Many organizations have safety insurance policies in place that dictate how you can retailer delicate info. When you are creating purposes for the cloud, you are in all probability anticipated to observe these insurance policies, and to try this you typically must externalize your information storage. Kubernetes has a built-in system to entry exterior secrets and techniques, and studying to make use of that’s key to a secure cloud-native app.
In this text, I’m going to reveal how you can construct a Quarkus reactive utility with externalized delicate info—as an illustration, a password or token—utilizing Kubernetes Secrets. A secret is an efficient instance of how cloud platforms can safe purposes by eradicating delicate information out of your static code. Note that you’ll find an answer to this tutorial in this GitHub repository.
[ Read next: How to explain Kubernetes Secrets ]
1. Scaffold a brand new reactive Quarkus challenge
Use the Quarkus command-line interface (CLI) to scaffold a brand new challenge. If you have not already put in the Quarkus CLI, observe these instructions in response to your working system.
Run the next Quarkus CLI in your challenge listing so as to add kubernetes-config
, resteasy-reactive
, and openshift
extensions:
$ quarkus create app quarkus-secret-example
-x resteasy-reactive,kubernetes-config,openshift
The output ought to appear like this:
Looking for the newly revealed extensions in registry.quarkus.io
chosen extensions:
- io.quarkus:quarkus-kubernetes-config
- io.quarkus:quarkus-resteasy-reactive
- io.quarkus:quarkus-openshiftmaking use of codestarts...
? java
? maven
? quarkus
? config-properties
? dockerfiles
? maven-wrapper
? resteasy-reactive-codestart-----------
[SUCCESS] ✅ quarkus challenge has been efficiently generated in:
--> /tmp/quarkus-secret-example
-----------
Navigate into this listing and get began: quarkus dev
2. Create a Secret in Kubernetes
To handle the Kubernetes Secrets, you have got three choices:
- Using kubectl
- Using Configuration File
- Node Kustomize
Use the kubectl
command to create a brand new database credential (a username and password). Run the next command:
$ kubectl create secret generic db-credentials
--from-literal=username=admin
--from-literal=password=secret
If you have not already put in a Kubernetes cluster domestically, or you haven’t any distant cluster, you may check in to the developer sandbox, a no-cost sandbox surroundings for Red Hat OpenShift and CodeReady Workspaces.
You can affirm that the Secret is created correctly by utilizing the next command:
$ kubectl get secret/db-credentials -o yaml
The output ought to appear like this:
apiVersion: v1
information:
password: c2VjcmV0
username: YWRtaW4=
sort: Secret
metadata:
creationTimestamp: "2022-05-02T13:46:18Z"
identify: db-credentials
namespace: doh-dev
resourceVersion: "1190920736"
uid: 936abd44-1097-4c1f-a9d8-8008a01c0add
kind: Opaque
The username and password are encoded by default.
3. Create a brand new RESTful API to entry the Secret
Now you may add a brand new RESTful (for Representational State Transfer) API to print out the username and password saved within the Kubernetes Secret. Quarkus allows builders to discuss with the key as a standard configuration utilizing a @ConfigureProperty
annotation.
Open a GreetingResource.java
file in src/most important/java/org/acme
. Then, add the next technique and configurations:
@ConfigProperty(identify = "username")
String username;@ConfigProperty(identify = "password")
String password;@GET
@Produces(MediaType.TEXT_PLAIN)
@Path("/securty")
public Map securty() {
HashMap<String, String> map = new HashMap<>();
map.put("db.username", username);
map.put("db.password", password);
return map;
}
Save the file.
4. Set the configurations for Kubernetes deployment
Open the utility.properties
file within the src/most important/assets
listing. Add the next configuration for the Kubernetes deployment. In the tutorial, I’ll reveal utilizing the developer sandbox, so the configurations tie to the OpenShift cluster.
If you wish to deploy it to the Kubernetes cluster, you may bundle the appliance by way of Docker container straight. Then, you could push the container picture to an exterior container registry (for instance, Docker Hub, quay.io, or Google container registry).
# Kubernetes Deployment
quarkus.kubernetes.deploy=true
quarkus.kubernetes.deployment-target=openshift
openshift.expose=true
quarkus.openshift.build-strategy=docker
quarkus.kubernetes-client.trust-certs=true# Kubernetes Secret
quarkus.kubernetes-config.secrets and techniques.enabled=true
quarkus.kubernetes-config.secrets and techniques=db-credentials
Save the file.
5. Build and deploy the appliance to Kubernetes
To construct and deploy the reactive utility, you can even use the next Quarkus CLI:
$ quarkus construct
This command triggers the appliance construct to generate a fast-jar
file. Then the appliance Jar
file is containerized utilizing a Dockerfile, which was already generated within the src/most important/docker
listing once you created the challenge. Finally, the appliance picture is pushed into the built-in container registry contained in the OpenShift cluster.
The output ought to finish with a BUILD SUCCESS
message.
When you deploy the appliance to the developer sandbox or regular OpenShift cluster, you’ll find the appliance within the Topology view within the Developer perspective, as proven within the determine under.
(Daniel Oh, CC BY-SA 4.0)
6. Verify the delicate info
To confirm that your Quarkus utility can discuss with the delicate info from the Kubernetes Secret, get the route URL utilizing the next kubectl
command:
$ kubectl get route
The output is much like this:
NAME HOST/PORT PATH SERVICES PORT TERMINATION WILDCARD
quarkus-secret-example quarkus-secret-example-doh-dev.apps.sandbox.x8i5.p1.openshiftapps.com quarkus-secret-example 8080 None
Use the curl
command to entry the RESTful API:
$ curl http://YOUR_ROUTE_URL/good day/safety
The output:
{db.password=secret, db.username=admin}
Awesome! The above username
and password
are the identical as these you saved within the db-credentials
secret.
Where to study extra
This information has proven how Quarkus allows builders to externalize delicate info utilizing Kubernetes Secrets. Find extra assets to develop cloud-native microservices utilizing Quarkus on Kubernetes right here:
You may watch this step-by-step tutorial video on how you can handle Kubernetes Secrets with Quarkus.
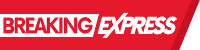