In the primary two articles of this sequence on Mycroft, an open supply, privacy-focused digital voice assistant, I lined the background behind voice assistants and a few of Mycroft’s core tenets. In Part three, I began outlining the Python code required to offer some fundamental performance to a talent that provides objects to OurGroceries, a grocery record app. And in Part four, I talked concerning the several types of intent parsers (and when to make use of every) and expanded the Python code so Mycroft may present audible suggestions whereas working by means of the talent.
In this fifth article, I’ll stroll by means of the remaining sections required to construct this talent. I will speak about undertaking dependencies, logging output for debugging functions, working with the Mycroft internet UI for setting values (akin to usernames and passwords), and tips on how to get this data into your Python code.
Dealing with undertaking dependencies
There are usually three sources for undertaking dependencies when writing a Mycroft talent:
- Python packages from PyPI
- System-level packages pulled from a repository
- Other Mycroft expertise
There are a few methods to cope with dependencies in Mycroft. You can use “requirements” recordsdata, or you need to use the manifest.yml
file.
Since many of the expertise within the Mycroft retailer use requirement recordsdata, I’ll merely contact on the manifest.yml
file. The manifest.yml
file is fairly simple. There is a dependencies:
part, and underneath this are three choices: python:
, system:
, and talent:
. Under every heading, it’s best to specify the names of required dependencies. An instance file may appear to be this:
dependencies:
# Pip dependencies on PyPI
python:
- requests
- gensim
system:
# For easy packages, that is all that's vital
all: pianobar piano-dev# Require the set up of different expertise earlier than putting in this talent
talent:
- my-other-skill
However, for the reason that majority of expertise use requirement recordsdata, I will use that choice for this undertaking, so you need to use it for example for different expertise you could want to use or create.
In Python, the necessities.txt
file, which lists all of the Python dependencies a undertaking requires, is quite common. This file is fairly easy; it could possibly both be an inventory of packages or an inventory with particular variations. I’ll specify a minimal model with some code I submitted to the ourgroceries
undertaking. There are three choices for this undertaking’s necessities.txt
:
ourgroceries==1.three.5
: Specifies that the bundle have to be model 1.three.5ourgroceries>=1.three.5
: Specifies that the bundle have to be model 1.three.5 or increasedourgroceries
: Allows any model of the bundle
My necessities.txt
makes use of ourgroceries>=1.three.5
to permit for future updates. Following this similar logic, your necessities.txt
may record totally different packages as a substitute of specifying a single bundle.
The entirety of my necessities.txt
file is one line:
ourgroceries>=1.three.5
You can even choose to make use of necessities.sh
. This is a shell script that can be utilized to put in packages, obtain modules from Git, or do any variety of issues. This file executes whereas putting in a brand new talent. The Zork skill has an instance of a necessities.sh
script. However, whereas you need to use this, if you wish to submit your talent to the shop, the necessities.sh
will likely be scrutinized pretty closely to mitigate safety points.
Debug your talent
There are a few methods to debug your talent. You can use the Mycroft logger, or you need to use commonplace Python debugging instruments. Both strategies can be found within the Mycroft command-line interface (CLI), which may be very useful for debugging.
Use Mycroft logger
To get began with the Mycroft logger, you simply must have the MycroftSkill
imported as a result of logger is a part of the bottom class. This signifies that so long as you might be working inside the category to your talent, logger is on the market. For instance, the next code demonstrates tips on how to create a really fundamental talent with a log entry:
from mycroft import MycroftSkillclass MyPretendSkill(MycroftSkill):
def __init__(self):
self.log.information("Skill starting up")def create_skill():
return MyPretendSkill()
Logger has all of the log ranges you may anticipate:
- debug: Provides the very best stage of element however is not logged by default
- information: Provides basic data when a talent is working as anticipated; it’s at all times logged
- warning: Indicates one thing is improper, however it’s not deadly
- error: Fatal issues; they’re displayed in purple within the CLI
- exception: Similar to errors besides they embrace stack traces
Along with exhibiting within the CLI, logger writes to expertise.log
. The file’s location varies relying on the way you put in Mycroft. Common areas are /var/log/mycroft/expertise.log
, ~/snap/mycroft/frequent/logs/expertise.log
, and /var/choose/mycroft/expertise.log
.
