Python follows the EAFP (Easier to Ask Forgiveness than Permission) quite than the LBYL (Look Before You Leap) philosophy. The Python philosophy of EAFP is considerably linked to its “duck typing” type of coding.
When a programmer creates information in code, whether or not it is a fixed or a variable, some programming languages must know what “type” of information it’s. For instance, in case you set a variable to 13, a pc does not know whether or not you imply for it for use as a phrase (“thirteen”) or as an integer (as in 13+12=25 or 13-1=12). This is why many languages require programmers to declare information earlier than it is used.
For instance, on this C++ code, the mynumber variable is an integer sort and the myword variable is a string:
#embrace <iostream>
#embrace <string>utilizing namespace std;
int mynumber = 13;
string myword = "13";int essential()
std::cout << 13+2 << endl;
std::cout << "My favourite number is " + myword << endl;
Python is intelligent, although, and it makes use of the “duck test”: if a variable walks like a duck and talks like a duck, then it is a duck. In different phrases, Applied to pc science, meaning Python examines information to find out its sort. Python is aware of that integers are used for math and phrases are utilized in communication, so the programmer does not have to clarify to Python how you can use the info it finds in variables. Python makes use of duck typing to determine it out by itself, and doesn’t try to do math on strings or print the contents of arrays (with out iteration), and so forth.
However, earlier than we talk about these ideas, let’s go over the fundamentals:
An analogy to know the idea of “type”
The idea of “typing” within the context of a programming language is usually mentioned, however typically, the deeper which means eludes us. So, let me attempt to clarify the idea utilizing an analogy.
In a pc program, objects and different objects are saved in reminiscence, and they’re typically referred to by some “variable name.” So, once you create an object of a specific class (in any of the favored programming languages), you’re mainly reserving a portion of reminiscence for that object to occupy, and then you definately discuss with this object with that variable title.
So, as an analogy, you may consider this area in reminiscence as a sort of container or field. For this train, let’s name it a field. So now we’ve got two issues with us—an object and a field which comprises it.
To take the argument additional, sometimes, a field have to be “designed” to have the ability to maintain the thing that it comprises (i.e., a field meant for holding matches will not be preferrred for holding footwear, or vice versa, despite the fact that it is bodily doable). So, we will agree that the thing and the field which comprises it should each be of an analogous sort?
This, actually, is the so-called “static typing.” Basically, it signifies that not solely the thing should have a “type,” however the variable title (a.okay.a. the field) should have one as nicely, and it needs to be the identical or related. (I’ll clarify why I say “similar” in a second). This is why, in statically typed languages like Java/ C++, it’s essential to outline the kind of the variable once you create it. In reality, you may create a variable title analogous to a field, even with out creating any object to place in it. You cannot do that in Python.
However, a dynamically typed language like Python works in a different way. Here you may consider the variable title, not like a field however quite analogous to a “tag” (considerably like a price ticket in a retailer). So, the tag doesn’t have a kind. Rather, in case you ask the tag what its sort is, it might most likely choose the thing it’s tagged to at that second. Why I say “at that moment” is as a result of, identical to in the true world, a tag hooked up to a shoe is also hooked up to another merchandise at a unique time. So the Python interpreter doesn’t assign any sort to a variable title, per se. But in case you ask a variable title its sort, then it will provide you with the kind of the thing it’s at present tied to. This is dynamic typing.
This dynamic vs. static typing has a direct impression on the way in which you write code. Just like in the true world the place you may’t put footwear in a field meant for matches, so it additionally goes in statically typed languages—you typically can not put objects of 1 sort in a variable title created for objects of one other sort.
Strongly typed vs. weakly typed languages
There is one other essential idea to deal with right here, specifically, strongly and weakly typed languages. The “strength” of typing has just about nothing to do with whether or not it’s dynamically or statically typed. It has extra to do with “casting” or the power to transform one sort of object to a different. Contrary to well-liked notion, Python is a quite strongly typed language, identical to C++ or Java. So in Python, for instance, you may’t add, say, an “integer” to a “string,” nonetheless, you are able to do this in a language like JavaScript. JavaScript, actually, is among the notoriously “weakly typed” languages. So, it needs to be clear that sturdy/weak typing is an altogether totally different scale than static/dynamic typing. In basic, scripted languages like Python are usually dynamically typed, whereas compiled languages are usually statically typed.
Duck typing and EAFP and LBYL
Python follows the duck typing type of coding.
Let’s once more take a real-world instance. Suppose you’ve got an object “Machine M.” Now, you do not know whether or not this Machine M has the aptitude to fly or not. The manner LBYL would proceed vs. EAFP is illustrated within the determine under:
Let’s make clear the idea with some Python code (with fictitious capabilities):
# LBYL:- Look Before You Leap
if can_fly():
fly()
else:
do_something_else()
# EAFP:- Easier to Ask Forgiveness than permission
attempt:
fly()
besides:
clean_up()
How duck typing helps EAFP
Duck typing is good for the EAFP type of coding. This is as a result of we do not care in regards to the “type” of an object; we care solely about its “behavior” and “capability.” By “behavior,” I mainly imply its attributes, and by “capability,” I imply its strategies.
To sum up:
If you see loads of if-else
blocks, then you’re an LBYL coder.
But in case you see loads of try-except
blocks, you’re most likely an EAFP coder.
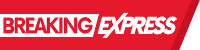