Modern functions require difficult interactions between long-running enterprise processes, inside companies, and third-party APIs. To say it has been a problem for builders is placing it mildly. Managing these processes means monitoring advanced states, making ready responses to asynchronous occasions, and speaking with typically unreliable exterior dependencies.
Developers sometimes tackle these advanced challenges with options which might be simply as convoluted, assembling unwieldy programs that leverage stateless companies, databases, retry algorithms, and job scheduling queues. Because these advanced programs obscure their very own enterprise logic, availability points are frequent, typically stemming from the applying’s dependence on scattered and unproven elements. Developer productiveness is recurrently sacrificed to maintain these sprawling, troubled programs from collapsing.
Designing a distributed utility
Cadence solves these points by providing a extremely scalable fault-oblivious code platform. Cadence abstracts away the standard challenges of implementing fault tolerance and sturdiness with its fault oblivious code.
A typical Cadence utility features a Cadence service, workflow, exercise staff, and exterior shoppers. If wanted, it is acceptable to co-locate the roles of workflow staff, exercise staff, and exterior shoppers in a single utility course of.
Cadence Service
(Ben Slater, CC BY-SA 4.0)
Cadence is centered on its multi-tenant service and the excessive scalability it permits. A strongly typed gRPC API exposes all Cadence service performance. A Cadence cluster can run a number of companies on a number of nodes, together with:
- Front finish: A stateless service that handles incoming employee requests, with situations backed by an exterior load balancer.
- History service: Handles core logic for workflow steps and exercise orchestration.
- Matching service: Matches workflow or exercise duties with staff prepared to finish them.
- Internal employee service: Meets inside necessities (similar to archiving) by introducing Cadence workflows and actions.
- Workers: Function as Cadence shopper apps that execute user-created workflow and exercise logic.
By default, Cadence helps Apache Cassandra, MySQL, PostgreSQL, CockroachDB, and TiDB to be used as persistence shops, in addition to ElasticSearch and OpenSearch for itemizing workflows with advanced predicates.
Because the Cadence service is multi-tenant, a single service can serve one or many functions. An area Cadence service occasion will be configured with docker-compose for native improvement. The Cadence service maintains workflow states, related sturdy timers, and inside “task list” queues to ship duties to exterior staff.
Beyond the Cadence service itself:
-
Workflow staff: hosts fault-oblivious code externally to the Cadence service. The Cadence service sends these staff “decision tasks.” The staff ship the duties to the workflow code and talk the finished “decisions” again to the Cadence service. Workflow code will be carried out in any language capable of talk with Cadence API: production-ready Java and Go shoppers are at present accessible.
-
Activity staff: hosts “activities”, or code that carry out utility particular actions similar to service calls, database document updates, and file downloads. Activities characteristic activity routing to particular processes, heartbeats, infinite retries, and limitless execution time. The Cadence service sends exercise duties to those staff, who full them and report completion.
-
External shoppers: allow the creation of workflow situations, or “executions”. External shoppers similar to UIs, microservices or CLIs use the BeginWorkflowExecution Cadence service API name to implement executions. External shoppers are additionally able to notifying workflows about asynchronous exterior occasions, synchronous workflow state queries, ready for synchronous workflow completion, workflow restarts, cancellation, and looking for particular workflows with List API.
Getting began with Cadence
In this instance we’ll use the Cadence Java shopper. The shopper is available from GitHub, and JavaDoc documentation can be found here. You can even examine for the latest release version.
To start, add cadence-client as a dependency to your pom.xml file like this:
<dependency><groupId>com.uber.cadence</groupId>
<artifactId>cadence-client</artifactId>
<model>LATEST.RELEASE.VERSION</model>
</dependency>
Alternatively, you should utilize construct.gradle:
compile group: ‘com.uber.cadence’, identify: ‘cadence-client’, model: ‘LATEST.RELEASE.VERSION’
Java Hello World with Cadence
The greatest method to get an thought of what Cadence is able to is to strive it, so this is a easy “Hello World” instance you’ll be able to strive. First, add the Cadence Java client dependency to your Java undertaking. Using Gradle, the dependency appears like this:
compile group: ‘com.uber.cadence’, identify: ‘cadence-client’, model: ‘<latest_version>’
Add these dependencies that the cadence-client requires as effectively:
compile group: ‘commons-configuration’, identify: ‘commons-configuration’, model: ‘1.9′
compile group: ‘ch.qos.logback’, identify: ‘logback-classic’, model: ‘1.2.3′
Then compile this code:
import com.uber.cadence.workflow.Workflow;
import com.uber.cadence.workflow.WorkflowTechnique;
import org.slf4j.Logger;
public class GettingStarted {
personal static Logger logger = Workflow.getLogger(GettingStarted.class);
public interface HelloWorld {
@WorkflowTechnique
void sayHello(String identify);
}
}
These Cadence Java samples can be found to assist in the event you encounter points with the construct information.
