Compiling software program is one thing that builders do rather a lot, and in open supply some customers even select to do it themselves. Linux podcaster Dann Washko calls supply code the “universal package format” as a result of it accommodates all of the parts essential to make an utility run on any platform. Of course, not all supply code is written for all programs, so it is solely “universal” throughout the subset of focused programs, however the level is that supply code is extraordinarily versatile. With open supply, you possibly can resolve how code is compiled and run.
When you are compiling code, you are normally coping with a number of supply information. Developers are inclined to preserve totally different courses or modules in separate information in order that they are often maintained individually, and presumably even utilized by totally different tasks. But while you’re compiling these information, lots of them get compiled right into a single executable.
This is normally performed by creating shared libraries, after which dynamically linking again to them from the executable. This retains the executable small by conserving modular features exterior, and ensures that libraries may be up to date independently of the purposes that use them.
Locating a shared object throughout compilation
When you are compiling with GCC, you normally want a library to be put in in your workstation for GCC to have the ability to find it. By default, GCC assumes that libraries are in a system library path, resembling /lib64
and /usr/lib64
. However, in the event you’re linking to a library of your individual that is not but put in, or if you might want to hyperlink to a library that is not put in in a normal location, then you need to assist GCC discover the information.
There are two choices vital for locating libraries in GCC:
-L
(capital L) provides an extra library path to GCC’s search areas.-l
(lowercase L) units the identify of the library you need to hyperlink towards.
For instance, suppose you have written a library known as libexample.so
, and also you need to use it when compiling your utility demo.c
. First, create an object file from demo.c
:
$ gcc -I ./embody -c src/demo.c
The -I
possibility provides a listing to GCC’s search path for header information. In this instance, I assume that customized header information are in an area listing known as embody
. The -c
possibility prevents GCC from working a linker, as a result of this activity is just to create an object file. And that is precisely what occurs:
$ ls
demo.o embody/ lib/ src/
Now you should use the -L
choice to set a path to your library, and compile:
$ gcc -L`pwd`/lib -o myDemo demo.o -lexample
Notice that the -L
possibility comes earlier than the -l
possibility. This is critical, as a result of if -L
hasn’t been added to GCC’s search path earlier than you inform GCC to search for a non-default library, GCC will not know to look in your customized location. The compilation succeeds as anticipated, however there’s an issue while you try and run it:
$ ./myDemo
./myDemo: error whereas loading shared libraries:
libexample.so: can not open shared object file:
No such file or listing
Troubleshooting with ldd
The ldd
utility prints shared object dependencies, and it may be helpful when troubleshooting points like this:
$ ldd ./myDemo
linux-vdso.so.1 (0x00007ffe151df000)
libexample.so => not discovered
libc.so.6 => /lib64/libc.so.6 (0x00007f514b60a000)
/lib64/ld-linux-x86-64.so.2 (0x00007f514b839000)
You already knew that libexample
could not be situated, however the ldd
output a minimum of affirms what’s anticipated from a working library. For occasion, libc.so.6
has been situated, and ldd
shows its full path.
LD_LIBRARY_PATH
The LD_LIBRARY_PATH
environment variable defines the trail to libraries. If you are working an utility that depends on a library that is not put in to a normal listing, you possibly can add to the system’s library search path utilizing LD_LIBRARY_PATH
.
There are a number of methods to set surroundings variables, however probably the most versatile is to position them earlier than you run a command. Look at what setting LD_LIBRARY_PATH
does for the ldd
command when it is analyzing a “broken” executable:
$ LD_LIBRARY_PATH=`pwd`/lib ldd ./
linux-vdso.so.1 (0x00007ffe515bb000)
libexample.so => /tmp/Demo/lib/libexample.so (0x0000...
libc.so.6 => /lib64/libc.so.6 (0x00007eff037ee000)
/lib64/ld-linux-x86-64.so.2 (0x00007eff03a22000)
It applies simply as properly to your customized command:
$ LD_LIBRARY_PATH=`pwd`/lib myDemo
good day world!
If you progress the library file or the executable, nevertheless, it breaks once more:
$ mv lib/libexample.so ~/.native/lib64
$ LD_LIBRARY_PATH=`pwd`/lib myDemo
./myDemo: error whereas loading shared libraries...
To repair it, it’s essential to regulate the LD_LIBRARY_PATH
to match the library’s new location:
$ LD_LIBRARY_PATH=~/.native/lib64 myDemo
good day world!
When to make use of LD_LIBRARY_PATH
In most instances, LD_LIBRARY_PATH
is not a variable you might want to set. By design, libraries are put in to /usr/lib64
and so purposes naturally search it for his or her required libraries. You may have to make use of LD_LIBRARY_PATH
in two instances:
- You’re compiling software program that should hyperlink towards a library that itself has simply been compiled and has not but been put in. Good construct programs, resembling Autotools and CMake, may help deal with this.
- You’re bundling software program that is designed to expire of a single listing, with no set up script or an set up script that locations libraries in non-standard directories. Several purposes have releases {that a} Linux person can obtain, copy to
/decide
, and run with “no install.” TheLD_PATH_LIBRARY
variable will get set by way of wrapper scripts so the person usually is not even conscious it has been set.
Compiling software program offers you plenty of flexibility in the way you run your system. The LD_LIBRARY_PATH
variable, together with the -L
and -l
GCC choices, are parts of that flexibility.
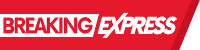