I just lately started driving a automobile that had historically used premium fuel (93 octane). According to the maker, although, it requires solely 91 octane. The factor is, within the US, you should buy solely 87, 89, or 93 octane. Where I stay, fuel costs soar 30 cents per gallon soar from one grade to the subsequent, so premium prices 60 cents greater than common. So why not attempt to avoid wasting cash?
It’s simple sufficient to attend till the fuel gauge exhibits that the tank is half full after which fill it with 89 octane, and there you could have 91 octane. But it will get difficult to know what to do subsequent—half a tank of 91 octane plus half a tank of 93 finally ends up being 92, and the place do you go from there? You could make persevering with calculations, however they get more and more messy. This is the place Python got here into the image.
I wished to provide you with a easy scheme by which I may fill the tank at some stage with 93 octane, then on the similar or another stage with 89 octane, with the first objective to by no means get beneath 91 octane with the ultimate combination. What I wanted to do was create some recurring calculation that makes use of the earlier octane worth for the previous fill-up. I suppose there can be some polynomial equation that may clear up this, however in Python, this feels like a loop.
#!/usr/bin/env python
# octane.pyo = 93.zero
newgas = 93.zero # this represents the octane of the final fillup
i = 1
whereas i < 21: # 20 iterations (journeys to the pump)
if newgas == 89.zero: # if the final fillup was with 89 octane
# change to 93
newgas = 93.zero
o = newgas/2 + o/2 # fill when gauge is half of full
else: # if it wasn't 89 octane, change to that
newgas = 89.zero
o = newgas/2 + o/2 # fill when gauge says half of full
print str(i) + ': '+ str(o)
i += 1
As you possibly can see, I’m initializing the variable o (the present octane combination within the tank) and the variable newgas (what I final stuffed the tank with) on the similar worth of 93. The loop then will repeat 20 occasions, for 20 fill-ups, switching from 89 octane and 93 octane for each different journey to the station.
1: 91.zero
2: 92.zero
three: 90.5
four: 91.75
5: 90.375
6: 91.6875
7: 90.34375
eight: 91.671875
9: 90.3359375
10: 91.66796875
11: 90.333984375
12: 91.6669921875
13: 90.3334960938
14: 91.6667480469
15: 90.3333740234
16: 91.6666870117
17: 90.3333435059
18: 91.6666717529
19: 90.3333358765
20: 91.6666679382
This exhibits is that I most likely want solely 10 or 15 loops to see stabilization. It additionally exhibits that quickly sufficient, I undershoot my 91 octane goal. It’s additionally fascinating to see this stabilization of the alternating combination values, and it seems this occurs with any scheme the place you select the identical quantities every time. In reality, it’s true even when the quantity of the fill-up is totally different for 89 and 93 octane.
So at this level, I started enjoying with fractions, reasoning that I’d most likely want a much bigger 93 octane fill-up than the 89 fill-up. I additionally didn’t need to make frequent journeys to the fuel station. What I ended up with (which appeared fairly good to me) was to attend till the tank was about 7⁄12 full and fill it with 89 octane, then wait till it was ¼ full and fill it with 93 octane.
Here is what the modifications within the loop appear to be:
if newgas == 89.zero:
newgas = 93.zero
o = three*newgas/four + o/four
else:
newgas = 89.zero
o = 5*newgas/12 + 7*o/12
Here are the numbers, beginning with the tenth fill-up:
10: 92.5122272978
11: 91.0487992571
12: 92.5121998143
13: 91.048783225
14: 92.5121958062
15: 91.048780887
As you possibly can see, this retains the ultimate octane very barely above 91 on a regular basis. Of course, my fuel gauge isn’t marked in twelfths, however 7⁄12 is barely lower than 5⁄eight, and I can deal with that.
An various easy answer might need been run the tank to empty and fill with 93 octane, then subsequent time solely half-fill it for 89—and maybe this will likely be my default plan. Personally, I’m not a fan of working the tank all the best way down since this isn’t all the time handy. On the opposite hand, it may simply work on a protracted journey. And generally I purchase fuel due to a sudden drop in costs. So ultimately, this scheme is certainly one of a sequence of choices that I can contemplate.
The most essential factor for Python customers: Don’t code whereas driving!
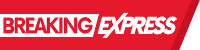