Are you confused by fancy programming phrases like features, courses, strategies, libraries, and modules? Do you battle with the scope of variables? Whether you are a self-taught programmer or a formally educated code monkey, the modularity of code will be complicated. But courses and libraries encourage modular code, and modular code can imply increase a group of multipurpose code blocks that you should utilize throughout many tasks to cut back your coding workload. In different phrases, if you happen to comply with together with this text’s research of Python features, you will discover methods to work smarter, and dealing smarter means working much less.
This article assumes sufficient Python familiarity to write down and run a easy script. If you have not used Python, learn my intro to Python article first.
Functions
Functions are an vital step towards modularity as a result of they’re formalized strategies of repetition. If there’s a job that must be achieved many times in your program, you may group the code right into a operate and name the operate as typically as you want it. This method, you solely have to write down the code as soon as, however you should utilize it as typically as you want.
Here is an instance of a easy operate:
#!/usr/bin/env python3import time
def Timer():
print("Time is " + str(time.time() ) )
Create a folder known as mymodularity and save the operate code as timestamp.py.
In addition to this operate, create a file known as __init__.py within the mymodularity listing. You can do that in a file supervisor or a Bash shell:
$ contact mymodularity/__init__.py
You have now created your individual Python library (a “module,” in Python lingo) in your Python package deal known as mymodularity. It’s not a really helpful module, as a result of all it does is import the time module and print a timestamp, nevertheless it’s a begin.
To use your operate, deal with it identical to another Python module. Here’s a small utility that assessments the accuracy of Python’s sleep() operate, utilizing your mymodularity package deal for assist. Save this file as sleeptest.py exterior the mymodularity listing (if you happen to put this into mymodularity, then it turns into a module in your package deal, and you do not need that).
#!/usr/bin/env python3import time
from mymodularity import timestampprint("Testing Python sleep()...")
# modularity
timestamp.Timer()
time.sleep(three)
timestamp.Timer()
In this easy script, you might be calling your timestamp module out of your mymodularity package deal (twice). When you import a module from a package deal, the standard syntax is to import the module you need from the package deal after which use the module title + a dot + the title of the operate you wish to name (e.g., timestamp.Timer()).
You’re calling your Timer() operate twice, so in case your timestamp module had been extra difficult than this easy instance, you would be saving your self various repeated code.
Save the file and run it:
$ python3 ./sleeptest.py
Testing Python sleep()...
Time is 1560711266.1526039
Time is 1560711269.1557732
According to your take a look at, the sleep operate in Python is fairly correct: after three seconds of sleep, the timestamp was efficiently and accurately incremented by three, with a little bit variance in microseconds.
The construction of a Python library might sound complicated, nevertheless it’s not magic. Python is programmed to deal with a folder filled with Python code accompanied by an __init__.py file as a package deal, and it is programmed to search for accessible modules in its present listing first. This is why the assertion from mymodularity import timestamp works: Python seems within the present listing for a folder known as mymodularity, then seems for a timestamp file ending in .py.
What you could have achieved on this instance is functionally the identical as this much less modular model:
#!/usr/bin/env python3import time
from mymodularity import timestampprint("Testing Python sleep()...")
# no modularity
print("Time is " + str(time.time() ) )
time.sleep(three)
print("Time is " + str(time.time() ) )
For a easy instance like this, there’s probably not a cause you would not write your sleep take a look at that method, however the perfect half about writing your individual module is that your code is generic so you may reuse it for different tasks.
You could make the code extra generic by passing data into the operate once you name it. For occasion, suppose you wish to use your module to check not the pc’s sleep operate, however a person’s sleep operate. Change your timestamp code so it accepts an incoming variable known as msg, which might be a string of textual content controlling how the timestamp is introduced every time it’s known as:
#!/usr/bin/env python3import time
# up to date code
def Timer(msg):
print(str(msg) + str(time.time() ) )
Now your operate is extra summary than earlier than. It nonetheless prints a timestamp, however what it prints for the person is undefined. That means it’s essential to outline it when calling the operate.
The msg parameter your Timer operate accepts is arbitrarily named. You may name the parameter m or message or textual content or something that is smart to you. The vital factor is that when the timestamp.Timer operate is named, it accepts some textual content as its enter, locations no matter it receives right into a variable, and makes use of the variable to perform its job.
Here’s a brand new utility to check the person’s capacity to sense the passage of time accurately:
#!/usr/bin/env python3from mymodularity import timestamp
print("Press the RETURN key. Count to 3, and press RETURN again.")
enter()
timestamp.Timer("Started timer at ")print("Count to 3...")
enter()
timestamp.Timer("You slept until ")
Save your new utility as response.py and run it:
$ python3 ./response.py
Press the RETURN key. Count to three, and press RETURN once more.Started timer at 1560714482.3772075
Count to three...You slept till 1560714484.1628013
Functions and required parameters
The new model of your timestamp module now requires a msg parameter. That’s vital as a result of your first utility is damaged as a result of it would not go a string to the timestamp.Timer operate:
$ python3 ./sleeptest.py
Testing Python sleep()...
