If you’ve ever puzzled concerning the efficiency of your Raspberry Pi, then you definitely may want a dashboard on your Pi. In this text, I reveal how one can shortly constructing an on-demand monitoring dashboard on your Raspberry Pi so you may see your CPU efficiency, reminiscence and disk utilization in actual time, and add extra views and actions later as you want them.
If you’re already utilizing Appsmith, you too can import the sample app instantly and get began.
Appsmith
Appsmith is an open supply, low-code app builder that helps builders construct inside apps like dashboards and admin panels simply and shortly. It’s an ideal selection on your dashboard, and reduces the time and complexity of conventional coding approaches.
For the dashboard on this instance, I show utilization stats for:
- CPU
- Percentage utilization
- Frequency or clock pace
- Count
- Temperature
- Memory
- Percentage utilization
- Percentage out there reminiscence
- Total reminiscence
- Free reminiscence
- Disk
- Percentage disk utilization
- Absolute disk area used
- Available disk area
- Total disk area
Creating an endpoint
You want a approach to get this knowledge out of your Raspberry Pi (RPi) and into Appsmith. The psutils Python library is helpful for monitoring and profiling, and the Flask-RESTful Flask extension creates a REST API.
Appsmith calls the REST API each few seconds to refresh knowledge routinely, and will get a JSON object in response with all desired stats as proven:
{ "cpu_count": 4,
"cpu_freq": [
600.0,
600.0,
1200.0 ],
"cpu_mem_avail": 463953920,
"cpu_mem_free": 115789824,
"cpu_mem_total": 971063296,
"cpu_mem_used": 436252672,
"cpu_percent": 1.8,
"disk_usage_free": 24678121472,
"disk_usage_percent": 17.7,
"disk_usage_total": 31307206656,
"disk_usage_used": 5292728320,
"sensor_temperatures": 52.616 }
1. Set up the REST API
If your Raspberry Pi doesn’t have Python on it but, open a terminal in your Pi and run this set up command:
$ sudo apt set up python3
Now arrange a Python virtual environment on your improvement:
$ python -m venv PiData
Next, activate the setting. You should do that after rebooting your Pi.
$ supply PiData/bin/activate
$ cd PiData
To set up Flask and Flask-RESTful and dependencies you’ll want later, create a file in your Python digital setting known as necessities.txt
and add these traces to it:
flask
flask-restful
gunicorn
Save the file, after which use pip
to put in them . You should do that after rebooting your Pi.
(PyData)$ python -m pip set up -r necessities.txt
Next, create a file named pi_stats.py
to accommodate the logic for retrieving the RPi’s system stats with psutils
. Paste this code into your pi_stat.py
file:
from flask import Flask
from flask_restful import Resource, Api
import psutil
app = Flask(__name__)
api = Api(app)
class PiData(Resource):
def get(self):
return "RPI Stat dashboard"
api.add_resource(PiData, '/get-stats')
if __name__ == '__main__':
app.run(debug=True)
Here’s what the code is doing:
- Use app = Flask(title) to outline the app that nests the API object.
- Use Flask-RESTful’s API method to outline the API object.
- Define PiData as a concrete Resource class in Flask-RESTful to show strategies for every supported HTTP technique.
- Attach the useful resource,
PiData
, to the API object,api
, withapi.add_resource(PiData, '/get-stats')
. - Whenever you hit the URL
/get-stats
,PiData
is returned because the response.
2. Read stats with psutils
To get the stats out of your Pi, you should utilize these built-in capabilities from psutils
:
cpu_percentage
,cpu_count
,cpu_freq
, andsensors_temperatures
capabilities for the share utilization, rely, clock pace, and temperature respectively, of the CPUsensors_temperatures
studies the temperature of all of the units related to the RPi. To get simply the CPU’s temperature, use the important thingcpu-thermal
.virtual_memory
for complete, out there, used, and free reminiscence stats in bytes.disk_usage
to return the whole, used, and free stats in bytes.
Combining all the capabilities in a Python dictionary appears like this:
system_info_data = {
'cpu_percent': psutil.cpu_percent(1),
'cpu_count': psutil.cpu_count(),
'cpu_freq': psutil.cpu_freq(),
'cpu_mem_total': reminiscence.complete,
'cpu_mem_avail': reminiscence.out there,
'cpu_mem_used': reminiscence.used,
'cpu_mem_free': reminiscence.free,
'disk_usage_total': disk.complete,
'disk_usage_used': disk.used,
'disk_usage_free': disk.free,
'disk_usage_percent': disk.p.c,
'sensor_temperatures': psutil.sensors_temperatures()['cpu-thermal'
][0].present, }
The subsequent part makes use of this dictionary.
