It’s fairly secure to say that a lot of the trendy internet wouldn’t exist with out JavaScript. It’s one of many three normal internet applied sciences (together with HTML and CSS) and permits anybody to create a lot of the interactive, dynamic content material we have now come to count on in our experiences with the World Wide Web. From frameworks like React to knowledge visualization libraries like D3, it is arduous to think about the net with out it.
There’s rather a lot to study, and a good way to start studying this widespread language is by writing a easy utility to turn into aware of some ideas. Recently, some Opensource.com correspondents have written about how one can study their favourite language by writing a easy guessing recreation, in order that’s an amazing place to begin!
Getting began
JavaScript is available in many flavors, however I will begin with the essential model, generally referred to as “Vanilla JavaScript.” JavaScript is primarily a client-side scripting language, so it may run in any normal browser with out putting in something. All you want is a code editor (Brackets is a good one to strive) and the net browser of your alternative.
HTML consumer interface
JavaScript runs in an internet browser and interacts with the opposite normal internet applied sciences, HTML and CSS. To create this recreation, you may first use HTML (Hypertext Markup Language) to create a easy interface in your gamers to make use of. In case you are not acquainted, HTML is a markup language used to offer construction to content material on the internet.
To begin, create an HTML file in your code. The file ought to have the .html
extension to let the browser know that it’s an HTML doc. You can name your file guessingGame.html
.
Use a number of primary HTML tags on this file to show the sport’s title, directions for how one can play, interactive components for the participant to make use of to enter and submit their guesses, and a placeholder for offering suggestions to the participant:
<!DOCTYPE>
<html>
<head>
<meta charset="UTF-8" />
<title> JavaScript Guessing Game </title>
</head>
<body>
<h1>Guess the Number!</h1>
<p>I'm considering of a quantity between 1 and 100. Can you guess what it's?</p>
<label for="guess">My Guess</label>
<input kind="number" id="guess">
<input kind="submit" id="submitGuess" worth="Check My Guess">
<p id="feedback"></p>
</body>
</html>
The <h1>
and <p>
components let the browser know what kind of textual content to show on the web page. The set of <h1>
tags signifies that the textual content between these two tags (Guess the Number!
) is a heading. The set of <p>
tags that observe signify that the quick block of textual content with the directions is a paragraph. The empty set of <p>
tags on the finish of this code block function a placeholder for the suggestions the sport will give the participant based mostly on their guess.
The <script> tag
There are some ways to incorporate JavaScript in an internet web page, however for a brief script like this one, you should utilize a set of <script>
tags and write the JavaScript immediately within the HTML file. Those <script>
tags ought to go proper earlier than the closing </physique>
tag close to the tip of the HTML file.
Now, you can begin to write down your JavaScript between these two script tags. The closing file seems like this:
<!DOCTYPE>
<html><head>
<meta charset="UTF-8" />
<title> JavaScript Guessing Game </title>
</head><body>
<h1>Guess the Number!</h1>
<p>I'm considering of a quantity between 1 and 100. Can you guess what it's?</p><form>
<label for="guess">My Guess</label>
<input kind="number" id="guess">
<input kind="submit" id="submitGuess" worth="Check My Guess">
</form><script>
const randomNumber = Math.ground(Math.random() * 100) + 1
console.log('Random Number', randomNumber)operate examineGuess()
submitGuess.addEventListener('click on', examineGuess)
</script></body>
</html>
To run this within the browser, both double-click on the file or go to the menu in your favourite internet browser and select File > Open File. (If you’re utilizing Brackets, you can too use the lightning-bolt image within the nook to open the file within the browser).
Pseudo-random quantity technology
The first step within the guessing recreation is to generate a quantity for the participant to guess. JavaScript contains a number of built-in international objects that enable you write code. To generate your random quantity, use the Math object.
Math has properties and capabilities for working with mathematical ideas in JavaScript. You will use two Math capabilities to generate the random quantity in your participant to guess.
Start with Math.random(), which generates a pseudo-random quantity between zero and 1. (Math.random is inclusive of zero however unique of 1. This implies that the operate may generate a zero, however it is going to by no means generate a 1.)
For this recreation, set the random quantity between 1 and 100 to slender down the participant’s choices. Take the decimal you simply generated and multiply it by 100 to supply a decimal between zero and…not fairly 100. But you may maintain that in a number of extra steps.
