When I made a decision I needed to play with shade this summer season, I thought of the truth that colours are often depicted on a shade wheel. This is often with pigment colours moderately than mild, and also you lose any sense of the variation in shade brightness or luminosity.
As an alternative choice to the colour wheel, I got here up with the concept of displaying the RGB spectrum on the surfaces of a dice utilizing a sequence of graphs. RGB values can be depicted on a three-dimensional graph with X-, Y-, and Z-axes. For instance, a floor would maintain B (or blue) at zero and the remaining axes would present what occurs as I plot values as colours for R (purple) and G (inexperienced) from zero to 255.
It seems this isn’t very tough to do utilizing Scribus and its Python Scripter functionality. I can create RGB colours, make rectangles displaying the colours, and prepare them in a 2D format. I made a decision to make worth jumps of 5 for the colours and make rectangles measuring 5 factors on a aspect. Thus, for every 2D graph, I’d make about 250 colours, and the dice would measure 250 factors to a aspect, or three.5 inches.
I used this little bit of Python code to perform that process for the Green–Red graph:
x = 300
y = 300
r = zero
g = zero
b = zeroif scribus.newDoc(scribus.PAPER_LETTER, (zero,zero,zero,zero),scribus.PORTRAIT, 1, scribus.UNIT_POINTS, scribus.NOFACINGPAGES, scribus.FIRSTPAGERIGHT):
whereas r < 256:
whereas g < 256:
newcolor = str(r) + '_' + str(g) + '_' + str(b)
if newcolor == '0_0_0':
newcolor = 'Black'
scribus.defineColorRGB(newcolor,r, g, b)
rect = scribus.createRect(x + g, y, 5, 5)
scribus.setFillColor(newcolor, rect)
scribus.setLineColor(newcolor, rect)
g = g + 5
g = zero
r = r + 5
y = y – 5
This script begins the graphical construction at 300, 300, which is in regards to the center of a US Letter-size web page horizontally and perhaps a 3rd of the way in which down from the highest; that is the origin of the graph. Then it builds the graph horizontally alongside the X-axis (the Green worth), then returns to the Y-axis, jumps up the web page 5 factors, and makes one other line of rectangles.
That appears to be like straightforward sufficient; I will simply fiddle with the numbers and make the opposite sides. But this is not only a matter of constructing two extra graphs, one with Blue–Green and one other with Red–Blue. I had in thoughts to create an unfolded dice so I might print it, minimize it, fold it, and create a 3D view of RGB. Therefore, the subsequent half (happening the web page) must have the origin (the Black nook) on the higher left, with Green horizontally and Blue vertically growing downward.
“Fiddling with the numbers” ended up being kind of trial and error to get what I needed. After creating the second graph, I wanted the third one, for Red–Blue, to have the origin within the higher left nook with Red growing to the left and Blue growing downward.
Here it’s:
Of course, that is simply the primary half of this dice. I wanted to make an identical form, besides that the origins must be White (moderately than Black) to signify the excessive values. It’s a kind of occasions once I want I had been smarter, since not solely did I must make an identical general form, it wanted to interface with the primary form in a mirror-image kind of approach (I believe). Sometimes trial and error is the one buddy you’ve got.
Here is how that got here out; I used a separate script since there wasn’t sufficient area on a US Letter-sized web page for each of them:
Now, it is off to the printer! This is the place you get a way of how effectively your shade printer does with RGB to CMYK transformation in addition to different points of printing color-dense areas.
Next, girls and boys, it is cut-and-paste time! I might use tape, however I did not wish to change the looks of the surfaces, so I left some tabs alongside the edges whereas chopping so I might glue them on the within. From expertise, I can say that printing on copy paper comes out with some undesirable wrinkles, so after my copy paper prototype, I printed the dice on heavier paper with a matte end.
Keep in thoughts that is only a view of the boundaries of the RGB area; to be extra correct, you would need to make a strong dice that you may slice within the center. For instance, this may be a slice by way of a strong RGB dice the place Blue = 120:
In the top, I had enjoyable doing this challenge. In case you wish to be part of the celebration, listed here are the 2 scripts.