3. Fetch knowledge from the Flask-RESTful API
To see knowledge out of your Pi within the API response, replace pi_stats.py
to incorporate the dictionary system_info_data
within the class PiData
:
from flask import Flask
from flask_restful import Resource, Api
import psutil
app = Flask(__name__)
api = Api(app)
class PiData(Resource):
def get(self):
reminiscence = psutil.virtual_memory()
disk = psutil.disk_usage("https://opensource.com/")
system_info_data = {
'cpu_percent': psutil.cpu_percent(1),
'cpu_count': psutil.cpu_count(),
'cpu_freq': psutil.cpu_freq(),
'cpu_mem_total': reminiscence.complete,
'cpu_mem_avail': reminiscence.out there,
'cpu_mem_used': reminiscence.used,
'cpu_mem_free': reminiscence.free,
'disk_usage_total': disk.complete,
'disk_usage_used': disk.used,
'disk_usage_free': disk.free,
'disk_usage_percent': disk.p.c,
'sensor_temperatures': psutil.sensors_temperatures()['cpu-thermal'][0].present, }
return system_info_data
api.add_resource(PiData, '/get-stats')
if __name__ == '__main__':
app.run(debug=True)
Your script’s prepared. Run the PiData.py
script:
$ python PyData.py
* Serving Flask app "PiData" (lazy loading)
* Environment: manufacturing
WARNING: This is a improvement server. Do not run this in a manufacturing setting.
* Debug mode: on
* Running on http://127.0.0.1:5000 (Press CTRL+C to give up)
* Restarting with stat
* Debugger is lively!
You have a working API!
4. Make the API out there to the web
You can work together together with your API in your native community. To attain it over the web, nonetheless, you should open a port in your firewall and ahead incoming visitors to the port made out there by Flask. However, because the output of your take a look at suggested, working a Flask app from Flask is supposed for improvement, not for manufacturing. To make your API out there to the web safely, you should utilize the gunicorn
manufacturing server, which you put in throughout the venture setup stage.
Now you can begin your Flask API. You should do that any time you’ve rebooted your Pi.
$ gunicorn -w 4 'PyData:app'
Serving on http://0.0.0.0:8000
To attain your Pi from the surface world, open a port in your community firewall and direct incoming visitors to the IP deal with of your PI, at port 8000.
First, get the interior IP deal with of your Pi:
$ ip addr present | grep inet
Internal IP addresses begin with 10 or 192 or 172.
Next, you should configure your firewall. There’s often a firewall embedded within the router you get out of your web service supplier (ISP). Generally, you entry your property router by means of an internet browser. Your router’s deal with is usually printed on the underside of the router, and it begins with both 192.168 or 10. Every machine is totally different, although, so there’s no approach for me to let you know precisely what it’s essential click on on to regulate your settings. For a full description of how one can configure your firewall, learn Seth Kenlon’s article Open ports and route traffic through your firewall.
Alternately, you should utilize localtunnel to make use of a dynamic port-forwarding service.
Once you’ve acquired visitors going to your Pi, you may question your API:
$ curl https://example.com/get-stats
{
"cpu_count": 4,
"cpu_freq": [
600.0,
600.0,
1200.0 ],
"cpu_mem_avail": 386273280,
...
If you have got gotten this far, the hardest half is over.
5. Repetition
If you reboot your Pi, you should observe these steps:
- Reactivate your Python setting with
supply
- Refresh the appliance dependencies with
pip
- Start the Flask software with
gunicorn
Your firewall settings are persistent, however in case you’re utilizing localtunnel, then you should additionally begin a brand new tunnel after a reboot.
You can automate these duties in case you like, however that’s a complete different tutorial. The closing part of this tutorial is to construct a UI on Appsmith utilizing the drag-and-drop widgets, and a little bit of Javascript, to bind your RPi knowledge to the UI. Believe me, it’s straightforward going from right here on out!
Build the dashboard on Appsmith.
(Keyur Paralkar, CC BY-SA 4.0)
To get to a dashboard like this, it’s essential join the uncovered API endpoint to Appsmith, construct the UI utilizing Appsmith’s widgets library, and bind the API’s response to your widgets. If you’re already utilizing Appsmith, you may simply import the sample app instantly and get began.
If you haven’t accomplished so already, sign up for a free Appsmith account. Alternately, you may self-host Appsmith.
Connect the API as an Appsmith datasource
Sign in to your Appsmith account.
- Find and click on the + button subsequent to QUERIES/JS within the left nav.
- Click Create a clean API.
