In the earlier articles on this sequence, I defined why tackling coding issues , as in the event that they have been hordes of zombies, is a mistake. I am utilizing a useful acronym explaining why it is higher to strategy issues incrementally. ZOMBIES stands for:
Z – Zero
O – One
M – Many (or extra advanced)
B – Boundary behaviors
I – Interface definition
E – Exercise distinctive habits
S – Simple eventualities, easy options
In the primary 4 articles on this sequence, I demonstrated the primary 5 ZOMBIES rules. The first article implemented Zero, which offers the best potential path by your code. The second article carried out tests with One and Many samples, the third article checked out Boundaries and Interfaces, and the fourth examined Exceptional behavior. In this text, I will check out the ultimate letter within the acronym: S, which stands for “simple scenarios, simple solutions.”
Simple eventualities, easy options in motion
If you return and look at all of the steps taken to implement the procuring API on this sequence, you will see a purposeful determination to all the time persist with the best potential eventualities. In the method, you find yourself with the best potential options.
There you may have it: ZOMBIES enable you ship sturdy, elegant options by adhering to simplicity.
Victory?
It may appear you are finished right here, and a much less conscientious engineer would very possible declare victory. But enlightened engineers all the time probe a bit deeper.
One train I all the time advocate is mutation testing. Before you wrap up this train and go on to combat new battles, it’s clever to provide your answer an excellent shakeout with mutation testing. And moreover, you must admit that mutation matches effectively in a battle in opposition to zombies.
Use the open supply Stryker.NET to run mutation assessments.
It appears like you may have one surviving mutant! That’s not an excellent signal.
What does this imply? Just if you thought you had a rock-solid, sturdy answer, Stryker.NET is telling you that not all the things is rosy in your neck of the woods.
Take a have a look at the pesky mutant who survived:
The mutation testing device took the assertion:
if(complete > 500.00) {
and mutated it to:
if(complete >= 500.00) {
Then it ran the assessments and realized that not one of the assessments complained in regards to the change. If there’s a change in processing logic and not one of the assessments complain in regards to the change, which means you may have a surviving mutant.
Why mutation issues
Why is a surviving mutant an indication of hassle? It’s as a result of the processing logic you craft governs the habits of your system. If the processing logic adjustments, the habits ought to change, too. And if the habits adjustments, the expectations encoded within the assessments must be violated. If these expectations are usually not violated, that implies that the expectations are usually not exact sufficient. You have a loophole in your processing logic.
To repair this, you want to “kill” the surviving mutant. How do you do this? Typically, the truth that a mutant survived means at the very least one expectation is lacking.
Look by your code to see what expectation, if any, is just not there:
- You clearly outlined the expectation newly created basket has zero gadgets (and, by implication, has a $zero grand complete).
- You additionally outlined the expectation that including one merchandise will end result within the basket having one merchandise, and if the merchandise worth is $10, the grand complete can be $10.
- Furthermore, you outlined the expectation that including two gadgets to the basket, one merchandise priced at $10 and the opposite at $20, leads to a grand complete of $30.
- You additionally declared expectations relating to the removing of things from the basket.
- Finally, you outlined the expectation that any order complete larger than $500 leads to a worth low cost. The enterprise coverage rule dictates that in such a case, the low cost is 10% of the order’s complete worth.
What is lacking? According to the mutation testing report, you by no means outlined an expectation relating to what enterprise coverage rule applies when the order complete is precisely $500. You outlined what occurs if the order complete is larger than the $500 threshold and what occurs when the order complete is lower than $500.
Define this edge-case expectation:
[Fact]
public void Add2ItemsTotal500GrandTotal500()
var expectedGrandTotal = 500.00;
var actualGrandTotal = 450;
Assert.Equal(expectedGrandTotal, actualGrandTotal);
The first stab fakes the expectation to make it fail. You now have 9 microtests; eight succeed, and the ninth take a look at fails:
[xUnit.net 00:00:00.57] assessments.UnitTest1.Add2ItemsTotal500GrandTotal500 [FAIL]
X assessments.UnitTest1.Add2ItemsTotal500GrandTotal500 [2ms]
Error Message:
Assert.Equal() Failure
Expected: 500
Actual: 450
[...]
