When Java SE eight (aka core Java eight) was launched in 2014, it launched adjustments that essentially impression programming in it. The adjustments have two intently linked components: the stream API and the purposeful programming constructs. This article makes use of code examples, from the fundamentals by means of superior options, to introduce every half and illustrate the interaction between them.
The fundamentals
The stream API is a concise and high-level approach to iterate over the weather in an information sequence. The packages java.util.stream and java.util.perform home the brand new libraries for the stream API and associated purposeful programming constructs. Of course, a code instance is value a thousand phrases.
The code section beneath populates a List with about 2,000 random integer values:
Another for loop might be used to iterate over the populated checklist to gather the even values into one other checklist. The stream API is a cleaner approach to do the identical:
List <Integer> evens = checklist
.stream() // streamify the checklist
.filter(n -> (n & 0x1) == zero) // filter out odd values
.accumulate(Collectors.toList()); // accumulate even values
The instance has three features from the stream API:
-
The stream perform can flip a Collection right into a stream, which is a conveyor belt of values accessible one after the other. The streamification is lazy (and subsequently environment friendly) in that the values are produced as wanted quite than all of sudden.
-
The filter perform determines which streamed values, if any, get by means of to the subsequent stage within the processing pipeline, the accumulate stage. The filter perform is higher-order in that its argument is a perform—on this instance, a lambda, which is an unnamed perform and on the heart of Java’s new purposeful programming constructs.
The lambda syntax departs radically from conventional Java:
n -> (n & 0x1) == zero
The arrow (a minus signal adopted instantly by a greater-than signal) separates the argument checklist on the left from the perform’s physique on the correct. The argument n is just not explicitly typed, though it might be; in any case, the compiler figures out that n is an Integer. If there have been a number of arguments, these can be enclosed in parentheses and separated by commas.
The physique, on this instance, checks whether or not an integer’s lowest-order (rightmost) bit is a zero, which signifies a good worth. A filter ought to return a boolean worth. There is not any express return within the perform’s physique, though there might be. If the physique has no express return, then the physique’s final expression is the returned worth. In this instance, written within the spirit of lambda programming, the physique consists of the one, easy boolean expression (n & 0x1) == zero.
-
The accumulate perform gathers the even values into a listing whose reference is evens. As an instance beneath illustrates, the accumulate perform is thread-safe and, subsequently, would work appropriately even when the filtering operation was shared amongst a number of threads.
Convenience features and straightforward multi-threading
In a manufacturing setting, an information stream may need a file or a community connection as its supply. For studying the stream API, Java supplies varieties resembling IntStream, which might generate streams with components of assorted varieties. Here is an IntStream instance:
IntStream // integer stream
.vary(1, 2048) // generate a stream of ints on this vary
.parallel() // partition the information for a number of threads
.filter(i -> ((i & 0x1) > zero)) // odd parity? cross by means of solely odds
.forEach(System.out::println); // print every
The IntStream kind features a vary perform that generates a stream of integer values inside a specified vary, on this case, from 1 by means of 2,048, with increments of 1. The parallel perform routinely partitions the work to be achieved amongst a number of threads, every of which does the filtering and printing. (The variety of threads sometimes matches the variety of CPUs on the host system.) The argument to the forEach perform is a methodology reference, on this case, a reference to the println methodology encapsulated in System.out, which is of kind PrintStream. The syntax for methodology and constructor references can be mentioned shortly.
Because of the multi-threading, the integer values are printed in an arbitrary order total however in sequence inside a given thread. For instance, if thread T1 prints 409 and 411, then T1 does so within the order 409–411, however another thread would possibly print 2,045 beforehand. The threads behind the parallel name execute concurrently, and the order of their output is subsequently indeterminate.
The map/cut back sample
The map/cut back sample has develop into common in processing giant datasets. A map/cut back macro operation is constructed from two micro-operations. The information first are scattered (mapped) amongst numerous employees, and the separate outcomes then are gathered collectively—maybe as a single worth, which might be the discount. Reduction can take completely different types, as the next examples illustrate.