Right now, your quantity continues to be a decimal, and also you need it to be a complete quantity. For that, you should utilize one other operate that’s a part of the Math object, Math.floor(). Math.ground()’s function is to return the most important integer that’s lower than or equal to the quantity you give it as an argument—which implies it rounds all the way down to the closest entire quantity:
Math.ground(Math.random() * 100)
That leaves you with a complete quantity between zero and 99, which is not fairly the vary you need. You can repair that along with your final step, which is so as to add 1 to the consequence. Voila! Now you will have a (considerably) randomly generated quantity between 1 and 100:
Math.ground(Math.random() * 100) + 1
Variables
Now you’ll want to retailer the randomly generated quantity with the intention to examine it to your participant’s guesses. To try this, you’ll be able to assign it to a variable.
JavaScript has several types of variables you’ll be able to select, relying on the way you need to use the variable. For this recreation, use const and let.
- let is used for variables if their worth can change all through the code.
- const is used for variables if their worth shouldn’t be modified.
There’s just a little extra to const and let, however that is all you’ll want to know for now.
The random quantity is generated solely as soon as within the recreation, so you’ll use a const variable to carry the worth. You need to give the variable a reputation that makes it clear what worth is being saved, so title it randomNumber
:
const randomNumber
A be aware on naming: Variables and performance names in JavaScript are written in camel case. If there is only one phrase, it’s written in all decrease case. If there’s a couple of phrase, the primary phrase is all decrease case, and any further phrases begin with a capital letter with no areas between the phrases.
Logging to the console
Normally, you do not need to present anybody the random quantity, however builders might need to know the quantity that was generated to make use of it to assist debug the code. With JavaScript, you should utilize one other built-in operate, console.log(), to output the quantity to the console in your browser.
Most browsers embody Developer Tools which you can open by urgent the F12 key in your keyboard. From there, it’s best to see a tab labeled Console. Any data logged to the console will seem right here. Since the code you will have written to this point will run as quickly because the browser masses, if you happen to have a look at the console, it’s best to see the random quantity that you just simply generated! Hooray!
Functions
Next, you want a option to get the participant’s guess from the quantity enter subject, examine it to the random quantity you simply generated, and provides the participant suggestions to allow them to know in the event that they guessed accurately. To try this, write a operate. A operate is code that’s grouped to carry out a activity. Functions are reusable, which implies if you’ll want to run the identical code a number of occasions, you’ll be able to name the operate as an alternative of rewriting the entire steps wanted to carry out the duty.
Depending on the JavaScript model you’re utilizing, there are lots of other ways to write down, or declare, a operate. Since that is an introduction to the language, declare your operate utilizing the essential operate syntax.
Start with the key phrase operate
after which give the operate a reputation. It’s good follow to make use of a reputation that’s an motion that describes what the operate does. In this case, you’re checking the participant’s guess, so an applicable title for this operate can be examineGuess
. After the operate title, write a set of parentheses after which a set of curly braces. You will write the physique of the operate between these curly braces:
operate examineGuess()
Access the DOM
One of the needs of JavaScript is to work together with HTML on a webpage. It does this by the Document Object Model (DOM), which is an object JavaScript makes use of to entry and alter the data on an internet web page. Right now, you’ll want to get the participant’s guess from the quantity enter subject you arrange within the HTML. You can try this utilizing the id
attribute you assigned to the HTML components, which on this case is guess
:
<enter kind="number" id="guess">
JavaScript can get the quantity the participant enters into the quantity enter subject by accessing its worth. You can do that by referring to the factor’s id and including .worth
to the tip. This time, use a let
variable to carry the worth of the consumer’s guess:
let myGuess = guess.worth
Whatever quantity the participant enters into the quantity enter subject can be assigned to the myGuess
variable within the examineGuess
operate.
Conditional statements
The subsequent step is to match the participant’s guess with the random quantity the sport generates. You additionally need to give the participant suggestions to allow them to know if their guess was too excessive, too low, or appropriate.
