Learning a brand new programming language may be enjoyable. Whenever I attempt to study a brand new one, I concentrate on defining variables, writing an announcement, and evaluating expressions. Once I’ve a basic understanding of these ideas, I can often determine the remainder alone. Most programming languages have some similarities, so as soon as one programming language, studying the following one is a matter of determining the distinctive particulars and recognizing the variations in it.
To assist me follow a brand new programming language, I like to jot down just a few take a look at applications. One pattern program I typically write is an easy “guess the number” program, the place the pc picks a quantity between one and 100 and asks me to guess the quantity. The program loops till I suppose appropriately.
The “guess the number” program workout routines a number of ideas in programming languages: find out how to assign values to variables, find out how to write statements, and find out how to carry out conditional analysis and loops. It’s an important sensible experiment for studying a brand new programming language.
Guess the quantity in Bash
Bash is the usual shell for many Linux methods. Aside from offering a wealthy command-line consumer interface, Bash additionally helps an entire programming language within the type of scripts.
If you are not conversant in Bash, I like to recommend these introductions:
You can discover Bash by writing a model of the “guess the number” recreation. Here is my implementation:
#!/bin/bashquantity=$(( $RANDOM % 100 + 1 ))
echo "Guess a number between 1 and 100"
guess=zero
whereas [ "zero$guess" -ne $quantity ] ; do
learn guess
[ "zero$guess" -lt $quantity ] && echo "Too low"
[ "zero$guess" -gt $quantity ] && echo "Too high"
accomplishedecho "That's right!"
exit zero
Breaking down the script
The first line within the script, #!/bin/bash
tells Linux to run this script utilizing the Bash shell. Every script begins with the #!
character pair, which signifies this can be a shell script. What instantly follows #!
is the shell to run. In this case, /bin/bash
is the Bash shell.
To assign a price to a variable, checklist the variable’s identify adopted by the =
signal. For instance, the assertion guess=zero
assigns a zero worth to the guess
variable.
You may immediate the consumer to enter a price utilizing the learn
assertion. If you write learn guess
, Bash waits for the consumer to enter some textual content then shops that worth within the guess
variable.
To reference the worth of a variable, use $
earlier than the variable identify. So, having saved a price within the guess
variable, you possibly can retrieve it utilizing $guess
.
You can use no matter names you want for variables, however Bash reserves just a few particular variable names for itself. One particular variable is RANDOM
, which generates a really massive random quantity each time you reference it.
If you wish to carry out an operation on the similar time you retailer a price, it’s worthwhile to enclose the assertion in particular brackets. This tells Bash to execute that assertion first, and the =
shops the ensuing worth within the variable. To consider a mathematical expression, use $(( ))
round your assertion. The double parentheses point out an arithmetic expression. In my instance, quantity=$(( $RANDOM % 100 + 1 ))
evaluates the expression $RANDOM % 100 + 1
after which shops the worth within the quantity
variable.
Standard arithmetic operators equivalent to +
(plus), -
(minus), *
(multiply), /
(divide), and %
(modulo) apply.
That means the assertion quantity=$(( $RANDOM % 100 + 1 ))
generates a random quantity between one and 100. The modulo operator (%
) returns the the rest after dividing two numbers. In this case, Bash divides a random quantity by 100, leaving a the rest within the vary zero to 99. By including one to that worth, you get a random quantity between one and 100.
Bash helps conditional expressions and move management like loops. In the “guess the number” recreation, Bash continues looping so long as the worth in guess
isn’t equal to quantity
. If the guess is lower than the random quantity, Bash prints “Too low,” and if the guess is bigger than the quantity, Bash prints “Too high.”
How it really works
Now that you have written your Bash script, you possibly can run it to play the “guess the number” recreation. Continue guessing till you discover the proper quantity:
Guess a quantity between 1 and 100
50
Too excessive
30
Too excessive
20
Too excessive
10
Too low
15
Too excessive
13
Too low
14
That's proper!
Every time you run the script, Bash will choose a special random quantity.
This “guess the number” recreation is a good introductory program when studying a brand new programming language as a result of it workout routines a number of widespread programming ideas in a fairly simple manner. By implementing this straightforward recreation in numerous programming languages, you possibly can exhibit some core ideas and examine particulars in every language.
Do you could have a favourite programming language? How would you write the “guess the number” recreation in it? Follow this text sequence to see examples of different programming languages which may curiosity you.
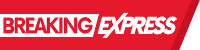