I do know, Python and JavaScript are what the youngsters are writing all their loopy “apps” with nowadays. But do not be so fast to dismiss C—it is a succesful and concise language that has rather a lot to supply. If you want pace, writing in C may very well be your reply. If you’re in search of job safety and the chance to learn to search out null pointer dereferences, C is also your reply! In this text, I am going to clarify tips on how to construction a C file and write a C fundamental perform that handles command line arguments like a champ.
Me: a crusty Unix system programmer.
You: somebody with an editor, a C compiler, and a while to kill.
Let’s do that.
A boring however right C program
A C program begins with a fundamental() perform, often saved in a file named fundamental.c.
/* fundamental.c */
int fundamental(int argc, char *argv[])
This program compiles however does not do something.
$ gcc fundamental.c
$ ./a.out -o foo -vv
$
Correct and boring.
Main features are distinctive
The fundamental() perform is the primary perform in your program that’s executed when it begins executing, but it surely’s not the primary perform executed. The first perform is _start(), which is usually offered by the C runtime library, linked in mechanically when your program is compiled. The particulars are extremely depending on the working system and compiler toolchain, so I’ll fake I did not point out it.
The fundamental() perform has two arguments that historically are known as argc and argv and return a signed integer. Most Unix environments count on applications to return zero (zero) on success and -1 (unfavourable one) on failure.
Argument | Name | Description |
---|---|---|
argc | Argument rely | Length of the argument vector |
argv | Argument vector | Array of character pointers |
The argument vector, argv, is a tokenized illustration of the command line that invoked your program. In the instance above, argv could be an inventory of the next strings:
argv = [ "/path/to/a.out", "-o", "foo", "-vv" ];
The argument vector is assured to at all times have at the very least one string within the first index, argv[0], which is the total path to this system executed.
Anatomy of a fundamental.c file
When I write a fundamental.c from scratch, it is often structured like this:
/* fundamental.c */
/* zero copyright/licensing */
/* 1 contains */
/* 2 defines */
/* three exterior declarations */
/* four typedefs */
/* 5 international variable declarations */
/* 6 perform prototypes */int fundamental(int argc, char *argv[])
/* Eight perform declarations */
I am going to speak about every of those numbered sections, apart from zero, beneath. If you must put copyright or licensing textual content in your supply, put it there.
Another factor I will not speak about including to your program is feedback.
"Comments lie."
- A cynical however sensible and good wanting programmer.
Instead of feedback, use significant perform and variable names.
Appealing to the inherent laziness of programmers, when you add feedback, you have doubled your upkeep load. If you alter or refactor the code, you have to replace or develop the feedback. Over time, the code mutates away from something resembling what the feedback describe.
If you must write feedback, don’t write about what the code is doing. Instead, write about why the code is doing what it is doing. Write feedback that you’d need to learn 5 years from now if you’ve forgotten all the things about this code. And the destiny of the world is relying on you. No stress.
1. Includes
The first issues I add to a fundamental.c file are contains to make a large number of normal C library features and variables obtainable to my program. The commonplace C library does numerous issues; discover header information in /usr/embody to search out out what it could possibly do for you.
The #embody string is a C preprocessor (cpp) directive that causes the inclusion of the referenced file, in its entirety, in the present file. Header information in C are often named with a .h extension and mustn’t include any executable code; solely macros, defines, typedefs, and exterior variable and performance prototypes. The string <header.h> tells cpp to search for a file known as header.h within the system-defined header path, often /usr/embody.
/* fundamental.c */
#embody <stdio.h>
#embody <stdlib.h>
#embody <unistd.h>
#embody <libgen.h>
#embody <errno.h>
#embody <string.h>
#embody <getopt.h>
#embody <sys/varieties.h>
This is the minimal set of world contains that I am going to embody by default for the next stuff:
#embody File | Stuff It Provides |
---|---|
stdio | Supplies FILE, stdin, stdout, stderr, and the fprint() household of features |
stdlib | Supplies malloc(), calloc(), and realloc() |
unistd | Supplies EXIT_FAILURE, EXIT_SUCCESS |
libgen | Supplies the basename() perform |
errno | Defines the exterior errno variable and all of the values it could possibly tackle |
string | Supplies memcpy(), memset(), and the strlen() household of features |
getopt | Supplies exterior optarg, opterr, optind, and getopt() perform |
sys/varieties | Typedef shortcuts like uint32_t and uint64_t |
2. Defines
/* fundamental.c */
<...>#outline OPTSTR "vi:o:f:h"
#outline USAGE_FMT "%s [-v] [-f hexflag] [-i inputfile] [-o outputfile] [-h]"
#outline ERR_FOPEN_INPUT "fopen(input, r)"
#outline ERR_FOPEN_OUTPUT "fopen(output, w)"
#outline ERR_DO_THE_NEEDFUL "do_the_needful blew up"
#outline DEFAULT_PROGNAME "george"
This does not make plenty of sense proper now, however the OPTSTR outline is the place I’ll state what command line switches this system will advocate. Consult the getopt(3) man web page to find out how OPTSTR will have an effect on getopt()‘s conduct.
