Simple compound instructions—reminiscent of stringing a number of instructions collectively in a sequence on the command line—are used usually. Such instructions are separated by semicolons, which outline the top of a command. To create a easy sequence of shell instructions on a single line, merely separate every command utilizing a semicolon, like this:
command1 ; command2 ; command3 ; command4 ;
You needn’t add a closing semicolon as a result of urgent the Enter key implies the top of the ultimate command, nevertheless it’s nice so as to add it for consistency.
All the instructions will run with no downside—so long as no error happens. But what occurs if an error occurs? We can anticipate and permit for errors utilizing the && and || management operators constructed into Bash. These two management operators present some stream management and allow us to change the code-execution sequence. The semicolon and the newline character are additionally thought of to be Bash management operators.
The && operator merely says “if command1 is successful, then run command2.” If command1 fails for any purpose, command2 will not run. That syntax appears like:
command1 && command2
This works as a result of each command returns a code to the shell that signifies whether or not it accomplished efficiently or failed throughout execution. By conference, a return code (RC) of zero (zero) signifies success and any constructive quantity signifies some kind of failure. Some sysadmin instruments simply return a 1 to point any failure, however many use different constructive numerical codes to point the kind of failure.
The Bash shell’s $? variable may be checked very simply by a script, by the following command in a listing of instructions, and even instantly by a sysadmin. Let’s have a look at RCs. We can run a easy command and instantly examine the RC, which is able to at all times pertain to the final command that ran.
[scholar@studentvm1 ~]$ ll ; echo "RC = $?"
complete 284
-rw-rw-r-- 1 scholar scholar 130 Sep 15 16:21 ascii-program.sh
drwxrwxr-x 2 scholar scholar 4096 Nov 10 11:09 bin
<snip>
drwxr-xr-x. 2 scholar scholar 4096 Aug 18 10:21 Videos
RC = zero
[scholar@studentvm1 ~]$
This RC is zero, which suggests the command accomplished efficiently. Now attempt the identical command on a listing the place we do not have permissions.
[scholar@studentvm1 ~]$ ll /root ; echo "RC = $?"
ls: can't open listing '/root': Permission denied
RC = 2
[scholar@studentvm1 ~]$
This RC’s which means may be discovered within the ls command’s man page.
Let’s attempt the && management operator because it may be utilized in a command-line program. We’ll begin with one thing easy: Create a brand new listing and, if that’s profitable, create a brand new file in it.
We want a listing the place we are able to create different directories. First, create a brief listing in your house listing the place you are able to do some testing.
[scholar@studentvm1 ~]$ cd ; mkdir testdir
Create a brand new listing in ~/testdir, which must be empty since you simply created it, after which create a brand new, empty file in that new listing. The following command will do these duties.
[scholar@studentvm1 ~]$ mkdir ~/testdir/testdir2 && contact ~/testdir/testdir2/testfile1
[scholar@studentvm1 ~]$ ll ~/testdir/testdir2/
complete zero
-rw-rw-r-- 1 scholar scholar zero Nov 12 14:13 testfile1
[scholar@studentvm1 ~]$
We know every thing labored because it ought to as a result of the testdir listing is accessible and writable. Change the permissions on testdir so it’s now not accessible to the consumer scholar as follows:
[scholar@studentvm1 ~]$ chmod 076 testdir ; ll | grep testdir
d---rwxrw-. three scholar scholar 4096 Nov 12 14:13 testdir
[scholar@studentvm1 ~]$
Using the grep command after the lengthy checklist (ll) reveals the itemizing for testdir. You can see that the consumer scholar now not has entry to the testdir listing. Now let’s run nearly the identical command as earlier than however change it to create a distinct listing title inside testdir.
[scholar@studentvm1 ~]$ mkdir ~/testdir/testdir3 && contact ~/testdir/testdir3/testfile1
mkdir: can't create listing ‘/house/scholar/testdir/testdir3’: Permission denied
[scholar@studentvm1 ~]$
Although we acquired an error message, utilizing the && management operator prevents the contact command from working as a result of there was an error in creating testdir3. This kind of command-line logical stream management can stop errors from compounding and making an actual mess of issues. But let’s make it a little bit extra sophisticated.
The || management operator permits us so as to add one other command that executes when the preliminary program assertion returns a code bigger than zero.
[scholar@studentvm1 ~]$ mkdir ~/testdir/testdir3 && contact ~/testdir/testdir3/testfile1 || echo "An error occurred while creating the directory."
mkdir: can't create listing ‘/house/scholar/testdir/testdir3’: Permission denied
An error occurred whereas creating the listing.
[scholar@studentvm1 ~]$
Our compound command syntax utilizing stream management takes this basic type after we use the && and || management operators:
previous instructions ; command1 && command2 || command3 ; following instructions
The compound command utilizing the management operators could also be preceded and adopted by different instructions that may be associated to those within the flow-control part however that are unaffected by the stream management. All of these instructions will execute with out regard to something that takes place contained in the flow-control compound command.
These flow-control operators could make working on the command line extra environment friendly by dealing with selections and letting us know when an issue has occurred. I take advantage of them instantly on the command line in addition to in scripts.
You can clear up as the foundation consumer to delete the listing and its contents.
[root@studentvm1 ~]# rm -rf /house/scholar/testdir
How do you utilize Bash management operators? Let us know within the remark part.
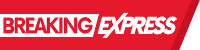