One of essentially the most gratifying components of studying a brand new programming language is lastly operating an executable and getting the specified output. When I found the programming language Go, I began by studying some pattern applications to get acquainted with the syntax, then wrote small check applications. Over time, this strategy helped me get accustomed to compiling and constructing this system.
The construct choices accessible for Go present methods to achieve extra management over the construct course of. They may also present further data to assist break the method into smaller components. In this text, I’ll display among the choices I’ve used. Note: I’m utilizing the phrases construct and compile to imply the identical factor.
Getting began with Go
I’m utilizing Go model 1.16.7; nonetheless, the command given right here ought to work on most up-to-date variations as nicely. If you would not have Go put in, you’ll be able to obtain it from the Go website and observe the directions for set up. Verify the model you could have put in by opening a immediate command and typing:
$ go model
The response ought to seem like this, relying in your model.
go model go1.16.7 linux/amd64
$
Basic compilation and execution of Go applications
I’ll begin with a pattern Go program that merely prints “Hello World” to the display screen.
$ cat hi there.go
bundle foremostimport "fmt"
func foremost() {
fmt.Println("Hello World")
}
$
Before discussing extra superior choices, I’ll clarify how you can compile a pattern Go program. I make use of the construct
choice adopted by the Go program supply file identify, which on this case is hi there.go
.
$ go construct hi there.go
If all the pieces is working appropriately, it is best to see an executable named hi there
created in your present listing. You can confirm that it’s in ELF binary executable format (on the Linux platform) through the use of the file command. You may also execute it and see that it outputs “Hello World.”
$ ls
hi there hi there.go
$
$ file ./hi there
./hi there: ELF 64-bit LSB executable, x86-64, model 1 (SYSV), statically linked, not stripped
$
$ ./hi there
Hello World
$
Go supplies a helpful run
choice in case you don’t want to have a ensuing binary and as a substitute wish to see if this system works appropriately and prints the specified output. Keep in thoughts that even when you don’t see the executable in your present listing, Go nonetheless compiles and produces the executable someplace, runs it, then removes it from the system. I’ll clarify in a later part of this text.
$ go run hi there.go
Hello World
$
$ ls
hi there.go
$
Under the hood
The above instructions labored like a breeze to run my program with minimal effort. However, if you wish to discover out what Go does beneath the hood to compile these applications, Go supplies a -x
choice that prints all the pieces Go does to provide the executable.
A fast look tells you that Go creates a brief working listing inside /tmp
, produces the executable, after which strikes it to the present listing the place the supply Go program was current.
$ go construct -x hi there.goWORK=/tmp/go-build1944767317
mkdir -p $WORK/b001/<< snip >>
mkdir -p $WORK/b001/exe/
cd .
/usr/lib/golang/pkg/instrument/linux_amd64/hyperlink -o $WORK
/b001/exe/a.out -importcfg $WORK/b001
/importcfg.hyperlink -buildmode=exe -buildid=K26hEYzgDkqJjx2Hf-wz/
nDueg0kBjIygx25rYwbK/W-eJaGIOdPEWgwC6o546
/K26hEYzgDkqJjx2Hf-wz -extld=gcc /root/.cache/go-build /cc
/cc72cb2f4fbb61229885fc434995964a7a4d6e10692a23cc0ada6707c5d3435b-d
/usr/lib/golang/pkg/instrument/linux_amd64/buildid -w $WORK
/b001/exe/a.out # inner
mv $WORK/b001/exe/a.out hi there
rm -r $WORK/b001/
This helps remedy the mysteries when a program runs however no ensuing executable is created throughout the present listing. Using -x
exhibits that the executable file was certainly created in a /tmp
working listing and was executed. However, not like the construct
choice, the executable didn’t transfer to the present listing, making it seem that no executable was created.
$ go run -x hi there.gomkdir -p $WORK/b001/exe/
cd .
/usr/lib/golang/pkg/instrument/linux_amd64/hyperlink -o $WORK/b001
/exe/hi there -importcfg $WORK/b001/importcfg.hyperlink -s -w -buildmode=exe -buildid=hK3wnAP20DapUDeuvAAS/E_TzkbzwXz6tM5dEC8Mx
/7HYBzuaDGVdaZwSMEWAa/hK3wnAP20DapUDeuvAAS -extld=gcc
/root/.cache/go-build/75/
7531fcf5e48444eed677bfc5cda1276a52b73c62ebac3aa99da3c4094fa57dc3-d
$WORK/b001/exe/hi there
Hello World
Mimic compilation with out producing the executable
Suppose you do not wish to compile this system and produce an precise binary, however you do wish to see all steps within the course of. You can achieve this through the use of the -n
construct choice, which prints the steps that it could usually run with out truly creating the binary.
$ go construct -n hi there.go
Save temp directories
A variety of work occurs within the /tmp
working listing, which is deleted as soon as the executable is created and run. But what if you wish to see which recordsdata have been created within the compilation course of? Go supplies a -work
choice that can be utilized when compiling a program. The -work
choice prints the working listing path along with operating this system, but it surely would not delete the working listing afterward, so you’ll be able to transfer to that listing and study all of the recordsdata created through the compile course of.