The USAGE_FMT outline is a printf()-style format string that’s referenced within the utilization() perform.
I additionally like to assemble string constants as #defines on this a part of the file. Collecting them makes it simpler to repair spelling, reuse messages, and internationalize messages, if required.
Finally, use all capital letters when naming a #outline to differentiate it from variable and performance names. You can run the phrases collectively if you would like or separate phrases with an underscore; simply be certain they’re all higher case.
three. External declarations
/* fundamental.c */
<...>extern int errno;
extern char *optarg;
extern int opterr, optind;
An extern declaration brings that title into the namespace of the present compilation unit (aka “file”) and permits this system to entry that variable. Here we have introduced within the definitions for 3 integer variables and a personality pointer. The choose prefaced variables are utilized by the getopt() perform, and errno is used as an out-of-band communication channel by the usual C library to speak why a perform may need failed.
four. Typedefs
/* fundamental.c */
<...>typedef struct options_t;
After exterior declarations, I wish to declare typedefs for buildings, unions, and enumerations. Naming a typedef is a faith all to itself; I strongly favor a _t suffix to point that the title is a sort. In this instance, I’ve declared options_t as a struct with 4 members. C is a whitespace-neutral programming language, so I exploit whitespace to line up subject names in the identical column. I similar to the way in which it seems to be. For the pointer declarations, I prepend the asterisk to the title to make it clear that it is a pointer.
5. Global variable declarations
/* fundamental.c */
<...>int dumb_global_variable = -11;
Global variables are a nasty thought and you must by no means use them. But if you must use a worldwide variable, declare them right here and you’ll want to give them a default worth. Seriously, do not use international variables.
6. Function prototypes
/* fundamental.c */
<...>void utilization(char *progname, int choose);
int do_the_needful(options_t *choices);
As you write features, including them after the fundamental() perform and never earlier than, embody the perform prototypes right here. Early C compilers used a single-pass technique, which meant that each image (variable or perform title) you utilized in your program needed to be declared earlier than you used it. Modern compilers are practically all multi-pass compilers that construct a whole image desk earlier than producing code, so utilizing perform prototypes isn’t strictly required. However, you generally do not get to decide on what compiler is used in your code, so write the perform prototypes and drive on.
As a matter in fact, I at all times embody a utilization() perform that fundamental() calls when it does not perceive one thing you handed in from the command line.
7. Command line parsing
/* fundamental.c */
<...>int fundamental(int argc, char *argv[])
OK, that is rather a lot. The goal of the fundamental() perform is to gather the arguments that the person offers, carry out minimal enter validation, after which cross the collected arguments to features that can use them. This instance declares an choices variable initialized with default values and parse the command line, updating choices as crucial.
The guts of this fundamental() perform is a whereas loop that makes use of getopt() to step via argv in search of command line choices and their arguments (if any). The OPTSTR #outline earlier within the file is the template that drives getopt()‘s conduct. The choose variable takes on the character worth of any command line choices discovered by getopt(), and this system’s response to the detection of the command line possibility occurs within the swap assertion.
Those of you paying consideration will now be questioning why choose is asserted as a 32-bit int however is anticipated to tackle an Eight-bit char? It seems that getopt() returns an int that takes on a unfavourable worth when it will get to the top of argv, which I verify towards EOF (the End of File marker). A char is a signed amount, however I like matching variables to their perform return values.
When a recognized command line possibility is detected, option-specific conduct occurs. Some choices have an argument, laid out in OPTSTR with a trailing colon. When an possibility has an argument, the subsequent string in argv is offered to this system through the externally outlined variable optarg. I exploit optarg to open information for studying and writing or changing a command line argument from a string to an integer worth.
There are a few factors for fashion right here:
- Initialize opterr to zero, which disables getopt from emiting a ?.
