Last summer season, my spouse and I offered the whole lot we owned and moved with our two canine to Hawaii. It’s been the whole lot we thought it could be: stunning solar, heat sand, cool surf—you identify it. We’ve additionally run into some issues we did not anticipate: WiFi issues.
Now, that is not a Hawaii downside. It’s restricted to the condominium we’re renting. We live in a single-room studio condominium hooked up to our landlord’s condominium. Part of the hire consists of free web! YAY! However, mentioned web is supplied by the WiFi router within the landlord’s condominium. BOO!
In all honesty, it really works OK. Ish. OK, it would not work properly, and I am unsure why. The router is actually on the opposite facet of the wall, however our sign is spotty, and we have now some bother staying related. Back residence, our WiFi router’s sign crossed via many partitions and a few flooring. Certainly, it coated an space bigger than the 600 sq. foot condominium we stay in!
What does techie do in such a scenario? Why, examine, after all!
Luckily the “everything we own” that we offered earlier than transferring right here didn’t embrace our Raspberry Pi Zero W. So small! So transportable! Of course, I took it to Hawaii with me. My brilliant concept was to make use of the Pi and its built-in WiFi adapter, write somewhat program in Go to measure the WiFi sign acquired from the router, and show that output. I will make it tremendous easy, fast, and soiled and fear later about making it higher. I simply wish to know what’s up with the WiFi, dang it!
Hunting round on Google for a minute turns up a comparatively helpful Go bundle for working with WiFi, mdlayher/wifi. Sounds promising!
Getting details about the WiFi interfaces
My plan is to question the WiFi interface statistics and return the sign power, so I want to seek out the interfaces on the system. Luckily the mdlayher/wifi bundle has a technique to question them, so I can try this by making a file named fundamental.go
:
bundle fundamentalimport (
"fmt""github.com/mdlayher/wifi"
)func fundamental()
c, err := wifi.New()
defer c.Close()if err != nil
interfaces, err := c.Interfaces()
for _, x := vary interfaces
fmt.Printf("%+vn", x)
So, what is going on on right here? After importing it, the mdlayher/wifi module can be utilized in the principle perform to create a brand new Client (sort *Client
). The new shopper (named c
) can then get an inventory of the interfaces on the system with c.Interfaces()
. Then it may well loop over the slice of Interface pointers and print details about them.
By including “+” to %+v
, it prints the names of the fields within the *Interface
struct, too, which helps me establish what I am seeing with out having to refer again to documentation.
Running the code above offers an inventory of the WiFi interfaces on my machine:
&
&Index:three Name:wlp2s0 HardwareAddr:5c:5f:67:f3:0a:a7 PHY:zero Device:1 Type:station Frequency:2412
Note that the MAC handle, HardwareAddr
, is identical for each traces, which means this is identical bodily hardware. This is confirmed by PHY: zero
. The Go wifi module’s docs observe that PHY
is the bodily system to which the interface belongs.
The first interface has no identify and is TYPE:P2P
. The second, named wpl2s0
is TYPE:Station
. The wifi module documentation lists the different types of interfaces and describes what they’re. According to the docs, the “P2P” sort signifies “an interface is a device within a peer-to-peer client network.” I consider, and please right me within the feedback if I am improper, that this interface is for WiFi Direct, an ordinary for permitting two WiFi units to attach with out an intermediate entry level.
The “Station” sort signifies “an interface is part of a managed basic service set (BSS) of client devices with a controlling access point.” This is the usual perform for a wi-fi system that most individuals are used to—as a shopper related to an entry level. This is the interface that issues for testing the standard of the WiFi.
Getting the Station data from the interface
Using this data, I can replace the loop over the interfaces to retrieve the data I am on the lookout for:
for _, x := vary interfaces
First, it checks that x.Type
(the Interface sort) is wifi.InterfaceTypeStation
—a Station interface (that is the one sort that issues for this train). This is an unlucky naming collision—the interface “type” is just not a “type” within the Golang sense. In reality, what I am engaged on here’s a Go sort
named InterfaceType
to symbolize the kind of interface. Whew, that took me a minute to determine!
So, assuming the interface is of the right sort, the station data might be retrieved with c.StationInfo(x)
utilizing the shopper StationInfo()
technique to get the information concerning the interface, x
.
This returns a slice of *StationInfo
pointers. I am unsure fairly why there is a slice. Perhaps the interface can have a number of StationInfo responses? In any case, I can loop over the slice and use the identical +%v
trick to print the keys and values for the StationInfo struct.
Running the above returns:
&
The factor I am thinking about is the “Signal” and probably “TransmitFailed” and “BeaconLoss.” The sign is reported in models of dBm (or decibel-milliwatts).
A fast apart: How to learn WiFi dBm
According to MetaGeek:
- –30 is the very best sign power—it is neither life like nor needed
- –67 is superb; it is for apps that want dependable packet supply, like streaming media
- –70 is truthful, the minimal dependable packet supply, advantageous for e-mail and net
- –80 is poor, absolute primary connectivity, unreliable packet supply
- –90 is unusable, approaching the “noise floor”
Note that dBm is logarithmic scale: -60 is 1,000x decrease than -30
Making this an actual “scanner”
So, my sign from above: –79. YIKES, not good. But that single consequence is just not particularly useful. That’s only a point-in-time reference and solely legitimate for the actual bodily house the place the WiFi community adapter was at that instantaneous. What could be extra helpful could be a steady studying, making it doable to see how the sign adjustments because the Raspberry Pi strikes round. The fundamental perform might be tweaked once more to perform this:
var i *wifi.Interfacefor _, x := vary interfaces
for
// c.StationInfo(x) returns a slice of all
// the staton details about the interface
information, err := c.StationInfo(i)
if err != nil
fmt.Printf("Station err: %sn", err)
for _, x := vary information
time.Sleep(time.Second)
First, I identify a variable i
of sort *wifi.Interface
. Since it is exterior the loop, I can use it to retailer the interface data. Any variable created contained in the loop is inaccessible exterior the scope of that loop.
Then, I can break the loop into two. The first loop ranges over the interfaces returned by c.Interfaces()
, and if that interface is a Station sort, it shops that within the i
variable created earlier and breaks out of the loop.
The second loop is an infinite loop, so it will simply run time and again till I hit Ctrl+C to finish this system. This loop takes that interface data and retrieves the station data, as earlier than, and prints out the sign data. Then it sleeps for one second and runs once more, printing the sign data time and again till I stop.
So, working that:
[chris@marvin wifi-monitor]$ go run fundamental.go
Signal: -81
Signal: -81
Signal: -79
Signal: -81
Oof. Not good.
Mapping the condominium
This data is sweet to know, no less than. With an hooked up display screen or E Ink show and a battery (or a looooong extension cable), I can stroll the Pi across the condominium and map out the place the lifeless spots are.
Spoiler alert: With the owner’s entry level within the condominium subsequent door, the massive lifeless spot for me is a cone form emanating from the fridge within the studio condominium’s kitchen space… the fridge that shares a wall with the owner’s condominium!
I feel in Dungeons and Dragons lingo, this can be a “Cone of Silence.” Or no less than a “Cone of Poor Internet.”
Anyway, this code might be compiled instantly on the Raspberry Pi with go construct -o wifi_scanner
, and the ensuing binary, wifi_scanner
, might be shared with every other ARM units (of the identical model). Alternatively, it may be compiled on a daily system with the appropriate libraries for ARM units.
Happy Pi scanning! May your WiFi router not be behind your fridge! You can discover the code used for this undertaking in my GitHub repo.
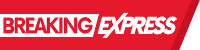