Some folks love studying new programming languages. Other folks cannot think about having to study even one. In this text, I will present you learn how to suppose like a coder in an effort to confidently study any programming language you need.
The fact is, as soon as you have realized learn how to program, the language you employ turns into much less of a hurdle and extra of a formality. In truth, that is simply one of many many causes educators say to teach kids to code early. Regardless of how easy their introductory language could also be, the logic stays the identical throughout all the things else kids (or grownup learners) are prone to encounter later.
With just a bit programming expertise, which you’ll achieve from any one among a number of introductory articles right here on Opensource.com, you’ll be able to go on to study any programming language in just some days (typically much less). Now, this is not magic, and also you do should put some effort into it. And admittedly, it takes rather a lot longer than just some days to study each library out there to a language or to study the nuances of packaging your code for supply. But getting began is less complicated than you may suppose, and the remaining comes naturally with follow.
When skilled programmers sit right down to study a brand new language, they’re on the lookout for 5 issues. Once you recognize these 5 issues, you are prepared to begin coding.
1. Syntax
The syntax of a language describes the construction of code. This encompasses each how the code is written on a line-by-line foundation in addition to the precise phrases used to assemble code statements.
Python, as an illustration, is thought for utilizing indentation to point the place one block ends and one other one begins:
whereas j < rows:
whereas okay < columns:
tile = Tile(okay * w)
board.add(tile)
okay += 1
j += 1
okay = zero
Lua simply makes use of the key phrase finish
:
for i,obj in ipairs(hit) do
if obj.shifting == 1 then
obj.x,obj.y = v.mouse.getPosition()
finish
finish
Java, C, C++, and comparable languages use braces:
whereas (std::getline(e,r))
wc++;
A language’s syntax additionally entails issues like together with libraries, setting variables, and terminating traces. With follow, you may study to acknowledge syntactical necessities (and conventions) nearly subliminally as you learn pattern code.
Take motion
When studying a brand new programming language, attempt to know its syntax. You do not should memorize it, simply know the place to look, must you neglect. It additionally helps to make use of an excellent IDE, as a result of a lot of them warn you of syntax errors as they happen.
2. Built-ins and conditionals
A programming language, identical to a pure language, has a finite variety of phrases it acknowledges as legitimate. This vocabulary may be expanded with further libraries, however the core language is aware of a selected set of key phrases. Most languages haven’t got as many key phrases as you in all probability suppose. Even in a really low-level language like C, there are solely 32 phrases, corresponding to for
, do
, whereas
, int
, float
, char
, break
, and so forth.
Knowing these key phrases offers you the flexibility to write down primary expressions, the constructing blocks of a program. Many of the built-in phrases show you how to assemble conditional statements, which affect the movement of your program. For occasion, if you wish to write a program that permits you to click on and drag an icon, then your code should detect when the consumer’s mouse cursor is positioned over an icon. The code that causes the mouse to seize the icon should execute solely if the mouse cursor is inside the similar coordinates because the icon’s outer edges. That’s a basic if/then assertion, however totally different languages can specific that otherwise.
Python makes use of a mix of if
, elif
, and else
however does not explicitly shut the assertion:
if var == 1:
# motion
elif var == 2:
# some motion
else:
# another motion
Bash makes use of if
, elif
, else
, and makes use of fi
to finish the assertion:
if [ "$var" = "foo" ]; then
# motion
elif [ "$var" = "bar" ]; then
# some motion
else
# another motion
fi
C and Java, nonetheless, use if
, else
, and else if
, enclosed by braces:
if (boolean) else if (boolean)
// some motion
else
While there are small variations in phrase selection and syntax, the fundamentals are at all times the identical. Learn the methods to outline circumstances within the programming language you are studying, together with if/then
, do...whereas
, and case
statements.
Take motion
Get conversant in the core set of key phrases a programming language understands. In follow, your code will comprise greater than only a language’s core phrases, as a result of there are nearly definitely libraries containing plenty of easy features that will help you do issues like print output to the display screen or show a window. The logic that drives these libraries, nonetheless, begins with a language’s built-in key phrases.
three. Data sorts
Code offers with information, so you could find out how a programming language acknowledges totally different varieties of information. All languages perceive integers and most perceive decimals and particular person characters (a, b, c, and so forth). These are sometimes denoted as int
, float
and double
, and char
, however in fact, the language’s built-in vocabulary informs you of learn how to refer to those entities.
Sometimes a language has further information sorts constructed into it, and different occasions complicated information sorts are enabled with libraries. For occasion, Python acknowledges a string of characters with the key phrase str
, however C code should embody the string.h
header file for string options.
Take motion
Libraries can unlock all method of information sorts to your code, however studying the fundamental ones included with a language is a wise start line.
four. Operators and parsers
Once you perceive the varieties of information a programming language offers in, you’ll be able to discover ways to analyze that information. Luckily, the self-discipline of arithmetic is fairly steady, so math operators are sometimes the identical (or a minimum of very comparable) throughout many languages. For occasion, including two integers is often executed with a +
image, and testing whether or not one integer is bigger than one other is often executed with the >
image. Testing for equality is often executed with ==
(sure, that is two equal symbols, as a result of a single equal image is often reserved to set a price).
There are notable exceptions to the plain in languages like Lisp and Bash, however as with all the things else, it is only a matter of psychological transliteration. Once you recognize how the expression is totally different, it is trivial so that you can adapt. A fast overview of a language’s math operators is often sufficient to get a really feel for the way math is completed.
You additionally must know learn how to evaluate and function on non-numerical information, corresponding to characters and strings. These are sometimes executed with a language’s core libraries. For occasion, Python options the break up()
technique, whereas C requires string.h
to supply the strtok()
perform.
Take motion
Learn the fundamental features and key phrases for manipulating primary information sorts, and search for core libraries that show you how to accomplish complicated actions.
5. Functions
Code often is not only a to-do record for a pc. Typically while you write code, you are seeking to current a pc with a set of theoretical circumstances and a set of directions for actions that have to be taken when every situation is met. While movement management with conditional statements and math and logic operators can do rather a lot, code is much more environment friendly as soon as features and courses are launched as a result of they allow you to outline subroutines. For occasion, ought to an utility require a affirmation dialogue field very often, it is rather a lot simpler to write down that field as soon as for instance of a category slightly than re-writing it every time you want it to look all through your code.
You must find out how courses and features are outlined within the programming language you are studying. More exactly, first, you want to study whether or not courses and features can be found within the programming language. Most trendy languages do help features, however courses are specialised constructs widespread to object-oriented languages.
Take motion
Learn the constructs out there in a language that show you how to write and use code effectively.
You can study something
Learning a programming language is, in itself, a type of subroutine of the coding course of. Once you perceive the idea behind how code works, the language you employ is only a medium for delivering logic. The strategy of studying a brand new language is sort of at all times the identical: study syntax by way of easy workout routines, study vocabulary so you’ll be able to construct as much as performing complicated actions, after which follow, follow, follow.
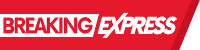