- At the highest of the web page, title your venture PiData.
- Get your API’s URL. If you’re utilizing localtunnel, then that’s a
localtunnel.me
deal with, and as at all times append/get-stats
to the tip for the stat knowledge. Paste it into the primary clean discipline on the web page, and click on the RUN button.
Confirm that you simply see a profitable response within the Response pane.
(Keyur Paralkar, CC BY-SA 4.0)
Build the UI
The interface for AppSmith is fairly intuitive, however I like to recommend going by means of building your first application on Appsmith tutorial in case you really feel misplaced.
For the title, drag and drop a Text, Image, and Divider widget onto the canvas. Arrange them like this:
(Keyur Paralkar, CC BY-SA 4.0)
The Text widget comprises the precise title of your web page. Type in one thing cooler than “Raspberry Pi Stats”.
The Image widget homes a definite brand for the dashboard. You can use no matter you need.
Use a Switch widget for a toggled dwell knowledge mode. Configure it within the Property pane to get knowledge from the API you’ve constructed.
For the physique, create a spot for CPU Stats with a Container widget utilizing the next widgets from the Widgets library on the left facet:
- Progress Bar
- Stat Box
- Chart
Do the identical for the Memory and Disk stats sections. You don’t want a Chart for disk stats, however don’t let that cease you from utilizing one if yow will discover makes use of for it.
Your closing association of widgets ought to look one thing like this:
(Keyur Paralkar, CC BY-SA 4.0)
The closing step is to bind the info from the API to the UI widgets you have got.
Bind knowledge to the widgets
Head again to the canvas and discover your widgets in sections for the three classes. Set the CPU Stats first.
To bind knowledge to the Progress Bar widget:
- Click the Progress Bar widget to see the Property pane on the suitable.
- Look for the Progress property.
- Click the JS button to activate Javascript.
- Paste
{{PiData.knowledge.cpu_percent ?? 0}}
within the discipline for Progress. That code references the stream of knowledge from of your API namedPiData
. Appsmith caches the response knowledge inside the.knowledge
operator ofPiData
. The keycpu_percent
comprises the info Appsmith makes use of to show the share of, on this case, CPU utilization. - Add a Text widget beneath the Progress Bar widget as a label.
(Keyur Paralkar, CC BY-SA 4.0)
There are three Stat Box widgets within the CPU part. Binding knowledge to every one is the very same as for the Progress Bar widget, besides that you simply bind a distinct knowledge attribute from the .knowledge
operator. Follow the identical process, with these exceptions:
{{${PiData.knowledge.cpu_freq[0]} ?? 0 }}
to indicate clock pace.{{${PiData.knowledge.cpu_count} ?? 0 }}
for CPU rely.{{${(PiData.knowledge.sensor_temperatures).toPrecision(3)} ?? 0 }}
for CPU temperature knowledge.
Assuming all goes to plan, you find yourself with a fairly dashboard like this one:
(Keyur Paralkar, CC BY-SA 4.0)
CPU utilization pattern
You can use a Chart widget to show the CPU utilization as a pattern line, and have it routinely replace over time.
First, click on the widget, discover the Chart Type property on the suitable, and alter it to LINE CHART. To see a pattern line, retailer cpu_percent
in an array of knowledge factors. Your API at present returns this as a single knowledge time limit, so use Appsmith’s storeValue
operate (an Appsmith-native implementation of a browser’s setItem
technique) to get an array.
Click the + button subsequent to QUERIES/JS and title it utils.
Paste this Javascript code into the Code discipline:
export default {
getLiveData: () => {
//When change is on:
if (Switch1.isSwitchedOn) {
setInterval(() => {
let utilData = appsmith.retailer.cpu_util_data;
PiData.run()
storeValue("cpu_util_data", [...utilData, {
x: PiData.data.cpu_percent,
y: PiData.data.cpu_percent
}]);
}, 1500, 'timerId')
} else {
clearInterval('timerId');
}
},
initialOnPageLoad: () => {
storeValue("cpu_util_data", []);
}
}
To initialize the Store
, you’ve created a JavaScript operate within the object known as initialOnPageLoad
, and also you’ve housed the storeValue
operate in it.
You retailer the values from cpu_util_data
into the storeValue
operate utilizing storeValue("cpu_util_data", []);
. This operate runs on web page load.
So far, the code shops one knowledge level from cpu_util_data
within the Store
every time the web page is refreshed. To retailer an array, you utilize the x
and y
subscripted variables, each storing values from the cpu_percent
knowledge attribute.
