Node.js could be very helpful in terms of constructing command-line interfaces (CLIs). In this put up, I am going to train you the best way to use Node.js to construct a CLI that asks some questions and creates a file primarily based on the solutions.
Get began
Let’s begin by making a model new npm bundle. (Npm is the JavaScript bundle supervisor.)
mkdir my-script
cd my-script
npm init
Npm will ask some questions. After that, we have to set up some packages.
npm set up --save chalk figlet inquirer shelljs
Here’s what these packages do:
- Chalk: Terminal string styling achieved proper
- Figlet: A program for making giant letters out of strange textual content
- Inquirer: A group of frequent interactive command-line person interfaces
- ShellJS: Portable Unix shell instructions for Node.js
Make an index.js file
Now we’ll create an index.js
file with the next content material:
#!/usr/bin/env nodeconst inquirer = require("inquirer");
const chalk = require("chalk");
const figlet = require("figlet");
const shell = require("shelljs");
Plan the CLI
It’s all the time good to plan what a CLI must do earlier than writing any code. This CLI will do only one factor: create a file.
The CLI will ask two questions—what’s the filename and what’s the extension?—then create the file, and present successful message with the created file path.
// index.jsconst run = async () => ;
run();
The first operate is the script introduction. Let’s use chalk
and figlet
to get the job achieved.
const init = () =>
console.log(
chalk.inexperienced(
figlet.textSync("Node JS CLI", )
)
);const run = async () => ;
run();
Second, we’ll write a operate that asks the questions.
const askQuestions = () => ;// ...
const run = async () => ;
Notice the constants FILENAME and EXTENSIONS that got here from inquirer
.
The subsequent step will create the file.
const createFile = (filename, extension) => ;// ...
const run = async () => ;
And final however not least, we’ll present the success message together with the file path.
const success = (filepath) =>
console.log(
chalk.white.bgGreen.daring(`Done! File created at $`)
);
;// ...
const run = async () => ;
Let’s check the script by operating node index.js
. Here’s what we get:
The full code
Here is the ultimate code:
#!/usr/bin/env nodeconst inquirer = require("inquirer");
const chalk = require("chalk");
const figlet = require("figlet");
const shell = require("shelljs");const init = () => ;
const askQuestions = () =>
const questions = [
title: "FILENAME",
kind: "input",
message: "What is the name of the file without extension?"
,
];
return inquirer.immediate(questions);
;const createFile = (filename, extension) =>
const filePath = `$/$filename.$extension`
shell.contact(filePath);
return filePath;
;const success = filepath => ;
const run = async () => ;
run();
Use the script anyplace
To execute this script anyplace, add a bin
part in your bundle.json
file and run npm hyperlink
.
Running npm hyperlink
makes this script out there anyplace.
That’s what occurs whenever you run this command:
/usr/bin/creator -> /usr/lib/node_modules/creator/index.js
/usr/lib/node_modules/creator -> /residence/hugo/code/creator
It hyperlinks the index.js
file as an executable. This is barely potential due to the primary line of the CLI script: #!/usr/bin/env node
.
Now we are able to run this script by calling:
$ creator
Wrapping up
As you may see, Node.js makes it very simple to construct good command-line instruments! If you wish to go even additional, test this different packages:
- meow – a easy command-line helper
- yargs – a command-line opt-string parser
- pkg – bundle your Node.js venture into an executable
Tell us about your expertise constructing a CLI within the feedback.
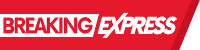