Instances of the Number class beneath characterize integer values with both EVEN or ODD parity:
public class Number
enum Parity EVEN, ODD
personal int worth;
public Number(int n)
public void setValue(int worth) this.worth = worth;
public int getValue()
public Parity getParity()
public void dump()
System.out.format("Value: %2nd (parity: %s)n", getValue(),
(getParity() == Parity.ODD ? "odd" : "even"));
The following code illustrates map/cut back with a Number stream, thereby exhibiting that the stream API can deal with not solely primitive varieties resembling int and float however programmer-defined class varieties as properly.
In the code section beneath, a listing of random integer values is streamified utilizing the parallelStream quite than the stream perform. The parallelStream variant, just like the parallel perform launched earlier, does computerized multithreading.
last int howMany = 200;
Random r = new Random();
Number[ ] nums = new Number[howMany];
for (int i = zero; i < howMany; i++) nums[i] = new Number(r.subsequentInt(100));
List<Number> checklistOfNums = Arrays.asList(nums); // listify the arrayInteger sum4All = checklistOfNums
.parallelStream() // computerized multi-threading
.mapToInt(Number::getValue) // methodology reference quite than lambda
.sum(); // cut back streamed values to a single worth
System.out.println("The sum of the randomly generated values is: " + sum4All);
The higher-order mapToInt perform may take a lambda as an argument, however on this case, it takes a technique reference as a substitute, which is Number::getValue. The getValue methodology expects no arguments and returns its int worth for a given Number occasion. The syntax is uncomplicated: the category identify Number adopted by a double colon and the strategy’s identify. Recall the sooner System.out::println instance, which has the double colon after the static discipline out within the System class.
The methodology reference Number::getValue might be changed by the lambda beneath. The argument n is among the Number situations within the stream:
mapToInt(n -> n.getValue())
In basic, lambdas and methodology references are interchangeable: if a higher-order perform resembling mapToInt can take one type as an argument, then this perform may take the opposite as properly. The two purposeful programming constructs have the identical function—to carry out some custom-made operation on information handed in as arguments. Choosing between the 2 is usually a matter of comfort. For instance, a lambda will be written with out an encapsulating class, whereas a technique can not. My behavior is to make use of a lambda except the suitable encapsulated methodology is already at hand.
The sum perform on the finish of the present instance does the discount in a thread-safe method by combining the partial sums from the parallelStream threads. However, the programmer is liable for making certain that, in the middle of the multi-threading induced by the parallelStream name, the programmer’s personal perform calls (on this case, to getValue) are thread-safe.
The final level deserves emphasis. Lambda syntax encourages the writing of pure features, that are features whose return values rely solely on the arguments, if any, handed in; a pure perform has no unwanted effects resembling updating a static discipline in a category. Pure features are thereby thread-safe, and the stream API works finest if the purposeful arguments handed to higher-order features, resembling filter and map, are pure features.
For finer-grained management, there may be one other stream API perform, named cut back, that might be used for summing the values within the Number stream:
This model of the cut back perform takes two arguments, the second of which is a perform:
- The first argument (on this case, zero) is the id worth, which serves because the preliminary worth for the discount operation and because the default worth ought to the stream run dry through the discount.
- The second argument is the accumulator, on this case, a lambda with two arguments: the primary argument (sofar) is the operating sum, and the second argument (subsequent) is the subsequent worth from the stream. The operating sum and subsequent worth then are added to replace the accumulator. Keep in thoughts that each the map and the cut back features now execute in a multi-threaded context due to the parallelStream name firstly.
In the examples up to now, stream values are collected after which lowered, however, normally, the Collectors within the stream API can accumulate values with out decreasing them to a single worth. The assortment exercise can produce arbitrarily wealthy information constructions, as the subsequent code section illustrates. The instance makes use of the identical checklistOfNums because the previous examples:
The numMap within the first line refers to a Map whose key’s a Number parity (ODD or EVEN) and whose worth is a List of Number situations with values having the designated parity. Once once more, the processing is multi-threaded by means of the parallelStream name, and the accumulate name then assembles (in a thread-safe method) the partial outcomes into the one Map to which numMap refers. The get methodology then is known as twice on the numMap, as soon as to get the evens and a second time to get the odds.