- Use exit(EXIT_FAILURE); or exit(EXIT_SUCCESS); in the course of fundamental().
- /* NOTREACHED */ is a lint directive that I like.
- Use return EXIT_SUCCESS; on the finish of features that return int.
- Explicitly forged implicit kind conversions.
The command line signature for this program, if it had been compiled, would look one thing like this:
$ ./a.out -h
a.out [-v] [-f hexflag] [-i inputfile] [-o outputfile] [-h]
In truth, that is what utilization() will emit to stderr as soon as compiled.
Eight. Function declarations
/* fundamental.c */
<...>void utilization(char *progname, int choose)
int do_the_needful(options_t *choices)
Finally, I write features that are not boilerplate. In this instance, perform do_the_needful() accepts a pointer to an options_t construction. I validate that the choices pointer isn’t NULL after which go on to validate the enter and output construction members. EXIT_FAILURE returns if both take a look at fails and, by setting the exterior international variable errno to a traditional error code, I sign to the caller a normal purpose. The comfort perform perror() can be utilized by the caller to emit human-readable-ish error messages based mostly on the worth of errno.
Functions ought to nearly at all times validate their enter not directly. If full validation is pricey, attempt to do it as soon as and deal with the validated information as immutable. The utilization() perform validates the progname argument utilizing a conditional project within the fprintf() name. The utilization() perform goes to exit anyway, so I do not trouble setting errno or making an enormous stink about utilizing an accurate program title.
The large class of errors I am making an attempt to keep away from right here is de-referencing a NULL pointer. This will trigger the working system to ship a particular sign to my course of known as SYSSEGV, which ends up in unavoidable demise. The final thing customers need to see is a crash resulting from SYSSEGV. It’s a lot better to catch a NULL pointer as a way to emit higher error messages and shut down this system gracefully.
Some individuals complain about having a number of return statements in a perform physique. They make arguments about “continuity of control flow” and different stuff. Honestly, if one thing goes mistaken in the course of a perform, it is a good time to return an error situation. Writing a ton of nested if statements to only have one return isn’t a “good idea.”™
Finally, should you write a perform that takes 4 or extra arguments, think about bundling them in a construction and passing a pointer to the construction. This makes the perform signatures easier, making them simpler to recollect and never screw up once they’re known as later. It additionally makes calling the perform barely quicker, since fewer issues have to be copied into the perform’s stack body. In observe, it will solely grow to be a consideration if the perform known as tens of millions or billions of instances. Don’t fear about it if that does not make sense.
In the do_the_needful() perform, I wrote a particular kind of remark that’s designed to be a placeholder reasonably than documenting the code:
/* XXX do needful stuff */
When you’re within the zone, generally you do not need to cease and write some significantly gnarly little bit of code. You’ll come again and do it later, simply not now. That’s the place I am going to go away myself somewhat breadcrumb. I insert a remark with a XXX prefix and a brief comment describing what must be achieved. Later on, when I’ve extra time, I am going to grep via supply in search of XXX. It does not matter what you employ, simply be certain it is not more likely to present up in your codebase in one other context, as a perform title or variable, as an example.
Putting all of it collectively
OK, this program nonetheless does nearly nothing if you compile and run it. But now you have got a stable skeleton to construct your personal command line parsing C applications.
/* fundamental.c - the entire itemizing */#embody <stdio.h>
#embody <stdlib.h>
#embody <unistd.h>
#embody <libgen.h>
#embody <errno.h>
#embody <string.h>
#embody <getopt.h>#outline OPTSTR "vi:o:f:h"
#outline USAGE_FMT "%s [-v] [-f hexflag] [-i inputfile] [-o outputfile] [-h]"
#outline ERR_FOPEN_INPUT "fopen(input, r)"
#outline ERR_FOPEN_OUTPUT "fopen(output, w)"
#outline ERR_DO_THE_NEEDFUL "do_the_needful blew up"
#outline DEFAULT_PROGNAME "george"extern int errno;
extern char *optarg;
extern int opterr, optind;typedef struct options_t;
int dumb_global_variable = -11;
void utilization(char *progname, int choose);
int do_the_needful(options_t *choices);int fundamental(int argc, char *argv[])
void utilization(char *progname, int choose)
int do_the_needful(options_t *choices)
Now you are prepared to put in writing C that will probably be simpler to keep up. If you have got any questions or suggestions, please share them within the feedback.
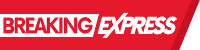