There could also be occasions once you wish to use the Mycroft logger outdoors the instantiated class. For instance, when you have some international features outlined outdoors the category, you may import LOG
particularly:
from mycroft import MycroftSkill
from mycroft.util import LOGdef my_global_funct():
LOG.information("This is being logged outside the class")class MyPretendSkill(MycroftSkill):
def __init__(self):
self.log.information("Skill starting up")def create_skill():
return MyPretendSkill()
Use Python’s debugging instruments
If you need one thing that stands out extra, you need to use the built-in Python print()
statements to debug. I’ve discovered that there are events the place the Mycroft logger is gradual to supply output. Other occasions, I simply need one thing that jumps out at me visually. In both case, I choose utilizing print()
statements when I’m debugging outdoors an IDE.
Take the next code, for instance:
if category_name is None:
self.log.information("---------------> Adding %s to %s" % (item_to_add, list_name))
print("-------------> Adding %s to %s" % (item_to_add, list_name))
This produces the next output within the mycroft-cli-client
:
~~~~ings:104 | Skill settings efficiently saved to /choose/mycroft/expertise/fallback-wolfram-alpha.mycroftai/settings.json
~~~~1 | mycroft.expertise.mycroft_skill.mycroft_skill:handle_settings_change:272 | Updating settings for talent AlarmSkill
~~~~save_settings:104 | Skill settings efficiently saved to /choose/mycroft/expertise/mycroft-alarm.mycroftai/settings.json
10:50:38.528 | INFO | 51831 | ConfigurationSkill | Remote configuration up to date
10:50:43.862 | INFO | 51831 | OurGroceriesSkill | ---------------> Adding scorching canines to my procuring
---------------> Adding scorching canines to my procuring
~~~~7.654 | INFO | 51831 | mycroft.expertise.skill_loader:reload:108 | ATTEMPTING TO RELOAD SKILL: ourgroceries-skill
~~~~831 | mycroft.expertise.skill_loader:_execute_instance_shutdown:146 | Skill ourgroceries-skill shut down efficiently
I discover that, because the textual content scrolls, it’s a lot simpler to visually establish a print assertion that doesn’t have the uniform header of the opposite messages. This is a private choice and never meant as any kind of advice for programming greatest practices.
Get enter from customers
Now that you know the way to see output out of your talent, it is time to get some environment-specific data out of your customers. In many instances, your talent will want some person data to perform correctly. Most of the time, this can be a username and password. Often, this data is required for the talent to initialize correctly.
Get person enter with internet-connected Mycroft
If your Mycroft system has a connection to the web, you need to use Mycroft’s internet UI to enter person data. Log into https://account.mycroft.ai and navigate to the skills part. Once you’ve gotten configured your talent appropriately, you will note one thing like this:
Here, you may uncover which gadgets have your talent put in. In my case, there are two gadgets: Arch Pi4
and Asus
. There are additionally enter textual content containers to get data from the person.
This interface is created mechanically when you have configured Mycroft’s Settings file. You have two selections for file sorts: you may create a settingsmeta.yaml
or a settingsmeta.json
. I choose the YAML syntax, so that’s what I used for this undertaking. Here is my settingsmeta.yaml
for this talent:
skillMetadata:
sections:
- title: OurGroceries Account
fields:
- sort: label
label: "Provide your OurGroceries username/password and then Connect with the button below."
- title: user_name
sort: textual content
label: username
worth: ''
- title: password
sort: password
label: Ourgroceries password
worth: ''
- title: default_list
sort: textual content
label: Default Shopping List
worth: ''
The construction of this file is fairly simple to know. Each file should begin with a skillsMetadata
heading. Next, there’s a sections
heading. Every new part is denoted by - title:
, which is YAML syntax for an merchandise on an inventory. Above, there may be solely a single part known as OurGroceries Account
, however you may have as many sections as you need.
Fields are used to each convey and retailer data. A area may be so simple as a label, which might present an instruction to the person. More fascinating for this talent, nevertheless, are the textual content
and password
fields. Text fields permit the person to view what they’re typing and are displayed in plain textual content. This is appropriate for non-sensitive data. Password fields should not particular to passwords however are supposed to cover delicate data. After the customers enter their data and click on the save
button, Mycroft replaces the settings.json
file created the primary time the talent initializes. The new file incorporates the values the person enter within the internet UI. The talent can even use this file to search for credentials and different data. If you might be having issues utilizing the right values in your talent, check out the settings.json
file for correct naming of variables and whether or not or not values are being saved within the JSON file.
Get person enter with offline Mycroft
As you will have surmised, with out web connectivity, it’s harder to obtain data from finish customers. There are just a few choices. First, you would write your talent such that, on the primary run, it prompts the person for the data your talent requires. You may then write this out to settings.json
in the event you want to use the built-in settings parser, or you would write this to a file of your alternative and your talent may deal with the parsing. Be conscious that in the event you write to settings.json
, there’s a probability that this file might be overwritten if Mycroft re-initializes your talent.
