The first compiled programming language I discovered was Fortran 77. While rising up, I taught myself write applications in BASIC on the Apple II and later in QBasic on DOS. But once I went to school to review physics, I discovered Fortran.
Fortran was once fairly frequent in scientific computing. And as soon as upon a time, all laptop methods had a Fortran compiler. Fortran was once as ubiquitous as Python is in the present day. So in the event you had been a physics pupil like me, working within the 1990s, you discovered Fortran.
I all the time thought Fortran was considerably just like BASIC, so I shortly took to Fortran each time I wanted to jot down a fast program to investigate lab knowledge or carry out another numerical evaluation. And once I acquired bored, I wrote a “Guess the number” recreation in Fortran, the place the pc picks a quantity between one and 100 and asks me to guess the quantity. The program loops till I suppose accurately.
The “Guess the number” program workouts a number of ideas in programming languages: assign values to variables, write statements, and carry out conditional analysis and loops. It’s a terrific sensible experiment for studying a brand new programming language.
The fundamentals of Fortran programming
While Fortran has been up to date through the years, I’m most aware of Fortran 77, the implementation I discovered years in the past. Fortran was created when programmers wrote applications on punched playing cards, so “classic” Fortran is proscribed to the info you may placed on a punched card. That means you may solely write traditional Fortran applications with these limitations:
- Only one line of supply code per card is allowed.
- Only columns 1–72 are acknowledged (the final eight columns, 73-80, are reserved for the cardboard sorter).
- Line numbers (“labels”) are in columns 1–5.
- Program statements go in columns 7–72.
- To proceed a program line, enter a continuation character (often
+
) in column 6. - To create a remark line, enter
C
or*
in column 1. - Only the characters
A
toZ
(uppercase),zero
to9
(numbers), and the particular characters= + - * / ( ) , . $ ' :
and house are used.
With these limitations, you possibly can nonetheless write very helpful and fascinating applications.
Guess the quantity in Fortran
Explore Fortran by writing the “Guess the number” recreation. This is my implementation:
CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC
C PROGRAM TO GUESS A NUMBER 1-100
CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC
PROGRAM GUESSNUM
INTEGER SEED, NUMBER, GUESSPRINT *, 'ENTER A RANDOM NUMBER SEED'
READ *, SEED
CALL SRAND(SEED)NUMBER = INT( RAND(zero) * 100 + 1 )
PRINT *, 'GUESS A NUMBER BETWEEN 1 AND 100'
10 READ *, GUESSIF (GUESS.LT.NUMBER) THEN
PRINT *, 'TOO LOW'
ELSE IF (GUESS.GT.NUMBER) THEN
PRINT *, 'TOO HIGH'
ENDIFIF (GUESS.NE.NUMBER) GOTO 10
PRINT *, 'THATS RIGHT!'
END
If you’re aware of different programming languages, you possibly can in all probability determine what this program is doing by studying the supply code. The first three traces are a remark block to point this system’s operate. The fourth line, PROGRAM GUESSNUM
, identifies this as a program, and it’s closed by the END
assertion on the final line.
After defining just a few variables, this system prompts the person to enter a random quantity seed. A Fortran program can’t initialize the random quantity generator from the working system, so you should all the time begin the random quantity generator with a “seed” worth and the SRAND
subroutine.
Fortran generates random numbers between zero and zero.999… with the RAND(zero)
operate. The zero
worth tells the RAND
operate to generate one other random quantity. Multiply this random quantity by 100 to generate a quantity between zero and 99.999…, after which add 1 to get a worth between 1 and 100.999… The INT
operate truncates this to an integer; thus, the variable NUMBER
is a random quantity between 1 and 100.
The program prompts the person, then enters a loop. Fortran does not assist the whereas
or do-while
loops out there in additional trendy programming languages. Instead, you should assemble your personal utilizing labels (line numbers) and GOTO
statements. That’s why the READ
assertion has a line quantity; you possibly can leap to this label with the GOTO
on the finish of the loop.
Punched playing cards didn’t have the <
(lower than) or >
(higher than) symbols, so Fortran adopted a distinct syntax to match values. To take a look at if one worth is lower than one other, use the .LT.
(lower than) comparability. To take a look at if a worth is bigger than one other, use .GT.
(higher than). Equal and never equal are .EQ.
and .NE.
, respectively.
In every iteration of the loop, this system checks the person’s guess. If the person’s guess is lower than the random quantity, this system prints TOO LOW
, and if the guess is bigger than the random quantity, this system prints TOO HIGH
. The loop continues till the person’s guess is identical because the random quantity.
When the loop exits, this system prints THATS RIGHT!
and ends instantly.
$ gfortran -Wall -o guess guess.f$ ./guess
ENTER A RANDOM NUMBER SEED
93759
GUESS A NUMBER BETWEEN 1 AND 100
50
TOO LOW
80
TOO HIGH
60
TOO LOW
70
TOO LOW
75
TOO HIGH
73
TOO LOW
74
THATS RIGHT!
Every time you run this system, the person must enter a distinct random quantity seed. If you all the time enter the identical seed, this system will all the time choose the identical random quantity.
Try it in different languages
This “guess the number” recreation is a superb introductory program when studying a brand new programming language as a result of it workouts a number of frequent programming ideas in a fairly easy manner. By implementing this straightforward recreation in numerous programming languages, you possibly can exhibit some core ideas and examine every language’s particulars.
Do you’ve got a favourite programming language? How would you write the “guess the number” recreation in it? Follow this text sequence to see examples of different programming languages that may curiosity you.
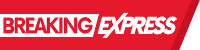