Rust is a comparatively new programming language, and it is already a preferred one winning over programmers from all industries. Still, it is also a language that builds on all the things that is come earlier than. Rust wasn’t made in a day, in any case, so although there are ideas in Rust that appear wildly completely different from what you might need discovered from Python, Java, C++, and so forth, all of them have a basis in the identical CPU and NUMA structure you’ve got all the time been (whether or not you recognize it or not) interacting with, and so a few of what’s new in Rust feels by some means acquainted.
Now, I’m not a programmer by commerce. I’m impatient but obsessive. If a language would not assist me get the outcomes I would like comparatively rapidly, I hardly ever discover myself impressed to make use of it after I have to get one thing achieved. Rust tries to convey into steadiness two conflicting issues: The trendy laptop’s want for safe and structured code, and the fashionable programmer’s want to do much less work whereas attaining extra success.
Install Rust
The rust-lang.org web site has nice documentation on putting in Rust, however normally, it is so simple as downloading the sh.rustup.rs
script and working it.
$ curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs$ much less sh.rustup.sh
$ sh ./sh.rustup.rs
No courses
Rust would not have courses and doesn’t use the class
key phrase. Rust does have the struct
knowledge kind, nonetheless, its goal is to function a type of template for a set of information. So as an alternative of making a category to characterize a digital object, you should utilize a struct:
struct Penguin {
genus: String,
species: String,
extinct: bool,
categorized: u64,
}
You can use this much like how a category is used. For occasion, as soon as a Penguin
struct is outlined, you’ll be able to create cases of it, and work together with that occasion:
struct Penguin {
genus: String,
species: String,
extinct: bool,
categorized: u64,
}fn major() {
let p = Penguin { genus: "Pygoscelis".to_owned(),
species: "R adeliæ".to_owned(),
extinct: false,
categorized: 1841 };println!("Species: {}", p.species);
println!("Genus: {}", p.genus);
println!("Classified in {}", p.categorized);
if p.extinct == true {
println!("Sadly this penguin has been made extinct.");
}
}
Using the impl
knowledge kind at the side of the struct
knowledge kind, you’ll be able to implement a struct containing features, and you’ll add inheritance and different class-like options.
Functions
Functions in Rust are loads like features in different languages. Each one represents a discreet set of duties you can name upon when wanted. The main operate should be referred to as major
.
Functions are declared utilizing the fn
key phrase, adopted by the operate’s title and any parameters the operate accepts.
fn foo() {
let n = 8;
println!("Eight is written as {}", n);
}
Passing info from one operate to a different will get achieved with parameters. For occasion, I’ve already created a Penguin
class, and I’ve bought an occasion of a penguin as p
, so passing the attributes of p
from one operate to a different requires me to specify p
as an accepted Penguin
kind for its vacation spot operate.
fn major() {
let p = Penguin { genus: "Pygoscelis".to_owned(),
species: "R adeliæ".to_owned(),
extinct: false, categorized: 1841 };
printer(p);
}fn printer(p: Penguin) {
println!("Species: {}", p.species);
println!("Genus: {}", p.genus);
println!("Classified in {}", p.categorized);
if p.extinct == true {
println!("Sadly this penguin has been made extinct.");
}
}
Variables
Rust creates immutable variables by default. That implies that a variable you create can’t be modified later. This code, humble although it might be, can’t be compiled:
fn major() {
let n = 6;
let n = 5;
}
However, you’ll be able to declare a mutable variable with the key phrase mut
, so this code compiles efficiently:
fn major() {
let mut n = 6;
println!("Value is {}", n);
n = 5;
println!("Value is {}", n);
}
Compiler
The Rust compiler, not less than when it comes to its error messages, is without doubt one of the nicest compilers accessible. When you get one thing improper in Rust, the compiler makes a honest effort to inform you what you probably did improper. I’ve really discovered many nuances of Rust (insofar as I perceive any nuance of Rust) simply by studying from compiler error messages. Even when an error message is simply too obscure to be taught from immediately, it is virtually all the time sufficient for an web search to clarify.
The best option to begin a Rust program is to make use of cargo
, the Rust bundle administration and construct system.
$ mkdir myproject
$ cd myproject
$ cargo init
This creates the fundamental infrastructure for a challenge, most notably a major.rs
file within the src
subdirectory. Open this file and paste within the instance code I’ve generated for this text:
struct Penguin {
genus: String,
species: String,
extinct: bool,
categorized: u64,
}fn major() {
let p = Penguin { genus: "Pygoscelis".to_owned(), species: "R adeliæ".to_owned(), extinct: false, categorized: 1841 };
printer(p);
foo();
}fn printer(p: Penguin) {
println!("Species: {}", p.species);
println!("Genus: {}", p.genus);
println!("Classified in {}", p.categorized);
if p.extinct == true {
println!("Sadly this penguin has been made extinct.");
}
}fn foo() {
let mut n = 6;
println!("Value is {}", n);
n = 8;
println!("Eight is written as {}", n);
}
To compile, use the cargo construct
command:
$ cargo construct
To run your challenge, execute the binary within the goal
subdirectory, or else simply use cargo run
:
$ cargo run
Species: R adeliæ
Genus: Pygoscelis
Classified in 1841
Value is 6
Eight is written as 8
Crates
Much of the comfort of any language comes from its libraries or modules. In Rust, libraries get distributed and tracked as “crates”. The crates.io web site is an effective registry of group crates.
To add a crate to your Rust challenge, listing them within the Cargo.toml
file. For occasion, to put in a random quantity operate, I take advantage of the rand
crate, with *
serving as a wildcard to make sure that I get the most recent model at compile time:
[package]
title = "myproject"
model = "0.1.0"
authors = ["Seth <[email protected]>"]
version = "2022"[dependencies]
rand = "*"
Using it in Rust code requires a use
assertion on the prime:
use rand::Rng;
Some pattern code that creates a random seed after which a random vary:
fn foo() {
let mut rng = rand::thread_rng();
let mut n = rng.gen_range(1..99);println!("Value is {}", n);
n = rng.gen_range(1..99);
println!("Value is {}", n);
}
You can use cargo run
to run it, which detects the code change and triggers a brand new construct. The construct course of downloads the rand
crate and all of the crates that it, in flip, relies upon upon, compiles the code, after which runs it:
$ cargo run
Updating crates.io index
Downloaded ppv-lite86 v0.2.16
Downloaded 1 crate (22.2 KB) in 1.40s
Compiling libc v0.2.112
Compiling cfg-if v1.0.0
Compiling ppv-lite86 v0.2.16
Compiling getrandom v0.2.3
Compiling rand_core v0.6.3
Compiling rand_chacha v0.3.1
Compiling rand v0.8.4
Compiling rustpenguin v0.1.0 (/dwelling/sek/Demo/rustpenguin)
Finished dev [unoptimized + debuginfo] goal(s) in 13.97s
Running `goal/debug/rustpenguin`Species: R adeliæ
Genus: Pygoscelis
Classified in 1841
Value is 70
Value is 35
Rust cheat sheet
Rust is a supremely nice language. Thanks to its integration with on-line registries, its useful compiler, and its virtually intuitive syntax, it feels appropriately trendy.
Make no mistake, although, it is also a fancy language, with strict knowledge varieties, strongly scoped variables, and plenty of built-in strategies. Rust is price taking a look at, and if you are going to discover Rust, then you must obtain our free Rust cheat sheet, so you might have a fast reference for the fundamentals. The sooner you get began, the earlier you may know Rust. And, after all, you must apply usually to keep away from getting rusty.
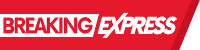