Object-relational mapping (ORM) makes life simpler for utility builders, in no small half as a result of it permits you to work together with a database in a language it’s possible you’ll know (comparable to Python) as a substitute of uncooked SQL queries. SQLAlchemy is a Python ORM toolkit that gives entry to SQL databases utilizing Python. It is a mature ORM instrument that provides the good thing about mannequin relationships, a robust question building paradigm, simple serialization, and rather more. Its ease of use, nonetheless, makes it simple to neglect what’s going on behind the scenes. Seemingly small decisions made utilizing SQLAlchemy can have essential efficiency implications.
This article explains a few of the prime efficiency points builders encounter when utilizing SQLAlchemy and repair them.
Retrieving a complete outcome set once you solely want the rely
Sometimes a developer simply wants a rely of outcomes, however as a substitute of using a database rely, all the outcomes are fetched and the rely is completed with len in Python.
rely = len(User.question.filter_by(acct_active=True).all())
Using SQLAlchemy’s rely technique as a substitute will do the rely on the server aspect, leading to far much less knowledge despatched to the shopper. Calling all() within the prior instance additionally ends in the instantiation of mannequin objects, which might turn out to be costly rapidly, given sufficient rows.
Unless greater than the rely is required, simply use the rely technique.
rely = User.question.filter_by(acct_active=True).rely()
Retrieving whole fashions once you solely want a couple of columns
In many circumstances, only some columns are wanted when issuing a question. Instead of returning whole mannequin situations, SQLAlchemy can fetch solely the columns you are serious about. This not solely reduces the quantity of information despatched but additionally avoids the necessity to instantiate whole objects. Working with tuples of column knowledge as a substitute of fashions will be fairly a bit sooner.
outcome = User.question.all()
for person in outcome:
print(person.identify, person.e mail)
Instead, choose solely what is required utilizing the with_entities technique.
outcome = User.question.with_entities(User.identify, User.e mail).all()
for (username, e mail) in outcome:
print(username, e mail)
Updating one object at a time inside a loop
Avoid utilizing loops to replace collections individually. While the database might execute a single replace in a short time, the roundtrip time between the appliance and database servers will rapidly add up. In normal, try for fewer queries the place affordable.
for person in users_to_update:
person.acct_active = True
db.session.add(person)
Use the majority replace technique as a substitute.
question = User.question.filter(person.id.in_([person.id for person in users_to_update]))
question.replace(, synchronize_session=False)
Triggering cascading deletes
ORM permits simple configuration of relationships on fashions, however there are some delicate behaviors that may be shocking. Most databases preserve relational integrity by means of international keys and varied cascade choices. SQLAlchemy means that you can outline fashions with international keys and cascade choices, however the ORM has its personal cascade logic that may preempt the database.
Consider the next fashions.
class Artist(Base):
__tablename__ = "artist"id = Column(Integer, primary_key=True)
songs = relationship("Song", cascade="all, delete")class Song(Base):
__tablename__ = "song"id = Column(Integer, primary_key=True)
artist_id = Column(Integer, ForeignKey("artist.id", ondelete="CASCADE"))
Deleting artists will trigger the ORM to challenge delete queries on the Song desk, thus stopping the deletes from taking place on account of the international key. This conduct can turn out to be a bottleneck with complicated relationships and a lot of information.
Include the passive_deletes possibility to make sure that the database is managing relationships. Be certain, nonetheless, that your database is able to this. SQLite, for instance, doesn’t handle international keys by default.
songs = relationship("Song", cascade="all, delete", passive_deletes=True)
Relying on lazy loading when keen loading must be used
Lazy loading is the default SQLAlchemy strategy to relationships. Building from the final instance, this means that loading an artist doesn’t concurrently load his or her songs. This is normally a good suggestion, however the separate queries will be wasteful if sure relationships at all times should be loaded.
Popular serialization frameworks like Marshmallow can set off a cascade of queries if relationships are allowed to load in a lazy vogue.
There are a couple of methods to manage this conduct. The easiest technique is thru the connection operate itself.
songs = relationship("Song", lazy="joined", cascade="all, delete")
This will trigger a left be a part of to be added to any question for artists, and because of this, the songs assortment will probably be instantly accessible. Although extra knowledge is returned to the shopper, there are probably far fewer roundtrips.
SQLAlchemy affords finer-grained management for conditions the place such a blanket strategy cannot be taken. The joinedload() operate can be utilized to toggle joined loading on a per-query foundation.
from sqlalchemy.orm import joinedloadartists = Artist.question.choices(joinedload(Artist.songs))
print(artists.songs) # Does not incur a roundtrip to load
Using the ORM for a bulk file import
The overhead of developing full mannequin situations turns into a serious bottleneck when importing 1000’s of information. Imagine, for instance, loading 1000’s of tune information from a file the place every tune has first been transformed to a dictionary.
for tune in songs:
db.session.add(Song(**tune))
Instead, bypass the ORM and use simply the parameter binding performance of core SQLAlchemy.
batch = []
insert_stmt = Song.__table__.insert()
for tune in songs:
if len(batch) > 1000:
db.session.execute(insert_stmt, batch)
batch.clear()
batch.append(tune)
if batch:
db.session.execute(insert_stmt, batch)
Keep in thoughts that this technique naturally skips any client-side ORM logic you would possibly depend upon, comparable to Python-based column defaults. While this technique is quicker than loading objects as full mannequin situations, your database might have bulk loading strategies which can be sooner. PostgreSQL, for instance, has the COPY command that gives maybe the perfect efficiency for loading giant numbers of information.
Calling commit or flush prematurely
There are many events when you want to affiliate a baby file to its father or mother, or vice versa. One apparent means of doing that is to flush the session in order that the file in query will probably be assigned an ID.
artist = Artist(identify="Bob Dylan")
tune = Song(title="Mr. Tambourine Man")db.session.add(artist)
db.session.flush()tune.artist_id = artist.id
Committing or flushing greater than as soon as per request is normally pointless and undesirable. A database flush includes forcing disk writes on the database server, and in most circumstances, the shopper will block till the server can acknowledge that the information has been written.
SQLAlchemy can monitor relationships and handle keys behind the scenes.
artist = Artist(identify="Bob Dylan")
tune = Song(title="Mr. Tambourine Man")artist.songs.append(tune)
Wrapping up
I hope this listing of frequent pitfalls will help you keep away from these points and preserve your utility operating easily. As at all times, when diagnosing a efficiency drawback, measurement is vital. Most databases provide efficiency diagnostics that may make it easier to pinpoint points, such because the PostgreSQL pg_stat_statements module.
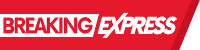