Container platforms and edge computing proceed to develop, powering main networks and purposes throughout the globe, and Java applied sciences have developed new options and improved efficiency to match steps with fashionable infrastructure. Java 17 (OpenJDK 17) was launched just lately (September 2021) with the next main options:
Developers are questioning the best way to begin implementing software logic utilizing the brand new options of Java 17 after which construct and run them on the identical OpenJDK 17 runtime. Luckily, Quarkus allows the builders to scaffold a brand new software with Java 17. It additionally gives a live coding functionality that enables builders to focus solely on implementing enterprise logic as a substitute of compiling, constructing, deploying, and restarting runtimes to use code modifications.
Note: If you haven’t already put in OpenJDK 17, download a binary in your working system.
This tutorial teaches you to make use of the Pseudo-Random Number Generators (PRNG) algorithms of Java 17 on Quarkus. Get began by scaffolding a brand new challenge utilizing the Quarkus command-line tool (CLI):
$ quarkus create app prng-example --java=17
The output seems like this:
...
[SUCCESS] ✅ quarkus challenge has been efficiently generated in:
--> /Users/danieloh/quarkus-demo/prng-instance
...
Unlike conventional Java frameworks, Quarkus gives stay coding options for builders to rebuild and deploy whereas the code modifications. In the top, this functionality accelerates internal loop improvement for Java builders. Run your Quarkus software utilizing Dev mode:
$ cd prng-example
$ quarkus dev
The output seems like this:
...
INFO [io.quarkus] (Quarkus Main Thread) Profile dev activated. Live Coding activated.
INFO [io.quarkus] (Quarkus Main Thread) Installed options: [cdi, resteasy, smallrye-context-propagation, vertx]--
Tests paused
Press [r] to renew testing, [o] Toggle take a look at output, [:] for the terminal, [h] for extra choices>
Java 17 allows builders to generate random integers inside a particular vary primarily based on the Xoshiro256PlusPlus PRNG algorithm. Add the next code to the hiya()
technique within the src/predominant/java/org/acme
listing:
RandomGenerator randomGenerator = RandomGeneratorFactory.of("Xoshiro256PlusPlus").create(999);for ( int i = 0; i < 10 ; i++) {
int end result = randomGenerator.subsequentInt(11);
System.out.println(end result);
}
Next, invoke the RESTful API (/hiya
) to substantiate that random integers are generated. Execute the next cURL command line in your native terminal or entry the endpoint URL utilizing an online browser:
$ curl localhost:8080/hiya
Go again to the terminal the place you’re operating Quarkus Dev mode. There you’ll see the next ten random numbers:
Note: You don’t have to rebuild code and restart the Java runtime in any respect. You’ll additionally see the output Hello RESTEasy within the terminal the place you ran the curl
command line.
Wrap up
This article exhibits how Quarkus permits builders to begin a brand new software improvement primarily based on OpenJDK 17. Furthermore, Quarkus will increase builders’ productiveness by stay coding. For a manufacturing deployment, builders could make a native executable primarily based on OpenJDK 17 and GraalVM.
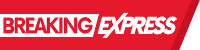