You can resolve what suggestions the participant will obtain by utilizing a sequence of conditional statements. A conditional assertion checks to see if a situation is met earlier than operating a code block. If the situation shouldn’t be met, the code stops, strikes on to examine the subsequent situation, or continues with the remainder of the code with out operating the code within the conditional block:
if (myGuess === randomNumber)
else if(myGuess > randomNumber)
suggestions.textContent = "Your guess was " + myGuess + ". That's too high. Try Again!"
else if(myGuess < randomNumber)
suggestions.textContent = "Your guess was " + myGuess + ". That's too low. Try Again!"
The first conditional block compares the participant’s guess to the random quantity the sport generates utilizing a comparability operator ===
. The comparability operator checks the worth on the fitting, compares it to the worth on the left, and returns the boolean true
in the event that they match and false
if they do not.
If the quantity matches (yay!), be sure that the participant is aware of. To do that, manipulate the DOM by including textual content to the <p>
tag that has the id attribute “feedback.” This works similar to guess.worth
above, besides as an alternative of getting data from the DOM, it adjustments the data in it. <p>
components do not have a price like <enter>
components—they’ve textual content as an alternative, so use .textContent
to entry the factor and set the textual content you need to show:
suggestions.textContent = "You got it right!"
Of course, there’s a good probability that the participant did not guess proper on the primary strive, so if myGuess
and randomNumber
do not match, give the participant a clue to assist them slender down their guesses. If the primary conditional fails, the code will skip the code block in that if
assertion and examine to see if the subsequent situation is true. That brings you to your else if
blocks:
else if(myGuess > randomNumber)
suggestions.textContent = "Your guess was " + myGuess + ". That's too high. Try Again!"
If you have been to learn this as a sentence, it is likely to be one thing like this: “If the participant’s guess is the same as the random quantity, allow them to know they acquired it proper. Otherwise (else), examine if the participant’s guess is larger than randomNumber
, and whether it is, show the participant’s guess and inform them it was too excessive.”
The final chance is that the participant’s guess was decrease than the random quantity. To examine that, add yet one more else if
block:
else if(myGuess < randomNumber)
suggestions.textContent = "Your guess was " + myGuess + ". That's too low. Try Again!"
User occasions and occasion listeners
If you have a look at your script, you may see that a few of the code runs mechanically when the web page masses, however a few of it doesn’t. You need to generate the random quantity earlier than the sport is performed, however you do not need to examine the participant’s guess till they’ve entered it into the quantity enter subject and are able to examine it.
The code to generate the random quantity and log it to the console is exterior of a operate, so it is going to run mechanically when the browser masses your script. However, for the code inside your operate to run, you must name it.
There are a number of methods to name a operate. Here, you need the operate to run when the participant clicks on the “Check My Guess” button. Clicking a button creates a consumer occasion, which the JavaScript code can then “listen” for in order that it is aware of when it must run a operate.
The final line of code provides an occasion listener to the button to “listen” for when the button is clicked. When it “hears” that occasion, it is going to run the operate assigned to the occasion listener:
submitGuess.addEventListener('click on', examineGuess)
Just like the opposite cases the place you entry DOM components, you should utilize the button’s id to inform JavaScript which factor to work together with. Then you should utilize the built-in addEventListener
operate to inform JavaScript what occasion to pay attention for.
You have already seen a operate that takes parameters, however take a second to have a look at how this works. Parameters are data operate must carry out its activity. Not all capabilities want parameters, however the addEventListener
operate wants two. The first parameter it takes is the title of the consumer occasion for which it is going to pay attention. The consumer can work together with the DOM in some ways, like typing, transferring the mouse, tabbing with the keyboard, or copying and pasting textual content. In this case, the consumer occasion you’re listening for is a button click on, so the primary parameter can be click on
.
The second piece of data addEventListener
wants is the title of the operate to run when the consumer clicks the button. In this case, it is the examineGuess
operate.
Now, when the participant presses the “Check My Guess” button, the examineGuess
operate will get the worth they entered within the quantity enter subject, examine it to the random quantity, and show suggestions within the browser to let the participant understand how they did. Awesome! Your recreation is able to play.
Learn JavaScript for enjoyable and revenue
This little bit of Vanilla JavaScript is only a small style of what this huge ecosystem has to supply. It’s a language effectively value investing time into studying, and I encourage you to proceed to dig in and study extra.
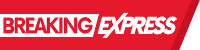