At the bottom of the testing pyramid are unit checks. Unit checks take a look at one unit of code at a time—normally one perform or technique.
Often, a single unit take a look at is designed to check one explicit circulation by a perform, or a particular department alternative. This allows simple mapping of a unit take a look at that fails and the bug that made it fail.
Ideally, unit checks use few or no exterior assets, isolating them and making them sooner.
Unit take a look at suites assist keep high-quality merchandise by signaling issues early within the growth course of. An efficient unit take a look at catches bugs earlier than the code has left the developer machine, or no less than in a steady integration atmosphere on a devoted department. This marks the distinction between good and dangerous unit checks: Good checks improve developer productiveness by catching bugs early and making testing sooner. Bad checks lower developer productiveness.
Productivity normally decreases when testing incidental options. The take a look at fails when the code modifications, even whether it is nonetheless right. This occurs as a result of the output is totally different, however in a manner that isn’t a part of the perform’s contract.
A great unit take a look at, subsequently, is one which helps implement the contract to which the perform is dedicated.
If a unit take a look at breaks, the contract is violated and ought to be both explicitly amended (by altering the documentation and checks), or fastened (by fixing the code and leaving the checks as is).
While limiting checks to implement solely the general public contract is an advanced ability to study, there are instruments that may assist.
One of those instruments is Hamcrest, a framework for writing assertions. Originally invented for Java-based unit checks, as we speak the Hamcrest framework helps a number of languages, together with Python.
Hamcrest is designed to make take a look at assertions simpler to write down and extra exact.
def add(a, b):
return a + bfrom hamcrest import assert_that, equal_to
def test_add():
assert_that(add(2, 2), equal_to(four))
This is a straightforward assertion, for easy performance. What if we wished to say one thing extra sophisticated?
def test_set_removal():
my_set =
my_set.take away(three)
assert_that(my_set, contains_inanyorder([1, 2, four]))
assert_that(my_set, is_not(has_item(three)))
Note that we are able to succinctly assert that the outcome has 1
, 2
, and four
in any order since units don’t assure order.
We additionally simply negate assertions with is_not
. This helps us write exact assertions, which permit us to restrict ourselves to implementing public contracts of capabilities.
Sometimes, nevertheless, not one of the built-in performance is exactly what we’d like. In these circumstances, Hamcrest permits us to write down our personal matchers.
Imagine the next perform:
def scale_one(a, b):
scale = random.randint(zero, 5)
choose = random.alternative([a,b])
return scale * choose
We can confidently assert that the outcome divides into no less than one of many inputs evenly.
A matcher inherits from hamcrest.core.base_matcher.BaseMatcher
, and overrides two strategies:
class DivisibleBy(hamcrest.core.base_matcher.BaseMatcher):def __init__(self, issue):
self.issue = issuedef _matches(self, merchandise):
return (merchandise % self.issue) == zerodef describe_to(self, description):
description.append_text('quantity divisible by')
description.append_text(repr(self.issue))
Writing high-quality describe_to
strategies is vital, since that is a part of the message that can present up if the take a look at fails.
def divisible_by(num):
return DivisibleBy(num)
By conference, we wrap matchers in a perform. Sometimes this provides us an opportunity to additional course of the inputs, however on this case, no additional processing is required.
def test_scale():
outcome = scale_one(three, 7)
assert_that(outcome,
any_of(divisible_by(three),
divisible_by(7)))
Note that we mixed our divisible_by
matcher with the built-in any_of
matcher to make sure that we take a look at solely what the contract commits to.
While modifying this text, I heard a rumor that the title “Hamcrest” was chosen as an anagram for “matches”. Hrm…
>>> assert_that("matches", contains_inanyorder(*"hamcrest")
Traceback (most up-to-date name final):
File "<stdin>", line 1, in <module>
File "/home/moshez/src/devops-python/build/devops/lib/python3.6/site-packages/hamcrest/core/assert_that.py", line 43, in assert_that
_assert_match(precise=arg1, matcher=arg2, purpose=arg3)
File "/home/moshez/src/devops-python/build/devops/lib/python3.6/site-packages/hamcrest/core/assert_that.py", line 57, in _assert_match
increase AssertionError(description)
AssertionError:
Expected: a sequence over ['h', 'a', 'm', 'c', 'r', 'e', 's', 't'] in any order
however: no merchandise matches: 'r' in ['m', 'a', 't', 'c', 'h', 'e', 's']
Researching extra, I discovered the supply of the rumor: It is an anagram for “matchers”.
>>> assert_that("matchers", contains_inanyorder(*"hamcrest"))
>>>
If you aren’t but writing unit checks on your Python code, now is an effective time to start out. If you’re writing unit checks on your Python code, utilizing Hamcrest will assist you to make your assertion exact—neither extra nor lower than what you plan to check. This will result in fewer false positives when modifying code and fewer time spent modifying checks for working code.
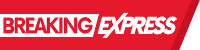