The Twisted Requests (treq) bundle is an HTTP consumer constructed on the favored Twisted library that’s used for asynchronous requests. Async libraries supply the power to do giant quantities of community requests in parallel with comparatively little CPU influence. This might be helpful in HTTP purchasers that must make a number of requests earlier than they’ve all the data they want. In this text, we’ll work by an instance of creating async calls to discover utilizing treq.
Defining an issue to resolve
I take pleasure in enjoying the real-time technique sport Clash Royale. While it isn’t open supply, it does have a public API that we will use to indicate how async requests can turn out to be useful.
Clash Royale is a cellular technique player-vs-player sport the place gamers play playing cards in an enviornment to win. Each card has completely different strengths and weaknesses, and completely different gamers desire completely different playing cards. Clash Royale remembers which card a participant performs essentially the most; that is their “favorite” card. Players come collectively in clans the place they will help one another. Supercell, Clash Royale’s developer, launched an HTTP-based API the place completely different statistics might be queried.
Here’s a query best-answered asynchronously: How can we write a program that may output the most well-liked favourite playing cards in a clan in order that we will begin to perceive our opponents (and see which playing cards are fashionable with our clan members)?
You can register an account to observe together with the tutorial, however you will nonetheless have the ability to perceive what we’re constructing when you do not. If you do wish to register an account, create an API token through the Clash Royale developer portal. Then select “Create New Key” below your profile, and enter a reputation, description, and a sound IP tackle. (An actual tackle is required, so I used this site to search out mine.) Since you must by no means save an API key in your code, preserve it as a separate file in ~/.crtoken:
$ ls ~/.crtoken
/residence/moshez/.crtoken
Twisted applications
Running a program based mostly on Twisted requires a variety of extra packages to make the expertise as easy as potential. I can’t cowl all of them on this tutorial, however every one is price exploring to be taught extra.
To make it simpler to see what’s going on, let’s begin with this introductory program that prints Hello world, after which we’ll speak by what it does:
import collections, json, os, sys, urllib.parse
from twisted.web import process, defer
import treqwith open(os.path.expanduser("~/.crtoken")) as fpin:
token = fpin.learn().strip()def fundamental(reactor):
print("Hello world")
return defer.succeed(None)process.react(fundamental, sys.argv[1:])
This imports many extra modules than we’d like for the “Hello world” instance. We will want these modules for the ultimate model of this system, which can accomplish the extra complicated process of asynchronously querying an API. After the import, this system reads the token from the file and shops it within the variable token. (We are usually not going to do something with the token proper now, however it’s good to see that syntax.) Next there is a fundamental perform that accepts a Twisted reactor. A reactor is form of like an interface to the complicated equipment of the Twisted bundle. In this case, the perform fundamental is distributed as a parameter, and it is fed an extra argument.
The fundamental returns a defer.succeed(None). This is the way it returns a price of the best kind: a deferred worth, however one which already has been “fired” or “called.” Because of that, this system will exit instantly after printing Hello world, as we’d like.
Next, we’ll take a look at the ideas of async features and guaranteeDeferred:
async def get_clan_details(clan):
print("Hello world", clan)def fundamental(reactor, clan):
return defer.guaranteeDeferred(get_clan_details(clan))process.react(fundamental, sys.argv[1:])
In this program, which ought to begin with the identical imports, we moved all of the logic to the async perform get_clan_details. Just like an everyday perform, an async perform has an implicit return None on the finish. However, async features, generally referred to as co-routines, are a completely different kind than Deferred. In order to let Twisted, which has existed since Python 1.5.2, use this contemporary function, we should adapt the co-routine utilizing guaranteeDeferred.
While we may write all of the logic with out utilizing co-routines, utilizing the async syntax will permit us to jot down code that’s simpler to know, and we might want to transfer quite a bit much less of the code into embedded callbacks.
The subsequent idea to introduce is that of await. Later, we’ll await a community name, however for simplicity, proper now, we’ll await on a timer. Twisted has a particular perform, process.deferLater, which can name a perform with given parameters after a while has handed.
The following program will take 5 seconds to finish:
async def get_clan_details(clan, reactor):
out = await process.deferLater(
reactor,
5,
lambda clan: f"Hello world ",
clan
)
print(out)def fundamental(reactor, clan):
return defer.guaranteeDeferred(get_clan_details(clan, reactor))process.react(fundamental, sys.argv[1:])
A notice about sorts: process.deferLater returns a Deferred, as do most Twisted features that don’t have the worth already accessible. When operating the Twisted occasion loop, we will await on each Deferred values in addition to co-routines.
The perform process.deferLater will wait 5 seconds after which name our lambda, calculating the string to print out.
Now we’ve got all of the Twisted constructing blocks wanted to jot down an environment friendly clan-analysis program!
