In my article A beginner’s guide to building DevOps pipelines with open source tools, I shared a narrative about constructing a DevOps pipeline from scratch. The core know-how driving that initiative was Jenkins, an open supply instrument to construct steady integration and steady supply (CI/CD) pipelines.
At Citi, there was a separate group that offered devoted Jenkins pipelines with a secure master-slave node setup, however the surroundings was solely used for high quality assurance (QA), staging, and manufacturing environments. The improvement surroundings was nonetheless very handbook, and our group wanted to automate it to achieve as a lot flexibility as potential whereas accelerating the event effort. This is the rationale we determined to construct a CI/CD pipeline for DevOps. And the open supply model of Jenkins was the apparent alternative as a result of its flexibility, openness, highly effective plugin-capabilities, and ease of use.
In this text, I’ll share a step-by-step walkthrough on how one can construct a CI/CD pipeline utilizing Jenkins.
What is a pipeline?
Before leaping into the tutorial, it is useful to know one thing about CI/CD pipelines.
To begin, it’s useful to know that Jenkins itself just isn’t a pipeline. Just creating a brand new Jenkins job doesn’t assemble a pipeline. Think about Jenkins like a distant management—it is the place you click on a button. What occurs once you do click on a button is dependent upon what the distant is constructed to manage. Jenkins gives a manner for different software APIs, software program libraries, construct instruments, and so forth. to plug into Jenkins, and it executes and automates the duties. On its personal, Jenkins doesn’t carry out any performance however will get an increasing number of highly effective as different instruments are plugged into it.
A pipeline is a separate idea that refers back to the teams of occasions or jobs which can be related collectively in a sequence:
A pipeline is a sequence of occasions or jobs that may be executed.
The best method to perceive a pipeline is to visualise a sequence of phases, like this:
Here, you need to see two acquainted ideas: Stage and Step.
- Stage: A block that incorporates a collection of steps. A stage block might be named something; it’s used to visualise the pipeline course of.
- Step: A job that claims what to do. Steps are outlined inside a stage block.
In the instance diagram above, Stage 1 might be named “Build,” “Gather Information,” or no matter, and the same thought is utilized for the opposite stage blocks. “Step” merely says what to execute, and this generally is a easy print command (e.g., echo “Hello, World”), a program-execution command (e.g., java HelloWorld), a shell-execution command (e.g., chmod 755 Hello), or every other command—so long as it’s acknowledged as an executable command by means of the Jenkins surroundings.
The Jenkins pipeline is offered as a codified script sometimes referred to as a Jenkinsfile, though the file title might be completely different. Here is an instance of a easy Jenkins pipeline file.
// Example of Jenkins pipeline scriptpipeline {
phases // End of phases
} // End of pipeline
It’s straightforward to see the construction of a Jenkins pipeline from this pattern script. Note that some instructions, like java, javac, and mvn, usually are not out there by default, they usually have to be put in and configured by means of Jenkins. Therefore:
A Jenkins pipeline is the best way to execute a Jenkins job sequentially in an outlined manner by codifying it and structuring it inside a number of blocks that may embody a number of steps containing duties.
OK. Now that you simply perceive what a Jenkins pipeline is, I will present you how one can create and execute a Jenkins pipeline. At the top of the tutorial, you should have constructed a Jenkins pipeline like this:
How to construct a Jenkins pipeline
To make this tutorial simpler to observe, I created a pattern GitHub repository and a video tutorial.
Before beginning this tutorial, you will want:
- Java Development Kit: If you do not have already got it, set up a JDK and add it to the surroundings path so a Java command (like java jar) might be executed by means of a terminal. This is important to leverage the Java Web Archive (WAR) model of Jenkins that’s used on this tutorial (though you should use every other distribution).
- Basic pc operations: You ought to know how one can sort some code, execute primary Linux instructions by means of the shell, and open a browser.
Let’s get began.
Step 1: Download Jenkins
Navigate to the Jenkins download page. Scroll all the way down to Generic Java bundle (.struggle) and click on on it to obtain the file; reserve it someplace the place you’ll be able to find it simply. (If you select one other Jenkins distribution, the remainder of tutorial steps must be just about the identical, aside from Step 2.) The motive to make use of the WAR file is that it’s a one-time executable file that’s simply executable and detachable.
Step 2: Execute Jenkins as a Java binary
Open a terminal window and enter the listing the place you downloaded Jenkins with cd <your path>. (Before you proceed, be certain JDK is put in and added to the surroundings path.) Execute the next command, which can run the WAR file as an executable binary:
java -jar ./jenkins.struggle
If the whole lot goes easily, Jenkins must be up and operating on the default port 8080.
