There are some ways to put in software program, however you get an possibility not out there elsewhere with open supply: You can compile the code your self. The traditional three-step course of to compile supply code:
$ ./configure
$ make
$ sudo make set up
Thanks to those instructions, you is perhaps shocked to search out that you just need not know easy methods to write and even learn code to compile it.
Install instructions to construct software program
As that is your first time compiling, there is a one-time preparatory step to put in the instructions for constructing software program. Specifically, you want a compiler. A compiler, resembling GCC or LLVM, turns supply code that appears like this:
#embody <iostream>utilizing namespace std;
int foremost() {
cout << "hello world";
}
Into machine language, the directions {that a} CPU makes use of to course of data. You can take a look at machine code, however it would not make any sense to you (except you are a CPU.)
You can get the GNU C compiler (GCC) and the LLVM compiler, together with different important instructions for compiling on Fedora, CentOS, Mageia, and related distributions, utilizing your package deal supervisor:
$ sudo dnf set up @improvement clang
On Debian, Elementary, Mint, and related distributions:
$ sudo apt set up build-essential clang
With your system arrange, there are a number of duties that you will repeat every time you need to compile your software program:
- Download the supply code
- Unarchive the supply code
- Compile
You have all of the instructions you want, so now you want some software program to compile.
1. Download supply code
Obtaining supply code for an software is very like getting any downloadable software program. You go to an internet site or a code administration web site like GitLab, SourceForge, or GitHub. Typically, open supply software program is accessible in each a work-in-progress (“current” or “nightly”) type in addition to a packaged “stable” launch model. Use the steady model when attainable except you’ve gotten purpose to consider in any other case or are ok with code to make things better after they break. The time period steady suggests the code bought examined and that the programmers of the applying really feel assured sufficient within the code to package deal it right into a .zip
or .tar
archive, give it an official quantity and generally a launch identify, and provide it for obtain to the overall non-programmer public.
For this train, I’m utilizing Angband, an open supply (GPLv2) ASCII dungeon crawler. It’s a easy software with simply sufficient issues to show what you could take into account when compiling software program for your self.
Download the supply code from the web site.
2. Unarchive the supply code
Source code is commonly delivered as an archive as a result of supply code often consists of a number of recordsdata. You need to extract it earlier than interacting with it, whether or not it is a tarball, a zipper file, a 7z file, or one thing else solely.
$ tar --extract --file Angband-x.y.z.tar.gz
Once you’ve got unarchived it, change the listing into the extracted listing and take a look round. There’s often a README
file on the high degree of the listing. This file, ideally, comprises steerage on what you could do to compile the code. The README
usually comprises data on these necessary facets of the code:
- Language: What language the code is in (as an example, C, C++, Rust, Python).
- Dependencies: What different software program you could have put in in your system for this software to construct and run.
- Instructions: The literal steps you could take to construct the software program. Occasionally, they embody this data inside a devoted file intuitively entitled
INSTALL
.
If the README
file would not comprise that data, take into account submitting a bug report with the developer. You’re not the one one who wants an introduction to supply code. Regardless of how skilled they’re, everyone seems to be new to supply code they’ve by no means seen earlier than, and documentation is necessary!
Angband’s maintainers hyperlink to on-line directions to explain easy methods to compile the code. This doc additionally describes what different software program you could have put in, though it would not precisely spell it out. The web site says, “There are several different front ends that you can optionally build (GCU, SDL, SDL2, and X11) using arguments to configure such as --enable-sdl
, --disable-x11
, etc.” This could imply one thing to you or seem like a international language, however that is the form of stuff you get used to after compiling code steadily. Whether or not you perceive what X11 or SDL2 is, they’re each necessities that you just see fairly usually after often compiling code over a number of months. You get snug with the concept that most software program wants different software program libraries as a result of they construct upon different applied sciences. In this case, although, Angband could be very versatile and compiles with or with out these non-obligatory dependencies, so for now, you may fake that there aren’t any further dependencies.
3. Compile the code
The canonical steps to construct code are:
$ ./configure
$ make
$ sudo make set up
Those are the steps for tasks constructed with Autotools, which is a framework created to standardize how supply code is delivered. Other frameworks (resembling Cmake) exist, nevertheless, and so they require completely different steps. When tasks stray from Autotools or Cmake, they have a tendency to warn you within the README
file.
Configure
Angband makes use of Autotools, so it is time to compile code!
