One of the nice trendy battles of knowledge science and machine studying is “Python vs. R.” There is little question that each have gained monumental floor lately to turn out to be prime programming languages for information science, predictive analytics, and machine studying. In truth, in line with a latest IEEE article, Python overtook C++ because the top programming language and R firmly secured its spot within the prime 10.
However, there are some basic variations between these two. R was developed primarily as a instrument for statistical evaluation and fast prototyping of a knowledge evaluation drawback. Python, then again, was developed as a basic function, trendy object-oriented language in the identical vein as C++ or Java however with an easier studying curve and extra versatile demeanor. Consequently, R continues to be extraordinarily well-liked amongst statisticians, quantitative biologists, physicists, and economists, whereas Python has slowly emerged as the highest language for day-to-day scripting, automation, backend internet improvement, analytics, and basic machine studying frameworks and has an in depth help base and open supply improvement neighborhood work.
Mimicking purposeful programming in a Python atmosphere
R’s nature as a functional programming language offers customers with an very simple and compact interface for fast calculations of possibilities and important descriptive/inferential statistics for a knowledge evaluation drawback. For instance, would not it’s nice to have the ability to clear up the next issues with only a single, compact operate name?
- How to calculate the imply/median/mode of a knowledge vector.
- How to calculate the cumulative chance of some occasion following a standard distribution. What if the distribution is Poisson?
- How to calculate the inter-quartile vary of a collection of knowledge factors.
- How to generate just a few random numbers following a Student’s t-distribution.
The R programming atmosphere can do all of those.
On the opposite hand, Python’s scripting means permits analysts to make use of these statistics in all kinds of analytics pipelines with limitless sophistication and creativity.
To mix the benefits of each worlds, you simply want a easy Python-based wrapper library that incorporates probably the most generally used features pertaining to chance distributions and descriptive statistics outlined in R-style. This allows you to name these features actually quick with out having to go to the right Python statistical libraries and determine the entire checklist of strategies and arguments.
Python wrapper script for many handy R-functions
I wrote a Python script to outline probably the most handy and extensively used R-functions in easy, statistical evaluation—in Python. After importing this script, it is possible for you to to make use of these R-functions naturally, identical to in an R programming atmosphere.
The objective of this script is to present easy Python subroutines mimicking R-style statistical features for shortly calculating density/level estimates, cumulative distributions, and quantiles and producing random variates for essential chance distributions.
To keep the spirit of R styling, the script makes use of no class hierarchy and solely uncooked features are outlined within the file. Therefore, a consumer can import this one Python script and use all of the features every time they’re wanted with a single title name.
Note that I take advantage of the phrase mimic. Under no circumstance am I claiming to emulate R’s true purposeful programming paradigm, which consists of a deep environmental setup and complicated relationships between these environments and objects. This script permits me (and I hope numerous different Python customers) to shortly fireplace up a Python program or Jupyter pocket book, import the script, and begin doing easy descriptive statistics very quickly. That’s the objective, nothing extra, nothing much less.
If you have coded in R (possibly in grad college) and are simply beginning to be taught and use Python for information evaluation, you may be joyful to see and use a number of the similar well-known features in your Jupyter pocket book in a fashion comparable to how you employ them in your R atmosphere.
Whatever your cause, utilizing this script is enjoyable.
Simple examples
To begin, simply import the script and begin working with lists of numbers as in the event that they have been information vectors in R.
from R_functions import *
lst=[20,12,16,32,27,65,44,45,22,18]
<extra code, extra statistics...>
Say you wish to calculate the Tuckey five-number abstract from a vector of knowledge factors. You simply name one easy operate, fivenum, and go on the vector. It will return the five-number abstract in a NumPy array.
lst=[20,12,16,32,27,65,44,45,22,18]
fivenum(lst)
> array([12. , 18.5, 24.5, 41. , 65. ])
Maybe you wish to know the reply to the next query:
Suppose a machine outputs 10 completed items per hour on common with a normal deviation of two. The output sample follows a close to regular distribution. What is the chance that the machine will output not less than 7 however not more than 12 models within the subsequent hour?
The reply is basically this:
You can receive the reply with only one line of code utilizing pnorm:
pnorm(12,10,2)-pnorm(7,10,2)
> zero.7745375447996848
Or possibly you should reply the next:
Suppose you’ve got a loaded coin with the chance of turning heads up 60% each time you toss it. You are taking part in a sport of 10 tosses. How do you plot and map out the probabilities of all of the doable variety of wins (from zero to 10) with this coin?
You can receive a pleasant bar chart with just some strains of code utilizing only one operate, dbinom:
probs=[]
import matplotlib.pyplot as plt
for i in vary(11):
probs.append(dbinom(i,10,zero.6))
plt.bar(vary(11),top=probs)
plt.grid(True)
plt.present()
Simple interface for chance calculations
R provides an very simple and intuitive interface for fast calculations from important chance distributions. The interface goes like this:
- ddistribution provides the density operate worth at some extent x
- pdistribution provides the cumulative worth at some extent x
- qdistribution provides the quantile operate worth at a chance p
- rdistribution generates one or a number of random variates
In our implementation, we keep on with this interface and its related argument checklist so you may execute these features precisely such as you would in an R atmosphere.
Currently carried out features
The following R-style features are carried out within the script for quick calling.
- Mean, median, variance, normal deviation
- Tuckey five-number abstract, IQR
- Covariance of a matrix or between two vectors
- Density, cumulative chance, quantile operate, and random variate era for the next distributions: regular, uniform, binomial, Poisson, F, Student’s t, Chi-square, beta, and gamma.
Work in progress
Obviously, this can be a work in progress, and I plan so as to add another handy R-functions to this script. For instance, in R, a single line of command lm can get you an extraordinary least-square fitted mannequin to a numerical dataset with all the mandatory inferential statistics (P-values, normal error, and many others.). This is powerfully temporary and compact! On the opposite hand, normal linear regression issues in Python are sometimes tackled utilizing Scikit-learn, which wants a bit extra scripting for this use, so I plan to include this single operate linear mannequin becoming characteristic utilizing Python’s statsmodels backend.
If you want and use this script in your work, please assist others discover it by starring or forking its GitHub repository. Also, you may test my different GitHub repos for enjoyable code snippets in Python, R, or MATLAB and a few machine studying sources.
If you’ve got any questions or concepts to share, please contact me at tirthajyoti[AT]gmail.com. If you might be, like me, obsessed with machine studying and information science, please add me on LinkedIn or follow me on Twitter.
Originally revealed on Towards Data Science. Reposted below CC BY-SA 4.0.
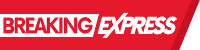