Next, put this logback config file into your classpath:
<configuration>
<appender identify="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<!-- encoders are assigned the sort
ch.qos.logback.basic.encoder.PatternLayoutEncoder by default -->
<encoder>
<sample>%d{HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n</sample>
</encoder>
</appender>
<logger identify="io.netty" degree="INFO"/>
<root degree="INFO">
<appender-ref ref="STDOUT" />
</root>
</configuration>
Now create the Hello World workflow. Add HelloWorldImpl with the sayHello methodology, which logs and returns “Hello …”:
import com.uber.cadence.employee.Worker;
import com.uber.cadence.workflow.Workflow;
import com.uber.cadence.workflow.WorkflowTechnique;
import org.slf4j.Logger;
public class GettingStarted {
personal static Logger logger = Workflow.getLogger(GettingStarted.class);
public interface HelloWorld {
@WorkflowTechnique
void sayHello(String identify);
}
public static class HelloWorldImpl implements HelloWorld {
@Override
public void sayHello(String identify) {
logger.information("Hello " + identify + "!");
}
}
}
Register the workflow implementation to the Cadence framework with a employee related to a Cadence service. Workers will hook up with a Cadence service operating regionally by default.
public static void principal(String[] args) {
WorkflowShopper workflowClient =
WorkflowShopper.newInstance(
new WorkflowServiceTChannel(ClientOptions.defaultInstance()),
WorkflowClientOptions.newBuilder().setDomain(DOMAIN).construct());
// Get employee to ballot the duty listing.
WorkerManufacturing facility manufacturing facility = WorkerManufacturing facility.newInstance(workflowClient);
Worker employee = manufacturing facility.newWorker(TASK_LIST);
employee.registerWorkflowImplementationTypes(HelloWorldImpl.class);
manufacturing facility.begin();
}
Now you are able to run the employee program. Here’s an instance log:
13:35:02.575 [main] INFO c.u.c.s.WorkflowServiceTChannel – Initialized TChannel for service cadence-frontend, LibraryVersion: 2.2.0, FeatureVersion: 1.0.0
13:35:02.671 [main] INFO c.u.cadence.inside.employee.Poller – begin(): Poller{choices=PollerChoices{maximumPollRateIntervalMilliseconds=1000, maximumPollRatePerSecond=0.0, pollBackoffCoefficient=2.0, pollBackoffInitialInterval=PT0.2S, pollBackoffMaximumInterval=PT20S, pollThreadCount=1, pollThreadNamePrefix=‘Workflow Poller activityList=”HelloWorldTaskList”, area=”test-domain”, sort=”workflow”‘}, id=45937@maxim-C02XD0AAJGH6}
13:35:02.673 [main] INFO c.u.cadence.inside.employee.Poller – begin(): Poller{choices=PollerChoices{maximumPollRateIntervalMilliseconds=1000, maximumPollRatePerSecond=0.0, pollBackoffCoefficient=2.0, pollBackoffInitialInterval=PT0.2S, pollBackoffMaximumInterval=PT20S, pollThreadCount=1, pollThreadNamePrefix=‘null’}, id=81b8d0ac-ff89-47e8-b842-3dd26337feea}
“Hello”‘is not printing, as a result of the employee solely hosts the workflow code. To execute the workflow, begin it with the Cadence CLI:
$ docker run --network=host --rm ubercadence/cli:grasp --do test-domain workflow begin --tasklist HelloWorldActivityList --workflow_type HelloWorld::sayHello --execution_timeout 3600 --input "World"
Started Workflow Id: bcacfabd-9f9a-46ac-9b25-83bcea5d7fd7, run Id: e7c40431-8e23-485b-9649-e8f161219efe
Now this system provides this output:
13:35:02.575 [main] INFO c.u.c.s.WorkflowServiceTChannel – Initialized TChannel for service cadence-frontend, LibraryVersion: 2.2.0, FeatureVersion: 1.0.0
13:35:02.671 [main] INFO c.u.cadence.inside.employee.Poller – begin(): Poller{choices=PollerChoices{maximumPollRateIntervalMilliseconds=1000, maximumPollRatePerSecond=0.0, pollBackoffCoefficient=2.0, pollBackoffInitialInterval=PT0.2S, pollBackoffMaximumInterval=PT20S, pollThreadCount=1, pollThreadNamePrefix=‘Workflow Poller activityList=”HelloWorldTaskList”, area=“test-domain”, sort=”workflow”‘}, id=45937@maxim-C02XD0AAJGH6}
13:35:02.673 [main] INFO c.u.cadence.inside.employee.Poller – begin(): Poller{choices=PollerChoices{maximumPollRateIntervalMilliseconds=1000, maximumPollRatePerSecond=0.0, pollBackoffCoefficient=2.0, pollBackoffInitialInterval=PT0.2S, pollBackoffMaximumInterval=PT20S, pollThreadCount=1, pollThreadNamePrefix=‘null’}, id=81b8d0ac-ff89-47e8-b842-3dd26337feea}
13:40:28.308 [workflow-root] INFO c.u.c.samples.whats up.GettingStarted – Hello World!