Step three: Create a brand new Jenkins job
Open an online browser and navigate to localhost:8080. Unless you’ve gotten a earlier Jenkins set up, it ought to go straight to the Jenkins dashboard. Click Create New Jobs. You may click on New Item on the left.
Step four: Create a pipeline job
In this step, you’ll be able to choose and outline what sort of Jenkins job you need to create. Select Pipeline and provides it a reputation (e.g., TestPipeline). Click OK to create a pipeline job.
You will see a Jenkins job configuration web page. Scroll down to search out Pipeline part. There are two methods to execute a Jenkins pipeline. One manner is by straight writing a pipeline script on Jenkins, and the opposite manner is by retrieving the Jenkins file from SCM (supply management administration). We will undergo each methods within the subsequent two steps.
Step 5: Configure and execute a pipeline job by means of a direct script
To execute the pipeline with a direct script, start by copying the contents of the sample Jenkinsfile from GitHub. Choose Pipeline script because the Destination and paste the Jenkinsfile contents in Script. Spend slightly time finding out how the Jenkins file is structured. Notice that there are three Stages: Build, Test, and Deploy, that are arbitrary and might be something. Inside every Stage, there are Steps; on this instance, they simply print some random messages.
Click Save to maintain the adjustments, and it ought to routinely take you again to the Job Overview.
To begin the method to construct the pipeline, click on Build Now. If the whole lot works, you will notice your first pipeline (just like the one under).
To see the output from the pipeline script construct, click on any of the Stages and click on Log. You will see a message like this.
Step 6: Configure and execute a pipeline job with SCM
Now, swap gears: In this step, you’ll Deploy the identical Jenkins job by copying the Jenkinsfile from a source-controlled GitHub. In the identical GitHub repository, decide up the repository URL by clicking Clone or obtain and copying its URL.
Click Configure to switch the prevailing job. Scroll to the Advanced Project Options setting, however this time, choose the Pipeline script from SCM choice within the Destination dropdown. Paste the GitHub repo’s URL within the Repository URL, and kind Jenkinsfile within the Script Path. Save by clicking the Save button.
To construct the pipeline, as soon as you’re again to the Task Overview web page, click on Build Now to execute the job once more. The end result would be the identical as earlier than, besides you’ve gotten one extra stage referred to as Declaration: Checkout SCM.
To see the pipeline’s output from the SCM construct, click on the Stage and think about the Log to test how the supply management cloning course of went.
Do greater than print messages
Congratulations! You’ve constructed your first Jenkins pipeline!
“But wait,” you say, “this is very limited. I cannot really do anything with it except print dummy messages.” That is OK. So far, this tutorial offered only a glimpse of what a Jenkins pipeline can do, however you’ll be able to prolong its capabilities by integrating it with different instruments. Here are a couple of concepts in your subsequent challenge:
- Build a multi-staged Java construct pipeline that takes from the phases of pulling dependencies from JAR repositories like Nexus or Artifactory, compiling Java codes, operating the unit assessments, packaging right into a JAR/WAR file, and deploying to a cloud server.
- Implement the superior code testing dashboard that can report again the well being of the challenge primarily based on the unit take a look at, load take a look at, and automatic person interface take a look at with Selenium.
- Construct a multi-pipeline or multi-user pipeline automating the duties of executing Ansible playbooks whereas permitting for approved customers to answer job in progress.
- Design an entire end-to-end DevOps pipeline that pulls the infrastructure useful resource recordsdata and configuration recordsdata saved in SCM like GitHub and executing the scripts by means of varied runtime packages.
Follow any of the tutorials on the finish of this text to get into these extra superior instances.
Manage Jenkins
From the principle Jenkins dashboard, click on Manage Jenkins.
Global instrument configuration
There are many choices out there, together with managing plugins, viewing the system log, and so forth. Click Global Tool Configuration.
Add extra capabilities
Here, you’ll be able to add the JDK path, Git, Gradle, and a lot extra. After you configure a instrument, it’s only a matter of including the command into your Jenkinsfile or executing it by means of your Jenkins script.
Where to go from right here?
This article put you on your method to making a CI/CD pipeline utilizing Jenkins, a cool open supply instrument. To discover out about lots of the different issues you are able to do with Jenkins, take a look at these different articles on Opensource.com:
You could also be excited about among the different articles I’ve written to complement your open supply journey:
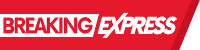