You additionally need this knowledge saved routinely by a set interval between saved values. When the operate setInterval is executed:
- The worth saved in
cpu_util_data
is fetched. - The API
PiData
is named. cpu_util_data
is up to date asx
andy
variables with the most recentcpu_percent
knowledge returned.- The worth of
cpu_util_data
is saved in the important thingutilData
. - Steps 1 by means of 4 are repeated if and provided that the operate is about to auto-execute. You set it to auto-execute with the Switch widget, which explains why there’s a
getLiveData
dad or mum operate.
Navigate to the Settings tab to seek out all of the dad or mum capabilities within the object and set initialOnPageLoad
to Yes within the RUN ON PAGE LOAD choice.
(Keyur Paralkar, CC BY-SA 4.0)
Now refresh the web page for affirmation
Return to the canvas. Click the Chart widget and find the Chart Data property. Paste the binding {{ appsmith.retailer.disk_util_data }}
into it. This will get your chart in case you run the article utils
your self a couple of occasions. To run this routinely:
- Find and click on the Live Data Switch widget in your dashboard’s title.
- Look for the
onChange
occasion. - Bind it to
{{ utils.getLiveData() }}
. The Javascript object isutils
, andgetLiveData
is the operate that prompts whenever you toggle the Switch on, which fetches dwell knowledge out of your Raspberry Pi. But there’s different dwell knowledge, too, so the identical change works for them. Read on to see how.
Bind all the info
Binding knowledge to the widgets within the Memory and Disk sections is just like how you probably did it for the CPU Stats part.
For Memory, bindings change to:
{{( PiData.knowledge.cpu_mem_avail/1000000000).toPrecision(2) * 100 ?? 0 }}
for the Progress Bar.{{ ${(PiData.knowledge.cpu_mem_used/1000000000).toPrecision(2)} ?? 0 }} GB
,{{ ${(PiData.knowledge.cpu_mem_free/1000000000).toPrecision(2)} ?? 0}} GB
, and{{ ${(PiData.knowledge.cpu_mem_total/1000000000).toPrecision(2)} ?? 0 }} GB
for the three Stat Box widgets.
For Disk, bindings on the Progress Bar, and Stat Box widgets change respectively to:
{{ PiData.knowledge.disk_usage_percent ?? 0 }}
{{ ${(PiData.knowledge.disk_usage_used/1000000000).toPrecision(2)} ?? 0 }} GB
{{ ${(PiData.knowledge.disk_usage_free/1000000000).toPrecision(2)} ?? 0 }} GB
and{{ ${(PiData.knowledge.disk_usage_total/1000000000).toPrecision(2)} ?? 0 }} GB
for the three Stat Box widgets.
The Chart right here wants updating the utils
object you created for CPU Stats with a storeValue
key known as disk_util_data
nested below getLiveData
that follows the identical logic as cpu_util_data
. For the disk utilization chart, we retailer disk_util_data that follows the identical logic as that of the CPU utilization pattern chart.
export default {
getLiveData: () => {
//When change is on:
if (Switch1.isSwitchedOn) {
setInterval(() => {
const cpuUtilData = appsmith.retailer.cpu_util_data;
const diskUtilData = appsmith.retailer.disk_util_data;
PiData.run();
storeValue("cpu_util_data", [...cpuUtilData, { x: PiData.data.cpu_percent,y: PiData.data.cpu_percent }]);
storeValue("disk_util_data", [...diskUtilData, { x: PiData.data.disk_usage_percent,y: PiData.data.disk_usage_percent }]);
}, 1500, 'timerId')
} else {
clearInterval('timerId');
}
},
initialOnPageLoad: () => {
storeValue("cpu_util_data", []);
storeValue("disk_util_data", []);
}
}
Visualizing the stream of knowledge triggered by the Switch toggling dwell knowledge on and off with the utils
Javascript object appears like this:
(Keyur Paralkar, CC BY-SA 4.0)
Toggled on, the charts change like this:
(Keyur Paralkar, CC BY-SA 4.0)
Pretty, minimalistic, and completely helpful.
Enjoy
As you get extra snug with psutils
, Javascript, and Appsmith, I believe you’ll discover you may tweak your dashboard simply and endlessly to do actually cool issues like:
- See developments from the earlier week, month, quarter, 12 months, or any customized vary that your RPi knowledge permits
- Build an alert bot for threshold breaches on any stat
- Monitor different units related to your Raspberry Pi
- Extend
psutils
to a different pc with Python put in - Monitor your property or workplace community utilizing one other library
- Monitor your backyard
- Track your personal life habits
Until the subsequent superior construct, joyful hacking!
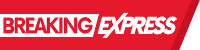