Test Run Failed.
Total assessments: 9
Passed: eight
Failed: 1
Total time: 1.5920 Seconds
Replace hard-coded values with an expectation of a affirmation instance:
[Fact]
public void Add2ItemsTotal500GrandTotal500()
You added two gadgets, one priced at $400, the opposite at $100, totaling $500. After calculating the grand complete, you count on that it will likely be $500.
Run the system. All 9 assessments move!
Total assessments: 9
Passed: 9
Failed: zero
Total time: 1.0440 Seconds
Now for the second of fact. Will this new expectation take away all mutants? Run the mutation testing and verify the outcomes:
Success! All 10 mutants have been killed. Great job; now you can ship this API with confidence.
Epilogue
If there’s one takeaway from this train, it is the rising idea of skillful procrastination. It’s an important idea, realizing that many people are inclined to rush mindlessly into envisioning the answer even earlier than our prospects have completed describing their drawback.
Positive procrastination
Procrastination would not come simply to software program engineers. We’re wanting to get our fingers soiled with the code. We know by coronary heart quite a few design patterns, anti-patterns, rules, and ready-made options. We’re itching to place them into executable code, and we lean towards doing it in massive batches. So it’s certainly a advantage to maintain our horses and punctiliously think about every step we make.
This train proves how ZOMBIES enable you take many deliberate small steps towards options. It’s one factor to pay attention to and to agree with the Yagni precept, however within the “heat of the battle,” these deep issues usually fly out the window, and you find yourself throwing in all the things and the kitchen sink. And that produces bloated, tightly coupled techniques.
Iteration and incrementation
Another important takeaway from this train is the conclusion that the one option to hold a system working always is by adopting an iterative strategy. You developed the procuring API by making use of some rework, which is to say, you proceeded with coding by making adjustments to code that you just already modified. This rework is unavoidable when iterating on an answer.
One of the issues many groups expertise is confusion associated to iteration and increments. These two ideas are essentially totally different.
An incremental strategy is predicated on the concept that you maintain a crisp set of necessities (or a blueprint) in your hand, and also you go and construct the answer by working incrementally. Basically, you construct it piece-by-piece, and when all items have been assembled, you place them collectively, and voila! The answer is able to be shipped!
In distinction, in an iterative strategy, you might be much less sure that you realize all that must be identified to ship the anticipated worth to the paying buyer. Because of that realization, you proceed gingerly. You’re cautious of breaking the system that already works (i.e., the system in a steady-state). If you disturb that steadiness, you all the time attempt to disturb it within the least intrusive, least invasive method. You deal with taking the smallest conceivable batches, then shortly wrapping up your work on every batch. You choose to have the system again to the steady-state in a matter of minutes, generally even seconds.
That’s why an iterative strategy so usually adheres to “faux it ’til you make it.” You hard-code many expectations so that you could confirm tiny change doesn’t disable the system from working. You then make the adjustments crucial to switch the hard-coded worth with actual processing.
As a rule of thumb, in an iterative strategy, you intention to craft an expectation (a microtest) in such a approach that it precipitates just one enchancment to the code. You go one enchancment by one enchancment, and with every enchancment, you train the system to ensure it’s in a working state. As you proceed in that style, you finally hit the stage the place all of the expectations have been met, and the code has been refactored in such a approach that it leaves no surviving mutants.
Once you get to that state, you might be pretty assured you can ship the answer.
Many because of inimitable Kent Beck, Ron Jeffries, and GeePaw Hill for being a relentless inspiration on my journey to software program engineering apprenticeship.
And might your journey be full of ZOMBIES.
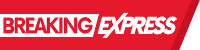