A system admin typically writes Bash scripts, some quick and a few fairly prolonged, to perform varied duties.
Have you ever checked out an set up script supplied by a software program vendor? They typically add lots of capabilities and logic to be able to make sure that the set up works correctly and doesn’t lead to harm to the client’s system. Over the years, I’ve amassed a set of assorted strategies for enhancing my Bash scripts, and I’d wish to share a few of them in hopes they may help others. Here is a set of small scripts created for example these easy examples.
Starting out
When I used to be beginning out, my Bash scripts have been nothing greater than a collection of instructions, normally meant to avoid wasting time with commonplace shell operations like deploying net content material. One such activity was extracting static content material into the house listing of an Apache net server. My script went one thing like this:
cp january_schedule.tar.gz /usr/apache/residence/calendar/
cd /usr/apache/residence/calendar/
tar zvxf january_schedule.tar.gz
While this saved me a while and typing, it definitely was not a really attention-grabbing or helpful script in the long run. Over time, I realized different methods to make use of Bash scripts to perform more difficult duties, akin to creating software program packages, putting in software program, or backing up a file server.
1. The conditional assertion
Just as with so many different programming languages, the conditional has been a robust and customary characteristic. A conditional is what permits logic to be carried out by a pc program. Most of my examples are primarily based on conditional logic.
The primary conditional makes use of an “if” assertion. This permits us to check for some situation that we will then use to govern how a script performs. For occasion, we will test for the existence of a Java bin listing, which might point out that Java is put in. If discovered, the executable path might be up to date with the placement to allow calls by Java purposes.
if [ -d "$JAVA_HOME/bin" ] ; then
PATH="$JAVA_HOME/bin:$PATH"
2. Limit execution
You may need to restrict a script to solely be run by a selected consumer. Although Linux has commonplace permissions for customers and teams, in addition to SELinux for enabling any such safety, you possibly can select to put logic inside a script. Perhaps you need to make sure that solely the proprietor of a selected net software can run its startup script. You might even use code to restrict a script to the basis consumer. Linux has a few surroundings variables that we will check on this logic. One is $USER, which gives the username. Another is $UID, which gives the consumer’s identification quantity (UID) and, within the case of a script, the UID of the executing consumer.
User
The first instance reveals how I might restrict a script to the consumer jboss1 in a multi-hosting surroundings with a number of software server cases. The conditional “if” assertion primarily asks, “Is the executing user not jboss1?” When the situation is discovered to be true, the primary echo assertion is named, adopted by the exit 1, which terminates the script.
if [ "$USER" != 'jboss1' ]; then
echo "Sorry, this script must be run as JBOSS1!"
exit 1
fi
echo "continue script"
Root
This subsequent instance script ensures that solely the basis consumer can execute it. Because the UID for root is zero, we will use the -gt choice within the conditional if assertion to ban all UIDs larger than zero.
if [ "$UID" -gt zero ]; then
echo "Sorry, this script must be run as ROOT!"
exit 1
fi
echo "continue script"
three. Use arguments
Just like every executable program, Bash scripts can take arguments as enter. Below are a number of examples. But first, it’s best to perceive that good programming signifies that we don’t simply write purposes that do what we wish; we should write purposes that can’t do what we don’t need. I like to make sure that a script doesn’t do something damaging within the case the place there is no such thing as a argument. Therefore, that is the primary test that y. The situation checks the variety of arguments, $#, for a price of zero and terminates the script if true.
if [ $# -eq zero ]; then
echo "No arguments provided"
exit 1
fi
echo "arguments found: $#"
Multiple arguments
You can go a couple of argument to a script. The inside variables that the script makes use of to reference every argument are merely incremented, akin to $1, $2, $three, and so forth. I’ll simply broaden my instance above with the next line to echo the primary three arguments. Obviously, further logic will probably be wanted for correct argument dealing with primarily based on the full quantity. This instance is straightforward for the sake of demonstration.
echo $1 $2 $three
While we’re discussing these argument variables, you might need questioned, “Did he skip zero?”
Well, sure, I did, however I’ve an incredible purpose! There is certainly a $zero variable, and it is extremely helpful. Its worth is solely the title of the script being executed.
echo $zero
An necessary purpose to reference the title of the script throughout execution is to generate a log file that features the script’s title in its personal title. The easiest type may simply be an echo assertion.
echo check >> $zero.log
However, you’ll most likely need to add a bit extra code to make sure that the log is written to a location with the title and knowledge that you simply discover useful to your use case.
four. User enter
Another helpful characteristic to make use of in a script is its skill to just accept enter throughout execution. The easiest is to supply the consumer some enter.
echo "enter a word please:"
learn phrase
echo $phrase
This additionally means that you can present selections to the consumer.
learn -p "Install Software ?? [Y/n]: " answ
if [ "$answ" == 'n' ]; then
exit 1
fi
echo "Installation starting..."
5. Exit on failure
Some years in the past, I wrote a script for putting in the most recent model of the Java Development Kit (JDK) on my pc. The script extracts the JDK archive to a selected listing, updates a symbolic hyperlink, and makes use of the options utility to make the system conscious of the brand new model. If the extraction of the JDK archive failed, persevering with might break Java system-wide. So, I needed the script to abort in such a state of affairs. I don’t need the script to make the subsequent set of system modifications until the archive was efficiently extracted. The following is an excerpt from that script:
tar kxzmf jdk-8u221-linux-x64.tar.gz -C /jdk --checkpoint=.500; ec=$?
if [ $ec -ne zero ]; then
echo "Installation failed - exiting."
exit 1
fi
A fast approach so that you can display the utilization of the $? variable is with this quick one-liner:
ls T; ec=$?; echo $ec
First, run contact T adopted by this command. The worth of ec will probably be zero. Then, delete T, rm T, and repeat the command. The worth of ec will now be 2 as a result of ls stories an error situation since T was not discovered.
You can reap the benefits of this error reporting to incorporate logic, as I’ve above, to manage the conduct of your scripts.
Takeaway
We may assume that we have to make use of languages, akin to Python, C, or Java, for larger performance, however that’s not essentially true. The Bash scripting language could be very highly effective. There is lots to be taught to maximise its usefulness. I hope these few examples will shed some mild on the potential of coding with Bash.
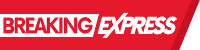