Async calls with treq
Since we will likely be utilizing the worldwide reactor, we now not want to simply accept the reactor as a parameter within the perform that calculates these statistics:
async def get_clan_details(clan):
The approach to make use of the token is as a “bearer” token within the headers:
headers = dict(headers=)
We need clan tags to be despatched, which will likely be strings. Clan tags start with #, in order that they have to be quoted earlier than they’re put in URLs. This is as a result of # has the particular that means “URL fragment”:
clan = urllib.parse.quote(clan)
The first step is to get the small print of the clan, together with the clan members:
res = await treq.get("https://api.clashroyale.com/v1/clans/" + clan,
headers=headers)
Notice that we’ve got to await the treq.get calls. We must be express about when to attend and get data since it’s an asynchronous community name. Just utilizing the await syntax to name a Deferred perform does not allow us to take full energy of asynchronicity (we’ll see the way to do it later).
Next, after getting the headers, we have to get the content material. The treq library provides us a helper technique that parses the JSON immediately:
content material = await res.json()
The content material consists of some metadata in regards to the clan, which isn’t fascinating for our present functions, and a memberList area that comprises the clan members. Note that whereas it has some knowledge in regards to the gamers, the present favourite card just isn’t a part of it. It does embody the distinctive “player tag” that we will use to retrieve additional knowledge.
We acquire all participant tags, and, since additionally they start with #, we URL-quote them:
player_tags = [urllib.parse.quote(participant['tag'])
for participant in content material['memberList']]
Finally, we come to the true energy of treq and Twisted: producing all requests for participant knowledge directly! That can actually velocity up duties like this one, which queries an API again and again. In circumstances of APIs with rate-limiting, this may be problematic.
There are instances once we must be thoughtful to our API house owners and never run up towards any price limits. There are strategies to assist rate-limiting explicitly in Twisted, however they’re past the scope of this tutorial. (One vital software is defer.DeferredSemaphore.)
requests = [treq.get("https://api.clashroyale.com/v1/players/" + tag,
headers=headers)
for tag in player_tags]
An apart: await, Deferred, and callbacks
For these curious in regards to the specifics of the returned object, here is a better take a look at what’s taking place.
Remember that requests don’t return the JSON physique immediately. Earlier, we used await in order that we didn’t have to fret about precisely what the requests return. They really return a Deferred. A Deferred can have an hooked up callback that may modify the Deferred. If the callback returns a Deferred, the ultimate worth of the Deferred would be the worth of the returned Deferred.
So, to every deferred, we connect a callback that may retrieve the JSON of the physique:
for request in requests:
request.addCallback(lambda outcome: outcome.json())
Attaching callbacks to Deferreds is a extra handbook method, which makes code that’s tougher to observe however makes use of the async options extra effectively. Specifically, as a result of we’re attaching all of the callbacks on the identical time, we don’t want to attend for the community calls, which doubtlessly can take a very long time, to point the way to post-process the outcome.
From Deferreds to values
We can’t calculate the most well-liked favourite playing cards till all outcomes have been gathered. We have a listing of Deferreds, however what we wish is a Deferred that will get a listing worth. This inversion is strictly what the Twisted perform defer.gatherResults does:
all_players = await defer.gatherResults(requests)
This seemingly harmless name is the place we use the complete energy of Twisted. The defer.gatherResults perform instantly returns a deferred that may fireplace solely when all of the constituent Deferreds have fired and can fireplace with the outcome. It even provides us free error-handling: if any of the Deferreds error out, it can instantly return a failed deferred, which can trigger the await to lift an exception.
Now that we’ve got all of the gamers’ particulars, we have to munch some knowledge. We get to make use of certainly one of Python’s coolest built-ins, collections.Counter. This class takes a listing of issues and counts what number of instances it has seen every factor, which is strictly what we’d like for vote counting or reputation contests:
favorite_card = collections.Counter([participant["currentFavouriteCard"]["name"]
for participant in all_players])
Finally, we print it:
print(json.dumps(favorite_card.most_common(), indent=four))
Putting all of it collectively
So, placing all of it collectively, we’ve got:
import collections, json, os, sys, urllib.parse
from twisted.web import process, defer
import treqwith open(os.path.expanduser("~/.crtoken")) as fpin:
token = fpin.learn().strip()async def get_clan_details(clan):
headers = headers=
clan = urllib.parse.quote(clan)
res = await treq.get("https://api.clashroyale.com/v1/clans/" + clan,
headers=headers)
content material = await res.json()
player_tags = [urllib.parse.quote(participant['tag'])
for participant in content material['memberList']]
requests = [treq.get("https://api.clashroyale.com/v1/players/" + tag,
headers=headers)
for tag in player_tags]
for request in requests:
request.addCallback(lambda outcome: outcome.json())
all_players = await defer.gatherResults(requests)
favorite_card = collections.Counter([participant["currentFavouriteCard"]["name"]
for participant in all_players])
print(json.dumps(favorite_card.most_common(), indent=four))def fundamental(reactor, clan):
return defer.guaranteeDeferred(get_clan_details(clan))process.react(fundamental, sys.argv[1:])
Thanks to the effectivity and expressive syntax of Twisted and treq, that is all of the code we have to make asynchronous calls to an API. And when you have been questioning in regards to the end result, my clan’s listing of favourite playing cards is Wizard, Mega Knight, Valkyrie, and Royal Giant, in descending order.
I hope you take pleasure in utilizing Twisted to jot down quicker API calls!
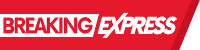