Awk is the ever-present Unix command for scanning and processing textual content containing predictable patterns. However, as a result of it options capabilities, it is also justifiably referred to as a programming language.
Confusingly, there’s multiple awk. (Or, in the event you imagine there might be just one, then there are a number of clones.) There’s awk, the unique program written by Aho, Weinberger, and Kernighan, after which there’s nawk, mawk, and the GNU model, gawk. The GNU model of awk is a extremely transportable, free software program model of the utility with a number of distinctive options, so this text is about GNU awk.
While its official title is gawk, on GNU+Linux techniques it is aliased to awk and serves because the default model of that command. On different techniques that do not ship with GNU awk, you will need to set up it and consult with it as gawk, somewhat than awk. This article makes use of the phrases awk and gawk interchangeably.
Being each a command and a programming language makes awk a robust device for duties that may in any other case be left to kind, minimize, uniq, and different widespread utilities. Luckily, there’s plenty of room in open supply for redundancy, so in the event you’re confronted with the query of whether or not or to not use awk, the reply might be a stable “maybe.”
The great thing about awk’s flexibility is that in the event you’ve already dedicated to utilizing awk for a activity, then you’ll be able to most likely keep in awk it doesn’t matter what comes up alongside the way in which. This contains the everlasting must kind information in a means aside from the order it was delivered to you.
Sample set
Before exploring awk’s sorting strategies, generate a pattern dataset to make use of. Keep it easy in order that you do not get distracted by edge circumstances and unintended complexity. This is the pattern set this text makes use of:
Aptenodytes;forsteri;Miller,JF;1778;Emperor
Pygoscelis;papua;Wagler;1832;Gentoo
Eudyptula;minor;Bonaparte;1867;Little Blue
Spheniscus;demersus;Brisson;1760;African
Megadyptes;antipodes;Milne-Edwards;1880;Yellow-eyed
Eudyptes;chrysocome;Viellot;1816;Sothern Rockhopper
Torvaldis;linux;Ewing,L;1996;Tux
It’s a small dataset, but it surely affords an excellent number of information varieties:
- A genus and species title, that are related to each other however thought-about separate
- A surname, generally with first initials after a comma
- An integer representing a date
- An arbitrary time period
- All fields separated by semi-colons
Depending in your academic background, you could think about this a 2D array or a desk or only a line-delimited assortment of information. How you consider it’s as much as you, as a result of awk does not count on something greater than textual content. It’s as much as you to inform awk the way you wish to parse it.
The kind cheat
If you simply wish to kind a textual content dataset by a particular, definable discipline (consider a “cell” in a spreadsheet), then you should utilize the sort command.
Fields and data
Regardless of the format of your enter, you will need to discover patterns in it to be able to concentrate on the components of the information which might be vital to you. In this instance, the information is delimited by two components: strains and fields. Each new line represents a brand new report, as you’ll probably see in a spreadsheet or database dump. Within every line, there are distinct fields (consider them as cells in a spreadsheet) which might be separated by semicolons (;).
Awk processes one report at a time, so when you’re structuring the directions you’ll give to awk, you’ll be able to concentrate on only one line. Establish what you wish to do with one line, then check it (both mentally or with awk) on the following line and some extra. You’ll find yourself with an excellent speculation on what your awk script should do with a view to give you the information construction you need.
In this case, it is easy to see that every discipline is separated by a semicolon. For simplicity’s sake, assume you wish to kind the listing by the very first discipline of every line.
Before you’ll be able to kind, you will need to have the ability to focus awk on simply the primary discipline of every line, in order that’s step one. The syntax of an awk command in a terminal is awk, adopted by related choices, adopted by your awk command, and ending with the file of information you wish to course of.
$ awk --field-separator=";" 'print $1;' penguins.listing
Aptenodytes
Pygoscelis
Eudyptula
Spheniscus
Megadyptes
Eudyptes
Torvaldis
Because the sector separator is a personality that has particular that means to the Bash shell, you will need to enclose the semicolon in quotes or precede it with a backslash. This command is helpful solely to show which you could concentrate on a particular discipline. You can strive the identical command utilizing the variety of one other discipline to view the contents of one other “column” of your information:
$ awk --field-separator=";" '' penguins.listing
Miller,JF
Wagler
Bonaparte
Brisson
Milne-Edwards
Viellot
Ewing,L
Nothing has been sorted but, however that is good groundwork.
Scripting
Awk is greater than only a command; it is a programming language with indices and arrays and capabilities. That’s vital as a result of it means you’ll be able to seize a listing of fields you wish to kind by, retailer the listing in reminiscence, course of it, after which print the ensuing information. For a posh sequence of actions resembling this, it is simpler to work in a textual content file, so create a brand new file referred to as kind.awk and enter this textual content:
#!/bin/gawk -fBEGIN
FS=";";
This establishes the file as an awk script that executes the strains contained within the file.