The utility perform dumpList once more makes use of the higher-order forEach perform from the stream API:
Here is a slice of this system’s output from a pattern run:
The sum of the randomly generated values is: 3322
The sum once more, utilizing a distinct methodology: 3322Evens:
Value: 72 (parity: even)
Value: 54 (parity: even)
...
Value: 92 (parity: even)Odds:
Value: 35 (parity: odd)
Value: 37 (parity: odd)
...
Value: 41 (parity: odd)
Functional constructs for code simplification
Functional constructs, resembling methodology references and lambdas, match properly into the stream API. These constructs characterize a significant simplification of higher-order features in Java. Even within the dangerous previous days, Java technically supported higher-order features by means of the Method and Constructor varieties, situations of which might be handed as arguments to different features. These varieties have been used—however not often in production-grade Java exactly due to their complexity. Invoking a Method, for instance, requires both an object reference (if the strategy is non-static) or at the least a category identifier (if the strategy is static). The arguments for the invoked Method then are handed to it as Object situations, which can require express downcasting if polymorphism (one other complexity!) is just not in play. By distinction, lambdas and methodology references are simple to cross as arguments to different features.
The new purposeful constructs have makes use of past the stream API, nevertheless. Consider a Java GUI program with a button for the person to push, for instance, to get the present time. The occasion handler for the button push may be written as follows:
JButton updateCurrentTime = new JButton("Update current time");
updateCurrentTime.addActionListener(new ActionListener() );
This brief code section is a problem to elucidate. Consider the second line during which the argument to the strategy addActionListener begins as follows:
new ActionListener() {
This appears mistaken in that MotionListener is an summary interface, and summary varieties can’t be instantiated with a name to new. However, it seems that one thing else totally is being instantiated: an unnamed interior class that implements this interface. If the code above have been encapsulated in a category named PreviousJava, then this unnamed interior class can be compiled as PreviousJava$1.class. The actionPerformed methodology is overridden within the unnamed interior class.
Now take into account this refreshing change with the brand new purposeful constructs:
updateCurrentTime.addActionListener(e -> currentTime.setText(new Date().toString()));
The argument e within the lambda is an MotionEvent occasion, and the lambda’s physique is a straightforward name to setText on the button.
Functional interfaces and composition
The lambdas used up to now have been written in place. For comfort, nevertheless, there will be references to lambdas simply as there are to encapsulated strategies. The following sequence of brief examples illustrate this.
Consider this interface definition:
@FunctionalInterface // non-compulsory, often omitted
interface BinaryIntOp
The annotation @FunctionalInterface applies to any interface that declares a single summary methodology; on this case, compute. Several commonplace interfaces (e.g., the Runnable interface with its single declared methodology, run) match the invoice. In this instance, compute is the declared methodology. The interface can be utilized because the goal kind in a reference declaration:
BinaryIntOp div = (arg1, arg2) -> arg1 / arg2;
div.compute(12, three); // four
The package deal java.util.perform supplies numerous purposeful interfaces. Some examples comply with.
The code section beneath introduces the parameterized Predicate purposeful interface. In this instance, the sort Predicate<String> with parameter String can seek advice from both a lambda with a String argument or a String methodology resembling isEmpty. In basic, a predicate is a perform that returns a boolean worth.
The isEmpty predicate evaluates to true simply in case a string’s size is zero; therefore, solely the empty string makes it by means of to the forEach stage within the pipeline.
