This is the story of how our group at Rookout constructed non-breaking breakpoints for Python and among the classes we realized alongside the best way. I will be presenting all concerning the nuts and bolts of debugging in Python at PyBay 2019 in San Francisco this month. Let’s dig in.
The coronary heart of Python debugging: sys.set_trace
There are many Python debuggers on the market. Some of the extra common embrace:
- pdb, a part of the Python customary library
- PyDev, the debugger behind the Eclipse and PyCharm IDEs
- ipdb, the IPython debugger
Despite the vary of decisions, virtually each Python debugger is predicated on only one operate: sys.set_trace. And let me inform you, sys.settrace may simply be essentially the most complicated operate within the Python customary library.
In less complicated phrases, settrace registers a hint operate for the interpreter, which can be referred to as in any of the next instances:
- Function name
- Line execution
- Function return
- Exception raised
A easy hint operate may appear to be this:
def simple_tracer(body, occasion, arg):
co = body.f_code
func_name = co.co_name
line_no = body.f_lineno
print(" f ".format(
e=occasion, f=func_name, l=line_no))
return simple_tracer
When taking a look at this operate, the primary issues that come to thoughts are its arguments and return values. The hint operate arguments are:
- body object, which is the complete state of the interpreter on the level of the operate’s execution
- occasion string, which will be name, line, return, or exception
- arg object, which is non-compulsory and will depend on the occasion kind
The hint operate returns itself as a result of the interpreter retains monitor of two sorts of hint capabilities:
- Global hint operate (per thread): This hint operate is ready for the present thread by sys.settrace and is invoked every time a brand new body is created by the interpreter (primarily on each operate name). While there is no documented strategy to set the hint operate for a special thread, you possibly can name threading.settrace to set the hint operate for all newly created threading module threads.
- Local hint operate (per body): This hint operate is ready by the interpreter to the worth returned by the worldwide hint operate upon body creation. There’s no documented strategy to set the native hint operate as soon as the body has been created.
This mechanism is designed to permit the debugger to have extra granular management over which frames are traced to scale back efficiency influence.
Building our debugger in three straightforward steps (or so we thought)
With all that background, writing your individual debugger utilizing a customized hint operate appears like a frightening activity. Luckily, pdb, the usual Python debugger, is constructed on prime of Bdb, a base class for constructing debuggers.
A naive breakpoints debugger primarily based on Bdb may appear to be this:
import bdb
import examineclass Debugger(bdb.Bdb):
def __init__(self):
Bdb.__init__(self)
self.breakpoints = dict()
self.set_trace()def set_breakpoint(self, filename, lineno, methodology):
self.set_break(filename, lineno)
attempt :
self.breakpoints[(filename, lineno)].add(methodology)
besides KeyError:
self.breakpoints[(filename, lineno)] = [methodology]def user_line(self, body):
if not self.break_here(body):
return# Get filename and lineno from body
(filename, lineno, _, _, _) = examine.getframeinfo(body)strategies = self.breakpoints[(filename, lineno)]
for methodology in strategies:
methodology(body)
All this does is:
- Inherits from Bdb and write a easy constructor initializing the bottom class and tracing.
- Adds a set_breakpoint methodology that makes use of Bdb to set the breakpoint and retains monitor of our breakpoints.
- Overrides the user_line methodology that is known as by Bdb on sure consumer traces. The operate makes positive it’s being referred to as for a breakpoint, will get the supply location, and invokes the registered breakpoints
How properly did the straightforward Bdb debugger work?
Rookout is about bringing a debugger-like consumer expertise to production-grade efficiency and use instances. So, how properly did our naive breakpoint debugger carry out?
To check it and measure the worldwide efficiency overhead, we wrote two easy check strategies and executed every of them 16 million instances beneath a number of situations. Keep in thoughts that no breakpoint was executed in any of the instances.
def empty_method():
godef simple_method():
a = 1
b = 2
c = three
d = four
e = 5
f = 6
g = 7
h = eight
i = 9
j = 10
Using the debugger takes a surprising period of time to finish. The dangerous outcomes make it clear that our naive Bdb debugger just isn’t but production-ready.
Optimizing the debugger
There are three essential methods to scale back debugger overhead:
- Limit native tracing as a lot as potential: Local tracing could be very pricey in comparison with international tracing because of the a lot bigger variety of occasions per line of code.
- Optimize “call” occasions and return management to the interpreter sooner: The essential work in name occasions is deciding whether or not or to not hint.
- Optimize “line” occasions and return management to the interpreter sooner: The essential work in line occasions is deciding whether or not or not we hit a breakpoint.
So we forked Bdb, lowered the characteristic set, simplified the code, optimized for decent code paths, and obtained spectacular outcomes. However, we had been nonetheless not glad. So, we took one other stab at it, migrated and optimized our code to .pyx, and compiled it utilizing Cython. The closing outcomes (as you possibly can see under) had been nonetheless not adequate. So, we ended up diving into CPython’s supply code and realizing we couldn’t make tracing quick sufficient for manufacturing use.
Rejecting Bdb in favor of bytecode manipulation
After our preliminary disappointment from the trial-and-error cycles of ordinary debugging strategies, we determined to look right into a much less apparent choice: bytecode manipulation.
The Python interpreter works in two essential phases:
- Compiling Python supply code into Python bytecode: This unreadable (for people) format is optimized for environment friendly execution and is commonly cached in these .pyc information now we have all come to like.
- Iterating via the bytecode within the interpreter loop: This executes one instruction at a time.
This is the sample we selected: use bytecode manipulation to set non-breaking breakpoints with no international overhead. This is finished by discovering the bytecode in reminiscence that represents the supply line we’re keen on and inserting a operate name simply earlier than the related instruction. This manner, the interpreter doesn’t should do any further work to assist our breakpoints.
This strategy just isn’t magic. Here’s a fast instance.
We begin with a quite simple operate:
def multiply(a, b):
end result = a * b
return end result
In documentation hidden within the inspect module (which has a number of helpful utilities), we study we will get the operate’s bytecode by accessing multiply.func_code.co_code:
'|x00x00|x01x00x14}x02x00|x02x00S'
This unreadable string will be improved utilizing the dis module within the Python customary library. By calling dis.dis(multiply.func_code.co_code), we get:
four zero LOAD_FAST zero (a)
three LOAD_FAST 1 (b)
6 BINARY_MULTIPLY
7 STORE_FAST 2 (end result)5 10 LOAD_FAST 2 (end result)
13 RETURN_VALUE
This will get us nearer to understanding what occurs behind the scenes of debugging however to not an easy answer. Unfortunately, Python doesn’t supply a way for altering a operate’s bytecode from throughout the interpreter. You can overwrite the operate object, however that is not adequate for almost all of real-world debugging situations. You should go about it in a roundabout manner utilizing a local extension.
Conclusion
When constructing a brand new instrument, you invariably find yourself studying rather a lot about how stuff works. It additionally makes you suppose out of the field and hold your thoughts open to sudden options.
Working on non-breaking breakpoints for Rookout has taught me rather a lot about compilers, debuggers, server frameworks, concurrency fashions, and far way more. If you have an interest in studying extra about bytecode manipulation, Google’s open supply cloud-debug-python has instruments for enhancing bytecode.
Liran Haimovitch will current “Understanding Python’s Debugging Internals” at PyBay, which can be held August 17-18 in San Francisco. Use code OpenSource35 for a reduction once you buy your ticket to allow them to know you came upon concerning the occasion from our neighborhood.
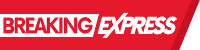