Go has popularized the mantra do not talk by sharing reminiscence; share reminiscence by speaking. The language does have the standard mutex (mutual exclusion assemble) to coordinate entry to shared reminiscence, nevertheless it favors using channels to share info amongst goroutines.
In this text, a quick have a look at goroutines, threads, and race circumstances units the scene for a have a look at two Go applications. In the primary program, goroutines talk by means of synchronized shared reminiscence, and the second makes use of channels for a similar goal. The code is accessible from my website in a .zip file with a README.
Threads and race circumstances
A thread is a sequence of executable directions, and threads inside the identical course of share an deal with house: Every thread in a multi-threaded course of has learn/write entry to the exact same reminiscence places. A memory-based race situation happens if two or extra threads (at the least one in every of which performs a write operation) have uncoordinated entry to the identical reminiscence location.
Consider this depiction of integer variable n
, whose worth is 777, and two threads attempting to change its contents:
n = n + 10 +-----+ n = n - 10
Thread1------------>| 777 |<------------Thread2
+-----+
n
On a multiprocessor machine, the 2 threads might execute actually on the identical time. The influence on variable n
is then indeterminate. It’s essential to notice that every tried replace consists of two machine-level operations: an arithmetic operation on n
‘s present worth (both including or subtracting 10), and a subsequent project operation that units n
to a brand new worth (both 787 or 767).
The paired operations executed within the two threads might interleave in varied inappropriate methods. Consider the next state of affairs, with every numbered merchandise as a single operation on the machine stage. For simplicity, assume that every operation takes one tick of the system clock:
- Thread1 does the addition to compute 787, which is saved in a short lived location (on the stack or in a CPU register).
- Thread2 does the subtraction to compute 767, additionally saved in a short lived location.
- Thread2 performs the project; the worth of
n
is now 767. - Thread1 performs the project; the worth of
n
is now 787.
By coming in final, Thread1 has gained the race towards Thread2. It’s clear that improper interleaving has occurred. Thread1 performs an addition operation, is delayed for 2 ticks, after which performs the project. By distinction, Thread2 performs the subtraction and subsequent project operations with out interruption. The repair is obvious: The arithmetic and project operations ought to happen as in the event that they had been a single, atomic operation. A assemble equivalent to a mutex supplies the required repair, and Go has the mutex.
Go applications are usually multi-threaded, though the threading happens beneath the floor. On the floor are goroutines. A goroutine is a inexperienced thread—a thread beneath the Go runtime management. By distinction, a native thread is instantly beneath OS management. But goroutines multiplex onto native threads that the OS schedules, which implies that memory-based race circumstances are attainable in Go. The first of two pattern applications illustrates this.
MiserSpendthrift1
The MiserSpendthrift1 program simulates shared entry to a checking account. In addition to fundamental
, there are two different goroutines:
- The miser goroutine repeatedly provides to the steadiness, one forex unit at a time.
- The spendthrift goroutine repeatedly subtracts from the steadiness, additionally one forex unit at a time.
The variety of occasions every goroutine performs its operation will depend on a command-line argument, which ought to be giant sufficient to be attention-grabbing (e.g., 100,000 to some million). The account steadiness is initialized to zero and will wind up as zero as a result of the deposits and withdrawals are for a similar quantity and are the identical in quantity.
Example 1. Using a mutex to coordinate entry to shared reminiscence
bundle fundamentalimport (
"os"
"fmt"
"runtime"
"strconv"
"sync"
)var accountBalance = zero // steadiness for shared checking account
var mutex = &sync.Mutex // mutual-exclusion lock// critical-section code with express locking/unlocking
func updateBalance(amt int)func reportAndExit(msg string)
func fundamental()
Flow-of-control within the MiserSpendthrift1 program (see above) may be described as follows:
The MiserSpendthrift1 program declares two international variables, one an integer variable to symbolize the shared checking account and the opposite a mutex to make sure coordinated goroutine entry to the account:
var accountBalance = zero // steadiness for shared checking account
var mutex = &sync.Mutex // mutual-exclusion lock
The mutex code happens within the updateBalance
operate to safeguard a essential part, which is a code phase that should be executed in single-threaded trend for this system to behave accurately:
func updateBalance(amt int)
The essential part is the assertion between the Lock()
and Unlock()
calls. Although a single line in Go supply code, this assertion entails two distinct operations: an arithmetic operation adopted by an project. These two operations should be executed collectively, one thread at a time, which the mutex code ensures. With the locking code in place, the accountBalance
is zero on the finish as a result of the variety of additions by 1 and subtractions by 1 is identical.