$ go run -work hi there.go
WORK=/tmp/go-build3209320645
Hello World
$
$ discover /tmp/go-build3209320645
/tmp/go-build3209320645
/tmp/go-build3209320645/b001
/tmp/go-build3209320645/b001/importcfg.hyperlink
/tmp/go-build3209320645/b001/exe
/tmp/go-build3209320645/b001/exe/hi there
$
$ /tmp/go-build3209320645/b001/exe/hi there
Hello World
$
Alternative compilation choices
What if, as a substitute of utilizing the construct/run magic of Go, you wish to compile this system by hand and find yourself with an executable that may be run immediately by your working system (on this case, Linux)? This course of might be divided into two components: compile and hyperlink. Use the instrument
choice to see the way it works.
First, use the instrument compile
choice to provide the ensuing ar
archive file, which accommodates the .o
intermediate file. Next, use the instrument hyperlink
choice on this hi there.o
file to provide the ultimate executable, which may then run.
$ go instrument compile hi there.go
$
$ file hi there.o
hi there.o: present ar archive
$
$ ar t hi there.o
__.PKGDEF
_go_.o
$
$ go instrument hyperlink -o hi there hi there.o
$
$ file hi there
hi there: ELF 64-bit LSB executable, x86-64, model 1 (SYSV), statically linked, not stripped
$
$ ./hi there
Hello World
$
To peek additional into the hyperlink course of of manufacturing the executable from the hi there.o
file, you need to use the -v
choice, which searches for the runtime.a
file included in each Go executable.
$ go instrument hyperlink -v -o hi there hi there.o
HEADER = -H5 -T0x401000 -R0x1000
looking for runtime.a in /usr/lib/golang/pkg/linux_amd64/runtime.a
82052 symbols, 18774 reachable
1 bundle symbols, 1106 hashed symbols, 77185 non-package symbols, 3760 exterior symbols
81968 liveness information
$
Cross-compilation choices
Now that I’ve defined the compilation of a Go program, I’ll display how Go means that you can construct an executable focused at totally different {hardware} architectures and working programs by offering two setting variables—GOOS and GOARCH—earlier than the precise construct
command.
Why does this matter? You can see an instance when an executable produced for the ARM (aarch64) structure will not run on an Intel (x86_64) structure and produces an Exec format error.
These choices make it trivial to provide cross-platform binaries.
$ GOOS=linux GOARCH=arm64 go construct hi there.go
$
$ file ./hi there
./hi there: ELF 64-bit LSB executable, ARM aarch64, model 1 (SYSV), statically linked, not stripped
$$ ./hi there
bash: ./hi there: can not execute binary file: Exec format error
$
$ uname -m
x86_64
$
You can learn my earlier weblog submit about my experiences with cross-compilation using Go to be taught extra.
View underlying meeting directions
The supply code will not be immediately transformed to an executable, although it generates an intermediate meeting format which is then assembled into an executable. In Go, that is mapped to an intermediate meeting format somewhat than the underlying {hardware} meeting directions.
To view this intermediate meeting format, use -gcflags
adopted by -S
given to the construct command. This command exhibits the meeting directions.
$ go construct -gcflags="-S" hi there.go
# command-line-arguments
"".foremost STEXT measurement=138 args=0x0 locals=0x58 funcid=0x0
0x0000 00000 (/check/hi there.go:5) TEXT "".foremost(SB), ABIInternal, $88-0
0x0000 00000 (/check/hi there.go:5) MOVQ (TLS), CX
0x0009 00009 (/check/hi there.go:5) CMPQ SP, 16(CX)
0x000d 00013 (/check/hi there.go:5) PCDATA $0, $-2
0x000d 00013 (/check/hi there.go:5) JLS 128<< snip >>
$
You may also use the objdump -s
choice, as proven under, to see the meeting directions for an executable program that was already compiled.
$ ls
hi there hi there.go
$
$
$ go instrument objdump -s foremost.foremost hi there
TEXT foremost.foremost(SB) /check/hi there.go
hi there.go:5 0x4975a0 64488b0c25f8ffffff MOVQ FS:0xfffffff8, CX
hi there.go:5 0x4975a9 483b6110 CMPQ 0x10(CX), SP
hi there.go:5 0x4975ad 7671 JBE 0x497620
hi there.go:5 0x4975af 4883ec58 SUBQ $0x58, SP
hi there.go:6 0x4975d8 4889442448 MOVQ AX, 0x48(SP)<< snip >>
$
Strip binaries to cut back their measurement
Go binaries are usually massive. For instance, a easy Hello World program produces a 1.9M-sized binary.
$ go construct hi there.go
$
$ du -sh hi there
1.9M hi there
$
$ file hi there
hi there: ELF 64-bit LSB executable, x86-64, model 1 (SYSV), statically linked, not stripped
$
To scale back the dimensions of the ensuing binary, you’ll be able to strip off data not wanted throughout execution. Using -ldflags
adopted by -s -w
flags makes the ensuing binary barely lighter, at 1.3M.
$ go construct -ldflags="-s -w" hi there.go
$
$ du -sh hi there
1.3M hi there
$
$ file hi there
hi there: ELF 64-bit LSB executable, x86-64, model 1 (SYSV), statically linked, stripped
$
Conclusion
I hope this text launched you to some helpful Go construct choices that may aid you perceive the Go compilation course of higher. For further data on the construct course of and different fascinating choices accessible, discuss with the assistance part:
$ go assist construct
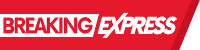