Protocol buffers (Protobufs), like XML and JSON, enable functions, which can be written in numerous languages and operating on completely different platforms, to alternate knowledge. For instance, a sending software written in Go might encode a Go-specific gross sales order in Protobuf, which a receiver written in Java then might decode to get a Java-specific illustration of the obtained order. Here is a sketch of the structure over a community connection:
Go gross sales order--->Pbuf-encode--->network--->Pbuf-decode--->Java gross sales order
Protobuf encoding, in distinction to its XML and JSON counterparts, is binary quite than textual content, which might complicate debugging. However, because the code examples on this article affirm, the Protobuf encoding is considerably extra environment friendly in dimension than both XML or JSON encoding.
Protobuf is environment friendly in one other approach. At the implementation stage, Protobuf and different encoding programs serialize and deserialize structured knowledge. Serialization transforms a language-specific knowledge construction right into a bytestream, and deserialization is the inverse operation that transforms a bytestream again right into a language-specific knowledge construction. Serialization and deserialization might develop into the bottleneck in knowledge interchange as a result of these operations are CPU-intensive. Efficient serialization and deserialization is one other Protobuf design aim.
Recent encoding applied sciences, similar to Protobuf and FlatBuffers, derive from the DCE/RPC (Distributed Computing Environment/Remote Procedure Call) initiative of the early 1990s. Like DCE/RPC, Protobuf contributes to each the IDL (interface definition language) and the encoding layer in knowledge interchange.
This article will take a look at these two layers then present code examples in Go and Java to flesh out Protobuf particulars and present that Protobuf is straightforward to make use of.
Protobuf as an IDL and encoding layer
DCE/RPC, like Protobuf, is designed to be language- and platform-neutral. The applicable libraries and utilities enable any language and platform to play within the DCE/RPC enviornment. Furthermore, the DCE/RPC structure is elegant. An IDL doc is the contract between the distant process on the one facet and callers on the opposite facet. Protobuf, too, facilities on an IDL doc.
An IDL doc is textual content and, in DCE/RPC, makes use of primary C syntax together with syntactic extensions for metadata (sq. brackets) and some new key phrases similar to interface. Here is an instance:
[uuid (2d6ead46-05e3-11ca-7dd1-426909beabcd), version(1.0)]
interface echo
This IDL doc declares a process named echo, which takes three arguments: the [in] arguments of sort handle_t (implementation pointer) and idl_char (array of ASCII characters) are handed to the distant process, whereas the [out] argument (additionally a string) is handed again from the process. In this instance, the echo process doesn’t explicitly return a price (the void to the left of echo) however might achieve this. A return worth, along with a number of [out] arguments, permits the distant process to return arbitrarily many values. The subsequent part introduces a Protobuf IDL, which differs in syntax however likewise serves as a contract in knowledge interchange.
The IDL doc, in each DCE/RPC and Protobuf, is the enter to utilities that create the infrastructure code for exchanging knowledge:
IDL document--->DCE/PRC or Protobuf utilities--->help code for knowledge interchange
As comparatively simple textual content, the IDL is likewise human-readable documentation concerning the specifics of the info interchange—specifically, the variety of knowledge gadgets exchanged and the info sort of every merchandise.
Protobuf can utilized in a contemporary RPC system similar to gRPC; however Protobuf by itself offers solely the IDL layer and the encoding layer for messages handed from a sender to a receiver. Protobuf encoding, just like the DCE/RPC authentic, is binary however extra environment friendly.
At current, XML and JSON encodings nonetheless dominate in knowledge interchange by applied sciences similar to net providers, which make use of in-place infrastructure similar to net servers, transport protocols (e.g., TCP, HTTP), and customary libraries and utilities for processing XML and JSON paperwork. Moreover, database programs of assorted flavors can retailer XML and JSON paperwork, and even legacy relational programs readily generate XML encodings of question outcomes. Every general-purpose programming language now has libraries that help XML and JSON. What, then, recommends a return to a binary encoding system similar to Protobuf?