If the mutex code is eliminated, then the ultimate worth of the accountBalance
is unpredictable. On two pattern runs with the lock code eliminated, the ultimate steadiness was 249 on the primary run and -87 on the second, thereby confirming memory-based race situation occurred.
The mutex code’s habits deserves a more in-depth look:
- To execute the essential part code, a goroutine should first seize the lock by executing the
mutex.Lock()
name. If the lock is held already, then the goroutine blocks till the lock turns into accessible; in any other case, the goroutine executes the mutex-protected essential part. - The mutex ensures mutual exclusion in that just one goroutine at a time can execute the locked code phase. The mutex ensures single-threaded execution of the essential part: the arithmetic operation adopted by the project operation.
- The name to
Unlock()
releases a held lock in order that some goroutine (maybe the one which simply launched the lock) can seize the lock anew.
In the MiserSpendthrift1 program, three goroutines (the miser, the spendthrift, and fundamental
) talk by means of the shared reminiscence location named accountBalance
. A mutex coordinates entry to this variable by the miser and the spendthrift, and fundamental
tries to entry the variable solely after each the miser and the spendthrift have terminated. Even with a comparatively giant command-line argument (e.g., 5 to 10 million), this system runs comparatively quick and yields the anticipated remaining worth of zero for the accountBalance
.
The bundle sync/atomic
has capabilities equivalent to AddInt32
with synchronization baked in. For instance, if the accountBalance
kind had been modified from int
to int32
, then the updateBalance
operate may very well be simplified as follows:
func updateBalance(amt int32) // argument should be int32 as nicely
atomic.AddInt32(&accountBalance, amt) // no express locking required
The MiserSpendthrift1 program makes use of express locking to spotlight the critical-section code and to underscore the necessity for thread synchronization to forestall a race situation. In a production-grade instance, a essential part may comprise a number of traces of supply code. In any case, a essential part ought to be as quick as attainable to maintain this system as concurrent as attainable.
MiserSpendthrift2
The MiserSpendthrift2 program once more has a world variable accountBalance
initialized to zero, and once more there are miser and spendthrift goroutines contending to replace the steadiness. However, this program doesn’t use a mutex to forestall a race situation. Instead, there may be now a banker goroutine that accesses the accountBalance
in response to requests from the miser and the spendthrift. These two goroutines not replace the accountBalance
instantly. Here is a sketch of the structure:
requests updates
miser/spendthrift---------->banker--------->steadiness
This structure, with assist from a thread-safe Go channel to serialize requests from the miser and the spendthrift, prevents a race situation on the accountBalance
.
Example 2. Using a thread-safe channel to coordinate entry to shared reminiscence
bundle fundamentalimport (
"os"
"fmt"
"runtime"
"strconv"
"sync"
)kind bankOp struct
var accountBalance = zero // shared account
var bankRequests chan *bankOp // channel to bankerfunc updateBalance(amt int) int
replace := &bankOp
bankRequests <- replace
newBalance := <-replace.affirm
return newBalance// For now a no-op, however might save steadiness to a file with a timestamp.
func logBalance(present int)func reportAndExit(msg string)
func fundamental() {
if len(os.Args) < 2
reportAndExit("nUsage: go ms1.go <variety of updates per thread>")
iterations, err := strconv.Atoi(os.Args[1])
if err != nil
reportAndExit("Bad command-line argument: " + os.Args[1]);
bankRequests = make(chan *bankOp, eight) // eight is channel buffer measurement
var wg sync.WaitGroup
// The banker: handles all requests for deposits and withdrawals by means of a channel.
