Flutter is a well-liked open supply toolkit for constructing cross-platform apps. In “Create a mobile app with Flutter,” I demonstrated easy methods to set up Flutter on Linux and create your first app. In this text, I will present you easy methods to add a listing of things in your app, with every merchandise opening a brand new display. This is a standard design methodology for cellular apps, so you have in all probability seen it earlier than, however this is a screenshot that will help you visualize it:
Flutter makes use of the Dart language. In among the code snippets under, you may see statements starting with slashes. Two slashes (/ /
) is for code feedback, which clarify sure items of code. Three slashes (/ / /
) denotes Dart’s documentation feedback, which clarify Dart lessons and their properties and different helpful info.
Examine a Flutter app’s most important elements
A typical entry level for a Flutter software is a most important()
perform, often present in a file referred to as lib/most important.dart
:
void most important()
runApp(MyApp());
This methodology is known as when the app is launched. It runs MyApp()
, a Statemuch lessWidget containing all crucial app settings within the MaterialApp()
widget (app theme, preliminary web page to open, and so forth):
class MyApp extends Statemuch lessWidget
The preliminary web page generated is known as MyHomePage()
. It’s a stateful widget that incorporates variables that may be handed to a widget constructor parameter (check out the code above, the place you move the variable title
to the web page constructor):
class MyHomePage extends StatefulWidget
StatefulWidget implies that this web page has its personal state: _MyHomePageState
. It enables you to name the setState()
methodology there to rebuild the web page’s consumer interface (UI):
class _MyHomePageState extends State<MyHomePage>
A construct()
perform in stateful and stateless widgets is answerable for UI look:
@override
Widget construct(BuildContext context)
Modify your app
It’s good observe to separate the most important()
methodology and different pages’ code into totally different information. To achieve this, you could create a brand new .dart file by right-clicking on the lib folder then choosing New > Dart File:
Name the file items_list_page
.
Switch again to your most important.dart
file, minimize the MyHomePage
and _MyHomePageState
code, and paste it into your new file. Next, set your cursor on StatefulWidget
(underlined under in crimson), press Alt+Enter, and choose package deal:flutter/materials.dart
:
This provides flutter/materials.dart
to your file so as to use the default materials widgets Flutter offers.
Then, right-click on MyHomePage class > Refactor > Rename… and rename this class to ItemsListPage
:
Flutter acknowledges that you simply renamed the StatefulWidget class and routinely renames its State class:
Return to the most important.dart
file and alter the title MyHomePage
to ItemsListPage
. Once you begin typing, your Flutter built-in growth atmosphere (in all probability IntelliJ IDEA Community Edition, Android Studio, and VS Code or VSCodium) suggests easy methods to autocomplete your code:
Press Enter to finish your enter. It will add the lacking import to the highest of the file routinely:
You’ve accomplished your preliminary setup. Now you could create a brand new .dart file within the lib folder and title it item_model
. (Note that lessons have UpperCamelCase names, however information have snake_case names.) Paste this code into the brand new file:
/// Class that shops checklist merchandise data:
/// [id] - distinctive identifier, quantity.
/// [icon] - icon to show in UI.
/// Create a listing in a Flutter cellular app - textual content title of the merchandise.
///Flutter is a well-liked open supply toolkit for constructing cross-platform apps. In "Create a mobile app with Flutter," I demonstrated easy methods to set up Flutter on Linux and create your first app. In this text, I will present you easy methods to add a listing of things in your app, with every merchandise opening a brand new display. This is a standard design methodology for cellular apps, so you have in all probability seen it earlier than, however this is a screenshot that will help you visualize it:
read more - textual content description of the merchandise.
class ItemMannequin
// class constructor
ItemMannequin(this.id, this.icon, this.title, this.description);// class fields
closing int id;
closing IconInformation icon;
closing String title;
closing String description;
Return to items_list_page.dart
, and exchange the prevailing _ItemsListPageState
code with:
class _ItemsListPageState extends State<ItemsListPage>// Hard-coded checklist of [ItemModel] to be displayed on our web page.