Consider the unfavorable decimal worth -128. In the two’s complement binary illustration, which dominates throughout programs and languages, this worth could be saved in a single Eight-bit byte: 10000000. The textual content encoding of this integer worth in XML or JSON requires a number of bytes. For instance, UTF-Eight encoding requires 4 bytes for the string, actually -128, which is one byte per character (in hex, the values are 0x2d, 0x31, 0x32, and 0x38). XML and JSON additionally add markup characters, similar to angle brackets and braces, to the combo. Details about Protobuf encoding are forthcoming, however the focal point now could be a basic one: Text encodings are typically considerably much less compact than binary ones.
A code instance in Go utilizing Protobuf
My code examples deal with Protobuf quite than RPC. Here is an summary of the primary instance:
- The IDL file named dataitem.proto defines a Protobuf message with six fields of various sorts: integer values with completely different ranges, floating-point values of a hard and fast dimension, and strings of two completely different lengths.
- The Protobuf compiler makes use of the IDL file to generate a Go-specific model (and, later, a Java-specific model) of the Protobuf message along with supporting capabilities.
- A Go app populates the native Go knowledge construction with randomly generated values after which serializes the end result to a neighborhood file. For comparability, XML and JSON encodings are also serialized to native recordsdata.
- As a check, the Go software reconstructs an occasion of its native knowledge construction by deserializing the contents of the Protobuf file.
- As a language-neutrality check, the Java software additionally deserializes the contents of the Protobuf file to get an occasion of a local knowledge construction.
This IDL file and two Go and one Java supply recordsdata can be found as a ZIP file on my website.
The all-important Protobuf IDL doc is proven under. The doc is saved within the file dataitem.proto, with the customary .proto extension.
Example 1. Protobuf IDL doc
syntax = "proto3";bundle principal;
message DataItem
int64 oddA = 1;
int64 evenA = 2;
int32 oddB = three;
int32 evenB = four;
float small = 5;
float large = 6;
string quick = 7;
string lengthy = Eight;
The IDL makes use of the present proto3 quite than the sooner proto2 syntax. The bundle title (on this case, principal) is optionally available however customary; it’s used to keep away from title conflicts. The structured message incorporates eight fields, every of which has a Protobuf knowledge sort (e.g., int64, string), a reputation (e.g., oddA, quick), and a numeric tag (aka key) after the equals signal =. The tags, that are 1 by Eight on this instance, are distinctive integer identifiers that decide the order by which the fields are serialized.
Protobuf messages could be nested to arbitrary ranges, and one message could be the sector sort within the different. Here’s an instance that makes use of the DataItem message as a subject sort:
message DataItems
repeated DataItem merchandise = 1;
A single DataItems message consists of repeated (none or extra) DataItem messages.
Protobuf additionally helps enumerated sorts for readability:
enum PartnershipStatus
The reserved qualifier ensures that the numeric values used to implement the three symbolic names can’t be reused.
To generate a language-specific model of a number of declared Protobuf message constructions, the IDL file containing these is handed to the protoc compiler (out there within the Protobuf GitHub repository). For the Go code, the supporting Protobuf library could be put in within the normal approach (with % because the command-line immediate):
% go get github.com/golang/protobuf/proto
The command to compile the Protobuf IDL file dataitem.proto into Go supply code is:
% protoc --go_out=. dataitem.proto
The flag –go_out directs the compiler to generate Go supply code; there are related flags for different languages. The end result, on this case, is a file named dataitem.pb.go, which is sufficiently small that the necessities could be copied right into a Go software. Here are the necessities from the generated code:
var _ = proto.Marshalsort DataItem struct
func (m *DataItem) Reset() *m = DataItem
func (m *DataItem) String() string
func (*DataItem) ProtoMessage()
func init()
The compiler-generated code has a Go construction DataItem, which exports the Go fields—the names are actually capitalized—that match the names declared within the Protobuf IDL. The construction fields have customary Go knowledge sorts: int32, int64, float32, and string. At the tip of every subject line, as a string, is metadata that describes the Protobuf sorts, offers the numeric tags from the Protobuf IDL doc, and offers details about JSON, which is mentioned later.
There are additionally capabilities; a very powerful is proto.Marshal for serializing an occasion of the DataItem construction into Protobuf format. The helper capabilities embrace Reset, which clears a DataItem construction, and String, which produces a one-line string illustration of a DataItem.
