A construct system is comprised of the instruments and processes used to transition from supply code to a operating software. This transition additionally includes altering the code’s viewers from the software program developer to the tip person, whether or not the tip person is a colleague in operations or a deployment system.
After creating a couple of construct techniques utilizing containers, I feel I’ve an honest, repeatable strategy that is value sharing. These construct techniques have been used for producing loadable software program photographs for embedded hardware and compiling machine studying algorithms, however the strategy is summary sufficient for use in any container-based construct system.
This strategy is about creating or organizing the construct system in a means that makes it straightforward to make use of and keep. It’s not concerning the tips wanted to cope with containerizing any explicit software program compilers or instruments. It applies to the frequent use case of software program builders constructing software program handy off a maintainable picture to different technical customers (whether or not they’re sysadmins, DevOps engineers, or another title). The construct system is abstracted away from the tip customers in order that they’ll concentrate on the software program.
Why containerize a construct system?
Creating a repeatable, container-based construct system can present an a variety of benefits to a software program group:
- Focus: I wish to concentrate on writing my software. When I name a software to “build,” I need the toolset to ship a ready-to-use binary. I do not wish to spend time troubleshooting the construct system. In reality, I might reasonably not know or care concerning the construct system.
- Identical construct habits: Whatever the use case, I wish to be certain that the complete group makes use of the identical variations of the toolset and will get the identical outcomes when constructing. Otherwise, I’m always coping with the case of “it works on my PC but not yours.” Using the identical toolset model and getting equivalent output for a given enter supply file set is crucial in a group venture.
- Easy setup and future migration: Even if an in depth set of directions is given to everybody to put in a toolset for a venture, chances are high somebody will get it fallacious. Or there could possibly be points because of how every individual has custom-made their Linux surroundings. This could be additional compounded by way of totally different Linux distributions throughout the group (or different working techniques). The points can get uglier rapidly when it comes time for transferring to the subsequent model of the toolset. Using containers and the rules on this article will make migration to newer variations a lot simpler.
Containerizing the construct techniques that I take advantage of on my initiatives has actually been helpful in my expertise, because it has alleviated the issues above. I have a tendency to make use of Docker for my container tooling, however there can nonetheless be points as a result of set up and community configuration being distinctive surroundings to surroundings, particularly when you work in a company surroundings involving some complicated proxy settings. But at the very least now I’ve fewer construct system issues to cope with.
Walking by a containerized construct system
I created a tutorial repository you may clone and study at a later time or comply with alongside by this text. I will be strolling by all of the information within the repository. The construct system is intentionally trivial (it runs gcc) to maintain the concentrate on the construct system structure.
Build system necessities
Two key facets that I feel are fascinating in a construct system are:
Build system structure
To adjust to the fundamental necessities above, right here is how I architect the construct system:
At the underside, the workdir represents any software program supply code that must be constructed by the software program developer finish customers. Typically, this workdir shall be a source-code repository. The finish customers can manipulate this supply code repository in any means they need earlier than invoking a construct. For instance, in the event that they’re utilizing git for model management, they may git checkout the function department they’re engaged on and add or modify information. This retains the construct system unbiased of the workdir.
The three blocks on the prime collectively symbolize the containerized construct system. The left-most (yellow) block on the prime represents the scripts (construct.sh and shell.sh) that the tip person will use to work together with the construct system.
In the center (the crimson block) is the Dockerfile and the related script build_docker_image.sh. The improvement operations folks (me, on this case) will sometimes execute this script and generate the container picture. (In reality, I am going to execute this many, many instances till I get the whole lot working proper, however that is one other story.) And then I’d distribute the picture to the tip customers, resembling by a container trusted registry. The finish customers will want this picture. In addition, they’ll clone the construct system repository (i.e., one that’s equal to the tutorial repository).
The run_build.sh script on the appropriate is executed contained in the container when the tip person invokes both construct.sh or shell.sh. I am going to clarify these scripts intimately subsequent. The key right here is that the tip person doesn’t must know something concerning the crimson or blue blocks or how a container works so as to use any of this.