The BEGIN assertion is a particular setup operate supplied by awk for duties that must happen solely as soon as. Defining the built-in variable FS, which stands for discipline separator and is similar worth you set in your awk command with –field-separator, solely must occur as soon as, so it is included within the BEGIN assertion.
Arrays in awk
You already know easy methods to collect the values of a particular discipline by utilizing the $ notation together with the sector quantity, however on this case, you could retailer it in an array somewhat than print it to the terminal. This is completed with an awk array. The vital factor about an awk array is that it incorporates keys and values. Imagine an array about this text; it might look one thing like this: creator:”seth”,title:”How to sort with awk”,size:1200. Elements like creator and title and size are keys, with the next contents being values.
The benefit to this within the context of sorting is which you could assign any discipline as the important thing and any report as the worth, after which use the built-in awk operate asorti() (kind by index) to kind by the important thing. For now, assume arbitrarily that you simply solely wish to kind by the second discipline.
Awk statements not preceded by the particular key phrases BEGIN or END are loops that occur at every report. This is the a part of the script that scans the information for patterns and processes it accordingly. Each time awk turns its consideration to a report, statements in (except preceded by BEGIN or END) are executed.
To add a key and worth to an array, create a variable (on this instance script, I name it ARRAY, which is not terribly unique, however very clear) containing an array, after which assign it a key in brackets and a price with an equals signal (=).
# dump every discipline into an array
ARRAY[$2] = $R;
In this assertion, the contents of the second discipline ($2) are used as the important thing time period, and the present report ($R) is used as the worth.
The asorti() operate
In addition to arrays, awk has a number of fundamental capabilities that you should utilize as fast and simple options for widespread duties. One of the capabilities launched in GNU awk, asorti(), supplies the power to kind an array by key (or index) or worth.
You can solely kind the array as soon as it has been populated, that means that this motion should not happen with each new report however solely the ultimate stage of your script. For this function, awk supplies the particular END key phrase. The inverse of BEGIN, an END assertion occurs solely as soon as and solely in any case data have been scanned.
Add this to your script:
END
The asorti() operate takes the contents of ARRAY, kinds it by index, and locations the ends in a brand new array referred to as SARRAY (an arbitrary title I invented for this text, that means Sorted ARRAY).
Next, the variable j (one other arbitrary title) is assigned the outcomes of the size() operate, which counts the variety of objects in SARRAY.
Finally, use a for loop to iterate via every merchandise in SARRAY utilizing the printf() operate to print every key, adopted by the corresponding worth of that key in ARRAY.
Running the script
To run your awk script, make it executable:
$ chmod +x sorter.awk
And then run it in opposition to the penguin.listing pattern information:
$ ./sorter.awk penguins.listing
antipodes Megadyptes;antipodes;Milne-Edwards;1880;Yellow-eyed
chrysocome Eudyptes;chrysocome;Viellot;1816;Sothern Rockhopper
demersus Spheniscus;demersus;Brisson;1760;African
forsteri Aptenodytes;forsteri;Miller,JF;1778;Emperor
linux Torvaldis;linux;Ewing,L;1996;Tux
minor Eudyptula;minor;Bonaparte;1867;Little Blue
papua Pygoscelis;papua;Wagler;1832;Gentoo
As you’ll be able to see, the information is sorted by the second discipline.
This is a little bit restrictive. It can be higher to have the flexibleness to decide on at runtime which discipline you wish to use as your sorting key so you can use this script on any dataset and get significant outcomes.
Adding command choices
You can add a command variable to an awk script by utilizing the literal worth var in your script. Change your script in order that your iterative clause makes use of var when creating your array:
# dump every discipline into an array
ARRAY[$var] = $R;
Try operating the script in order that it kinds by the third discipline by utilizing the -v var choice if you execute it:
$ ./sorter.awk -v var=three penguins.listing
Bonaparte Eudyptula;minor;Bonaparte;1867;Little Blue
Brisson Spheniscus;demersus;Brisson;1760;African
Ewing,L Torvaldis;linux;Ewing,L;1996;Tux
Miller,JF Aptenodytes;forsteri;Miller,JF;1778;Emperor
Milne-Edwards Megadyptes;antipodes;Milne-Edwards;1880;Yellow-eyed
Viellot Eudyptes;chrysocome;Viellot;1816;Sothern Rockhopper
Wagler Pygoscelis;papua;Wagler;1832;Gentoo
Fixes
This article has demonstrated easy methods to kind information in pure GNU awk. The script might be improved so, if it is helpful to you, spend a while researching awk functions on gawk’s man web page and customizing the script for higher output.
Here is the whole script up to now:
#!/usr/bin/awk -f
# GPLv3 seems right here
# utilization: ./sorter.awk -v var=NUM FILEBEGIN FS=";";
# dump every discipline into an array
ARRAY[$var] = $R;END
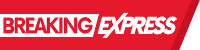