The metadata that describes Protobuf encoding deserves a better look earlier than analyzing the Go program in additional element.
Protobuf encoding
A Protobuf message is structured as a group of key/worth pairs, with the numeric tag as the important thing and the corresponding subject as the worth. The subject names, similar to oddA and small, are for human readability, however the protoc compiler does use the sector names in producing language-specific counterparts. For instance, the oddA and small names within the Protobuf IDL develop into the fields OddA and Small, respectively, within the Go construction.
The keys and their values each get encoded, however with an essential distinction: some numeric values have a fixed-size encoding of 32 or 64 bits, whereas others (together with the message tags) are varint encoded—the variety of bits depends upon the integer’s absolute worth. For instance, the integer values 1 by 15 require Eight bits to encode in varint, whereas the values 16 by 2047 require 16 bits. The varint encoding, related in spirit (however not intimately) to UTF-Eight encoding, favors small integer values over massive ones. (For an in depth evaluation, see the Protobuf encoding guide.) The upshot is that a Protobuf message ought to have small integer values in fields, if attainable, and as few keys as attainable, however one key per subject is unavoidable.
Table 1 under offers the gist of Protobuf encoding:
Table 1. Protobuf knowledge sorts
Encoding | Sample sorts | Length |
---|---|---|
varint |
int32, uint32, int64 |
Variable size |
fastened |
fastened32, float, double |
Fixed 32-bit or 64-bit size |
byte sequence |
string, bytes |
Sequence size |
Integer sorts that aren’t explicitly fastened are varint encoded; therefore, in a varint sort similar to uint32 (u for unsigned), the quantity 32 describes the integer’s vary (on this case, zero to 232 – 1) quite than its bit dimension, which differs relying on the worth. For fastened sorts similar to fastened32 or double, in contrast, the Protobuf encoding requires 32 and 64 bits, respectively. Strings in Protobuf are byte sequences; therefore, the dimensions of the sector encoding is the size of the byte sequence.
Another effectivity deserves point out. Recall the sooner instance by which a DataItems message consists of repeated DataItem situations:
message DataItems
repeated DataItem merchandise = 1;
The repeated signifies that the DataItem situations are packed: the gathering has a single tag, on this case, 1. A DataItems message with repeated DataItem situations is thus extra environment friendly than a message with a number of however separate DataItem fields, every of which might require a tag of its personal.
With this background in thoughts, let’s return to the Go program.
The dataItem program intimately
The dataItem program creates a DataItem occasion and populates the fields with randomly generated values of the suitable sorts. Go has a rand bundle with capabilities for producing pseudo-random integer and floating-point values, and my randString perform generates pseudo-random strings of specified lengths from a personality set. The design aim is to have a DataItem occasion with subject values of various sorts and bit sizes. For instance, the OddA and EvenA values are 64-bit non-negative integer values of wierd and even parity, respectively; however the OddB and EvenB variants are 32 bits in dimension and maintain small integer values between zero and 2047. The random floating-point values are 32 bits in dimension, and the strings are 16 (Short) and 32 (Long) characters in size. Here is the code section that populates the DataItem construction with random values:
// variable-length integers
n1 := rand.Int63() // larger integer
if (n1 & 1) == zero n1++ // guarantee it is odd
...
n3 := rand.Int31() % UpperBound // smaller integer
if (n3 & 1) == zero // guarantee it is odd// fixed-length floats
...
t1 := rand.Float32()
t2 := rand.Float32()
...
// strings
str1 := randString(StrShort)
str2 := randString(StrLong)// the message
dataItem := &DataItem
Once created and populated with values, the DataItem occasion is encoded in XML, JSON, and Protobuf, with every encoding written to a neighborhood file:
func encodeAndserialize(dataItem *DataItem)
The three serializing capabilities use the time period marshal, which is roughly synonymous with serialize. As the code signifies, every of the three Marshal capabilities returns an array of bytes, which then are written to a file. (Possible errors are ignored for simplicity.) On a pattern run, the file sizes had been:
dataitem.xml: 262 bytes
dataitem.json: 212 bytes
dataitem.pbuf: 88 bytes
The Protobuf encoding is considerably smaller than the opposite two. The XML and JSON serializations may very well be lowered barely in dimension by eliminating indentation characters, on this case, blanks and newlines.