Build system particulars
The tutorial repository’s file construction maps to this structure. I’ve used this prototype construction for comparatively complicated construct techniques, so its simplicity isn’t a limitation in any means. Below, I’ve listed the tree construction of the related information from the repository. The dockerize-tutorial folder could possibly be changed with some other identify equivalent to a construct system. From inside this folder, I invoke both construct.sh or shell.sh with the one argument that’s the path to the workdir.
dockerize-tutorial/
├── construct.sh
├── shell.sh
└── swbuilder
├── build_docker_image.sh
├── install_swbuilder.dockerfile
└── scripts
└── run_build.sh
Note that I’ve intentionally excluded the example_workdir above, which you will discover within the tutorial repository. Actual supply code would sometimes reside in a separate repository and never be a part of the construct software repository; I included it on this repository, so I did not must cope with two repositories within the tutorial.
Doing the tutorial isn’t needed when you’re solely within the ideas, as I am going to clarify all of the information. But if you wish to comply with alongside (and have Docker put in), first construct the container picture swbuilder:v1 with:
cd dockerize-tutorial/swbuilder/
./build_docker_image.sh
docker picture ls # ensuing picture shall be swbuilder:v1
Then invoke construct.sh as:
cd dockerize-tutorial
./construct.sh ~/repos/dockerize-tutorial/example_workdir
The code for build.sh is under. This script instantiates a container from the container picture swbuilder:v1. It performs two quantity mappings: one from the example_workdir folder to a quantity contained in the container at path /workdir, and the second from dockerize-tutorial/swbuilder/scripts outdoors the container to /scripts contained in the container.
docker container run
--volume $(pwd)/swbuilder/scripts:/scripts
--volume $1:/workdir
--user $(id -u $):$(id -g $)
--rm -it --name build_swbuilder swbuilder:v1
construct
In addition, the construct.sh additionally invokes the container to run together with your username (and group, which the tutorial assumes to be the identical) in order that you’ll not have points with file permissions when accessing the generated construct output.
Note that shell.sh is equivalent besides for 2 issues: construct.sh creates a container named build_swbuilder whereas shell.sh creates one named shell_swbuilder. This is in order that there are not any conflicts if both script is invoked whereas the opposite one is operating.
The different key distinction between the 2 scripts is the final argument: construct.sh passes within the argument construct whereas shell.sh passes within the argument shell. If you have a look at the Dockerfile that’s used to create the container picture, the final line incorporates the next ENTRYPOINT. This implies that the docker container run invocation above will end in executing the run_build.sh script with both construct or shell as the only enter argument.
# run bash script and course of the enter command
ENTRYPOINT [ "/bin/bash", "/scripts/run_build.sh"]
run_build.sh makes use of this enter argument to both begin the Bash shell or invoke gcc to carry out the construct of the trivial helloworld.c venture. An actual construct system would sometimes invoke a Makefile and never run gcc instantly.
cd /workdirif [ $1 = "shell" ]; then
echo "Starting Bash Shell"
/bin/bash
elif [ $1 = "build" ]; then
echo "Performing SW Build"
gcc helloworld.c -o helloworld -Wall
fi
You might actually move multiple argument in case your use case calls for it. For the construct techniques I’ve handled, the construct is often for a given venture with a selected make invocation. In the case of a construct system the place the construct invocation is complicated, you may have run_build.sh name a selected script inside workdir that the tip person has to put in writing.
A word concerning the scripts folder
You could also be questioning why the scripts folder is positioned deep within the tree construction reasonably than on the prime degree of the repository. Either strategy would work, however I did not wish to encourage the tip person to poke round and alter issues there. Placing it deeper is a approach to make it tougher to poke round. Also, I might have added a .dockerignore file to disregard the scripts folder, because it would not have to be a part of the container context. But because it’s tiny, I did not trouble.
Simple but versatile
While the strategy is straightforward, I’ve used it for a couple of reasonably totally different construct techniques and located it to be fairly versatile. The facets which might be going to be comparatively secure (e.g., a given toolset that modifications only some instances a 12 months) are mounted contained in the container picture. The facets which might be extra fluid are saved outdoors the container picture as scripts. This permits me to simply modify how the toolset is invoked by updating the script and pushing the modifications to the construct system repository. All the person must do is to drag the modifications to their native construct system repository, which is usually fairly quick (not like updating a Docker picture). The construction lends itself to having as many volumes and scripts as are wanted whereas abstracting the complexity away from the tip person.
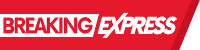