In 1972, Dennis Ritchie was at Bell Labs, the place just a few years earlier, he and his fellow group members invented Unix. After creating an everlasting OS (nonetheless in use right this moment), he wanted a great way to program these Unix computer systems in order that they might carry out new duties. It appears unusual now, however on the time, there have been comparatively few programming languages; Fortran, Lisp, Algol, and B had been common however inadequate for what the Bell Labs researchers wished to do. Demonstrating a trait that may grow to be referred to as a main attribute of programmers, Dennis Ritchie created his personal answer. He referred to as it C, and practically 50 years later, it is nonetheless in widespread use.
Why it is best to study C
Today, there are lots of languages that present programmers extra options than C. The most evident one is C++, a fairly blatantly named language that constructed upon C to create a pleasant object-oriented language. There are many others, although, and there is a good motive they exist. Computers are good at constant repetition, so something predictable sufficient to be constructed right into a language means much less work for programmers. Why spend two traces recasting an int
to a lengthy
in C when one line of C++ (lengthy x = lengthy(n);
) can do the identical?
And but C continues to be helpful right this moment.
First of all, C is a reasonably minimal and easy language. There aren’t very superior ideas past the fundamentals of programming, largely as a result of C is actually one of many foundations of recent programming languages. For occasion, C options arrays, but it surely does not supply a dictionary (except you write it your self). When you study C, you study the constructing blocks of programming that may provide help to acknowledge the improved and elaborate designs of current languages.
Because C is a minimal language, your functions are more likely to get a lift in efficiency that they would not see with many different languages. It’s straightforward to get caught up within the race to the underside if you’re fascinated with how briskly your code executes, so it is necessary to ask whether or not you want extra velocity for a particular job. And with C, you’ve much less to obsess over in every line of code, in comparison with, say, Python or Java. C is quick. There’s a very good motive the Linux kernel is written in C.
Finally, C is simple to get began with, particularly in the event you’re working Linux. You can already run C code as a result of Linux programs embody the GNU C library (glibc
). To write and construct it, all it’s essential to do is set up a compiler, open a textual content editor, and begin coding.
Getting began with C
If you are working Linux, you possibly can set up a C compiler utilizing your bundle supervisor. On Fedora or RHEL:
$ sudo dnf set up gcc
On Debian and related:
$ sudo apt set up build-essential
On macOS, you possibly can install Homebrew and use it to put in GCC:
$ brew set up gcc
On Windows, you possibly can set up a minimal set of GNU utilities, GCC included, with MinGW.
Verify you have put in GCC on Linux or macOS:
$ gcc --version
gcc (GCC) x.y.z
Copyright (C) 20XX Free Software Foundation, Inc.
On Windows, present the complete path to the EXE file:
PS> C:MinGWbingcc.exe --version
gcc.exe (MinGW.org GCC Build-2) x.y.z
Copyright (C) 20XX Free Software Foundation, Inc.
C syntax
C is not a scripting language. It’s compiled, which means that it will get processed by a C compiler to supply a binary executable file. This is completely different from a scripting language like Bash or a hybrid language like Python.
In C, you create capabilities to hold out your required job. A operate named major
is executed by default.
Here’s a easy “hello world” program written in C:
#embody <stdio.h>int major()
printf("Hello world");
return zero;
The first line features a header file, basically free and really low-level C code that you may reuse in your personal packages, referred to as stdio.h
(customary enter and output). A operate referred to as major
is created and populated with a rudimentary print assertion. Save this textual content to a file referred to as hiya.c
, then compile it with GCC:
$ gcc hiya.c --output hiya
Try working your C program:
Return values
It’s a part of the Unix philosophy operate “returns” one thing to you after it executes: nothing upon success and one thing else (an error message, for instance) upon failure. These return codes are sometimes represented with numbers (integers, to be exact): zero represents nothing, and any quantity increased than zero represents some non-successful state.
There’s a very good motive Unix and Linux are designed to count on silence upon success. It’s so to at all times plan for achievement by assuming no errors nor warnings will get in your approach when executing a collection of instructions. Similarly, capabilities in C count on no errors by design.
