & Central Park South, enter the next:
127.zero.zero.1:6379> GEORADIUSBYMEMBER NYC:stations:location NYC:station:281 1000 ft WITHDIST
1) 1) "NYC:station:281"
2) "0.0000"
2) 1) "NYC:station:3132"
2) "793.4223"
three) 1) "NYC:station:2006"
2) "911.9752"
four) 1) "NYC:station:3136"
2) "940.3399"
5) 1) "NYC:station:3457"
2) "671.4900"
While this instance targeted on utilizing Python and Redis to parse information and construct an index of bikesharing system areas, it could actually simply be generalized to find eating places, public transit, or every other sort of place builders need to assist customers discover.
This article is predicated on my presentation at Open Source 101 in Raleigh this 12 months.
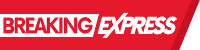