The subsequent code segments illustrate how easy lambdas or methodology references will be composed into richer ones. Consider this sequence of assignments to references of the IntUnaryOperator kind, which takes an integer argument and returns an integer worth:
IntUnaryOperator doubled = n -> n * 2;
IntUnaryOperator tripled = n -> n * three;
IntUnaryOperator squared = n -> n * n;
IntUnaryOperator is a FunctionalInterface whose single declared methodology is applyAsInt. The three references doubled, tripled, and squared now can be utilized standalone or in numerous compositions:
int arg = 5;
doubled.applyAsInt(arg); // 10
tripled.applyAsInt(arg); // 15
squared.applyAsInt(arg); // 25
Here are some pattern compositions:
int arg = 5;
doubled.compose(squared).applyAsInt(arg); // doubled-the-squared: 50
tripled.compose(doubled).applyAsInt(arg); // tripled-the-doubled: 30
doubled.andThen(squared).applyAsInt(arg); // doubled-andThen-squared: 100
squared.andThen(tripled).applyAsInt(arg); // squared-andThen-tripled: 75
Compositions might be achieved with in-place lambdas, however the references make the code cleaner.
Constructor references
Constructor references are one more of the purposeful programming constructs, however these references are helpful in additional delicate contexts than lambdas and methodology references. Once once more, a code instance appears the easiest way to make clear.
Consider this POJO class:
The class has a single constructor, which requires a String argument. Given an array of names, the purpose is to generate an array of BedRocker components, one per identify. Here is the code section that makes use of purposeful constructs to take action:
At a excessive degree, this code section transforms names into BedRocker array components. In element, the code works as follows. The Stream interface (within the package deal java.util.stream) will be parameterized, on this case, to generate a stream of BedRocker gadgets named bedrockers.
The Arrays.asList utility once more is used to streamify an array, names, with every stream merchandise then handed to the map perform whose argument now’s the constructor reference BedRocker::new. This constructor reference acts as an object manufacturing facility by producing and initializing, on every name, a BedRocker occasion. After the second line executes, the stream named bedrockers consists of 5 BedRocker gadgets.
The instance will be clarified additional by specializing in the higher-order map perform. In a typical case, a mapping transforms a price of 1 kind (e.g., an int) into a distinct worth of the identical kind (e.g., an integer’s successor):
map(n -> n + 1) // map n to its successor
In the BedRocker instance, nevertheless, the transformation is extra dramatic as a result of a price of 1 kind (a String representing a reputation) is mapped to a price of a completely different kind, on this case, a BedRocker occasion with the string as its identify. The transformation is finished by means of a constructor name, which is enabled by the constructor reference:
map(BedRocker::new) // map a String to a BedRocker
The worth handed to the constructor is among the names within the names array.
The second line of this code instance additionally illustrates the by-now-familiar transformation of an array first right into a List after which right into a Stream:
Stream<BedRocker> bedrockers = Arrays.asList(names).stream().map(BedRocker::new);
The third line goes the opposite method—the stream bedrockers is reworked into an array by invoking the toArray methodology with the array constructor reference BedRocker[]::new:
BedRocker[ ] arrayBR = bedrockers.toArray(BedRocker[]::new);
This constructor reference doesn’t create a single BedRocker occasion, however quite a whole array of those: the constructor reference is now BedRocker[]::new quite than BedRocker::new. For affirmation, the arrayBR is reworked right into a List, which once more is streamified in order that forEach can be utilized to print the BedRocker names:
Fred
Wilma
Peebles
Dino
Baby Puss
The instance’s delicate transformations of knowledge constructions are achieved with however few traces of code, underscoring the ability of assorted higher-order features that may take a lambda, a technique reference, or a constructor reference as an argument
Currying
To curry a perform is to cut back (sometimes by one) the variety of express arguments required for no matter work the perform does. (The time period honors the logician Haskell Curry.) In basic, features are simpler to name and are extra sturdy if they’ve fewer arguments. (Recall some nightmarish perform that expects a half-dozen or so arguments!) Accordingly, currying ought to be seen as an effort to simplify a perform name. The interface varieties within the java.util.perform package deal are fitted to currying, as the subsequent instance exhibits.
