The dependency administration instrument Composer gives a number of methods to incorporate Git repositories inside a PHP: Hypertext Preprocessor (PHP) venture.
In many instances, repositories have been created on Packagist, so requiring them with Composer could be very easy. But what do you do when a repository has not been created as a package deal on Packagist? You use Composer to require the package deal straight from the repository. This article explains how.
Note: Some of the terminology on this submit is complicated as a result of a number of phrases are used to explain various things. Here is a fast vocabulary record that can assist:
- Project: The customized software program you might be constructing. This could be a web site, a command-line utility, an utility, or the rest you dream up.
- Package: Any third-party software program you wish to obtain and use inside your venture. It could be a library, Drupal theme, WordPress plugin, or some other variety of issues.
- Git repository: Also referred to as the Git repo, that is the version-control host for a package deal. Common hosts embrace GitHub, GitLab, or Bitbucket, however any URL-accessible Git repository will work for this tutorial.
- Composer repositories: In a
composer.json
file, there’s an non-obligatory property named “repositories.” This property is the place you may outline new locations for Composer to look when downloading packages.
When including a Git repo to your venture with Composer, you’ll find your self in two conditions: Either the repo accommodates a composer.json
file, which defines how the repo ought to be dealt with when required, or it doesn’t. You can add the Git repository to your venture in each instances, with completely different strategies.
Git repo with composer.json
When a repository features a composer.json
file, it defines facets of itself which can be vital to how Composer manages the package deal. Here is an instance of a easy composer.json
file a package deal might embrace:
{
"name": "mynamespace/my-custom-library",
"type": "library"
}
This instance reveals two vital properties {that a} composer.json
file can outline:
- Name: The package deal’s namespaced title. In this case, “mynamespace” is the namespace for the package deal “my-custom-library.”
- Type: The kind of package deal the repo represents. Package sorts are used for set up logic. Out of the field, Composer permits for the next package deal sorts: library, venture, metapackage, and composer-plugin.
You can confirm this by wanting on the composer.json
file of any fashionable GitHub venture. They every outline their package deal title and the package deal kind close to the highest of the file. When a repository has this data outlined in its composer.json
file, requiring the repository inside your venture is sort of easy.
Require a Git repository that has a composer.json file
After you’ve got recognized a Git repository with a composer.json
file, you may require that repository as a package deal inside your venture.
Within your venture’s composer.json
file, you must outline a brand new property (assuming it does not exist already) named “repositories.” The worth of the repositories property is an array of objects, every containing details about the repository you wish to embrace in your venture. Consider this composer.json
file on your customized venture.
{
"name": "mynamespace/my-project-that-uses-composer",
"repositories": [
{
"type": "vcs",
"url": "https://github.com/mynamespace/my-custom-library.git"
}
],
"require": {
"mynamespace/my-custom-library": "dev-master"
}
}
You are doing two vital issues right here. First, you are defining a brand new repository that Composer can reference when requiring packages. And second, you are requiring the package deal from the newly outlined repository.
Note: The package deal model is part of the require assertion and never part of the repository property. You can require a selected department of a repo by selecting a model named “dev-<branch name>”.
If you have been to run composer set up
within the context of this file, Composer would search for a venture on the outlined URL. If that URL represents a Git repo that accommodates a composer.json
file that defines its title and sort, Composer will obtain that package deal to your venture and place it within the applicable location.
Custom package deal sorts
The instance package deal proven above is of the kind “library,” however with WordPress and Drupal you are coping with plugins, modules, and themes. When requiring particular package deal sorts in your venture, it is vital to put in them in particular areas inside the venture file construction. Wouldn’t it’s good in case you might persuade Composer to deal with some of these packages as particular instances? Well, you are in luck. There is an official Composer plugin that can do this for you.
The Installers plugin for Composer accommodates the customized logic required for dealing with many various package deal sorts for a big number of initiatives. It is extraordinarily useful when working with initiatives which have well-known and supported package deal set up steps.
This venture means that you can outline package deal sorts like drupal-theme, drupal-module, wordpress-plugin, wordpress-theme, and lots of extra, for a wide range of initiatives. In the case of a drupal-theme package deal, the Installers plugin will place the required repo inside the /themes/contrib
folder of your Drupal set up.
Here is an instance of a composer.json
file which may reside inside a Drupal theme venture as its personal Git repository:
{
"name": "mynamespace/whatever-i-call-my-theme",
"type": "drupal-theme",
"description": "Drupal 8 theme",
"license": "GPL-2.0+"
}
Note that the one significant distinction right here is that the kind is now drupal-theme. With the drupal-theme kind outlined, any venture that makes use of the Installers plugin can simply require your repo in its Drupal venture, and it is going to be handled as a contributed theme.