In the Angband listing, first, run the configuration script included with the supply:
$ ./configure
This step scans your system to search out the dependencies that Angband requires to construct accurately. Some dependencies are so primary that your pc would not be working with out them, whereas others are specialised. At the tip of the method, the script provides you a report on what it has discovered:
[...]
configure: creating ./config.standing
config.standing: creating mk/buildsys.mk
config.standing: creating mk/additional.mk
config.standing: creating src/autoconf.hConfiguration:
Install path: /usr/native
binary path: /usr/native/video games
config path: /usr/native/and many others/angband/
lib path: /usr/native/share/angband/
doc path: /usr/native/share/doc/angband/
var path: (not used)
(save and rating recordsdata in ~/.angband/Angband/)-- Frontends --
- Curses Yes
- X11 Yes
- SDL2 Disabled
- SDL Disabled
- Windows Disabled
- Test No
- Stats No
- Spoilers Yes- SDL2 sound Disabled
- SDL sound Disabled
Some of that output could make sense to you, a few of it could not. Either approach, you in all probability discover that SDL2 and SDL are marked as Disabled
, and each Test and Stats are marked with No
. Although destructive, this is not essentially a nasty factor. This, primarily, is the distinction between a Warning and an Error. Had the configure script encountered one thing that might stop it from constructing the code, it might have alerted you with an error.
If you need to optimize your construct somewhat, you may select to resolve these destructive messages. By looking by means of the Angband documentation, you may resolve that Test and Stats aren’t truly of curiosity to you (they’re developer choices, particular to Angband). However, with somewhat on-line analysis, you may uncover that SDL2 can be a pleasant characteristic to have.
To resolve a dependency when compiling code, you could set up the lacking part and the improvement libraries for that lacking part. In different phrases, Angband wants SDL2 to play sound, however it wants SDL2-devel
(known as libsdl2-dev
, on Debian techniques) to construct. Install each along with your package deal supervisor:
$ sudo dnf set up sdl2 sdl2-devel
Try the configuration script once more:
$ ./configure --enable-sdl2
[...]
Configuration:
[...]
- Curses Yes
- X11 Yes
- SDL2 Yes
- SDL Disabled
- Windows Disabled
- Test No
- Stats No
- Spoilers Yes- SDL sound Disabled
- SDL2 sound Yes
Make
Once all the things’s configured, run the make
command:
$ make
This often takes some time, however it gives plenty of visible suggestions, so you may know code is getting compiled.
Install
The ultimate step is to put in the code you’ve got simply compiled. There’s nothing magical about putting in code. All that occurs is that plenty of recordsdata get copied to very particular directories. That’s true whether or not you are compiling from supply code or working a flowery graphical set up wizard. Because the code is getting copied to system-level directories, you have to have root (administrative) privileges, which get granted by the sudo
command.
$ sudo make set up
Run the applying
Once the applying will get put in, you may run it. According to the Angband documentation, the command to start out the sport is angband
, so attempt it out:
$ angband
Compiling code
I compile most of my very own purposes, whether or not on my Slackware desktop pc or my CentOS laptop computer utilizing NetBSD’s pkgsrc system. I discover that by compiling software program myself, I will be as specific as I need to be concerning the options included within the software, the way it’s configured, which library model it makes use of, and so forth. It’s rewarding, and it helps me preserve updated with new releases and, as a result of I generally discover bugs within the course of, it helps me become involved with plenty of completely different open supply tasks.
It’s uncommon that you don’t have any different possibility however to compile software program. Most open supply tasks present each the supply code (that is why it is known as “open source”) and installable packages. Compiling from supply code is a selection you get to make for your self, perhaps since you need new options not but out there within the newest launch or simply since you want to compile code your self.
Homework
Angband can use both Autotools or Cmake, so if you wish to expertise one other approach of constructing code, do that:
$ mkdir construct
$ cd construct
$ cmake ..
$ make
$ sudo make set up
You may attempt compiling with the LLVM compiler as a substitute of the GNU C compiler. For now, I’ll go away that as an train so that you can examine by yourself (trace: attempt setting the CC environment variable.)
Once you end exploring the supply code of Angband and at the very least a number of of its dungeons (you’ve got earned some downtime), take a look at another codebases. Many will use Autotools or Cmake, whereas others could use one thing completely different. See what you may construct!
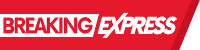