References of the IntBinaryOperator interface kind are for features that take two integer arguments and return an integer worth:
IntBinaryOperator mult2 = (n1, n2) -> n1 * n2;
mult2.applyAsInt(10, 20); // 200
mult2.applyAsInt(10, 30); // 300
The reference identify mult2 underscores that two express arguments are required, on this instance, 10 and 20.
The beforehand launched IntUnaryOperator is easier than an IntBinaryOperator as a result of the previous requires only one argument, whereas the latter requires two arguments. Both return an integer worth. The purpose, subsequently, is to curry the two-argument IntBinraryOperator named mult2 right into a one-argument IntUnaryOperator model curriedMult2.
Consider the sort IntFunction<R>. A perform of this kind takes an integer argument and returns a results of kind R, which might be one other perform—certainly, an IntBinaryOperator. Having a lambda return one other lambda is simple:
arg1 -> (arg2 -> arg1 * arg2) // parentheses might be omitted
The full lambda begins with arg1, and this lambda’s physique—and returned worth—is one other lambda, which begins with arg2. The returned lambda takes only one argument (arg2) however returns the product of two numbers (arg1 and arg2). The following overview, adopted by the code, ought to make clear.
Here is an outline of how mult2 will be curried:
- A lambda of kind IntFunction<IntUnaryOperator> is written and referred to as with an integer worth resembling 10. The returned IntUnaryOperator caches the worth 10 and thereby turns into the curried model of mult2, on this instance, curriedMult2.
- The curriedMult2 perform then is known as with a single express argument (e.g., 20), which is multiplied with the cached argument (on this case, 10) to supply the product returned.
Here are the small print in code:
// Create a perform that takes one argument n1 and returns a one-argument
// perform n2 -> n1 * n2 that returns an int (the product n1 * n2).
IntFunction<IntUnaryOperator> curriedMult2Maker = n1 -> (n2 -> n1 * n2);
Calling the curriedMult2Maker generates the specified IntUnaryOperator perform:
// Use the curriedMult2Maker to get a curried model of mult2.
// The argument 10 is n1 from the lambda above.
IntUnaryOperator curriedMult2 = curriedMult2Maker2.apply(10);
The worth 10 is now cached within the curriedMult2 perform in order that the express integer argument in a curriedMult2 name can be multiplied by 10:
curriedMult2.applyAsInt(20); // 200 = 10 * 20
curriedMult2.applyAsInt(80); // 800 = 10 * 80
The cached worth will be modified at will:
curriedMult2 = curriedMult2Maker.apply(50); // cache 50
curriedMult2.applyAsInt(101); // 5050 = 101 * 50
Of course, a number of curried variations of mult2, every an IntUnaryOperator, will be created on this method.
Currying takes benefit of a robust function about lambdas: a lambda is well written to return no matter kind of worth is required, together with one other lambda.
Wrapping up
Java stays a class-based object-oriented programming language. But with the stream API and its supporting purposeful constructs, Java takes a decisive (and welcomed) step towards purposeful languages resembling Lisp. The result’s a Java higher suited to course of the large information streams so frequent in trendy programming. This step within the purposeful route additionally makes it simpler to put in writing clear, concise Java within the pipeline type highlighted in earlier code examples:
informationStream
.parallelStream() // multi-threaded for effectivity
.filter(...) // stage 1
.map(...) // stage 2
.filter(...) // stage three
...
.accumulate(...); // or, maybe, cut back: stage N
The computerized multi-threading, illustrated with the parallel and parallelStream calls, is constructed upon Java’s fork/be part of framework, which helps job stealing for effectivity. Suppose that the thread pool behind a parallelStream name consists of eight threads and that the informationStream is partitioned eight methods. Some thread (e.g., T1) would possibly work quicker than one other (e.g., T7), which implies that a few of T7’s duties must be moved into T1’s work queue. This occurs routinely at runtime.
The programmer’s chief accountability on this simple multi-threading world is to put in writing thread-safe features handed as arguments to the higher-order features that dominate within the stream API. Lambdas, specifically, encourage the writing of pure—and, subsequently, thread-safe—features.
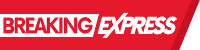