If you need a dwelling safety system to let you know if somebody is lurking round your property, you do not want an costly, proprietary resolution from a third-party vendor. You can arrange your personal system utilizing a Raspberry Pi, a passive infrared (PIR) movement sensor, and an LTE modem that may ship SMS messages at any time when it detects motion.
Prerequisites
You will want:
- A Raspberry Pi with Ethernet connection and Raspberry Pi OS
- An HC-SR501 PIR movement sensor
- 1 purple LED
- 1 inexperienced LED
- An LTE modem (I used the Teltonika TRM240)
- A SIM card
The PIR movement sensor
The PIR movement sensor will sense movement when one thing that emits infrared rays (e.g., a human, animal, or something that emits warmth) strikes within the vary of the sensor’s area or attain. PIR movement sensors are low energy and cheap, in order that they’re utilized in many merchandise that detect movement. They cannot say how many individuals are within the space and the way shut they’re to the sensor; they simply detect movement.
A PIR sensor has two potentiometers, one for setting the delay time and one other for sensitivity. The delay time adjustment units how lengthy the output ought to stay excessive after detecting movement; it may be anyplace from 5 seconds to 5 minutes. The sensitivity adjustment units the detection vary, which could be anyplace from three to seven meters.
The LTE modem
Long-term evolution (LTE) is a typical for wi-fi broadband communication based mostly on the GSM/EDGE and UMTS/HSPA applied sciences. The LTE modem I am utilizing is a USB machine that may add 3G or 4G (LTE) mobile connectivity to a Raspberry PI pc. In this venture, I am not utilizing the modem for mobile connectivity, however to ship messages to my cellphone when movement is detected. I can management the modem with serial communication and AT instructions; the latter ship messages from the modem to my cellphone quantity.
How to arrange your house safety system
Step 1: Install the software program
First, set up the mandatory software program in your Raspberry Pi. In the Raspberry Pi’s terminal, enter:
sudo apt set up python3 python3-gpiozero python-serial -y
Step 2: Set up the modem
Insert your SIM card into your LTE modem by following these instructions for the TRM240. Make certain to mount the antenna on the modem for a greater sign.
Step three: Connect the modem to the Raspberry Pi
Connect the LTE modem to one of many Raspberry Pi’s USB ports, then look forward to the machine in addition up. You ought to see 4 new USB ports within the /dev
listing. You can examine them by executing this command within the terminal:
ls /dev/ttyUSB*
You ought to now see these units:
You will use the ttyUSB2 port to speak with the machine by sending AT instructions.
Step four: Connect the sensors to the Raspberry Pi
-
Connect the PIR sensor:
Connect the VCC and GND pins to the respective pins on the Raspberry Pi, and join the movement sensor’s output pin to the eight pin on the Raspberry Pi. See beneath for a schematic on these connections, and you may be taught extra about Raspberry Pi pin numbering within the GPIO Zero docs. -
Connect the LED lights:
If you need indicator LEDs to mild up when movement is detected, join the cathode (brief leg, flat aspect) of the LED to a floor pin; join the anode (longer leg) to a current-limiting resistor; and join the opposite aspect of the resistor to a GPIO pin (the limiting resistor could be positioned both aspect of the LED). Connect the purple LED to the 38 pin on the board and the inexperienced LED to the 40 pin.
Note that this step is non-obligatory. If you don’t need indicator LEDs when movement is detected, delete the LED sections from the instance code beneath.
Step 5: Launch this system
Using the terminal (or any textual content editor), create a file named motion_sensor.py
, and paste within the example code beneath.
Find and alter these fields:
If you used completely different pins to attach the sensors, be sure to vary the code accordingly.
When all the things is about and able to go, begin this system from the terminal by getting into:
python3 motion_sensor.py
If you do not have the required privileges to start out this system, do that command:
sudo python3 motion_sensor.py
Example code
from gpiozero import MotionSensor, LED
from time import sleep, time
from sys import exit
import serial
import threading# Raspberry Pi GPIO pin config
sensor = MotionSensor(14)
inexperienced = LED(21)
purple = LED(20)# Modem configuration
machine = '/dev/ttyUSB2'
message = '<message>'
phone_number = '<phone_number>'
sms_timeout = 120 # min seconds between SMS messagesdef setup():
port.shut()strive:
port.open()
besides serial.SerialException as e:
print('Error opening machine: ' + str(e))
return False# Turn off echo mode
port.write(b'ATE0 r')
if not check_response('OK', 10):
print('Failed on ATE0')
return False# Enter SMS textual content mode
port.write(b'AT+CMGF=1 r')
if not check_response('OK', 6):
print('Failed on CMGF')
return False# Switch character set to 'worldwide reference alphabet'
# Note: this nonetheless would not assist all characters
port.write(b'AT+CSCS="IRA" r')
if not check_response('OK', 6):
print('Failed on CSCS')
return Falsereturn True
def check_response(string, quantity):
end result = ''strive:
end result = port.learn(quantity).decode()
besides:
return Falseif not string in end result:
strive:
# Write 'ESC' to exit SMS enter mode, simply in case
port.write(b'x1B r')
besides:
return Falsereturn string in end result
def send_sms():
international currently_sending, last_msg_time
currently_sending = Truestrive:
port.write('AT+CMGS="" r'.format(phone_number).encode())
if not check_response('>', 6):
print('Failed on CMGS')
currently_sending = False
return# Write the message terminated by 'Ctrl+Z' or '1A' in ASCII
port.write('x1A r'.format(message).encode())whereas True:
end result = port.readline().decode()if 'OK' in end result:
print('> SMS despatched efficiently')
last_msg_time = time()
currently_sending = False
returnif 'ERROR' in end result:
print('> Failed to ship SMS []'.format(end result.rstrip()))
currently_sending = False
return
besides:
# Initiate setup if the received whereas this system was working
setup()
currently_sending = Falsedef on_motion():
print('Motion detected!')
inexperienced.off()
purple.on()if time() - last_msg_time > sms_timeout and not currently_sending:
print('> Sending SMS...')
threading.Thread(goal=send_sms).begin()def no_motion():
inexperienced.on()
purple.off()print('* Setting up...')
inexperienced.on()
purple.on()port = serial.Serial()
port.port = machine
port.baudrate = 115200
port.timeout = 2last_msg_time = zero
currently_sending = Falseif not setup():
print('* Retrying...')
if not setup():
print('* Try restarting the modem')
exit(1)print('* Do not transfer, organising the PIR sensor...')
sensor.wait_for_no_motion()print('* Device prepared! ', finish='', flush=True)
inexperienced.on()
purple.off()sensor.when_motion = on_motion
sensor.when_no_motion = no_motion
enter('Press Enter or Ctrl+C to exitnn')
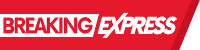