Traceback (most up-to-date name final):
File "./sleeptest.py", line eight, in <module>
timestamp.Timer()
TypeError: Timer() lacking 1 required positional argument: 'msg'
Can you repair your sleeptest.py utility so it runs accurately with the up to date model of your module?
Variables and features
By design, features restrict the scope of variables. In different phrases, if a variable is created inside a operate, that variable is on the market to solely that operate. If you attempt to use a variable that seems in a operate exterior the operate, an error happens.
Here’s a modification of the response.py utility, with an try to print the msg variable from the timestamp.Timer() operate:
#!/usr/bin/env python3from mymodularity import timestamp
print("Press the RETURN key. Count to 3, and press RETURN again.")
enter()
timestamp.Timer("Started timer at ")print("Count to 3...")
enter()
timestamp.Timer("You slept for ")print(msg)
Try working it to see the error:
$ python3 ./response.py
Press the RETURN key. Count to three, and press RETURN once more.Started timer at 1560719527.7862902
Count to three...You slept for 1560719528.135406
Traceback (most up-to-date name final):
File "./response.py", line 15, in <module>
print(msg)
NameError: title 'msg' is not outlined
The utility returns a NameError message as a result of msg will not be outlined. This might sound complicated since you wrote code that outlined msg, however you could have better perception into your code than Python does. Code that calls a operate, whether or not the operate seems inside the similar file or if it is packaged up as a module, would not know what occurs contained in the operate. A operate independently performs its calculations and returns what it has been programmed to return. Any variables concerned are native solely: they exist solely inside the operate and solely so long as it takes the operate to perform its goal.
Return statements
If your utility wants data contained solely in a operate, use a return assertion to have the operate present significant knowledge after it runs.
They say time is cash, so modify your timestamp operate to permit for an imaginary charging system:
#!/usr/bin/env python3import time
def Timer(msg):
print(str(msg) + str(time.time() ) )
cost = .02
return cost
The timestamp module now expenses two cents for every name, however most significantly, it returns the quantity charged every time it’s known as.
Here’s an indication of how a return assertion can be utilized:
#!/usr/bin/env python3from mymodularity import timestamp
print("Press RETURN for the time (costs 2 cents).")
print("Press Q RETURN to quit.")whole = zero
whereas True:
kbd = enter()
if kbd.decrease() == "q":
print("You owe $" + str(whole) )
exit()
else:
cost = timestamp.Timer("Time is ")
whole = whole+cost
In this pattern code, the variable cost is assigned because the endpoint for the timestamp.Timer() operate, so it receives regardless of the operate returns. In this case, the operate returns a quantity, so a brand new variable known as whole is used to maintain monitor of what number of adjustments have been made. When the applying receives the sign to give up, it prints the full expenses:
$ python3 ./cost.py
Press RETURN for the time (prices 2 cents).
Press Q RETURN to give up.Time is 1560722430.345412
Time is 1560722430.933996
Time is 1560722434.6027434
Time is 1560722438.612629
Time is 1560722439.3649364
q
You owe $zero.1
Inline features
Functions do not need to be created in separate information. If you are simply writing a brief script particular to at least one job, it could make extra sense to simply write your features in the identical file. The solely distinction is that you do not have to import your individual module, however in any other case the operate works the identical method. Here’s the newest iteration of the time take a look at utility as one file:
#!/usr/bin/env python3import time
whole = zero
def Timer(msg):
print(str(msg) + str(time.time() ) )
cost = .02
return costprint("Press RETURN for the time (costs 2 cents).")
print("Press Q RETURN to quit.")whereas True:
kbd = enter()
if kbd.decrease() == "q":
print("You owe $" + str(whole) )
exit()
else:
cost = Timer("Time is ")
whole = whole+cost
It has no exterior dependencies (the time module is included within the Python distribution), and produces the identical outcomes because the modular model. The benefit is that all the pieces is situated in a single file, and the drawback is that you just can’t use the Timer() operate in another script you might be writing until you copy and paste it manually.
Global variables
A variable created exterior a operate has nothing limiting its scope, so it’s thought-about a world variable.
An instance of a worldwide variable is the whole variable within the cost.py instance used to trace present expenses. The working whole is created exterior any operate, so it’s sure to the applying moderately than to a selected operate.
A operate inside the utility has entry to your world variable, however to get the variable into your imported module, you have to ship it there the identical method you ship your msg variable.
Global variables are handy as a result of they appear to be accessible at any time when and wherever you want them, however it may be tough to maintain monitor of their scope and to know which of them are nonetheless hanging round in system reminiscence lengthy after they’re now not wanted (though Python usually has superb rubbish assortment).
Global variables are vital, although, as a result of not all variables will be native to a operate or class. That’s straightforward now that you know the way to ship variables to features and get values again.
Wrapping up features
You’ve discovered quite a bit about features, so begin placing them into your scripts—if not as separate modules, then as blocks of code you do not have to write down a number of instances inside one script. In the subsequent article on this collection, I am going to get into Python courses.
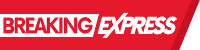