Django is the excellent net framework by which all different frameworks are measured. One of the preferred names in Python API improvement, Django has surged in recognition since its begin in 2005.
Django is maintained by the Django Software Foundation and has skilled nice neighborhood help, with over 11,600 members worldwide. On Stack Overflow, Django has round 191,000 tagged questions. Websites like Spotify, YouTube, and Instagram depend on Django for utility and information administration.
This article demonstrates a easy API to fetch information from a server utilizing the GET technique of the HTTP protocol.
Set up a undertaking
First, create a construction in your Django utility; you are able to do this at any location in your system:
$ mkdir myproject
$ cd myproject
Then, create a digital setting to isolate bundle dependencies regionally inside the undertaking listing:
$ python3 -m venv env
$ supply env/bin/activate
On Windows, use the command envScriptsactivate to activate your Python digital setting.
Install Django and the Django REST framework
Next, set up the Python modules for Django and Django REST:
$ pip3 set up django
$ pip3 set up djangorestframework
Instantiate a brand new Django undertaking
Now that you’ve got a piece setting in your app, you have to instantiate a brand new Django undertaking. Unlike a minimal framework like Flask, Django consists of devoted instructions for this course of (word the trailing . character within the first command):
$ django-admin startproject tutorial .
$ cd tutorial
$ django-admin startapp quickstart
Django makes use of a database as its backend, so it’s best to sync your database earlier than starting improvement. The database could be managed with the handle.py script that was created once you ran the django-admin command. Because you are presently within the tutorial listing, use the ../ notation to run the script, situated one listing up:
$ python3 ../handle.py makemigrations
No modifications detected
$ python4 ../handle.py migrate
Operations to carry out:
Apply all migrations: admin, auth, contenttypes, classes
Running migrations:
Applying contenttypes.0001_initial... OK
Applying auth.0001_initial... OK
Applying admin.0001_initial... OK
Applying admin.0002_logentry_remove_auto_add... OK
Applying admin.0003_logentry_add_action_flag_choices... OK
Applying contenttypes.0002_remove_content_type_name... OK
Applying auth.0002_alter_permission_name_max_length... OK
Applying auth.0003_alter_user_email_max_length... OK
Applying auth.0004_alter_user_username_opts... OK
Applying auth.0005_alter_user_last_login_null... OK
Applying auth.0006_require_contenttypes_0002... OK
Applying auth.0007_alter_validators_add_error_messages... OK
Applying auth.0008_alter_user_username_max_length... OK
Applying auth.0009_alter_user_last_name_max_length... OK
Applying auth.0010_alter_group_name_max_length... OK
Applying auth.0011_update_proxy_permissions... OK
Applying classes.0001_initial... OK
Create customers in Django
Create an preliminary person named admin with the instance password of password123:
$ python3 ../handle.py createsuperuser
--e-mail admin@instance.com
--username admin
Create a password once you’re prompted.
Implement serializers and views in Django
For Django to have the ability to cross data over to an HTTP GET request, the knowledge object have to be translated into legitimate response information. Django implements serializers for this.
In your undertaking, outline some serializers by creating a brand new module named quickstart/serializers.py, which you may use for information representations:
from django.contrib.auth.fashions import User, Group
from rest_framework import serializersclass UserSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
mannequin = User
fields = ['url', 'username', 'e-mail', 'teams']class GroupSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
mannequin = Group
fields = ['url', 'title']
A view in Django is a operate that takes an online request and returns an online response. The response could be HTML, or an HTTP redirect, or an HTTP error, a JSON or XML doc, a picture or TAR file, or anything you will get over the web. To create a view, open quickstart/views.py and enter the next code. This file already exists and has some boilerplate textual content in it, so hold that and append this textual content to the file:
from django.contrib.auth.fashions import User, Group
from rest_framework import viewsets
from tutorial.quickstart.serializers import UserSerializer, GroupSerializerclass UserViewSet(viewsets.ModelViewSet):
"""
API endpoint permits customers to be considered or edited.
"""
queryset = User.objects.all().order_by('-date_joined')
serializer_class = UserSerializerclass GroupViewSet(viewsets.ModelViewSet):
"""
API endpoint permits teams to be considered or edited.
"""
queryset = Group.objects.all()
serializer_class = GroupSerializer
Generate URLs with Django
Now you’ll be able to generate URLs so folks can entry your fledgling API. Open urls.py in a textual content editor and exchange the default pattern code with this code:
from django.urls import embody, path
from rest_framework import routers
from tutorial.quickstart import viewsrouter = routers.DefaultRouter()
router.register(r'customers', views.UserViewSet)
router.register(r'teams', views.GroupViewSet)# Use automated URL routing
# Can additionally embody login URLs for the browsable API
urlpatterns = [
path('', embody(router.urls)),
path('api-auth/', embody('rest_framework.urls', namespace='rest_framework'))
]
Adjust your Django undertaking settings
The settings module for this instance undertaking is saved in tutorial/settings.py, so open that in a textual content editor and add rest_framework to the tip of the INSTALLED_APPS record:
INSTALLED_APPS = [
...
'rest_framework',
]
Test your Django API
You’re now prepared to check the API you’ve got constructed. First, begin up the built-in server from the command line:
$ python3 handle.py runserver
You can entry your API by navigating to the URL http://localhost:8000/users utilizing curl:
$ curl --get http://localhost:8000/customers/?format=json
[]
Or use Firefox or the open source web browser of your alternative:
For extra in-depth data about RESTful APIs utilizing Django and Python, see the superb Django documentation.
Why ought to I exploit Django?
The main advantages of Django:
- The dimension of the Django neighborhood is ever-growing, so you’ve numerous sources for steering, even on an advanced undertaking.
- Features like templating, routing, kinds, authentication, and administration instruments are included by default. You do not must hunt for exterior instruments or fear about third-party instruments introducing compatibility points.
- Simple constructs for customers, loops, and situations assist you to deal with writing code.
- It’s a mature and optimized framework that’s extraordinarily quick and dependable.
The main drawbacks of Django are:
- Django is advanced! From a developer’s perspective, Django could be trickier to be taught than an easier framework.
- There’s an enormous ecosystem round Django. This is nice when you’re comfy with Django, however it may be overwhelming once you’re nonetheless studying.
Django is a good choice in your utility or API. Download it, get aware of it, and begin growing a tremendous undertaking!
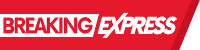