You can see this for your self with one small modification to make your program seem to fail:
embody <stdio.h>int major()
Compile it:
$ gcc hiya.c --output failer
Now run it utilizing a built-in Linux check for achievement. The &&
operator executes the second half of a command solely upon success. For instance:
$ echo "success" && echo "it worked"
success
it labored
The ||
check executes the second half of a command upon failure.
$ ls blah || echo "it did not work"
ls: can't entry 'blah': No such file or listing
it didn't work
Now attempt your program, which does not return zero upon success; it returns 1 as a substitute:
$ ./failer && echo "it worked"
String is: hiya
The program executed efficiently, but didn’t set off the second command.
Variables and kinds
In some languages, you possibly can create variables with out specifying what sort of knowledge they comprise. Those languages have been designed such that the interpreter runs some assessments in opposition to a variable in an try to find what sort of knowledge it incorporates. For occasion, Python is aware of that var=1
defines an integer if you create an expression that provides var
to one thing that’s clearly an integer. It equally is aware of that the phrase world
is a string if you concatenate hiya
and world
.
C does not do any of those investigations for you; it’s essential to outline your variable sort. There are a number of forms of variables, together with integers (int), characters (char), float, and Boolean.
You can also discover there isn’t any string sort. Unlike Python and Java and Lua and lots of others, C does not have a string sort and as a substitute sees strings as an array of characters.
Here’s some easy code that establishes a char
array variable, after which prints it to your display utilizing printf together with a brief message:
#embody <stdio.h>int major() {
char var[6] = "hello";
printf("Your string is: %srn",var);
You could discover that this code pattern permits six characters for a five-letter phrase. This is as a result of there is a hidden terminator on the finish of the string, which takes up one byte within the array. You can run the code by compiling and executing it:
$ gcc hiya.c --output hiya
$ ./hiya
hiya
Functions
As with different languages, C capabilities take non-compulsory parameters. You can go parameters from one operate to a different by defining the kind of knowledge you desire a operate to simply accept:
#embody <stdio.h>int printmsg(char a[])
int major()
char a[6] = "hello";
printmsg(a);
return zero;
The approach this code pattern breaks one operate into two is not very helpful, but it surely demonstrates that major
runs by default and go knowledge between capabilities.
Conditionals
In real-world programming, you normally need your code to make choices primarily based on knowledge. This is finished with conditional statements, and the if
assertion is among the most elementary of them.
To make this instance program extra dynamic, you possibly can embody the string.h
header file, which incorporates code to look at (because the identify implies) strings. Try testing whether or not the string handed to the printmsg
operate is larger than zero by utilizing the strlen
operate from the string.h
file:
#embody <stdio.h>
#embody <string.h>int printmsg(char a[])
size_t len = strlen(a);
if ( len > zero)int major()
char a[6] = "hello";
printmsg(a);
return 1;
As applied on this instance, the pattern situation won’t ever be unfaithful as a result of the string supplied is at all times “hello,” the size of which is at all times better than zero. The closing contact to this humble re-implementation of the echo
command is to simply accept enter from the consumer.
Command arguments
The stdio.h
file incorporates code that gives two arguments every time a program is launched: a rely of what number of objects are contained within the command (argc
) and an array containing every merchandise (argv
). For instance, suppose you difficulty this imaginary command:
$ foo -i bar
The argc
is three, and the contents of argv
are:
argv[0] = foo
argv[1] = -i
argv[2] = bar
Can you modify the instance C program to simply accept argv[2]
because the string as a substitute of defaulting to hiya
?
Imperative programming
C is an crucial programming language. It is not object-oriented, and it has no class construction. Using C can train you numerous about how knowledge is processed and higher handle the info you generate as your code runs. Use C sufficient, and you will finally have the ability to write libraries that different languages, reminiscent of Python and Lua, can use.
To study extra about C, it’s essential to use it. Look in /usr/embody/
for helpful C header recordsdata, and see what small duties you are able to do to make C helpful to you. As you study, use our C cheat sheet by Jim Hall of FreeDOS. It’s bought all of the fundamentals on one double-sided sheet, so you possibly can instantly entry all of the necessities of C syntax when you observe.
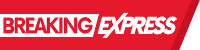