If you are utilizing Python for any quantity of growth, you might have in all probability issued a command in a terminal, even when solely to launch a Python script or set up a Python module with pip. Commands could also be easy and singular:
$ ls
Commands additionally would possibly take an argument:
$ ls instance
Commands may have choices or flags:
$ ls --color instance
Sometimes choices even have arguments:
$ sudo firewall-cmd --list-all --zone house
Arguments
The POSIX shell routinely splits no matter you kind as a command into an array. For occasion, right here is a straightforward command:
$ ls instance
The command ls is place $zero, and the argument instance is in place $1.
You might write a loop to iterate over every merchandise; decide whether or not it’s the command, an choice, or an argument; and take motion accordingly. Luckily, a module referred to as argparse already exists for that.
Argparse
The argparse module is straightforward to combine into your Python packages and has a number of comfort options. For occasion, in case your person modifications the order of choices or makes use of one choice that takes no arguments (referred to as a Boolean, which means the choice toggles a setting on or off) after which one other that requires an argument (corresponding to –color crimson, for instance), argparse can deal with the range. If your person forgets an choice that is required, the argparse module can present a pleasant error message.
Using argparse in your software begins with defining what choices you wish to present your person. There are a number of completely different sorts of arguments you may settle for, however the syntax is constant and easy.
Here’s a easy instance:
#!/usr/bin/env python
import argparse
import sysdef getOptions(args=sys.argv[1:]):
parser = argparse.ArgumentParser(description="Parses command.")
parser.add_argument("-i", "--input", assist="Your input file.")
parser.add_argument("-o", "--output", assist="Your destination output file.")
parser.add_argument("-n", "--number", kind=int, assist="A number.")
parser.add_argument("-v", "--verbose",dest='verbose',motion='store_true', assist="Verbose mode.")
choices = parser.parse_args(args)
return choices
This code pattern creates a operate referred to as getOptions and tells Python to have a look at every potential argument preceded by some recognizable string (corresponding to –input or -i). Any choice that Python finds is returned out of the operate as an choices object (choices is an arbitrary title and has no particular which means; it is only a information object containing a abstract of all of the arguments that the operate has parsed).
By default, any argument given by the person is seen by Python as a string. If it’s good to ingest an integer (a quantity), you will need to specify that an choice expects kind=int, as within the –number choice within the pattern code.
If you might have an argument that simply turns a function on or off, then you will need to use the boolean kind, as with the –verbose flag within the pattern code. This type of choice merely shops True or False, specifying whether or not or not the person used the flag. If the choice is used, then stored_true is activated.
Once the getOptions operate runs, you need to use the contents of the choices object and have your program make choices based mostly on how the person invoked the command. You can see the contents of choices with a check print assertion. Add this to the underside of your instance file:
print(getOptions())
Then run the code with some arguments:
$ python3 ./instance.py -i foo -n four
Namespace(enter='foo', quantity=four, output=None, verbose=False)
Retrieving values
The choices object within the pattern code incorporates any worth supplied by the person (or a derived Boolean worth) in keys named after the lengthy choice. In the pattern code, as an illustration, the –number choice might be retrieved by taking a look at choices.quantity.
choices = getOptions(sys.argv[1:])if choices.verbose:
print("Verbose mode on")
else:
print("Verbose mode off")print(choices.enter)
print(choices.output)
print(choices.quantity)# Insert Useful Python Code Here...
The Boolean choice, –verbose within the instance, is set by testing whether or not choices.verbose is True (which means the person did use the –verbose flag) or False (the person didn’t use the –verbose flag), and taking some motion accordingly.
Help and suggestions
Argparse additionally features a built-in –help (-h for brief) choice that gives a useful tip on how the command is used. This is derived out of your code, so it takes no additional work to generate this assist system:
$ ./instance.py --assist
utilization: instance.py [-h] [-i INPUT] [-o OUTPUT] [-n NUMBER] [-v]Parses command.
elective arguments:
-h, --assist present this assist message and exit
-i INPUT, --enter INPUT
Your enter file.
-o OUTPUT, --output OUTPUT
Your vacation spot output file.
-n NUMBER, --number NUMBER
A quantity.
-v, --verbose Verbose mode.
Python parsing like a professional
This a easy instance that demonstrates the best way to cope with parsing arguments in a Python software and the best way to shortly and effectively doc their syntax. The subsequent time you write a fast Python script, give it some choices with argparse. You’ll thank your self later, and your command will really feel much less like a fast hack and extra like a “real” Unix command!
Here’s the pattern code, which you need to use for testing:
#!/usr/bin/env python3
# GNU All-Permissive License
# Copying and distribution of this file, with or with out modification,
# are permitted in any medium with out royalty supplied the copyright
# discover and this discover are preserved. This file is obtainable as-is,
# with none guarantee.import argparse
import sysdef getOptions(args=sys.argv[1:]):
parser = argparse.ArgumentParser(description="Parses command.")
parser.add_argument("-i", "--input", assist="Your input file.")
parser.add_argument("-o", "--output", assist="Your destination output file.")
parser.add_argument("-n", "--number", kind=int, assist="A number.")
parser.add_argument("-v", "--verbose",dest='verbose',motion='store_true', assist="Verbose mode.")
choices = parser.parse_args(args)
return choiceschoices = getOptions(sys.argv[1:])
if choices.verbose:
print("Verbose mode on")
else:
print("Verbose mode off")print(choices.enter)
print(choices.output)
print(choices.quantity)
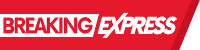