In my earlier article about rustup, I confirmed you find out how to set up the Rust toolchain. Well, what good is the toolchain should you received’t be utilizing it to get extra hands-on with Rust? Learning any language entails studying present code and writing a number of pattern applications. That’s a great way to grow to be proficient in a language. However, there is a third method: debugging code.
Learning via debugging entails attempting to compile a pre-written (buggy) pattern program, understanding the errors generated by the compiler, fixing the pattern code, after which re-compiling it. Repeat that course of till the code efficiently compiles and runs.
Rustlings is an open supply mission by the Rust crew that helps you be taught Rust via the method of debugging. It additionally offers you with a number of hints alongside the best way. If you are a newbie to Rust and have both began or accomplished studying the Rust e-book, then rustlings is the perfect subsequent step. Rustlings helps you apply what you have realized from the e-book, and transfer to engaged on greater tasks.
Installing rustlings
I’m utilizing (and suggest) a Fedora machine to strive rustlings, however any Linux distribution works. To set up rustlings, it’s essential to obtain and run its set up script. It’s really helpful that you simply do that as a standard person (not root) with none particular privileges.
Remember, for you to have the ability to use rustlings, you want the Rust toolchain out there in your system. If you do not have that already, please check with my article on rustup.
Once you are prepared, obtain the set up script:
$ curl -L https://uncooked.githubusercontent.com/rust-lang/rustlings/predominant/set up.sh > rustlings_install.sh
$ file rustlings_install.sh
rustlings_install.sh: Bourne-Again shell script, ASCII textual content executable
Inspect the script to be taught what it does:
$ much less rustlings_install.sh
And then run it to put in:
$ bash rustlings_install.sh
[...]
Installing /house/tux/.cargo/bin/rustlings
Installed package deal `rustlings v4.8.0 (/house/tux/rustlings)` (executable `rustlings`)
All accomplished!
Run ‘rustlings’ to get began.
Rustlings workouts
The set up offers you with the rustlings
command. Run it together with the --help
flag to see what choices can be found.
$ rustlings --help
The set up script additionally clones the rustlings Git repository, and installs all of the dependencies required to run the pattern applications. You can view the pattern applications throughout the workouts listing beneath rustlings
:
$ cd rustlings
$ pwd
/house/tux/rustlings
$ ls
AUTHORS.md Cargo.toml CONTRIBUTING.md data.toml set up.sh README.md goal Cargo.lock CHANGELOG.md workouts set up.ps1 LICENSE src exams
$ ls -m workouts/
advanced_errors, clippy, collections, conversions, enums, error_handling, features, generics, if, intro, macros, mod.rs,
modules, move_semantics, choice, primitive_types, quiz1.rs, quiz2.rs, quiz3.rs, quiz4.rs, README.md,
standard_library_types, strings, structs, exams, threads, traits, variables
List all workouts from the command line
The rustlings
command offers you with a listing
command which shows every pattern program, its full path, and the standing (which defaults to pending.)
$ rustlings listing
Name Path Status
intro1 workouts/intro/intro1.rs Pending
intro2 workouts/intro/intro2.rs Pending
variables1 workouts/variables/variables1.rs Pending
variables2 workouts/variables/variables2.rs Pending
variables3 workouts/variables/variables3.rs Pending
[...]
Near the tip of the output, you are given a progress report so you possibly can monitor your work.
Progress: You accomplished 0 / 84 workouts (0.00 %).
View pattern applications
The rustings listing
command reveals you what applications can be found, so at any time you possibly can view the code of these applications by copying the whole paths into your terminal as an argument for the cat or less instructions:
$ cat workouts/intro/intro1.rs
Verify your applications
Now you can begin debugging applications. You can try this utilizing the confirm
command. Notice that rustlings chooses the primary program within the listing (intro1.rs
), tries to compile it, and succeeds:
$ rustlings confirm
Progress: [-----------------------------------] 0/84
✅ Successfully ran workouts/intro/intro1.rs!You can hold engaged on this train,
or bounce into the following one by eradicating the `I AM NOT DONE` remark:6 | // Execute the command `rustlings trace intro1` for a touch.
7 |
8 | // I AM NOT DONE
9 |
As you possibly can see from the output, despite the fact that the pattern code compiles, there’s work but to be accomplished. Each pattern program has the next remark in its supply file:
$ grep "NOT DONE" workouts/intro/intro1.rs
// I AM NOT DONE
Although the compilation of the primary program labored effective, rustlings will not transfer to the following program till you take away the I AM NOT DONE
remark.
Moving to the following train
Once you might have eliminated the remark from intro1.rs
, you possibly can transfer to the following train by operating the rustlings confirm
command once more. This time, you might discover that rustlings tries to compile the following program (intro2.rs
) within the collection, however runs into an error. You’re anticipated to debug that problem, repair it, after which transfer ahead. This is a crucial step, permitting you to grasp why Rust says a program has bugs.
$ rustlings confirm
Progress: [>------------------------] 1/84
⚠️ Compiling of workouts/intro/intro2.rs failed! Please strive once more. Here's the output:
error: 1 positional argument in format string, however no arguments got
--> workouts/intro/intro2.rs:8:21
|
8 | println!("Hello {}!");
| ^^error: aborting as a result of earlier error
Getting a touch
Rustlings has a helpful trace
argument, which tells you precisely what’s fallacious with the pattern program, and find out how to repair it. You can consider it as an add-on assist choice, along with what the compiler error message tells you.
$ rustlings trace intro2
Add an argument after the format string.
Based on the above trace, fixing this system is simple. All it’s good to do is add a further argument to the println
assertion. This diff ought to enable you to perceive the modifications:
< println!("Hello {}!", "world");
---
> println!("Hello {}!");
Once you make that change, and eliminated the NOT DONE
remark from the supply code, you possibly can run rustlings confirm
once more to compile and run the code.
$ rustlings confirm
Progress: [>-------------------------------------] 1/84
✅ Successfully ran workouts/intro/intro2.rs!
Track progress
You aren’t going to complete all the workouts in a day, and it is easy to lose monitor of the place you left off. You can run the listing
command to see the standing of your work.
$ rustlings listing
Name Path Status
intro1 workouts/intro/intro1.rs Done
intro2 workouts/intro/intro2.rs Done
variables1 workouts/variables/variables1.rs Pending
variables2 workouts/variables/variables2.rs Pending
variables3 workouts/variables/variables3.rs Pending
[...]
Run particular workouts
If you do not wish to begin from the start and skip just a few workouts, rustlings permits you to deal with particular workouts utilizing the rustlings run
command. This runs a selected program with out requiring the earlier lesson to be verified. For instance:
$ rustlings run intro2
Hello world!
✅ Successfully ran workouts/intro/intro2.rs
$ rustlings run variables1
Typing train names can grow to be tedious, however rustlings offers you with a helpful subsequent
command so you possibly can transfer to the following train within the collection.
$ rustlings run subsequent
Alternative watch command
If you do not wish to hold typing confirm
after every modification you make, you possibly can run the watch
command in a terminal window, after which hold modifying the supply code to repair points. The watch
command detects these modifications, and retains re-compiling this system to see whether or not the problem has been fastened.
$ rustlings watch
Learn by debugging
The Rust compiler is understood to offer very significant error messages, which helps you perceive points in your code. This normally results in sooner debugging. Rustlings is a good way to follow Rust, to get used to studying error messages, and perceive the Rust language. Check out the current options of Rustlings 5.0.0 on GitHub.
[ Practice programming with Rust. Download our Rust cheat sheet. ]
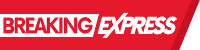