Below is the dataitem.json file ensuing ultimately from the json.MarshalIndent name, with added feedback beginning with ##:
Although the serialized knowledge goes into native recordsdata, the identical method can be used to write down the info to the output stream of a community connection.
Testing serialization/deserialization
The Go program subsequent runs an elementary check by deserializing the bytes, which had been written earlier to the dataitem.pbuf file, right into a DataItem occasion. Here is the code section, with the error-checking components eliminated:
filebytes, err := ioutil.LearnFile(PbufFile) // get the bytes from the file
...
testItem.Reset() // clear the DataItem construction
err = proto.Unmarshal(filebytes, testItem) // deserialize right into a DataItem occasion
The proto.Unmarshal perform for deserializing Protbuf is the inverse of the proto.Marshal perform. The authentic DataItem and the deserialized clone are printed to verify an actual match:
Original:
2041519981506242154 3041486079683013705 1192 1879
zero.572123 zero.326855
boPb#T0O8Xd&Ps5EnSZqDg4Qztvo7IIs 9vH66AiGSQgCDxk&Deserialized:
2041519981506242154 3041486079683013705 1192 1879
zero.572123 zero.326855
boPb#T0O8Xd&Ps5EnSZqDg4Qztvo7IIs 9vH66AiGSQgCDxk&
A Protobuf consumer in Java
The instance in Java is to verify Protobuf’s language neutrality. The authentic IDL file may very well be used to generate the Java help code, which entails nested lessons. To suppress warnings, nonetheless, a slight addition could be made. Here is the revision, which specifies a DataMsg because the title for the outer class, with the inside class mechanically named DataItem after the Protobuf message:
syntax = "proto3";bundle principal;
choice java_outer_classname = "DataMsg";
message DataItem {
...
With this alteration in place, the protoc compilation is similar as earlier than, besides the specified output is now Java quite than Go:
% protoc --java_out=. dataitem.proto
The ensuing supply file (in a subdirectory named principal) is DataMsg.java and about 1,120 traces in size: Java just isn’t terse. Compiling after which operating the Java code requires a JAR file with the library help for Protobuf. This file is obtainable within the Maven repository.
With the items in place, my check code is comparatively quick (and out there within the ZIP file as Main.java):
bundle principal;
import java.io.FileInputStream;public class Main
public static void principal(String[] args)
String path = "dataitem.pbuf"; // from the Go program's serialization
strive
catch(Exception e)
Production-grade testing can be way more thorough, after all, however even this preliminary check confirms the language-neutrality of Protobuf: the dataitem.pbuf file outcomes from the Go program’s serialization of a Go DataItem, and the bytes on this file are deserialized to provide a DataItem occasion in Java. The output from the Java check is similar as that from the Go check.
Wrapping up with the numPairs program
Let’s finish with an instance that highlights Protobuf effectivity but in addition underscores the associated fee concerned in any encoding expertise. Consider this Protobuf IDL file:
syntax = "proto3";
bundle principal;message NumPairs
message NumPair
int32 odd = 1;
int32 even = 2;
A NumPair message consists of two int32 values along with an integer tag for every subject. A NumPairs message is a sequence of embedded NumPair messages.
The numPairs program in Go (under) creates 2 million NumPair situations, with every appended to the NumPairs message. This message could be serialized and deserialized within the normal approach.