go func() ()// miser increments the steadiness
wg.Add(1) // increment WaitGroup counter
go func() ()// spendthrift decrements the steadiness
wg.Add(1) // increment WaitGroup counter
go func() ()wg.Wait() // await completion of miser and spendthrift
fmt.Println("Final balance: ", accountBalance) // affirm the steadiness is zero
}
The adjustments within the MiserSpendthrift2 program may be summarized as follows. There is a BankOp
construction:
kind bankOp struct
that the miser and the spendthrift goroutines use to make replace requests. The howMuch
area is the replace quantity, both 1 (miser) or -1 (spendthrift). The affirm
area is a channel that the banker goroutine makes use of in responding to a miser or a spendthrift request; this channel carries the brand new steadiness again to the requester as affirmation. For effectivity, the deal with of a bankOp
construction, moderately than a replica of it, is distributed over the bankRequests
channel, which is asserted as follows:
var bankRequests chan *bankOp // channel of tips to a bankOp
Channels are synchronized—that’s, thread-safe—by default.
The miser and the spendthrift once more name the updateBalance
operate to be able to change the account steadiness. This operate not has any express thread synchronization:
func updateBalance(amt int) int
The bankRequests
channel has a buffer measurement of eight to reduce blocking. The channel can maintain as much as eight unread requests earlier than additional makes an attempt so as to add one other bankOp
pointer are blocked. In the meantime, the banker goroutine ought to be processing the requests as they arrive; a request is eliminated mechanically from the channel when the banker reads it. The affirm
channel isn’t buffered, nevertheless. The requester blocks till the affirmation message—the up to date steadiness saved domestically within the newBalanace
variable—arrives from the banker.
Local variables and parameters within the updateBalance
operate (replace
, newBalance
, and amt
) are thereby thread-safe as a result of each goroutine will get its personal copies of them. The channels, too, are thread-safe in order that the physique of the updateBalance
operate not requires express locking. What a aid for the programmer!
The banker goroutine loops indefinitely, awaiting requests from the miser and spendthrift goroutines:
for
choose
// different circumstances may very well be added (e.g., golf outings)
While the miser and spendthrift goroutines are nonetheless energetic, solely the banker goroutine has entry to the accountBalance
, which implies that a race situation on this reminiscence location can’t come up. Only after the miser and spendthrift end their work and terminate does the fundamental
goroutine print the ultimate worth of the accountBalance
and exit. When fundamental
terminates, so does the banker goroutine.
Locks or channels?
The MiserSpendthrift2 program adheres to the Go mantra by favoring channels over synchronized shared reminiscence. To make certain, locked reminiscence may be tough. The mutex API is low-level and thus vulnerable to errors equivalent to locking however forgetting to unlock—with impasse as a attainable consequence. More delicate errors embrace locking solely a part of a essential part (underlocking) and locking code that doesn’t belong to a essential part (overlocking). Thread-safe capabilities equivalent to atomic.AddInt32
scale back these dangers as a result of the locking and unlocking happen mechanically. Yet the problem stays of how one can motive about low-level reminiscence locking in difficult applications.
The Go mantra brings challenges of its personal. If the 2 miser/spendthrift applications are run with a sufficiently giant command-line argument, the distinction in efficiency is noteworthy. The mutex could also be low-level, nevertheless it performs nicely. Go channels are interesting as a result of they supply built-in thread security and encourage single-threaded entry to shared essential sources such because the accountBalance
within the two pattern applications. Channels, nevertheless, incur a efficiency penalty in comparison with mutexes.
It’s uncommon in programming that one device suits all duties. Go accordingly comes with choices for thread security, starting from low-level locking by means of high-level channels.
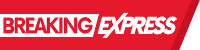