In C++, studying and writing to recordsdata will be completed by utilizing I/O streams together with the stream operators >>
and <<
. When studying or writing to recordsdata, these operators are utilized to an occasion of a category representing a file on the arduous drive. This stream-based method has an enormous benefit: From a C ++ perspective, it would not matter what you might be studying or writing to, whether or not it is a file, a database, the console, or one other PC you might be related to over the community. Therefore, realizing easy methods to write recordsdata utilizing stream operators will be transferred to different areas.
I/O stream courses
The C++ customary library supplies the category ios_base. This class acts as the bottom class for all I/O stream-compatible courses, reminiscent of basic_ofstream and basic_ifstream. This instance will use the specialised sorts for studying/writing characters, ifstream
and ofstream
.
ofstream
means output file stream, and it may be accessed with the insertion operator,<<
.ifstream
means enter file stream, and it may be accessed with the extraction operator,>>
.
Both sorts are outlined contained in the header <fstream>
.
A category that inherits from ios_base
will be regarded as a knowledge sink when writing to it or as a knowledge supply when studying from it, fully indifferent from the information itself. This object-oriented method makes ideas reminiscent of separation of concerns and dependency injection straightforward to implement.
A easy instance
This instance program is sort of easy: It creates an ofstream
, writes to it, creates an ifstream
, and reads from it:
#embody <iostream> // cout, cin, cerr and so on...
#embody <fstream> // ifstream, ofstream
#embody <string>int major()
std::string sFilename = "MyFile.txt";/******************************************
* *
* WRITING *
* *
******************************************/std::ofstream fileSink(sFilename); // Creates an output file stream
if (!fileSink)
/* std::endl will robotically append the right EOL */
fileSink << "Hello Open Source World!" << std::endl;/******************************************
* *
* READING *
* *
******************************************/
std::ifstream fileSource(sFilename); // Creates an enter file streamif (!fileSource)
elseexit(zero);
This code is accessible on GitHub. When you compile and execute it, you must get the next output:
This is a simplified, beginner-friendly instance. If you need to use this code in your personal software, please notice the next:
- The file streams are robotically closed on the finish of this system. If you need to proceed with the execution, you must shut them manually by calling the
shut()
technique. - These file stream courses inherit (over a number of ranges) from basic_ios, which overloads the
!
operator. This helps you to implement a easy examine if you happen to can entry the stream. On cppreference.com, yow will discover an summary of when this examine will (and will not) succeed, and you’ll implement additional error dealing with. - By default,
ifstream
stops at white house and skips it. To learn line by line till you attain EOF, use thegetline(...)
-method. - For studying and writing binary recordsdata, go the
std::ios::binary
flag to the constructor: This prevents EOL characters from being appended to every line.
Writing from the methods perspective
When writing recordsdata, the information is written to the system’s in-memory write buffer. When the system receives the system name sync, this buffer’s contents are written to the arduous drive. This mechanism can be the explanation you should not take away a USB stick with out telling the system. Usually, sync known as regularly by a daemon. If you actually need to be on the secure facet, you can too name sync manually:
#embody <unistd.h> // must be includedsync();
Summary
Reading and writing to recordsdata in C++ will not be that sophisticated. Moreover, if you understand how to cope with I/O streams, you additionally know (in precept) easy methods to cope with any type of I/O machine. Libraries for numerous sorts of I/O gadgets allow you to use stream operators for straightforward entry. This is why it’s helpful to understand how I/O steams work.
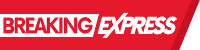