Require any Git repository with Composer
What occurs when the repo you wish to embrace in your venture doesn’t outline something about itself with a composer.json
file? When a repo doesn’t outline its title or kind, you need to outline that data for the repo inside your venture’s composer.json
file. Take a take a look at this instance:
{
"name": "mynamespace/my-project-that-uses-composer",
"repositories": [
{
"type": "package",
"package": {
"name": "mynamespace/my-custom-theme",
"version": "1.2.3",
"type": "drupal-theme",
"source": {
"url": "https://github.com/mynamespace/my-custom-theme.git",
"type": "git",
"reference": "master"
}
}
}
],
"require": {
"mynamespace/my-custom-theme": "^1",
"composer/installers": "^1"
}
}
Notice that your repository kind is now “package.” That is the place you’ll outline all the things in regards to the package deal you wish to require.
Create a brand new object named “package” the place you outline all of the important data that Composer must know to have the ability to embrace this arbitrary git repo inside your venture, together with:
- Name: The namespaced package deal title. It ought to most likely match the repository you are requiring however does not must.
- Type: The kind of package deal. This displays the way you need Composer to deal with this repository.
- Version: A model quantity for the repo. You might want to make this up.
- Source: An object that accommodates the next repository data:
- URL: The Git or different version-control system (VCS) URL the place the package deal repo may be discovered
- Type: The VCS kind for the package deal, resembling git, svn, cvs, and so forth
- Reference: The department or tag you wish to obtain
I like to recommend reviewing the official documentation on Composer package deal repositories. Note that it’s potential to incorporate zip information as Composer packages as effectively. Essentially, you are actually accountable for all elements of how Composer treats this repository. Since the repository itself will not be offering Composer with any data, you might be accountable for figuring out virtually all the things, together with the present model quantity for the package deal.
This strategy means that you can embrace virtually something as a Composer package deal in your venture, but it surely has some notable drawbacks:
- Composer is not going to replace the package deal until you alter the
model
discipline.
- Composer is not going to replace the commit references. If you utilize
grasp
as a reference, you’ll have to delete the package deal to power an replace, and you’ll have to cope with an unstable lock file.
Custom package deal variations
Maintaining the package deal model in your composer.json
file is not at all times obligatory. Composer is wise sufficient to search for GitHub releases and use them because the package deal variations. But ultimately you’ll possible wish to embrace a easy venture that has only some branches and no official releases.
When a repository doesn’t have releases, you’ll be accountable for deciding what model the repository department represents to your venture. In different phrases, if you’d like composer to replace the package deal, you will have to increment the “model” outlined in your venture’s composer.json
file earlier than operating composer replace
.
Overriding a Git repository’s composer.json
When defining a brand new Composer repository of the kind package deal, you may override a package deal’s personal composer.json
definitions. Consider a Git repository that defines itself as a library in its composer.json
, however you recognize that the code is definitely a drupal-theme. You can use the above strategy to incorporate the Git repository inside your venture as a drupal-theme, permitting Composer to deal with the code appropriately when required.
Example: Require Guzzle as a drupal-theme simply to show that you could.
{
"name": "mynamespace/my-project-that-uses-composer",
"repositories": [
{
"type": "package",
"package": {
"name": "mynamespace/guzzle-theme",
"version": "1.2.3",
"type": "drupal-theme",
"source": {
"url": "https://github.com/guzzle/guzzle.git",
"type": "git",
"reference": "master"
}
}
}
],
"require": {
"mynamespace/guzzle-theme": "^1",
"composer/installers": "^1"
}
}
This works! You’ve downloaded the Guzzle library and positioned it inside the /themes
folder of your Drupal venture. This will not be a really sensible instance, but it surely highlights how a lot management the package deal kind strategy gives.
Summary
Composer affords loads of choices for together with arbitrary packages inside a venture. Determining how these packages are included within the venture primarily comes right down to who defines the package deal data. If the Git repository features a composer.json
file that defines its title and sort, you may have Composer depend on the repository itself for the definition.
But if you wish to embrace a repository that doesn’t outline its title and sort, then it’s as much as your venture to outline and keep that data on your personal inside use. Alternatively, if a repository does not outline a composer.json
file, take into account submitting a pull request that provides it.
This article initially appeared on the Daggerhart Lab blog and is republished with permission.
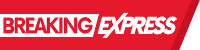