closing List<ItemMannequin> _items = [
ItemMannequin(zero, Icons.account_balance, 'Balance', 'Some data'),
ItemMannequin(1, Icons.account_balance_wallet, 'Balance pockets', 'Some data'),
ItemMannequin(2, Icons.alarm, 'Alarm', 'Some data'),
ItemMannequin(Three, Icons.my_location, 'My location', 'Some data'),
ItemMannequin(four, Icons.laptop computer, 'Laptop', 'Some data'),
ItemMannequin(5, Icons.backup, 'Backup', 'Some data'),
ItemMannequin(6, Icons.settings, 'Settings', 'Some data'),
ItemMannequin(7, Icons.name, 'Call', 'Some data'),
ItemMannequin(eight, Icons.restore, 'Restore', 'Some data'),
ItemMannequin(9, Icons.camera_alt, 'Camera', 'Some data'),
];@override
Widget construct(BuildContext context)
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
physique: ListView.builder( // Widget which creates [ItemWidget] in scrollable checklist.
itemCount: _items.size, // Number of widget to be created.
merchandiseBuilder: (context, merchandiseIndex) => // Builder perform for each merchandise with index.
ItemWidget(_items[merchandiseIndex], () ),
));
// Method which makes use of BuildContext to push (open) new MaterialsPageRoute (illustration of the display in Flutter navigation mannequin) with ItemParticularsPage (StateFullWidget with UI for web page) in builder.
_onItemFaucet(BuildContext context, int merchandiseIndex)// Statemuch lessWidget with UI for our ItemMannequin-s in ListView.
class ItemWidget extends Statemuch lessWidget
Consider shifting ItemWidget
to a separate file within the lib folder to enhance the readability of your code.
The solely factor lacking is the ItemParticularsPage
class. Create a brand new file within the lib folder and title it item_details_page
. Then copy and paste this code there:
import 'package deal:flutter/materials.dart';import 'item_model.dart';
/// Widget for displaying detailed data of [ItemModel]
class ItemParticularsPage extends StatefulWidget
closing ItemMannequin mannequin;const ItemParticularsPage(this.mannequin, ) : tremendous(key: key);
@override
_ItemParticularsPageState createState() => _ItemParticularsPageState();class _ItemParticularsPageState extends State<ItemParticularsPage>
@override
Widget construct(BuildContext context)
Almost nothing new right here. Notice that _ItemParticularsPageState
is utilizing the widget.merchandise.title
code. It allows referring to the StatefulWidget
fields in its State
class.
Add some animation
Now, it is time to add some primary animation:
- Go to
ItemWidget
code. - Put the cursor on the
Icon()
widget within theconstruct()
methodology. - Press Alt+Enter and choose “Wrap with widget…”
Start typing “Hero” and choose the suggestion for Hero((Key key, @required this, tag, this.create))
:
Next, add the tag property tag: mannequin.id
to the Hero widget:
And the ultimate step is to make the identical change within the item_details_page.dart
file:
The earlier steps wrapped the Icon()
widget with the Hero()
widget. Do you bear in mind in ItemMannequin
you added the id area
however did not use it wherever? The Hero widget takes a novel tag for the kid widget. If Hero detects that totally different app screens (MaterialsPageRoute) have a Hero widget with the identical tag, it’s going to routinely animate the transition between these pages.
Test it out by working the app on an Android emulator or bodily machine. When you open and shut the merchandise particulars web page, you may see a pleasant animation of the icon:
Wrapping up
In this tutorial, you realized:
- The elements of an ordinary, routinely created app
- How so as to add a number of pages that move information amongst one another
- How so as to add a easy animation for these pages
If you need to study extra, try Flutter’s docs (with hyperlinks to pattern initiatives, movies, and “recipes” for creating Flutter apps) and the source code, which is open supply beneath a BSD Three-Clause License.
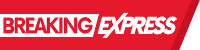