Success! Now run this workflow execution:
$ docker run --network=host --rm ubercadence/cli:grasp --do test-domain workflow begin --tasklist HelloWorldActivityList --workflow_type HelloWorld::sayHello --execution_timeout 3600 --input "Cadence"Started Workflow Id: d2083532-9c68-49ab-90e1-d960175377a7, run Id: 331bfa04-834b-45a7-861e-bcb9f6ddae3e
You ought to get this output:
13:35:02.575 [main] INFO c.u.c.s.WorkflowServiceTChannel – Initialized TChannel for service cadence-frontend, LibraryVersion: 2.2.0, FeatureVersion: 1.0.0
13:35:02.671 [main] INFO c.u.cadence.inside.employee.Poller – begin(): Poller{choices=PollerChoices{maximumPollRateIntervalMilliseconds=1000, maximumPollRatePerSecond=0.0, pollBackoffCoefficient=2.0, pollBackoffInitialInterval=PT0.2S, pollBackoffMaximumInterval=PT20S, pollThreadCount=1, pollThreadNamePrefix=‘Workflow Poller activityList=”HelloWorldTaskList”, area=”test-domain”, sort=”workflow”‘}, id=45937@maxim-C02XD0AAJGH6}
13:35:02.673 [main] INFO c.u.cadence.inside.employee.Poller – begin(): Poller{choices=PollerChoices{maximumPollRateIntervalMilliseconds=1000, maximumPollRatePerSecond=0.0, pollBackoffCoefficient=2.0, pollBackoffInitialInterval=PT0.2S, pollBackoffMaximumInterval=PT20S, pollThreadCount=1, pollThreadNamePrefix=‘null’}, id=81b8d0ac-ff89-47e8-b842-3dd26337feea}
13:40:28.308 [workflow-root] INFO c.u.c.samples.whats up.GettingStarted – Hello World!
13:42:34.994 [workflow-root] INFO c.u.c.samples.whats up.GettingStarted – Hello Cadence!
Lastly, use this CLI to listing the workflow:
$ docker run --network=host --rm ubercadence/cli:grasp --do test-domain workflow listingWORKFLOW TYPE | WORKFLOW ID | RUN ID | START TIME | EXECUTION TIME | END TIME
HelloWorld::sayHello | d2083532-9c68-49ab-90e1-d960175377a7 | 331bfa04-834b-45a7-861e-bcb9f6ddae3e | 20:42:34 | 20:42:34 | 20:42:35
HelloWorld::sayHello | bcacfabd-9f9a-46ac-9b25-83bcea5d7fd7 | e7c40431-8e23-485b-9649-e8f161219efe | 20:40:28 | 20:40:28 | 20:40:29
Look over the workflow execution historical past as effectively:
$ docker run --network=host --rm ubercadence/cli:grasp --do test-domain workflow showid 1965109f-607f-4b14-a5f2-24399a7b8fa7
1 WorkflowExecutionStarted {WorkflowSort:{Name:HelloWorld::sayHello},
ActivityList:{Name:HelloWorldActivityList},
Input:["World"],
ExecutionStartToCloseTimeoutSeconds:3600,
TaskStartToCloseTimeoutSeconds:10,
ContinuedFailureDetails:[],
LastCompletionResult:[],
Identity:cadence-cli@linuxkit-025000000001,
Attempt:0,
FirstDecisionTaskBackoffSeconds:0}
2 DecisionTaskScheduled {ActivityList:{Name:HelloWorldActivityList},
BeginToCloseTimeoutSeconds:10,
Attempt:0}
3 DecisionTaskStarted {ScheduledEventId:2,
Identity:45937@maxim-C02XD0AAJGH6,
RequestId:481a14e5-67a4-436e-9a23-7f7fb7f87ef3}
4 DecisionTaskCompleted {ExecutionContext:[],
ScheduledEventId:2,
StartedOccasionId:3,
Identity:45937@maxim-C02XD0AAJGH6}
5 WorkflowExecutionCompleted {Result:[],
DecisionTaskCompletedEventId:4}
It could also be a easy workflow, however wanting on the historical past is sort of informative. The historical past’s worth as a troubleshooting, analytics, and compliance software solely will increase with the complexity of the workflow. As a greatest observe, mechanically archive the historical past to a long-term blob retailer when workflows full.
Try Cadence
Cadence presents transformative benefits for organizations and utility improvement groups charged with creating and managing high-scale distributed functions constructed for prime sturdiness, availability, and scalability. Cadence is out there to all as free and open supply software program, making it easy for groups to discover its capabilities and decide if Cadence is a powerful match for his or her organizations.
Using Cadence is so simple as cloning the Git repository for the Cadence server or the container image. For extra particulars on getting began, go to: https://cadenceworkflow.io/docs/get-started/.
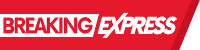