Here’s the primary half:
#!/usr/bin/env python
# black2rgb.py
"""
Creates one-half of RGB dice with Black at origin
"""import scribus
x = 300
y = 300
r = zero
g = zero
b = zeroif scribus.newDoc(scribus.PAPER_LETTER, (zero,zero,zero,zero),scribus.PORTRAIT, 1, scribus.UNIT_POINTS, scribus.NOFACINGPAGES, scribus.FIRSTPAGERIGHT):
whereas r < 256:
whereas g < 256:
newcolor = str(r) + '_' + str(g) + '_' + str(b)
if newcolor == '0_0_0':
newcolor = 'Black'
scribus.defineColorRGB(newcolor,r, g, b)
rect = scribus.createRect(x + g, y, 5, 5)
scribus.setFillColor(newcolor, rect)
scribus.setLineColor(newcolor, rect)
g = g + 5
g = zero
r = r + 5
y = y - 5
r = zero
g = zero
y = 305whereas b < 256:
whereas g < 256:
newcolor = str(r) + '_' + str(g) + '_' + str(b)
if newcolor == '0_0_0':
newcolor = 'Black'
scribus.defineColorRGB(newcolor,r, g, b)
rect = scribus.createRect(x + g, y, 5, 5)
scribus.setFillColor(newcolor, rect)
scribus.setLineColor(newcolor, rect)
g = g + 5
g = zero
b = b + 5
y = y + 5
r = 255
g = zero
y = 305
x = 39
b = zerowhereas b < 256:
whereas r >= zero:
newcolor = str(r) + '_' + str(g) + '_' + str(b)
if newcolor == '0_0_0':
newcolor = 'Black'
scribus.defineColorRGB(newcolor,r, g, b)
rect = scribus.createRect(x, y, 5, 5)
scribus.setFillColor(newcolor, rect)
scribus.setLineColor(newcolor, rect)
r = r - 5
x = x+5
b = b + 5
x = 39.5
r = 255
y = y + 5
scribus.setReduncooked(True)
scribus.redrawAll()
Now the second half:
#!/usr/bin/env python
# white2rgb.py
"""
Creates one-half of RGB dice with White at origin
"""import scribus
x = 300
y = 300
r = 255
g = 255
b = 255if scribus.newDoc(scribus.PAPER_LETTER, (zero,zero,zero,zero),scribus.PORTRAIT, 1, scribus.UNIT_POINTS, scribus.NOFACINGPAGES, scribus.FIRSTPAGERIGHT):
whereas g >= zero:
whereas r >= zero:
newcolor = str(r) + '_' + str(g) + '_' + str(b)
if newcolor == '255_255_255':
newcolor = 'White'
scribus.defineColorRGB(newcolor,r, g, b)
rect = scribus.createRect(x + 255 - r, y, 5, 5)
scribus.setFillColor(newcolor, rect)
scribus.setLineColor(newcolor, rect)
r = r - 5
r = 255
g = g - 5
y = y - 5
r = 255
g = 255
y = 305whereas b >= zero:
whereas r >= zero:
newcolor = str(r) + '_' + str(g) + '_' + str(b)
if newcolor == '255_255_255':
newcolor = 'White'
scribus.defineColorRGB(newcolor,r, g, b)
rect = scribus.createRect(x + 255 - r, y, 5, 5)
scribus.setFillColor(newcolor, rect)
scribus.setLineColor(newcolor, rect)
r = r - 5
r = 255
b = b - 5
y = y + 5
r = 255
g = zero
y = 305
x = 39
b = 255whereas b >= zero:
whereas g < 256:
newcolor = str(r) + '_' + str(g) + '_' + str(b)
if newcolor == '255_255_255':
newcolor = 'White'
scribus.defineColorRGB(newcolor,r, g, b)
rect = scribus.createRect(x + g, y, 5, 5)
scribus.setFillColor(newcolor, rect)
scribus.setLineColor(newcolor, rect)
g = g + 5
g = zero
b = b - 5
y = y + 5
scribus.setReduncooked(True)
scribus.redrawAll()
Since I used to be creating numerous colours, I wasn’t shocked to see that the Scribus file is way bigger than the PDF I made out of it. For instance, my Scribus SLA file was three.0MB, whereas the PDF I generated from it was solely 70KB.
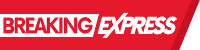