Automatic Garbage Collection (GC) is among the most essential options that makes Java so in style. This article explains why GC is crucial. It consists of computerized and generational GC, how the Java Virtual Machine (JVM) divides heap reminiscence, and eventually, how GC works contained in the JVM.
Java reminiscence allocation
Java reminiscence is split into 4 sections:
- Heap: The reminiscence for object cases is allotted within the heap. When the thing declaration is made, there will not be any reminiscence allotted within the heap. Instead, a reference is created for that object within the stack.
- Stack: This part allocates the reminiscence for strategies, native variables, and sophistication occasion variables.
- Code: Bytecode resides on this part.
- Static: Static knowledge and strategies are positioned on this part.
What is computerized Garbage Collection (GC)?
Automatic GC is a course of wherein the referenced and unreferenced objects in heap reminiscence are recognized, after which unreferenced objects are thought of for deletion. The time period referenced objects means some a part of your program is utilizing these objects. Unreferenced objects will not be at present being utilized by this system.
Programming languages like C and C++ require guide allocation and deallocation of reminiscence. This is routinely dealt with by GC in Java, though you’ll be able to set off GC manually with the system.gc();
name in your code.
The basic steps of GC are:
1. Mark used and unused objects
In this step, the used and unused objects are marked individually. This is a time-consuming course of, as all objects in reminiscence should be scanned to find out whether or not they’re in use or not.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
2. Sweep/Delete objects
There are two variations of sweep and delete.
Simple deletion: Only unreferenced objects are eliminated. However, the reminiscence allocation for brand spanking new objects turns into troublesome because the free area is scattered throughout accessible reminiscence.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
Deletion with compaction: Apart from deleting unreferenced objects, referenced objects are compacted. Memory allocation for brand spanking new objects is comparatively simple, and reminiscence allocation efficiency is improved.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
What is generational Garbage Collection (GC), and why is it wanted?
As seen within the sweep and delete mannequin, scanning all objects for reminiscence reclamation from unused objects turns into troublesome as soon as the objects continue to grow. An experimental research exhibits that almost all objects created throughout this system execution are short-lived.
The existence of short-lived objects can be utilized to enhance the efficiency of GC. For that, the JVM divides the reminiscence into totally different generations. Next, it categorizes the objects primarily based on these reminiscence generations and performs the GC accordingly. This method is called generational GC.
Heap reminiscence generations and the generational Garbage Collection (GC) course of
To enhance the efficiency of the GC mark and sweep steps, the JVM divides the heap reminiscence into three generations:
- Young Generation
- Old Generation
- Permanent Generation
(Jayashree Huttanagoudar, CC BY-SA 4.0)
Here is an outline of every era and its key options.
Young Generation
All created objects are current right here. The younger era is additional divided into:
- Eden: All newly created objects are allotted with the reminiscence right here.
- Survivor area (S0 and S1): After surviving one GC, the dwell objects are moved to considered one of these survivor areas.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
The generational GC that occurs within the Young Generation is called Minor GC. All Minor GC cycles are “Stop the World” occasions that trigger the opposite functions to pause till it completes the GC cycle. This is why Minor GC cycles are quicker.
To summarize: Eden area has all newly created objects. Once Eden is full, the primary Minor GC cycle is triggered.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
Minor GC: The dwell and lifeless objects are marked throughout this cycle. The dwell objects are moved to survivor area S0. Once all dwell objects are moved to S0, the unreferenced objects are deleted.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
The age of objects in S0 is 1 as a result of they’ve survived one Minor GC. Now Eden and S1 are empty.
Once cleared, the Eden area is once more full of new dwell objects. As time elapses, some objects in Eden and S0 change into lifeless (unreferenced), and Eden’s area is full once more, triggering the Minor GC.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
This time the lifeless and dwell objects in Eden and S0 are marked. The dwell objects from Eden are moved to S1 with an age increment of 1. The dwell objects from S0 are additionally moved to S1 with an age increment of two (as a result of they’ve now survived two Minor GCs). At this level, S0 and Eden are empty. After each Minor GC, Eden and one of many survivor areas are empty.
The similar cycle of making new objects in Eden continues. When the following Minor GC happens, Eden and S1 are cleared by transferring the aged objects to S0. The survivor areas change after each Minor GC.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
This course of continues till the age of one of many surviving objects reaches a sure threshold, at which level it’s moved to the so-called the Old Generation with a course of known as promotion.
Further, the -Xmn
flag units the Young Generation measurement.
Old Generation (Tenured Generation)
This era accommodates the objects which have survived a number of Minor GCs and aged to achieve an anticipated threshold.
(Jayashree Huttanagoudar, CC BY-SA 4.0)
In the instance diagram above, the brink is 8. The GC within the Old Generation is called a Major GC. Use the flags -Xms
and -Xmx
to set the preliminary and most measurement of the heap reminiscence.
Permanent Generation
The Permanent Generation area shops metadata associated to library lessons and strategies of an software, J2SE, and what’s in use by the JVM itself. The JVM populates this knowledge at runtime primarily based on which lessons and strategies are in use. Once the JVM finds the unused lessons, they’re unloaded or collected, making area for used lessons.
Use the flags -XX:PermGen
and -XX:MaxPermGen
to set the preliminary and most measurement of the Permanent Generation.
Metaspace
Metaspace was launched in Java 8u and changed PermGen. The benefit of that is computerized resizing, which avoids OutOfMemory errors.
Wrap up
This article discusses the varied reminiscence generations of JVM and the way they’re useful for computerized generational Garbage Collection (GC). Understanding how Java handles reminiscence is not all the time vital, however it will possibly make it easier to envision how the JVM offers together with your variables and sophistication cases. This understanding means that you can plan and troubleshoot your code and comprehend potential limitations inherent in a selected platform.
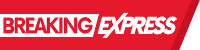