Example 2. The numPairs program
bundle principalimport (
"math/rand"
"time"
"encoding/xml"
"encoding/json"
"io/ioutil"
"github.com/golang/protobuf/proto"
)// protoc-generated code: begin
var _ = proto.Marshal
sort NumPairs struct
Pair []*NumPair `protobuf:"bytes,1,rep,name=pair" json:"pair,omitempty"`func (m *NumPairs) Reset() *m = NumPairs
func (m *NumPairs) String() string
func (*NumPairs) ProtoMessage()
func (m *NumPairs) GetPair() []*NumPairsort NumPair struct
Odd int32 `protobuf:"varint,1,opt,name=odd" json:"odd,omitempty"`
Even int32 `protobuf:"varint,2,opt,name=even" json:"even,omitempty"`func (m *NumPair) Reset()
func (m *NumPair) String() string
func (*NumPair) ProtoMessage()
func init()
// protoc-generated code: endvar numPairsStruct NumPairs
var numPairs = &numPairsStructfunc encodeAndserialize()
// XML encoding
filename := "./pairs.xml"
bytes, _ := xml.MarshalIndent(numPairs, "", " ")
ioutil.WriteFile(filename, bytes, 0644)// JSON encoding
filename = "./pairs.json"
bytes, _ = json.MarshalIndent(numPairs, "", " ")
ioutil.WriteFile(filename, bytes, 0644)// ProtoBuf encoding
filename = "./pairs.pbuf"
bytes, _ = proto.Marshal(numPairs)
ioutil.WriteFile(filename, bytes, 0644)const HowMany = 200 * 100 * 100 // two million
func principal()
rand.Seed(time.Now().UnixNano())// uncomment the modulus operations to get the extra environment friendly model
for i := zero; i < HowMany; i++
encodeAndserialize()
The randomly generated odd and even values in every NumPair vary from zero to 2 billion and alter. In phrases of uncooked quite than encoded knowledge, the integers generated within the Go program add as much as 16MB: two integers per NumPair for a complete of four million integers in all, and every worth is 4 bytes in dimension.
For comparability, the desk under has entries for the XML, JSON, and Protobuf encodings of the two million NumPair situations within the pattern NumsPairs message. The uncooked knowledge is included, as effectively. Because the numPairs program generates random values, output differs throughout pattern runs however is near the sizes proven within the desk.
Table 2. Encoding overhead for 16MB of integers
Encoding | File | Byte dimension | Pbuf/different ratio |
---|---|---|---|
None |
pairs.uncooked |
16MB |
169% |
Protobuf |
pairs.pbuf |
27MB |
— |
JSON |
pairs.json |
100MB |
27% |
XML |
pairs.xml |
126MB |
21% |
As anticipated, Protobuf shines subsequent to XML and JSON. The Protobuf encoding is a couple of quarter of the JSON one and a couple of fifth of the XML one. But the uncooked knowledge clarify that Protobuf incurs the overhead of encoding: the serialized Protobuf message is 11MB bigger than the uncooked knowledge. Any encoding, together with Protobuf, entails structuring the info, which unavoidably provides bytes.
Each of the serialized 2 million NumPair situations entails 4 integer values: one apiece for the Even and Odd fields within the Go construction, and one tag per every subject within the Protobuf encoding. As uncooked quite than encoded knowledge, this may come to 16 bytes per occasion, and there are 2 million situations within the pattern NumPairs message. But the Protobuf tags, just like the int32 values within the NumPair fields, use varint encoding and, due to this fact, range in byte size; specifically, small integer values (which embrace the tags, on this case) require fewer than 4 bytes to encode.
If the numPairs program is revised in order that the 2 NumPair fields maintain values lower than 2048, which have encodings of both one or two bytes, then the Protobuf encoding drops from 27MB to 16MB—the very dimension of the uncooked knowledge. The desk under summarizes the brand new encoding sizes from a pattern run.
Table three. Encoding with 16MB of integers < 2048
Encoding | File | Byte dimension | Pbuf/different ratio |
---|---|---|---|
None |
pairs.uncooked |
16MB |
100% |
Protobuf |
pairs.pbuf |
16MB |
— |
JSON |
pairs.json |
77MB |
21% |
XML |
pairs.xml |
103MB |
15% |
In abstract, the modified numPairs program, with subject values lower than 2048, reduces the four-byte dimension for every integer worth within the uncooked knowledge. But the Protobuf encoding nonetheless requires tags, which add bytes to the Protobuf message. Protobuf encoding does have a value in message dimension, however this value could be lowered by the varint issue if comparatively small integer values, whether or not in fields or keys, are being encoded.
For reasonably sized messages consisting of structured knowledge with blended sorts—and comparatively small integer values—Protobuf has a transparent benefit over choices similar to XML and JSON. In different circumstances, the info might not be suited to Protobuf encoding. For instance, if two functions have to share an enormous set of textual content data or massive integer values, then compression quite than encoding expertise could be the technique to go.
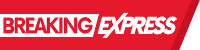