Another technique is having static values in a settings.json
or one other file that’s saved with the undertaking. This has some apparent safety implications, but when your repository is safe, this can be a viable choice.
The third and ultimate choice is to allow the person to edit the file instantly. This might be completed by means of Network File System (NFS) or Samba file sharing protocols, or you would merely grant the suitable permissions to a safe shell (SSH) person who may use any Unix editor to make modifications.
Since this undertaking requires entry to the web, I can’t discover these choices. If you’ve gotten questions, you may at all times interact the group on Mattermost.
Access settings out of your talent
Provided that the opposite elements within the chain are working (i.e., the customers up to date their settings by way of the online UI, and Mycroft up to date settings.json
primarily based on these settings), utilizing user-provided settings is straightforward to know.
As I discussed within the third article (the place I mentioned the __init__
and initialize
strategies), it’s unimaginable to retrieve values from settings.json
with the __init__(self)
technique. Therefore, it’s essential to use one other technique to deal with the settings. In my case, I created an appropriately named _create_initial_grocery_connection
technique:
def _create_initial_grocery_connection(self):
"""
This will get the username/password from the config file and will get the session cookie
for any interactions
:return: None
"""
self.username = self.settings.get('user_name')
self.password = self.settings.get('password')
self.ourgroceries_object = OurGroceries(self.username, self.password)
asyncio.run(self.ourgroceries_object.login())
As you may see, you may extract data from settings.json
through the use of self.settings.get()
. The solely factor to notice is that the worth you go in should match the title in settingsmeta.yaml
. In this case, as a result of I’m not utilizing the username or password outdoors this technique, I may have opted to not make these variables a part of the category scope (i.e., I may have known as them password
as a substitute of self.password
). This is as a result of I’m setting the ourgroceries_object
to the category scope, and it incorporates all the data required for the remainder of the talent to perform.
Wrapping up
Voice assistants are increasing right into a multi-million (if not -billion) greenback enterprise and a few analysts suppose a majority of houses within the subsequent few years can have one (or extra). With Apple, Google, Facebook, and others continuously within the information for privateness violations, to not point out the fixed stream of information breaches reported, you will need to have an open supply, privacy-focused different to the large gamers. Mycroft places your privateness first, and its small however devoted group of contributors is making inroads into the commonest situations for voice assistants.
This sequence dove into the nitty-gritty of talent improvement, speaking concerning the significance of considering issues by means of earlier than you begin and having a very good define. Knowing the place you’re going within the massive image helps you manage your code. Breaking the duties down into particular person items can also be a key a part of your technique. Sometimes, it is a good suggestion to put in writing bits or important chunks outdoors the Mycroft talent surroundings to make sure that your code will work as anticipated. This is just not vital however could be a nice start line for people who find themselves new to talent improvement.
The sequence additionally explored intent parsers and tips on how to perceive when to make use of each. The Padatious and Adapt parsers every have strengths and weaknesses.
- Padatious intents depend on phrases and entities inside these phrases to know what the person is trying to perform, and they’re typically the default used for Mycroft expertise.
- On the opposite hand, Adapt makes use of common expressions to perform related targets. When you want Mycroft to be context-aware, Adapt is the one technique to go. It can also be extraordinarily good at parsing advanced utterances. However, it is advisable take nice care when utilizing common expressions, or you’ll find yourself with sudden outcomes.
I additionally lined the fundamentals of coping with a undertaking. It’s an vital step in advanced talent improvement to make sure that a talent has all the right dependencies to work. Ensuring most portability is paramount for a talent, and dependency decision is a key a part of that, as your talent might not work correctly with unhappy dependencies.
Finally, I defined tips on how to get skill-specific settings from customers, whether or not the system is internet-connected or not. Which technique you select actually relies on your use case.
While it was not my goal to offer an encyclopedia of Mycroft talent improvement, by working by means of this sequence, it’s best to have a really stable basis for creating most expertise you wish to create. I hope the concrete examples on this sequence will present you tips on how to deal with the vast majority of duties you could wish to accomplish throughout talent improvement. I did not go line-by-line by means of the entire talent however the code is hosted on GitLab if you would like to discover it additional. Comments and questions are at all times welcome. I’m very a lot nonetheless studying and rising as a fledgling Mycroft developer, so hit me up on Twitter or the Mycroft Mattermost occasion, and let’s be taught collectively!
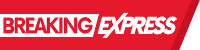